Spring Cloud OpenFeign的高级配置:重试机制与自定义处理策略
发布时间: 2023-12-19 22:45:12 阅读量: 10 订阅数: 23 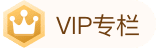
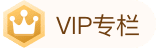
# 1. 理解Spring Cloud OpenFeign的重试机制
## 1.1 介绍Spring Cloud OpenFeign的重试机制
Spring Cloud OpenFeign是一个基于Netflix Feign实现的声明式、模板化的HTTP客户端,它简化了使用Spring Cloud的服务间调用。在实际应用中,网络不稳定或者服务负载过大导致部分请求失败是很常见的。Spring Cloud OpenFeign提供了重试机制来增加网络请求的可靠性。在本节中,我们将学习Spring Cloud OpenFeign的重试机制,以及如何配置和使用它来提高服务调用的稳定性。
## 1.2 如何配置Feign的重试机制
在Spring Cloud OpenFeign中,我们可以通过配置来启用和定制Feign的重试机制。通过设置Feign的相关属性,我们可以控制重试的次数、间隔、以及对异常的过滤等。本节将介绍如何通过配置来启用和定制Feign的重试机制,以及各项配置属性的含义和作用。
## 1.3 示例:在Spring Cloud项目中使用Feign的重试机制
在本节中,我们将以一个实际的Spring Cloud项目为例,演示如何在项目中使用Feign的重试机制。我们将创建一个简单的服务间调用场景,并展示如何配置Feign的重试属性,并观察调用结果。通过这个实例,读者能够更加直观地理解Feign的重试机制以及如何在项目中应用它。
# 2. 定制Feign的重试策略
在使用Spring Cloud OpenFeign时,我们可以通过定制Feign的重试策略来满足特定的需求。本章节将介绍可用的重试策略选项,以及如何自定义Feign的重试策略。
### 2.1 可用的重试策略选项
在默认情况下,Spring Cloud OpenFeign使用的是`Retryer.Default`作为重试策略。该重试策略会在发生请求失败时进行重试,重试的次数取决于配置的最大重试次数,默认值为5次。重试间隔时间按指数级递增,并且具有一个随机的抖动因子,以避免请求的同时发送。
除了默认的重试策略外,Feign还提供了以下可用的重试策略选项:
- `Retryer.NEVER_RETRY`:不进行任何重试,请求失败后立即抛出异常。
- `Retryer.Once`:最多进行一次重试。
- `Retryer.Retriable`:最多进行指定的重试次数。
### 2.2 如何自定义Feign的重试策略
要自定义Feign的重试策略,我们需要实现`Retryer`接口,并根据自己的需求实现其中的方法。下面是一个自定义重试策略的示例:
```java
import feign.RetryableException;
import feign.Retryer;
public class CustomRetryer implements Retryer {
private final int maxAttempts;
private final long backoff;
private int attempt;
public CustomRetryer(int maxAttempts, long backoff) {
this.maxAttempts = maxAttempts;
this.backoff = backoff;
this.attempt = 1;
}
@Override
public void continueOrPropagate(RetryableException e) {
if (attempt++ < maxAttempts) {
try {
Thread.sleep(backoff);
} catch (InterruptedException ignored) {
Thread.currentThread().interrupt();
}
throw e;
}
throw e;
}
@Override
public Retryer clone() {
return new CustomRetryer(maxAttempts, backoff);
}
}
```
在上述示例中,我们实现了`Retryer`接口,并重写了`continueOrPropagate`和`clone`方法。其中,`continueOrPropagate`方法会判断是否继续重试请求,如果重试次数不超过设定的最大次数,会进行一定时间的休眠后再次抛出`RetryableException`异常,以实现重试。`clone`方法用于克隆重试器以供复用。
### 2.3 示例:实现自定义的Feign重试策略
下面通过一个示例来演示如何实现自定义的Feign重试策略。
首先,定义一个Feign客户端接口:
```java
import org.springframework.cloud.openfeign.FeignClient;
import org.springframework.web.bind.annotation.GetMapping;
@FeignClient(name = "example", url = "http://localhost:8080")
public interface ExampleClient {
@GetMapping("/api/example")
String getExampleData();
}
```
然后,在Spring Boot应用中配置Feign以使用自定义的重试策略:
```java
import feign.Retryer;
import org.springframework.cloud.openfeign.EnableFeignClients;
import org.springframework.context.annotation.Bean;
import org.springframework.context.annotation.Configuration;
@Configuration
@EnableFeignClients
public class FeignConfig {
@Bean
public Retryer retryer() {
return new CustomRetryer(3, 1000);
}
}
```
最后,在Spring Boot应用的控制器中使用Feign客户端进行请求调用:
```java
import org.springframework.web.bind.annotation.GetMapping;
import org.springframework.web.bind.annotation.RestController;
@RestController
public class ExampleController {
private final ExampleClient exampleClient;
public ExampleController(ExampleClient exampleClient)
```
0
0
相关推荐
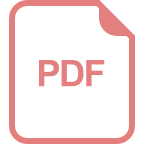
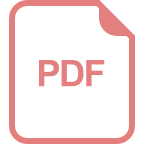





