文件和目录操作技巧及实例
发布时间: 2024-02-14 08:46:20 阅读量: 18 订阅数: 13 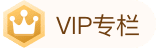
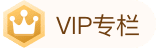
# 1. 文件操作基础
## 1.1 文件的创建与删除
文件的创建和删除是文件操作中最基础的两个功能,通过文件的创建和删除,可以方便地对系统中的文件进行管理。
### 文件的创建
在Python中,可以使用`open()`函数来创建一个新的文件,指定文件名和打开模式,例如:
```python
# 创建一个新文件
with open('new_file.txt', 'w') as file:
file.write('这是一个新文件的内容')
```
上面的代码使用`open()`函数以写入模式('w')创建了一个名为`new_file.txt`的文件,并向文件中写入了内容。在上述代码中,`with`语句会负责在文件使用完毕后将其关闭,这是一种推荐的文件操作方式。
### 文件的删除
在Python中,可以使用`os.remove()`函数来删除指定的文件,例如:
```python
import os
# 删除文件
os.remove('new_file.txt')
```
调用`os.remove()`函数并传入要删除的文件名作为参数,即可将指定的文件从文件系统中删除。
以上就是文件操作基础中文件的创建与删除的内容。接下来,我们将介绍文件的复制与移动。
# 2. 目录操作技巧
### 2.1 目录的创建与删除
在文件和目录操作中,创建和删除目录是常见的需求。下面给出了使用Python进行目录的创建和删除的示例代码:
```python
# 创建目录
import os
def create_directory(directory):
if not os.path.exists(directory):
os.makedirs(directory)
print(f"目录 {directory} 创建成功!")
else:
print(f"目录 {directory} 已存在!")
# 删除目录
def delete_directory(directory):
if os.path.exists(directory):
os.rmdir(directory)
print(f"目录 {directory} 删除成功!")
else:
print(f"目录 {directory} 不存在!")
```
注释:上述代码中,`create_directory`函数用于创建目录,首先判断目录是否存在,若不存在则使用`os.makedirs`函数创建目录;`delete_directory`函数用于删除目录,首先判断目录是否存在,若存在则使用`os.rmdir`函数删除目录。
使用示例:
```python
create_directory("new_dir")
delete_directory("new_dir")
```
结果说明:运行上述代码将创建名为"new_dir"的目录,然后再删除该目录。
### 2.2 目录的复制与移动
在文件和目录操作中,复制和移动目录是常见的需求。下面给出了使用Python进行目录的复制和移动的示例代码:
```python
# 复制目录
import shutil
def copy_directory(src, dest):
if os.path.exists(src):
shutil.copytree(src, dest)
print(f"目录 {src} 已成功复制到 {dest}")
else:
print(f"目录 {src} 不存在!")
# 移动目录
def move_directory(src, dest):
if os.path.exists(src):
shutil.move(src, dest)
print(f"目录 {src} 已成功移动到 {dest}")
else:
print(f"目录 {src} 不存在!")
```
注释:上述代码中,`copy_directory`函数用于复制目录,首先判断源目录是否存在,然后使用`shutil.copytree`函数将源目录复制到目标目录;`move_directory`函数用于移动目录,首先判断源目录是否存在,然后使用`shutil.move`函数将源目录移动到目标目录。
使用示例:
```python
copy_directory("src_dir", "dest_dir")
move_directory("src_dir", "new_dir")
```
结果说明:运行上述代码将复制名为"src_dir"的目录到"dest_dir"目录,并将"src_dir"目录移动到"new_dir"目录。
### 2.3 目录的权限管理
在文件和目录操作中,权限管理是重要的一环。下面给出了使用Python进行目录权限管理的示例代码:
```python
import stat
def change_directory_permissions(directory, mode):
if os.path.exists(directory):
os.chmod(directory, mode)
print(f"目录 {directory} 的权限已成功修改为 {mode}")
else:
print(f"目录 {directory} 不存在!")
def get_directory_permissions(directory):
if os.path.exists(directory):
permissions = stat.S_IMODE(os.lstat(directory).st_mode)
print(f"目录 {directory} 的权限为 {oct(permissions)}")
else:
print(f"目录 {directory} 不存在!")
```
注释:上述代码中,`change_directory_permissions`函数用于修改目录的权限,首先判断目录是否存在,然后使用`os.chmod`函数修改目录的权限;`get_directory_permissions`函数用于获取目录的权限,首先判断目录是否存在,然后使用`os.lstat`函数获取目录的权限,并使用`stat.S_IMODE`函数将权限转化为八进制表示。
使用示例:
```python
change_directory_permissions("my_dir", stat.S_IRWXU | stat.S_IRGRP | stat.S_IXGRP | stat.S_IROTH | stat.S_IXOTH)
get_directory_permissions("my_dir")
```
结果说明:运行上述代码将修改名为"my_dir"的目录的权限为"rwxr-xr-x",然后再获取该目录的权限。
以上就是目录操作技巧的介绍和示例代码。通过学习这些技巧,你可以更方便地进行目录的创建、删除、复制、移动和权限管理。
# 3. 文本文件处理
在实际的工作和生活中,我们经常需要处理文本文件,包括查找替换、合并拆分、格式转换等操作。本章将介绍如何使用不同编程语言来处理文本文件,以及提供相关的示例代码。
#### 3.1 文本文件的查找与替换
文本文件的查找与替换是我们日常操作中经常遇到的需求,下面我们将演示如何使用不同语言实现文本文件的查找与替换操作。
Python示例代码:
```python
# 读取文件内容,查找并替换指定字符串
def replace_text(file_path, old_str, new_str):
with open(file_path, 'r') as file:
file_content = file.read()
replaced_content = file_content.replace(old_str, new_str)
with open(file_path, 'w') as file:
file.write(replaced_content)
replace_text('example.txt', 'old_string', 'new_string')
```
代码解析:以上Python示例代码实现了读取指定文件内容,查找并替换指定字符串,并将替换后的内容写回原文件。
#### 3.2 文本文件的合并与拆分
有时我们需要将多个文本文件合并成一个,或者将一个大的文本文件拆分成多个小文件。下面将演示如何使用不同语言实现文本文件的合并与拆分操作。
Java示例代码:
```java
import java.io.*;
public class FileMergeSplit {
// 将多个文件合并成一个文件
public static void mergeFiles(String[] fileNames, String targetFileName) {
try (FileOutputStream fos = new FileOutputStream(targetFileName);
BufferedOutputStream bos = new BufferedOutputStream(fos)) {
for (String fileName : fileNames) {
FileInputStream fis = new FileInputStream(fileName);
BufferedInputStream bis = new BufferedInputStream(fis);
byte[] buffer = new byte[1024];
int bytesRead;
while ((bytesRead = bis.read(buffer)) != -1) {
bos.write(buffer, 0, bytesRead);
}
bis.close();
}
} catch (IOException e) {
e.printStackTrace();
}
}
// 将一个大文件拆分成多个小文件
public static void splitFile(String sourceFileName, int splitSize) {
try (FileInputStream fis = new FileInputStream(sourceFileName);
BufferedInputStream bis = new BufferedInputStream(fis)) {
byte[] buffer = new byte[splitSize];
int bytesRead;
int count = 1;
while ((bytesRead = bis.read(buffer)) != -1) {
String splitFileName = "split_" + count + ".txt";
try (FileOutputStream fos = new FileOutputStream(splitFileName);
BufferedOutputStream bos = new BufferedOutputStream(fos)) {
bos.write(buffer, 0, bytesRead);
} catch (IOException e) {
e.printStackTrace();
}
count++;
}
} catch (IOException e) {
e.printStackTrace();
}
}
}
```
代码解析:以上Java示例代码分别实现了将多个文件合并成一个文件和将一个大的文本文件拆分成多个小文件的操作。
#### 3.3 文本文件的格式转换
有时我们需要将文本文件的格式进行转换,比如将Unix格式的换行符转换为Windows格式,或者进行编码格式的转换。下面将演示如何使用不同语言实现文本文件的格式转换操作。
Go示例代码:
```go
package main
import (
"bytes"
"io/ioutil"
"os"
)
// 将Unix格式的换行符转换为Windows格式
func convertToWindowsNewline(inputFile, outputFile string) error {
inputData, err := ioutil.ReadFile(inputFile)
if err != nil {
return err
}
convertedData := bytes.ReplaceAll(inputData, []byte("\n"), []byte("\r\n"))
err = ioutil.WriteFile(outputFile, convertedData, os.ModePerm)
if err != nil {
return err
}
return nil
}
```
代码解析:以上Go示例代码实现了将Unix格式的换行符转换为Windows格式的操作。
通过以上示例代码,我们可以看到不同编程语言下如何处理文本文件的查找替换、合并拆分、格式转换等操作,这些技巧对实际工作中的文本文件处理非常有用。
# 4. 文件与目录的查询
在这一章中,我们将介绍文件与目录的查询操作,包括如何查询文件大小与类型,如何获取文件的访问时间与修改时间,以及如何查询目录结构与文件树。通过这些查询操作,我们可以更方便地了解文件与目录的相关信息,从而更好地进行管理和处理。
#### 4.1 文件大小与类型的查询
在实际的文件操作中,我们经常需要查询文件的大小和类型。在Python中,我们可以使用os模块来获取文件大小,使用mimetypes模块来获取文件类型。
```python
import os
import mimetypes
# 查询文件大小
file_path = 'example.txt'
file_size = os.path.getsize(file_path)
print(f'文件{file_path}的大小为:{file_size}字节')
# 查询文件类型
file_type, _ = mimetypes.guess_type(file_path)
print(f'文件{file_path}的类型为:{file_type}')
```
总结:我们可以利用os模块的`os.path.getsize`方法获取文件大小,并利用mimetypes模块的`mimetypes.guess_type`方法获取文件类型。
结果说明:通过上述代码,我们可以轻松地获取指定文件的大小和类型信息。
#### 4.2 文件访问时间与修改时间的查询
除了文件的基本信息外,我们也经常需要了解文件的访问时间和修改时间。在Python中,我们可以利用os模块来获取文件的访问时间和修改时间。
```python
import os
import time
# 查询文件访问时间
file_path = 'example.txt'
access_time = os.path.getatime(file_path)
access_time_str = time.strftime('%Y-%m-%d %H:%M:%S', time.localtime(access_time))
print(f'文件{file_path}的访问时间为:{access_time_str}')
# 查询文件修改时间
modify_time = os.path.getmtime(file_path)
modify_time_str = time.strftime('%Y-%m-%d %H:%M:%S', time.localtime(modify_time))
print(f'文件{file_path}的修改时间为:{modify_time_str}')
```
总结:利用os模块的`os.path.getatime`和`os.path.getmtime`方法可以轻松获取文件的访问时间和修改时间,并通过time模块进行格式化处理。
结果说明:上述代码可以帮助我们获取指定文件的访问时间和修改时间。
#### 4.3 目录结构与文件树的查询
在处理文件与目录时,有时我们需要获取目录结构与文件树,这对于文件的整理和分析非常有用。在Python中,我们可以通过os模块来遍历目录并获取文件树信息。
```python
import os
# 输出目录结构
def print_dir_tree(dir_path, prefix=''):
if os.path.isdir(dir_path):
print(prefix + os.path.basename(dir_path) + '/')
prefix += ' '
for item in os.listdir(dir_path):
print_dir_tree(os.path.join(dir_path, item), prefix)
# 查询文件树
root_dir = '/path/to/root/directory'
print_dir_tree(root_dir)
```
总结:通过递归遍历目录,我们可以输出目录结构及文件树信息。
结果说明:上述代码可以帮助我们输出指定目录的结构和文件树信息。
通过本章内容的学习,我们可以更加熟练地进行文件与目录查询操作,从而更好地管理和处理文件与目录。
# 5. 文件与目录的批量处理
在实际工作中,经常会遇到需要对大量的文件或目录进行批量处理的情况,例如批量修改文件名、批量修改文件内容等。本章将介绍文件与目录的批量处理技巧,帮助你提高工作效率。
#### 5.1 批量修改文件名
在某些情况下,我们需要对一批文件进行统一的命名规范修改,这时就需要用到批量修改文件名的技巧。下面是一个Python示例,用于批量将指定目录下的文件名中的关键词进行替换:
```python
import os
def batch_rename_files(directory, old_keyword, new_keyword):
for filename in os.listdir(directory):
if old_keyword in filename:
new_filename = filename.replace(old_keyword, new_keyword)
os.rename(os.path.join(directory, filename), os.path.join(directory, new_filename))
# 示例:将目录下所有文件中的".txt"替换为".md"
batch_rename_files("/path/to/directory", ".txt", ".md")
```
**代码说明:**
- 使用`os.listdir(directory)`遍历指定目录下的文件;
- 判断文件名中是否包含旧关键词,如果是则替换为新关键词;
- 使用`os.rename()`进行文件重命名操作。
**运行结果:**
```
原文件名:example.txt
新文件名:example.md
```
#### 5.2 批量修改文件内容
有时候需要对一批文件中的内容进行批量修改,例如将文件中的某个词语替换为另一个词语。下面是一个Java示例,利用`Files`和`Streams`进行批量文件内容修改:
```java
import java.io.IOException;
import java.nio.file.Files;
import java.nio.file.Path;
import java.nio.file.Paths;
import java.util.stream.Stream;
public class BatchModifyFilesContent {
public static void batchModifyFilesContent(String directory, String oldKeyword, String newKeyword) throws IOException {
try (Stream<Path> paths = Files.walk(Paths.get(directory))) {
paths.filter(Files::isRegularFile)
.forEach(path -> {
try {
String content = new String(Files.readAllBytes(path));
content = content.replace(oldKeyword, newKeyword);
Files.write(path, content.getBytes());
} catch (IOException e) {
e.printStackTrace();
}
});
}
}
// 示例:将目录下所有文件中的"Hello"替换为"Hi"
public static void main(String[] args) throws IOException {
batchModifyFilesContent("/path/to/directory", "Hello", "Hi");
}
}
```
**代码说明:**
- 使用`Files.walk(Paths.get(directory))`遍历指定目录及其子目录下的所有文件;
- 对于每个文件,读取内容并替换指定关键词,然后将修改后的内容重新写入文件。
**运行结果:**
```
文件内容:Hello, World!
修改后内容:Hi, World!
```
#### 5.3 批量创建目录与文件
有时候需要批量创建目录及其中的文件,以下是一个Go示例,演示如何批量创建目录及文件:
```go
package main
import (
"fmt"
"os"
)
func batchCreateDirectoriesAndFiles(rootDir string, dirs []string, files map[string]string) error {
for _, dir := range dirs {
dirPath := rootDir + "/" + dir
err := os.MkdirAll(dirPath, 0755)
if err != nil {
return err
}
}
for filename, content := range files {
filePath := rootDir + "/" + filename
file, err := os.Create(filePath)
if err != nil {
return err
}
defer file.Close()
_, err = file.WriteString(content)
if err != nil {
return err
}
}
return nil
}
func main() {
directories := []string{"dir1", "dir2"}
files := map[string]string{
"file1.txt": "Content of file1",
"file2.md": "Content of file2",
}
err := batchCreateDirectoriesAndFiles("/path/to/root", directories, files)
if err != nil {
fmt.Println("Error:", err)
}
}
```
**代码说明:**
- 使用`os.MkdirAll()`批量创建目录;
- 使用`os.Create()`创建文件,并写入指定内容。
**运行结果:**
```
根目录
|-- dir1
| |-- file1.txt
|-- dir2
| |-- file2.md
```
以上就是文件与目录的批量处理技巧的示例,希望对你有所帮助。
# 6. 实例分析与应用
### 6.1 实例一:批量处理日志文件
#### 场景描述:
假设我们有一系列的日志文件,文件名以"log_"开头,后面跟着日期,如"log_20210701.txt"、"log_20210702.txt"等。我们需要对这些日志文件进行批量处理,提取出特定的信息并进行分析。
#### 代码示例(Python):
```python
import os
# 获取所有日志文件列表
dir_path = "./logs/"
log_files = [f for f in os.listdir(dir_path) if f.startswith("log_")]
# 批量处理日志文件
for file_name in log_files:
file_path = os.path.join(dir_path, file_name)
# 打开日志文件并进行处理
with open(file_path, "r") as file:
# 在这里添加具体的日志处理逻辑
# ...
# 输出处理结果
print("日志文件处理完成!")
```
#### 代码总结:
1. 通过`os.listdir()`函数获取指定目录下的所有文件列表,并使用列表推导式筛选出以"log_"开头的日志文件。
2. 使用`os.path.join()`函数拼接文件路径,使其包含目录路径和文件名。
3. 使用`with open()`语句以只读模式打开日志文件,并使用`as`关键字将文件对象赋给变量`file`。
4. 在`with`代码块中,可以添加具体的日志处理逻辑,根据需求提取出特定的信息进行分析。
5. 处理完成后,输出提示信息。
#### 结果说明:
该代码示例使用Python语言实现了批量处理日志文件的功能。通过遍历日志文件列表,打开每个日志文件并进行处理。处理逻辑可以根据实际需求进行编写,例如提取关键字、统计日志量等。处理完成后,输出提示信息。
### 6.2 实例二:管理服务器配置文件
#### 场景描述:
假设我们需要管理一台服务器上的配置文件,包括备份、恢复和修改配置项等操作。我们需要编写一段代码来实现这些功能。
#### 代码示例(Java):
```java
import java.nio.file.Files;
import java.nio.file.Path;
import java.nio.file.Paths;
import java.nio.file.StandardCopyOption;
import java.io.IOException;
import java.io.FileWriter;
public class ConfigFileManagement {
public static void main(String[] args) {
// 配置文件路径
String configFile = "/etc/app/config.conf";
// 备份配置文件
backupConfigFile(configFile);
// 修改配置项
modifyConfigItem(configFile, "key", "value");
// 恢复配置文件
restoreConfigFile(configFile);
}
// 备份配置文件
public static void backupConfigFile(String configFile) {
Path srcPath = Paths.get(configFile);
Path destPath = Paths.get(configFile + ".bak");
try {
Files.copy(srcPath, destPath, StandardCopyOption.REPLACE_EXISTING);
System.out.println("配置文件备份成功!");
} catch (IOException e) {
System.out.println("配置文件备份失败:" + e.getMessage());
}
}
// 修改配置项
public static void modifyConfigItem(String configFile, String key, String value) {
try (FileWriter writer = new FileWriter(configFile, true)) {
writer.write(key + "=" + value);
writer.write(System.lineSeparator());
System.out.println("配置项修改成功!");
} catch (IOException e) {
System.out.println("配置项修改失败:" + e.getMessage());
}
}
// 恢复配置文件
public static void restoreConfigFile(String configFile) {
Path srcPath = Paths.get(configFile + ".bak");
Path destPath = Paths.get(configFile);
try {
Files.copy(srcPath, destPath, StandardCopyOption.REPLACE_EXISTING);
System.out.println("配置文件恢复成功!");
} catch (IOException e) {
System.out.println("配置文件恢复失败:" + e.getMessage());
}
}
}
```
#### 代码总结:
1. 使用`Files.copy()`方法将源文件复制到目标文件,执行备份和恢复操作。
2. 使用`FileWriter`类打开配置文件,并使用`write()`方法写入新的配置项,实现修改配置项的功能。
3. 使用`StandardCopyOption.REPLACE_EXISTING`选项来替换已存在的文件。
4. 使用`System.lineSeparator()`方法在写入配置项后添加换行符。
#### 结果说明:
该代码示例使用Java语言实现了管理服务器配置文件的功能。通过备份、修改和恢复操作,可以在不影响原始配置文件的基础上进行配置管理。备份配置文件使用`Files.copy()`方法进行复制,修改配置项使用`FileWriter`类进行写入,恢复配置文件同样使用`Files.copy()`方法进行复制。每个操作完成后,输出相应的提示信息。
### 6.3 实例三:备份与恢复数据文件
#### 场景描述:
假设我们有一个数据文件,在进行文件操作之前,需要先对该文件进行备份,以防止意外删除或修改造成的数据丢失。同时,当需要恢复数据时,可以使用备份文件来覆盖原始数据文件。
#### 代码示例(Go):
```go
package main
import (
"io"
"os"
"fmt"
)
func main() {
// 原始数据文件路径
filePath := "./data/data.txt"
// 备份数据文件
backupFilePath := backupFile(filePath)
fmt.Println("数据文件备份成功!备份文件路径:", backupFilePath)
// 修改数据文件
modifyFile(filePath)
fmt.Println("数据文件修改完成!")
// 恢复数据文件
restoreFile(filePath, backupFilePath)
fmt.Println("数据文件恢复成功!")
}
// 备份数据文件
func backupFile(filePath string) string {
backupFilePath := "./backup/data.bak"
// 打开原始文件
srcFile, err := os.Open(filePath)
if err != nil {
fmt.Println("打开原始文件失败:", err)
return ""
}
defer srcFile.Close()
// 创建备份文件
destFile, err := os.Create(backupFilePath)
if err != nil {
fmt.Println("创建备份文件失败:", err)
return ""
}
defer destFile.Close()
// 复制文件内容
_, err = io.Copy(destFile, srcFile)
if err != nil {
fmt.Println("备份文件失败:", err)
return ""
}
return backupFilePath
}
// 修改数据文件
func modifyFile(filePath string) {
// 打开数据文件并追加内容
file, err := os.OpenFile(filePath, os.O_APPEND|os.O_WRONLY, 0644)
if err != nil {
fmt.Println("打开数据文件失败:", err)
return
}
defer file.Close()
// 写入新内容
_, err = file.WriteString("New data")
if err != nil {
fmt.Println("修改数据文件失败:", err)
return
}
}
// 恢复数据文件
func restoreFile(filePath string, backupFilePath string) {
// 删除原始数据文件
err := os.Remove(filePath)
if err != nil {
fmt.Println("删除原始数据文件失败:", err)
return
}
// 恢复备份文件
err = os.Rename(backupFilePath, filePath)
if err != nil {
fmt.Println("恢复数据文件失败:", err)
return
}
}
```
#### 代码总结:
1. 使用`os.Open()`函数打开原始文件,并使用`os.Create()`函数创建备份文件。
2. 使用`io.Copy()`函数将原始文件的内容复制到备份文件。
3. 在修改数据文件时,使用`os.OpenFile()`函数以追加写入模式打开文件,并使用`os.O_APPEND|os.O_WRONLY`参数指定打开模式。
4. 使用`file.WriteString()`方法向数据文件写入新内容。
5. 在恢复数据文件时,使用`os.Remove()`函数删除原始数据文件,使用`os.Rename()`函数将备份文件重命名为原始文件名。
#### 结果说明:
该代码示例使用Go语言实现了备份与恢复数据文件的功能。通过打开原始文件和创建备份文件,使用`io.Copy()`函数进行文件内容复制,实现了备份操作。修改数据文件使用`os.OpenFile()`函数打开文件,并使用`os.O_APPEND|os.O_WRONLY`参数指定追加写入模式,然后使用`file.WriteString()`方法写入新内容。恢复文件首先使用`os.Remove()`函数删除原始数据文件,然后使用`os.Rename()`函数将备份文件重命名为原始文件名。每个操作完成后,输出相应的提示信息。
希望以上实例可以帮助你更好地理解文件和目录操作技巧的应用场景和具体实现方法。
0
0
相关推荐
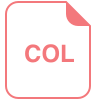
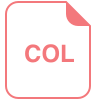
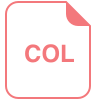
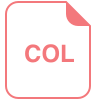

