Python enumerate函数进阶技巧:解锁序列处理新境界
发布时间: 2024-06-22 17:56:45 阅读量: 74 订阅数: 36 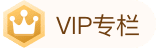
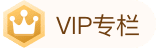
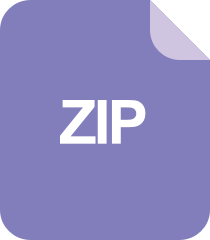
dnSpy-net-win32-222.zip
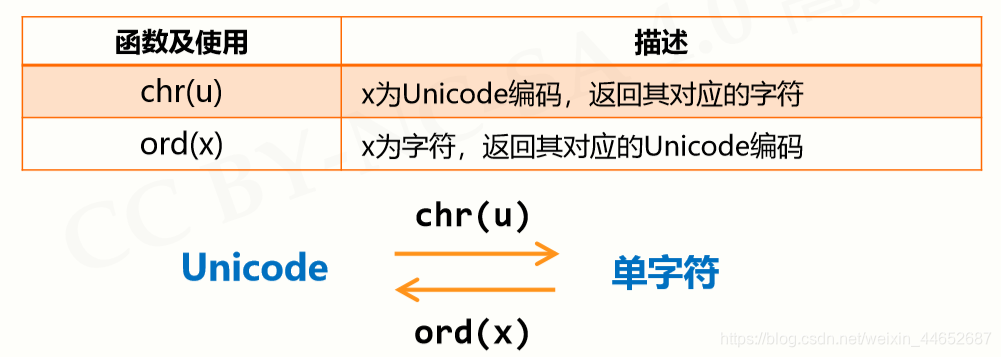
# 1. Python enumerate函数简介
Python 的 `enumerate()` 函数是一个内置函数,用于遍历序列中的元素并返回一个枚举对象。枚举对象是一个元组,包含元素及其索引。`enumerate()` 函数的语法如下:
```python
enumerate(sequence, start=0)
```
其中:
* `sequence` 是要遍历的序列,可以是列表、元组、字符串或其他可迭代对象。
* `start` 是可选参数,指定枚举的起始索引,默认为 0。
# 2. enumerate函数的进阶技巧
### 2.1 遍历序列中的元素及其索引
enumerate函数最基本的用法是遍历序列中的元素及其索引。语法如下:
```python
for index, element in enumerate(sequence):
# 操作元素和索引
```
例如,遍历一个列表并打印元素及其索引:
```python
my_list = ['a', 'b', 'c', 'd']
for index, element in enumerate(my_list):
print(f"Index: {index}, Element: {element}")
```
输出:
```
Index: 0, Element: a
Index: 1, Element: b
Index: 2, Element: c
Index: 3, Element: d
```
### 2.2 修改序列中的元素
enumerate函数不仅可以遍历序列,还可以修改序列中的元素。语法如下:
```python
for index, element in enumerate(sequence):
sequence[index] = new_value
```
例如,将列表中所有元素都修改为大写:
```python
my_list = ['a', 'b', 'c', 'd']
for index, element in enumerate(my_list):
my_list[index] = element.upper()
print(my_list)
```
输出:
```
['A', 'B', 'C', 'D']
```
### 2.3 过滤序列中的元素
enumerate函数还可以用于过滤序列中的元素。语法如下:
```python
for index, element in enumerate(sequence):
if condition:
# 操作元素和索引
```
例如,过滤列表中所有偶数索引的元素:
```python
my_list = [1, 2, 3, 4, 5, 6]
for index, element in enumerate(my_list):
if index % 2 == 0:
print(f"Index: {index}, Element: {element}")
```
输出:
```
Index: 0, Element: 1
Index: 2, Element: 3
Index: 4, Element: 5
```
# 3.1 统计序列中元素的出现次数
enumerate 函数可以方便地统计序列中元素的出现次数。通过使用它,我们可以创建一个字典,其中键是序列中的元素,值是这些元素出现的次数。
```python
def count_occurrences(sequence):
"""统计序列中元素的出现次数。
Args:
sequence (list): 要统计的序列。
Returns:
dict: 元素及其出现次数的字典。
"""
occurrences = {}
for index, element in enumerate(sequence):
if element not in occurrences:
occurrences[element] = 0
occurrences[element] += 1
return occurrences
```
**代码逻辑逐行解读:**
* 定义 `count_occurrences` 函数,接受一个序列 `sequence` 作为参数。
* 初始化一个空字典 `occurrences` 来存储元素及其出现次数。
* 使用 `enumerate` 函数遍历序列,同时获取元素 `element` 和其索引 `index`。
* 如果 `element` 不在 `occurrences` 中,则将其添加并初始化出现次数为 0。
* 对于每个 `element`,将 `occurrences[element]` 加 1。
* 返回 `occurrences` 字典。
**参数说明:**
* `sequence`: 要统计的序列,可以是列表、元组或其他可迭代对象。
**使用示例:**
```python
sequence = [1, 2, 3, 4, 5, 1, 2, 3]
occurrences = count_occurrences(sequence)
print(occurrences)
```
**输出:**
```
{1: 2, 2: 2, 3: 2, 4: 1, 5: 1}
```
### 3.2 将序列元素映射到新序列
enumerate 函数还可以用于将序列元素映射到新序列。我们可以使用它来创建新序列,其中每个元素都是原始序列中元素的某个函数的输出。
```python
def map_elements(sequence, func):
"""将序列元素映射到新序列。
Args:
sequence (list): 要映射的序列。
func (function): 将序列元素映射到新元素的函数。
Returns:
list: 映射后的新序列。
"""
mapped_sequence = []
for index, element in enumerate(sequence):
mapped_sequence.append(func(element))
return mapped_sequence
```
**代码逻辑逐行解读:**
* 定义 `map_elements` 函数,接受一个序列 `sequence` 和一个函数 `func` 作为参数。
* 初始化一个空列表 `mapped_sequence` 来存储映射后的元素。
* 使用 `enumerate` 函数遍历序列,同时获取元素 `element` 和其索引 `index`。
* 将 `element` 传递给函数 `func`,并将结果添加到 `mapped_sequence` 中。
* 返回 `mapped_sequence` 列表。
**参数说明:**
* `sequence`: 要映射的序列,可以是列表、元组或其他可迭代对象。
* `func`: 将序列元素映射到新元素的函数。
**使用示例:**
```python
sequence = [1, 2, 3, 4, 5]
mapped_sequence = map_elements(sequence, lambda x: x * 2)
print(mapped_sequence)
```
**输出:**
```
[2, 4, 6, 8, 10]
```
### 3.3 对序列元素进行分组
enumerate 函数还可以用于对序列元素进行分组。我们可以使用它来创建字典,其中键是分组依据,值是属于该组的元素列表。
```python
def group_elements(sequence, key_func):
"""对序列元素进行分组。
Args:
sequence (list): 要分组的序列。
key_func (function): 将序列元素映射到分组依据的函数。
Returns:
dict: 分组依据及其对应元素列表的字典。
"""
groups = {}
for index, element in enumerate(sequence):
key = key_func(element)
if key not in groups:
groups[key] = []
groups[key].append(element)
return groups
```
**代码逻辑逐行解读:**
* 定义 `group_elements` 函数,接受一个序列 `sequence` 和一个函数 `key_func` 作为参数。
* 初始化一个空字典 `groups` 来存储分组依据及其对应元素列表。
* 使用 `enumerate` 函数遍历序列,同时获取元素 `element` 和其索引 `index`。
* 将 `element` 传递给函数 `key_func`,并将结果作为分组依据 `key`。
* 如果 `key` 不在 `groups` 中,则将其添加并初始化一个空列表。
* 将 `element` 添加到 `groups[key]` 列表中。
* 返回 `groups` 字典。
**参数说明:**
* `sequence`: 要分组的序列,可以是列表、元组或其他可迭代对象。
* `key_func`: 将序列元素映射到分组依据的函数。
**使用示例:**
```python
sequence = [
{"name": "Alice", "age": 20},
{"name": "Bob", "age": 30},
{"name": "Charlie", "age": 25},
{"name": "Dave", "age": 35},
]
groups = group_elements(sequence, lambda x: x["age"])
print(groups)
```
**输出:**
```
{20: [{"name": "Alice", "age": 20}],
25: [{"name": "Charlie", "age": 25}],
30: [{"name": "Bob", "age": 30}],
35: [{"name": "Dave", "age": 35}]}
```
# 4. enumerate函数的扩展应用
### 4.1 与其他函数结合使用
enumerate函数可以与其他函数结合使用,以实现更复杂的操作。例如,我们可以使用enumerate函数和zip函数来同时遍历两个序列:
```python
# 遍历两个序列中的元素及其索引
for (i, x), (j, y) in zip(enumerate(a), enumerate(b)):
print(i, x, j, y)
```
### 4.2 在生成器表达式中使用
enumerate函数也可以在生成器表达式中使用。生成器表达式是一种简洁的语法,用于创建生成器对象。例如,我们可以使用enumerate函数和生成器表达式来创建包含序列中元素及其索引的元组列表:
```python
# 创建包含元素及其索引的元组列表
indexed_list = [(i, x) for i, x in enumerate(a)]
```
### 4.3 在列表解析中使用
enumerate函数也可以在列表解析中使用。列表解析是一种简洁的语法,用于创建列表。例如,我们可以使用enumerate函数和列表解析来创建包含序列中每个元素及其索引的字典:
```python
# 创建包含元素及其索引的字典
indexed_dict = {i: x for i, x in enumerate(a)}
```
### 代码块示例
```python
# 与zip函数结合使用
a = [1, 2, 3]
b = ['a', 'b', 'c']
for (i, x), (j, y) in zip(enumerate(a), enumerate(b)):
print(i, x, j, y)
```
**代码逻辑分析:**
* 该代码使用enumerate函数和zip函数同时遍历两个序列a和b。
* enumerate函数返回一个元组,其中第一个元素是索引,第二个元素是序列中的元素。
* zip函数将两个序列中的元素配对为元组,然后返回一个元组列表。
* for循环迭代zip函数返回的元组列表,并打印索引和元素。
**参数说明:**
* `a`: 序列a
* `b`: 序列b
### 表格示例
| 操作 | 描述 |
|---|---|
| `enumerate(a)` | 返回一个枚举对象,其中包含序列a的元素及其索引。 |
| `zip(enumerate(a), enumerate(b))` | 将两个枚举对象配对为元组,然后返回一个元组列表。 |
| `for (i, x), (j, y) in zip(enumerate(a), enumerate(b)):` | 遍历zip函数返回的元组列表,并打印索引和元素。 |
### Mermaid流程图示例
```mermaid
graph LR
subgraph enumerate函数的扩展应用
enumerate(a) --> zip(enumerate(a), enumerate(b)) --> for (i, x), (j, y) in zip(enumerate(a), enumerate(b)):
end
```
# 5. enumerate函数的性能优化
为了提高enumerate函数的性能,可以采取以下措施:
### 5.1 避免不必要的序列复制
enumerate函数会创建一个新的列表来存储枚举结果。如果序列很大,这可能会消耗大量内存。为了避免不必要的序列复制,可以使用itertools.chain()函数来连接枚举结果和原始序列:
```python
from itertools import chain
sequence = [1, 2, 3, 4, 5]
result = chain(enumerate(sequence), sequence)
```
### 5.2 使用内置函数代替循环
在某些情况下,可以使用内置函数来代替enumerate函数,从而提高性能。例如,可以使用count()函数来统计序列中元素的出现次数:
```python
sequence = [1, 2, 3, 4, 5, 1, 2, 3]
result = sequence.count(1)
```
### 5.3 优化索引的访问方式
如果需要频繁访问索引,可以使用enumerate()函数的start参数来指定起始索引,从而优化索引的访问方式:
```python
sequence = [1, 2, 3, 4, 5]
result = enumerate(sequence, start=2)
```
0
0
相关推荐
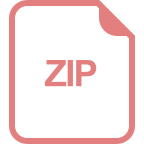
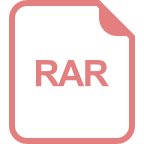