IntelliJ IDEA中的RESTful API开发与测试
发布时间: 2023-12-19 19:38:07 阅读量: 41 订阅数: 28 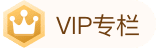
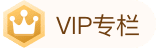
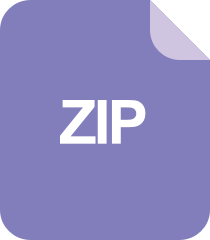
基于IntelliJ IDEA的Restful Fast Request插件设计源码
## 第一章:理解RESTful API概念
### 第二章:IntelliJ IDEA中的RESTful API开发环境配置
在本章中,我们将介绍如何在IntelliJ IDEA中配置RESTful API开发环境。我们将会讨论如何安装和配置IntelliJ IDEA,以及如何集成RESTful API开发插件。最后,我们将会学习如何创建新的RESTful API项目。让我们一起来深入了解吧!
### 第三章:使用IntelliJ IDEA进行RESTful API开发
在这一章节中,我们将介绍如何在IntelliJ IDEA中进行RESTful API的开发。我们将会逐步完成RESTful API接口设计、控制器编写以及数据库交互与持久化的过程。
#### 3.1 设计RESTful API接口
在进行RESTful API开发之前,首先需要设计好API的接口。RESTful API接口设计通常包括HTTP方法、URL路径、请求参数、响应格式等内容。我们将使用IntelliJ IDEA的RESTful API开发插件来进行接口设计,保证接口的合理性和易用性。
##### 示例代码:
```java
@RestController
@RequestMapping("/users")
public class UserController {
@Autowired
private UserService userService;
@GetMapping("/{id}")
public ResponseEntity<User> getUserById(@PathVariable Long id) {
User user = userService.getUserById(id);
if (user != null) {
return ResponseEntity.ok(user);
} else {
return ResponseEntity.notFound().build();
}
}
@PostMapping
public ResponseEntity<User> createUser(@RequestBody User user) {
User createdUser = userService.createUser(user);
return ResponseEntity.status(HttpStatus.CREATED).body(createdUser);
}
@PutMapping("/{id}")
public ResponseEntity<User> updateUser(@PathVariable Long id, @RequestBody User user) {
User updatedUser = userService.updateUser(id, user);
if (updatedUser != null) {
return ResponseEntity.ok(updatedUser);
} else {
return ResponseEntity.notFound().build();
}
}
@DeleteMapping("/{id}")
public ResponseEntity<Void> deleteUser(@PathVariable Long id) {
userService.deleteUser(id);
return ResponseEntity.noContent().build();
}
}
```
##### 代码说明:
- 上述代码定义了一个名为UserController的RESTful API控制器,处理与用户相关的API请求。
- 使用@GetMapping、@PostMapping、@PutMapping和@DeleteMapping注解来分别处理GET、POST、PUT和DELETE请求。
- 充分利用了Spring Framework提供的ResponseEntity类来构建标准的HTTP响应。
#### 3.2 编写RESTful API的控制器
在IntelliJ IDEA中,可以通过创建Spring MVC Controller来编写RESTful API的控制器。在Controller中可以定义路径、请求方法、请求参数等信息,并调用对应的服务方法进行业务处理。
##### 示例代码:
```java
@RestController
@RequestMapping("/products")
public class ProductController {
@Autowired
private ProductService productService;
@GetMapping("/{id}")
public ResponseEntity<Product> getProductById(@PathVariable Long id) {
Product product = productService.getProductById(id);
if (product != null) {
return ResponseEntity.ok(product);
} else {
return ResponseEntity.notFound().build();
}
}
@PostMapping
public ResponseEntity<Product> createProduct(@RequestBody Product product) {
Product createdProduc
```
0
0
相关推荐
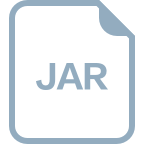
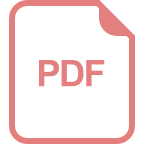





