Python enumerate() 函数实战秘籍:遍历列表、元组和字典的终极指南
发布时间: 2024-06-24 07:56:50 阅读量: 5 订阅数: 11 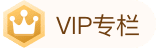
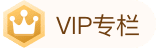
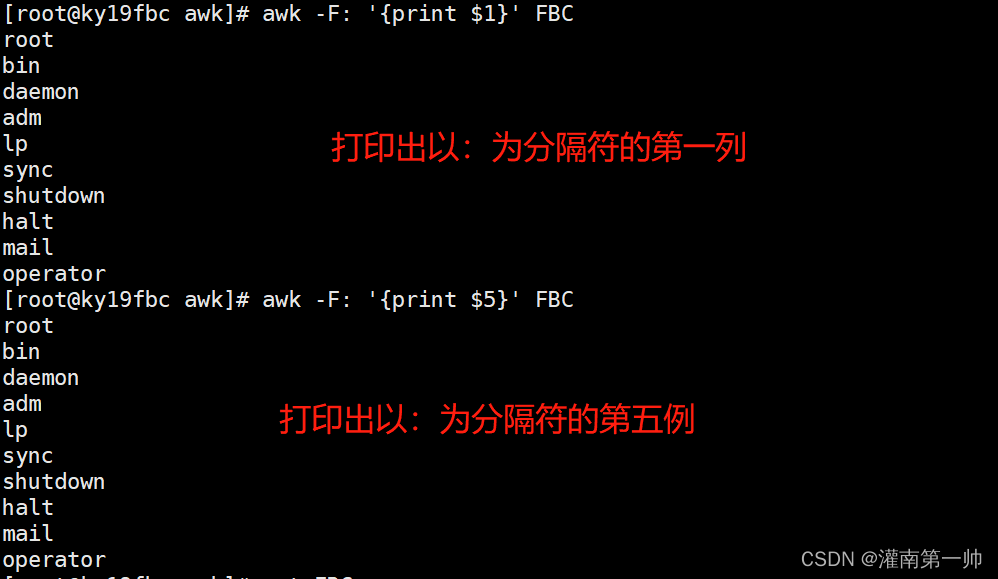
# 1. Python enumerate() 函数概述
Python 的 `enumerate()` 函数是一个强大的工具,用于遍历序列,同时返回索引和元素。它可以用于各种场景,包括:
- **获取索引和元素:** `enumerate()` 函数返回一个枚举对象,其中包含序列的索引和元素。
- **跳过指定元素:** 通过使用 `if` 语句,可以跳过序列中的特定元素。
- **修改列表元素:** 遍历枚举对象时,可以修改列表中的元素,从而实现原地修改。
# 2. 遍历列表
### 2.1 获取索引和元素
`enumerate()` 函数最基本的用法是遍历列表,同时返回元素的索引和元素本身。语法如下:
```python
for index, element in enumerate(list):
# 操作索引和元素
```
例如,遍历列表 `[1, 2, 3, 4, 5]`:
```python
for index, element in enumerate([1, 2, 3, 4, 5]):
print(f"索引:{index}, 元素:{element}")
```
输出:
```
索引:0, 元素:1
索引:1, 元素:2
索引:2, 元素:3
索引:3, 元素:4
索引:4, 元素:5
```
### 2.2 跳过指定元素
`enumerate()` 函数的 `start` 参数允许你从指定的索引开始遍历列表。例如,跳过前两个元素:
```python
for index, element in enumerate([1, 2, 3, 4, 5], start=2):
print(f"索引:{index}, 元素:{element}")
```
输出:
```
索引:2, 元素:3
索引:3, 元素:4
索引:4, 元素:5
```
### 2.3 修改列表元素
在遍历列表时,你可以使用 `enumerate()` 函数修改列表元素。例如,将列表中的偶数元素替换为 0:
```python
my_list = [1, 2, 3, 4, 5]
for index, element in enumerate(my_list):
if index % 2 == 0:
my_list[index] = 0
print(my_list)
```
输出:
```
[0, 2, 3, 0, 5]
```
# 3. 遍历元组
元组是 Python 中不可变的有序序列,用于存储一组值。与列表类似,元组也可以使用 enumerate() 函数进行遍历,但由于其不可变性,遍历元组时存在一些独特的考虑因素。
### 3.1 获取索引和元素
遍历元组时,enumerate() 函数的工作方式与遍历列表类似。它返回一个包含索引和元素的元组的迭代器。以下代码演示了如何遍历元组:
```python
my_tuple = (1, 2, 3, 4, 5)
for index, element in enumerate(my_tuple):
print(f"Index: {index}, Element: {element}")
```
输出:
```
Index: 0, Element: 1
Index: 1, Element: 2
Index: 2, Element: 3
Index: 3, Element: 4
Index: 4, Element: 5
```
### 3.2 解包元组
与列表不同,元组不能直接解包到变量中。但是,可以使用 enumerate() 函数来实现类似的效果。以下代码演示了如何使用 enumerate() 函数解包元组:
```python
my_tuple = (1, 2, 3, 4, 5)
for index, element in enumerate(my_tuple):
print(f"Index: {index}, Element: {element}")
# 解包元组
idx, elem = index, element
print
```
0
0
相关推荐
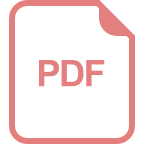
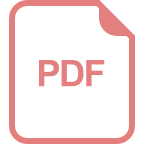
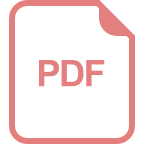





