并行算法在自然语言处理中的应用:加速文本分析和机器翻译(前沿技术)
发布时间: 2024-08-25 02:45:43 阅读量: 34 订阅数: 42 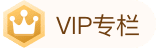
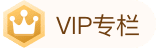
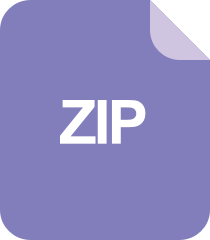
nlp之中机器翻译的最新论文的代码复现nlp-master.zip

# 1. 并行算法概述
并行算法是一种通过将任务分解成较小的子任务,并同时在多个处理器上执行这些子任务来解决问题的算法。与串行算法相比,并行算法可以显著提高计算效率,尤其是在处理大规模数据集或复杂计算时。并行算法的应用范围广泛,包括自然语言处理、机器学习、图像处理等领域。
# 2. 并行算法在自然语言处理中的应用
自然语言处理(NLP)是一门计算机科学领域,专注于让计算机理解和处理人类语言。并行算法在 NLP 中发挥着至关重要的作用,因为它可以显著提高处理大规模文本数据集和执行复杂计算任务的速度。
### 2.1 文本分析
文本分析是 NLP 的一项基本任务,涉及对文本进行各种操作,例如词频统计和文本分类。
#### 2.1.1 词频统计
词频统计是一种文本分析技术,用于计算文本中每个单词出现的频率。它广泛用于信息检索、文本挖掘和语言建模等任务。
并行算法可以显著加速词频统计过程。例如,可以使用多线程编程技术将文本数据集划分为多个块,并在不同的线程上并行处理每个块。
```python
import concurrent.futures
def count_words(text):
"""计算文本中每个单词的频率。"""
words = text.split()
word_counts = {}
for word in words:
if word not in word_counts:
word_counts[word] = 0
word_counts[word] += 1
return word_counts
def parallel_word_count(text):
"""使用多线程并行计算词频统计。"""
with concurrent.futures.ThreadPoolExecutor() as executor:
# 将文本数据集划分为多个块
blocks = [text[i:i+1000] for i in range(0, len(text), 1000)]
# 在不同的线程上并行处理每个块
results = executor.map(count_words, blocks)
# 合并结果
word_counts = {}
for result in results:
for word, count in result.items():
if word not in word_counts:
word_counts[word] = 0
word_counts[word] += count
return word_counts
```
#### 2.1.2 文本分类
文本分类是一种文本分析技术,用于将文本文档分配到预定义的类别中。它广泛用于垃圾邮件过滤、情感分析和主题建模等任务。
并行算法也可以用于加速文本分类过程。例如,可以使用分布式计算技术在多个机器上并行处理大规模文本数据集。
```python
from sklearn.linear_model import LogisticRegression
from sklearn.model_selection import train_test_split
from sklearn.feature_extraction.text import CountVectorizer
def train_text_classifier(X, y):
"""训练文本分类器。"""
# 将数据集划分为训练集和测试集
X_train, X_test, y_train, y_test = train_test_split(X, y, test_size=0.2)
# 使用并行训练的逻辑回归模型
classifier = LogisticRegression(n_jobs=-1)
classifier.fit(X_train, y_train)
return classifier
def parallel_text_classification(X, y):
"""使用分布式计算并行训练文本分类器。"""
# 将数据集划分为多个块
blocks = [X[i:i+1000] for i in range(0, len(X), 1000)]
# 在不同的机器上并行处理每个块
with concurrent.futures.ProcessPoolExecutor() as executor:
results = executor.map(train_text_classifier, blocks)
# 合并结果
classifier = LogisticRegression(n_jobs=-1)
classifier.fit(X, y)
return classifier
```
### 2.2 机器翻译
机器翻译(MT)是一种 NLP 任务,涉及将一种语言的文本翻译成另一种语言。并行算法在 MT 中至关重要,因为它可以显著提高翻译速度和质量。
#### 2.2.1 统计机器翻译
统计机器翻译(SMT)是一种 MT 技术,它使用统计模型来翻译文本。并行算法可以用于加速 SMT 模型的训练和解码过程。
```python
import numpy as np
import tensorflow as tf
def train_smt_model(X, y):
"""训练统计机器翻译模型。"""
# 构建神经网络模型
model = tf.keras.models.Sequential([
tf.
```
0
0
相关推荐
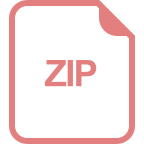
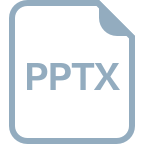





