Spring Boot中的国际化与本地化处理
发布时间: 2024-02-22 11:58:06 阅读量: 43 订阅数: 24 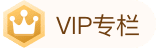
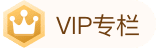
# 1. Spring Boot国际化与本地化简介
国际化与本地化是在开发多语言应用程序中不可或缺的部分。在Spring Boot中,处理国际化与本地化是非常重要和实用的话题。本章将介绍Spring Boot国际化与本地化的基本概念和重要性,以及Spring Boot如何支持这些功能。
## 1.1 什么是国际化与本地化
国际化(Internationalization)是指使软件能够适应不同语言、地区、文化背景的过程。而本地化(Localization)是指根据用户所在的地区或语言环境,将软件的界面、信息等进行相应的翻译和调整,以便用户更好地理解和使用软件。
## 1.2 国际化与本地化在Spring Boot中的作用和重要性
在一个全球化的世界中,开发多语言应用程序是非常有必要的。Spring Boot作为一个流行的Java开发框架,能够很好地支持国际化与本地化,使得开发人员能够轻松地处理多语言环境下的应用开发,提升用户体验。
## 1.3 Spring Boot如何支持国际化与本地化
Spring Boot提供了丰富的国际化与本地化支持,包括配置国际化信息、处理文本信息、日期时间处理、错误信息处理等功能。开发者可以通过配置属性文件、数据库或者其他方式来管理多语言资源,以及在代码中处理不同语言环境下的文本、日期、时间格式等。通过合理地利用Spring Boot的国际化与本地化功能,可以更好地满足用户的不同需求。
# 2. 在Spring Boot中配置国际化信息
国际化信息在Spring Boot应用程序中起着至关重要的作用。通过合适的配置,我们可以轻松实现应用程序的多语言支持。在本章节中,我们将介绍在Spring Boot中如何配置国际化信息的几种方式。
### 2.1 属性文件方式配置国际化信息
在Spring Boot中,我们可以通过属性文件的方式来配置国际化信息。这种方式简单直观,适用于大多数场景。下面是一个示例:
**application.properties**
```properties
spring.messages.basename=messages
```
**messages.properties**
```properties
greeting.message=Hello, World!
```
**Java 代码示例**
```java
import org.springframework.beans.factory.annotation.Value;
import org.springframework.context.MessageSource;
import org.springframework.context.i18n.LocaleContextHolder;
import org.springframework.stereotype.Component;
@Component
public class MessageUtil {
@Value("${greeting.message}")
private String greetingMessage;
private final MessageSource messageSource;
public MessageUtil(MessageSource messageSource) {
this.messageSource = messageSource;
}
public String getMessage(String key) {
return messageSource.getMessage(key, null, LocaleContextHolder.getLocale());
}
public String getGreetingMessage() {
return greetingMessage;
}
}
```
**代码解析**:
- 我们在`application.properties`中指定了基本名称为`messages`,这意味着Spring Boot会查找`messages.properties`来加载国际化信息。
- 在`messages.properties`中定义了一个`greeting.message`的属性,表示我们要国际化的问候语。
- 在`MessageUtil`类中通过`MessageSource`来获取国际化信息,同时也演示了如何直接读取属性文件中的值。
**结果说明**:通过以上配置和代码,可以实现在Spring Boot应用中获取并显示国际化的问候信息。
### 2.2 数据库方式配置国际化信息
除了属性文件外,我们还可以将国际化信息存储在数据库中,这种方式更灵活,适用于需要动态管理国际化内容的场景。
在实际应用中,我们可以创建一个`message`表,包含`locale`, `key`和`value`字段来存储多语言信息。接下来,我们演示一个简化的示例:
**数据库表结构**
```sql
CREATE TABLE message (
id INT AUTO_INCREMENT PRIMARY KEY,
locale VARCHAR(10) NOT NULL,
message_key VARCHAR(50) NOT NULL,
message_value VARCHAR(255) NOT NULL
);
```
**Java 代码示例**
```java
import org.springframework.jdbc.core.JdbcTemplate;
import org.springframework.stereotype.Component;
@Component
public class DBMessageUtil {
private final JdbcTemplate jdbcTemplate;
public DBMessageUtil(JdbcTemplate jdbcTemplate) {
this.jdbcTemplate = jdbcTemplate;
}
public String getMessage(String locale, String key) {
String query = "SELECT message_value FROM message WHERE locale = ? AND message_key = ?";
return jdbcTemplate.queryForObject(query, String.class, locale, key);
}
}
```
**代码解析**:
- 我们创建了一个`message`表用于存储国际化信息。
- 在`DBMessageUtil`类中,通过`JdbcTemplate`来执行数据库查询获取对应的国际化信息。
**结果说明**:通过这种方式,我们可以动态管理国际化信息,灵活地支持多语言内容的配置。
### 2.3 Spring Boot中多语言资源文件的命名规范
在Spring Boot中,多语言资源文件的命名规范对于国际化的正常运行至关重要。通常,我们可以按照以下规则来命名多语言资源文件:
- `messages.properties`: 默认的国际化文件,包含通用性的国际化信息。
- `messages_en.properties`: 英文版本的国际化文件。
- `messages_zh.properties`: 中文版本的国际化文件。
- 其他语言版本的文件按照`messages_语言代码.properties`的格式命名。
这样的命名规范可以让Spring Boot根据不同的`Locale`来加载对应的国际化信息,实现多语言支持。
在接下来的章节中,我们将继续探讨在Spring Boot应用中如何处理文本信息的国际化,欢迎继续阅读。
# 3. 在Spring Boot中的文本信息本地化
在Spring Boot应用程序中,国际化不仅限于处理文本信息,也包括处理日期、时间、货币等。本章将重点介绍如何在Spring Boot中处理文本信息的国际化。
#### 3.1 如何在代码中处理国际化文本信息
在Spring Boot中,我们可以使用MessageSource类来加载不同语言下的文本资源文件,并根据不同的Locale来获取相应的文本信息。
```java
import org.springframework.context.MessageSource;
import org.springframework.context.i18n.LocaleContextHolder;
import org.springframework.stereotype.Controller;
import org.springframework.web.bind.annotation.GetMapping;
@Controller
public class HelloController {
private final MessageSource messageSource;
public HelloController(MessageSource messageSource) {
this.messageSource = messageSource;
}
@GetMapping("/hello")
public String hello() {
String message = messageSource.getMessage("hello.message", null, LocaleContextHolder.getLocale());
return message;
}
}
```
在上面的示例中,我们通过MessageSource类获取名为"hello.message"的文本信息,并根据当前的Locale来获取对应语言的文本。
#### 3.2 消息源和消息解析器的配置和使用
在Spring Boot中,我们需要配置消息源和消息解析器来处理国际化的文本信息。
```java
import org.springframework.context.MessageSource;
import org.springframework.context.annotation.Bean;
import org.springframework.context.annotation.Configuration;
import org.springframework.context.support.ReloadableResourceBundleMessageSource;
@Configuration
public class MessageConfig {
@Bean
public MessageSource messageSource() {
ReloadableResourceBundleMessageSource messageSource = new ReloadableResourceBundleMessageSource();
messageSource.setBasename("classpath:messages");
messageSource.setDefaultEncoding("UTF-8");
return messageSource;
}
}
```
在上面的配置中,我们定义了一个MessageSource Bean,并指定了资源文件的基本名称和编码方式。
#### 3.3 在HTML页面中的文本信息国际化处理
除了在后端Java代码中处理国际化文本信息外,我们还可以在HTML页面中使用Thymeleaf模板引擎来实现文本信息的国际化。
```html
<!DOCTYPE html>
<html xmlns:th="http://www.thymeleaf.org">
<head>
<title th:text="#{app.title}">Spring Boot App</title>
</head>
<body>
<h1 th:text="#{hello.message}">Hello, World!</h1>
</body>
</html>
```
在上面的HTML示例中,我们使用Thymeleaf的`th:text`属性来指定文本信息的key,Thymeleaf会根据不同的Locale自动加载对应语言的文本信息。
通过以上方式,我们可以实现在Spring Boot应用中对文本信息进行国际化处理,让我们的应用更具可扩展性和用户友好性。
# 4. Spring Boot中日期、时间和货币的本地化处理
在实际的应用中,对于日期、时间和货币等信息的本地化处理也是非常重要的。Spring Boot提供了丰富的工具和方法来帮助我们进行这些本地化的操作,下面将介绍相关的内容。
### 4.1 日期和时间的本地化格式化与解析
在Spring Boot中,我们可以使用`@DateTimeFormat`和`@JsonFormat`注解来对日期和时间进行本地化格式化处理。例如,我们可以使用`@DateTimeFormat`注解对接收到的日期进行格式化,或者使用`@JsonFormat`注解在序列化JSON时对日期进行格式化。
```java
@RestController
public class DateController {
@GetMapping("/date")
public String formatDate(@DateTimeFormat(pattern = "yyyy-MM-dd HH:mm:ss") Date date) {
return "Formatted Date: " + date;
}
}
```
### 4.2 货币的本地化格式化与解析
对于货币信息的本地化处理,我们可以使用`NumberFormat`类来实现货币格式化与解析。通过`NumberFormat.getCurrencyInstance()`方法可以获取当前地区的货币格式化实例,进而对货币金额进行格式化操作。
```java
public class CurrencyExample {
public static void main(String[] args) {
double amount = 1234.56;
Locale locale = Locale.US;
NumberFormat currencyFormat = NumberFormat.getCurrencyInstance(locale);
String formattedCurrency = currencyFormat.format(amount);
System.out.println("Formatted Currency: " + formattedCurrency);
}
}
```
### 4.3 时区处理与本地化
在处理国际化信息时,时区也是一个需要考虑的因素。Spring Boot提供了`@DateTimeFormat`注解的`iso`属性来支持时区的处理,同时在配置文件中可以设置`spring.jackson.time-zone`来指定默认的时区。
```java
@RestController
public class TimeZoneController {
@JsonFormat(pattern = "yyyy-MM-dd HH:mm:ss", timezone = "Asia/Shanghai")
@GetMapping("/timezone")
public Date getTimeWithTimeZone() {
return new Date();
}
}
```
通过以上的方式,我们可以在Spring Boot应用中方便地进行日期、时间和货币的本地化处理,保证在不同地区的用户都能够获得符合本地习惯的信息显示。
# 5. 在Spring Boot中处理多国家/地区的错误信息
在实际项目中,经常需要处理多国家/地区的错误信息,这就需要对错误信息进行国际化处理,以便在不同的语言环境下给用户提供友好的错误提示信息。在Spring Boot中,我们可以通过以下方式来处理多国家/地区的错误信息:
#### 5.1 错误信息的国际化配置
首先,我们需要在项目中准备好各国家/地区对应的错误信息资源文件,例如`messages_en.properties`、`messages_zh_CN.properties`等。然后,通过Spring Boot的国际化配置,指定错误消息的基础名称和默认语言,以及支持的其他语言。以下是一个简单的配置示例:
```java
@Configuration
public class ErrorConfig implements WebMvcConfigurer {
@Bean
public LocaleResolver localeResolver() {
SessionLocaleResolver slr = new SessionLocaleResolver();
slr.setDefaultLocale(Locale.US); // 设置默认语言
return slr;
}
@Bean
public MessageSource messageSource() {
ReloadableResourceBundleMessageSource messageSource = new ReloadableResourceBundleMessageSource();
messageSource.setBasename("classpath:messages");
messageSource.setDefaultEncoding("UTF-8");
messageSource.setCacheSeconds(60);
return messageSource;
}
@Override
public void addInterceptors(InterceptorRegistry registry) {
LocaleChangeInterceptor localeChangeInterceptor = new LocaleChangeInterceptor();
localeChangeInterceptor.setParamName("lang");
registry.addInterceptor(localeChangeInterceptor);
}
}
```
在上述代码中,我们配置了默认的语言为英语(Locale.US),并指定了消息资源文件的基础名称为`messages`,Spring Boot会根据请求中的语言参数(比如`lang=en`或`lang=zh_CN`)来自动加载对应的错误消息资源文件。
#### 5.2 自定义错误信息的本地化处理
除了使用默认的错误消息资源文件外,我们也可以在代码中根据具体情况自定义错误信息的国际化处理。比如,在控制器中手动获取错误消息并进行本地化处理:
```java
@Controller
public class MyController {
@Autowired
private MessageSource messageSource;
@GetMapping("/handleError")
public String handleError(Locale locale, Model model) {
String errorMessage = messageSource.getMessage("error.message", null, locale);
model.addAttribute("errorMessage", errorMessage);
return "errorPage";
}
}
```
在上面的代码中,我们通过`MessageSource`来获取指定错误消息的本地化文本,并将其传递到视图中展示给用户。
#### 5.3 在RESTful API中处理国际化错误信息
在RESTful API中,我们也需要对错误信息进行国际化处理。通常可以通过`@ControllerAdvice`注解和`MessageSource`来实现全局的错误信息国际化,例如:
```java
@ControllerAdvice
public class RestResponseEntityExceptionHandler extends ResponseEntityExceptionHandler {
@Autowired
private MessageSource messageSource;
@ExceptionHandler(value = { MyException.class })
protected ResponseEntity<Object> handleMyException(MyException ex, WebRequest request, Locale locale) {
String errorMessage = messageSource.getMessage("error.message", null, locale);
ErrorDetails errorDetails = new ErrorDetails(new Date(), errorMessage, request.getDescription(false));
return new ResponseEntity<>(errorDetails, HttpStatus.BAD_REQUEST);
}
}
```
在上述代码中,我们通过`MessageSource`获取错误消息的本地化文本,并封装成`ErrorDetails`返回给API调用者。
通过以上几种方式,我们可以灵活地处理多国家/地区的错误信息,为用户提供友好的错误提示,从而增强项目的国际化能力。
# 6. 在Spring Boot中的国际化与本地化实际应用
在本章中,我们将通过实例演示来展示在Spring Boot中如何应用国际化与本地化处理,让读者更加深入地了解这些技术的实际应用场景。
**6.1 实例演示:Spring Boot Web应用的国际化与本地化**
在这个实例中,我们将演示如何在一个Spring Boot Web应用中实现国际化与本地化。我们会展示如何配置国际化资源文件、在HTML页面中引用国际化文本信息等。
```java
// 配置国际化资源文件
@Configuration
public class MessageConfig {
@Bean
public MessageSource messageSource() {
ResourceBundleMessageSource messageSource = new ResourceBundleMessageSource();
messageSource.setBasename("messages");
messageSource.setDefaultEncoding("UTF-8");
return messageSource;
}
}
// 控制器代码
@Controller
public class HelloController {
@Autowired
private MessageSource messageSource;
@GetMapping("/")
public String hello(Model model, Locale locale) {
String greeting = messageSource.getMessage("greeting", null, locale);
model.addAttribute("greeting", greeting);
return "hello";
}
}
```
**6.2 实例演示:Spring Boot数据处理中的国际化问题**
在这个实例中,我们将展示如何处理Spring Boot应用中数据的国际化问题,包括日期、时间和货币的本地化处理。
```java
// 货币本地化处理
NumberFormat currencyFormat = NumberFormat.getCurrencyInstance(locale);
String formattedCurrency = currencyFormat.format(1000.50);
System.out.println("Formatted Currency: " + formattedCurrency);
// 日期本地化处理
DateTimeFormatter dateFormatter =
DateTimeFormatter.ofLocalizedDate(FormatStyle.MEDIUM).withLocale(locale);
String formattedDate = dateFormatter.format(LocalDate.now());
System.out.println("Formatted Date: " + formattedDate);
```
**6.3 实例演示:Spring Boot中的国际化与本地化最佳实践**
在这个实例中,我们将分享一些在实际项目中使用Spring Boot进行国际化与本地化的最佳实践,包括如何优化国际化资源文件的管理、如何处理多语言错误信息等。
```java
// 多语言错误信息处理
@ResponseStatus(HttpStatus.NOT_FOUND)
public class ResourceNotFoundException extends RuntimeException {
// 自定义错误信息的本地化处理
public ResourceNotFoundException(String messageKey) {
super(messageKey);
}
}
```
通过以上实例演示,读者可以更好地理解如何在实际项目中应用Spring Boot的国际化与本地化处理技术,从而提升应用的用户体验和可维护性。
0
0
相关推荐
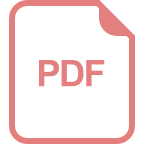
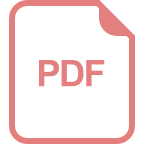
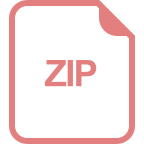
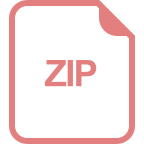
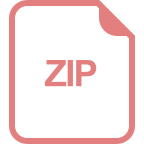
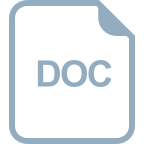
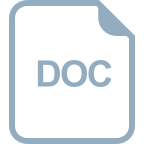
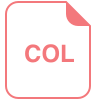