多维数组的数据结构应用:解锁强大的数据处理能力
发布时间: 2024-07-14 08:44:26 阅读量: 35 订阅数: 27 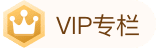
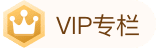
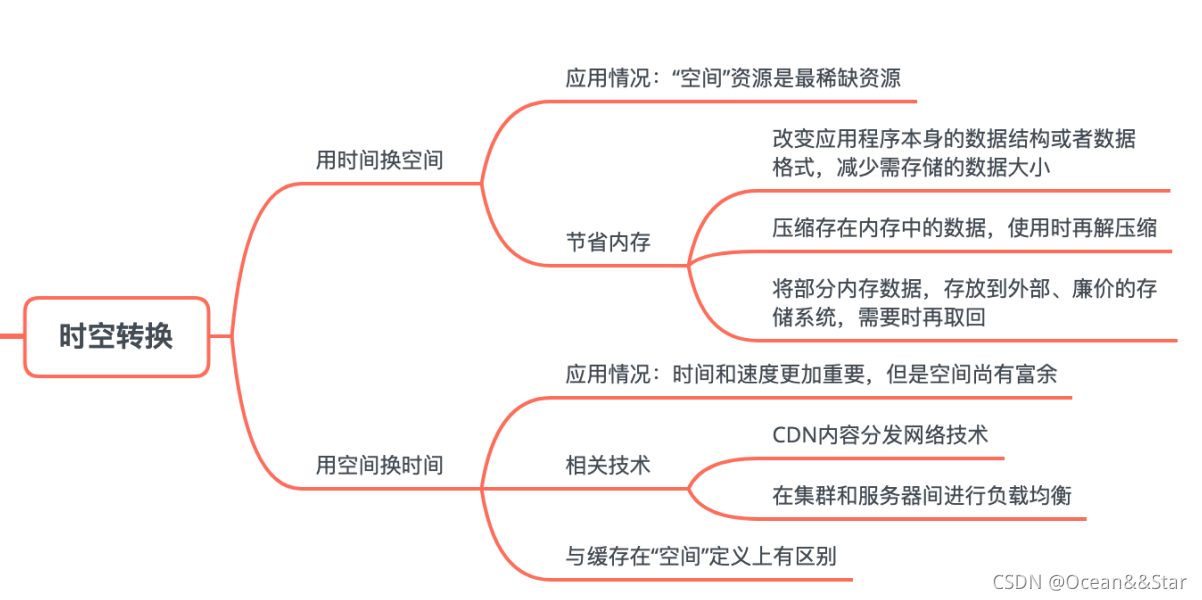
# 1. 多维数组的基本概念**
多维数组是一种数据结构,它可以存储具有多个维度的值。与一维数组不同,多维数组允许您根据多个索引访问元素。这使其成为存储和处理复杂数据结构的理想选择。
多维数组通常使用嵌套数组来表示。例如,一个二维数组可以表示为一个数组,其中每个元素都是一个一维数组。同样,一个三维数组可以表示为一个数组,其中每个元素都是一个二维数组,以此类推。
多维数组的维度数称为其秩。一维数组的秩为 1,二维数组的秩为 2,依此类推。数组的形状是由其每个维度中的元素数量定义的。例如,一个形状为 (3, 4) 的二维数组包含 3 行和 4 列,总共 12 个元素。
# 2. 多维数组的应用技巧
### 2.1 数组的遍历和访问
#### 2.1.1 一维数组的遍历和访问
一维数组的遍历和访问相对简单,可以使用 for 循环或 while 循环逐个访问数组中的元素。
```python
# 一维数组的遍历
arr = [1, 2, 3, 4, 5]
for element in arr:
print(element)
```
#### 2.1.2 多维数组的遍历和访问
多维数组的遍历和访问需要使用嵌套循环,对于一个二维数组,可以使用两个 for 循环来访问每个元素。
```python
# 二维数组的遍历
arr = [[1, 2, 3], [4, 5, 6], [7, 8, 9]]
for row in arr:
for element in row:
print(element)
```
### 2.2 数组的排序和查找
#### 2.2.1 一维数组的排序和查找
一维数组的排序和查找可以使用内置的 sort() 方法和 bisect.bisect() 方法。
```python
# 一维数组的排序
arr = [5, 2, 8, 3, 1]
arr.sort()
print(arr) # [1, 2, 3, 5, 8]
# 一维数组的查找
arr = [1, 3, 5, 7, 9]
index = bisect.bisect(arr, 4)
print(index) # 2
```
#### 2.2.2 多维数组的排序和查找
多维数组的排序和查找需要先将多维数组转换为一维数组,然后再使用一维数组的排序和查找方法。
```python
# 二维数组的排序
arr = [[1, 2, 3], [4, 5, 6], [7, 8, 9]]
flattened_arr = [item for sublist in arr for item in sublist]
flattened_arr.sort()
print(flattened_arr) # [1, 2, 3, 4, 5, 6, 7, 8, 9]
# 二维数组的查找
arr = [[1, 3, 5], [7, 9, 11], [13, 15, 17]]
flattened_arr = [item for sublist in arr for item in sublist]
index = bisect.bisect(flattened_arr, 10)
print(index) # 6
```
### 2.3 数组的插入和删除
#### 2.3.1 一维数组的插入和删除
一维数组的插入和删除可以使用 insert() 和 pop() 方法。
```python
# 一维数组的插入
arr = [1, 2, 3, 4, 5]
arr.insert(2, 2.5)
print(arr) # [1, 2, 2.5, 3, 4, 5]
# 一维数组的删除
arr = [1, 2, 3, 4, 5]
arr.pop(2)
print(arr) # [1, 2, 4, 5]
```
#### 2.3.2 多维数组的插入和删除
多维数组的插入和删除需要先将多维数组转换为一维数组,然后再使用一维数组的插入和删除方法。
```python
# 二维数组的插入
arr = [[1, 2, 3], [4, 5, 6], [7, 8, 9]]
flattened_arr = [item for sublist in arr for item in sublist]
flattened_arr.insert(5, 6.5)
arr = [flattened_arr[i:i + 3] for i in range(0, len(flattened_arr), 3)]
print(arr) # [[1, 2, 3], [4, 5, 6.5], [6, 7, 8], [9]]
# 二维数组的删除
arr = [[1, 2, 3], [4, 5, 6], [7, 8, 9]]
flattened_arr = [item for sublist in arr for item in sublist]
flattened_arr.pop(5)
arr = [flattened_arr[i:i + 3] for i in range(0, len(flattened_arr), 3)]
print(arr) # [[1, 2, 3], [4, 5, 6], [7, 8]]
```
# 3.1 数据统计和分析
多维数组在数据统计和分析中发挥着至关重要的作用。它可以有效地组织和处理大量数据,提取有价值的见解。
#### 3.1.1 一维数组中的数据统计和分析
一维数组存储一组同类型的数据元素,可以方便地进行数据统计和分析。例如,可以计算数组中元素的平均值、中位数、最大值和最小值。
```python
import numpy as np
# 创建一维数组
data = np.array([1, 3, 5, 7, 9])
# 计算平均值
mean = np.mean(data)
print("平均值:", mean)
# 计算中位数
median = np.median(data)
print("中位数:", median)
# 计算最大值
max_value = np.max(data)
print("最大值:", max_value)
# 计算最小值
min_value = np.min(data)
print("最小值:", min_value)
```
#### 3.1.2 多维数组中的数据统计和分析
多维数组可以存储更复杂的数据结构,例如表格或矩阵。这使得它可以进行更高级的数据统计和分析。例如,可以计算多维数组中每个行的平均值、每个列的总和,或不同维度之间的相关性。
```python
import numpy as np
# 创建多维数组
data = np.array([[1, 3, 5], [7, 9, 11], [13, 15, 17]])
# 计算每行的平均值
row_means = np.mean(data, axis=1)
print("每行的平均值:", row_means)
# 计算每列的总和
column_sums = np.sum(data, axis=0)
print("每列的总和:", column_sums)
# 计算不同维度之间的相关性
correlation = np.corrcoef(data)
print("不同维度之间的相关性:", correlation)
```
# 4. 多维数组的高级应用**
**4.1 稀疏矩阵**
**4.1.1 稀疏矩阵的概念和特点**
稀疏矩阵是一种特殊的多维数组,其特点是矩阵中非零元素的个数远少于零元素的个数。稀疏矩阵在许多科学计算和数据处理应用中都很常见,例如有限元分析、图像处理和机器学习。
稀疏矩阵的优点在于它可以节省大量的存储空间和计算时间。由于稀疏矩阵中大部分元素都是零,因此可以只存储非零元素及其位置,从而减少了存储空间。此外,在对稀疏矩阵进行操作时,可以跳过零元素,从而提高计算效率。
**4.1.2 稀疏矩阵的存储和操作**
存储稀疏矩阵有两种常见的方法:
* **坐标列表(COO)格式:**这种格式将稀疏矩阵的非零元素存储在一个列表中,每个元素包含其行号、列号和值。
* **压缩行存储(CSR)格式:**这种格式将稀疏矩阵的非零元素存储在一个值数组和两个索引数组中。值数组存储非零元素的值,索引数组存储非零元素在行中的起始位置和结束位置。
以下是使用 CSR 格式存储稀疏矩阵的示例代码:
```python
import numpy as np
# 创建一个稀疏矩阵
A = np.array([[0, 1, 0], [0, 0, 2], [0, 0, 0]])
# 转换为 CSR 格式
A_csr = A.tocsr()
# 获取稀疏矩阵的非零元素值
values = A_csr.data
# 获取稀疏矩阵的非零元素行索引
rows = A_csr.indices
# 获取稀疏矩阵的非零元素列索引
cols = A_csr.indptr
# 遍历稀疏矩阵的非零元素
for i in range(len(values)):
print(f"({rows[i]}, {cols[i]}): {values[i]}")
```
**代码逻辑逐行解读:**
1. `import numpy as np`:导入 NumPy 库,用于创建和操作稀疏矩阵。
2. `A = np.array([[0, 1, 0], [0, 0, 2], [0, 0, 0]])`:创建一个稀疏矩阵 A。
3. `A_csr = A.tocsr()`:将稀疏矩阵 A 转换为 CSR 格式。
4. `values = A_csr.data`:获取稀疏矩阵的非零元素值。
5. `rows = A_csr.indices`:获取稀疏矩阵的非零元素行索引。
6. `cols = A_csr.indptr`:获取稀疏矩阵的非零元素列索引。
7. `for i in range(len(values))`:遍历稀疏矩阵的非零元素。
8. `print(f"({rows[i]}, {cols[i]}): {values[i]}")`:打印每个非零元素及其位置。
**4.2 张量**
**4.2.1 张量的概念和特点**
张量是多维数组的推广,它可以具有任意数量的维度。张量在机器学习、深度学习和数据分析等领域有着广泛的应用。
与多维数组不同,张量中的元素可以具有不同的数据类型,并且可以进行复杂的数学运算。张量还支持广播操作,这允许在不同形状的张量之间进行元素级的运算。
**4.2.2 张量的操作和应用**
张量支持各种操作,包括:
* **元素级运算:**对张量中的每个元素执行算术运算,例如加法、减法和乘法。
* **广播运算:**将不同形状的张量扩展到相同形状,以便进行元素级的运算。
* **卷积运算:**在张量上应用卷积核,用于图像处理和特征提取。
* **池化运算:**对张量中的元素进行聚合,用于减少张量的尺寸和提取特征。
以下是使用 TensorFlow 创建和操作张量的示例代码:
```python
import tensorflow as tf
# 创建一个张量
t = tf.constant([[1, 2], [3, 4]])
# 获取张量的形状
shape = t.shape
# 获取张量的元素类型
dtype = t.dtype
# 对张量进行元素级加法
t_plus = t + 1
# 对张量进行广播加法
t_broadcast = t + tf.constant(5)
# 对张量进行卷积运算
t_conv = tf.nn.conv2d(t, tf.constant([[1, 2], [3, 4]]), strides=[1, 1, 1, 1], padding="SAME")
# 对张量进行池化运算
t_pool = tf.nn.max_pool(t, ksize=[1, 2, 2, 1], strides=[1, 2, 2, 1], padding="SAME")
```
**代码逻辑逐行解读:**
1. `import tensorflow as tf`:导入 TensorFlow 库,用于创建和操作张量。
2. `t = tf.constant([[1, 2], [3, 4]])`:创建一个张量 t。
3. `shape = t.shape`:获取张量的形状。
4. `dtype = t.dtype`:获取张量的元素类型。
5. `t_plus = t + 1`:对张量进行元素级加法。
6. `t_broadcast = t + tf.constant(5)`:对张量进行广播加法。
7. `t_conv = tf.nn.conv2d(t, tf.constant([[1, 2], [3, 4]]), strides=[1, 1, 1, 1], padding="SAME")`:对张量进行卷积运算。
8. `t_pool = tf.nn.max_pool(t, ksize=[1, 2, 2, 1], strides=[1, 2, 2, 1], padding="SAME")`:对张量进行池化运算。
# 5. 多维数组的优化和性能提升**
**5.1 数组的内存优化**
**5.1.1 数组的连续存储**
连续存储是指将多维数组中的元素按照顺序存储在内存中,避免了内存碎片化。通过使用 `np.contiguous()` 函数可以将非连续的数组转换为连续存储的数组:
```python
import numpy as np
# 创建一个非连续的数组
arr = np.array([[1, 2, 3], [4, 5, 6]], order='F')
print(arr.flags['C_CONTIGUOUS']) # False
# 转换为连续存储的数组
arr = np.ascontiguousarray(arr)
print(arr.flags['C_CONTIGUOUS']) # True
```
**5.1.2 数组的内存池管理**
内存池管理技术可以避免频繁的内存分配和释放操作,提高内存利用率。NumPy 提供了 `np.memmap()` 函数来创建内存映射数组,该数组直接映射到磁盘文件,无需加载到内存中:
```python
import numpy as np
# 创建一个内存映射数组
arr = np.memmap('data.bin', dtype='int32', shape=(10000, 10000))
# 对数组进行操作
arr[0, 0] = 100
```
**5.2 数组的并行处理**
**5.2.1 数组的并行遍历**
NumPy 提供了 `np.nditer()` 函数来并行遍历多维数组:
```python
import numpy as np
from concurrent.futures import ThreadPoolExecutor
# 创建一个多维数组
arr = np.arange(1000000).reshape(1000, 1000)
# 并行遍历数组
with ThreadPoolExecutor() as executor:
for i, j, value in np.nditer(arr):
executor.submit(lambda i, j, value: print(f"({i}, {j}): {value}"), i, j, value)
```
**5.2.2 数组的并行排序和查找**
NumPy 的 `np.argsort()` 和 `np.searchsorted()` 函数支持并行排序和查找:
```python
import numpy as np
from concurrent.futures import ProcessPoolExecutor
# 创建一个多维数组
arr = np.arange(1000000).reshape(1000, 1000)
# 并行排序数组
with ProcessPoolExecutor() as executor:
sorted_indices = list(executor.map(np.argsort, arr))
# 并行查找数组中的元素
with ProcessPoolExecutor() as executor:
found_indices = list(executor.map(np.searchsorted, arr, [100, 200]))
```
0
0
相关推荐
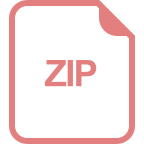
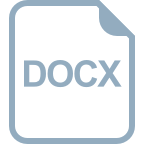
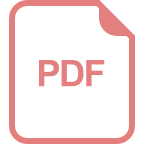






