【PyCharm Installation Guide】: One-Stop Solution for PyCharm Installation and Configuration Issues
发布时间: 2024-09-14 23:13:02 阅读量: 106 订阅数: 44 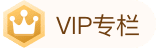
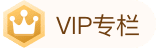
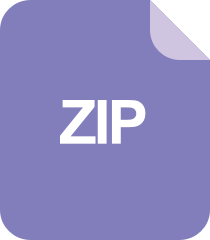
pycharm-guide:PyCharm中文指南:安装|破解|效率|技巧
# 1. Introduction to PyCharm
PyCharm is a powerful Python Integrated Development Environment (IDE) developed by JetBrains. It offers a range of tools and features for Python developers, including code editing, debugging, version control, and unit testing. PyCharm is compatible with various platforms, including Windows, macOS, and Linux.
Key features of PyCharm include:
* An intuitive code editor with syntax highlighting, code completion, and error detection.
* A robust debugger supporting breakpoints, step-by-step debugging, and variable inspection.
* Integrated version control systems supporting Git, Mercurial, and Subversion.
* An integrated unit testing framework for writing and running unit tests.
# 2. Installing PyCharm
### 2.1 System Requirements
Before installing PyCharm, ensure your system meets the following minimum requirements:
| Operating System | Minimum Version | Recommended Version |
|---|---|---|
| Windows | Windows 7 | Windows 10 or higher |
| macOS | macOS 10.12 | macOS 10.15 or higher |
| Linux | Ubuntu 16.04 | Ubuntu 20.04 or higher |
Additionally, make sure the following software is installed on your system:
- Python 3.6 or higher
- Java 8 or higher
### 2.2 Downloading PyCharm
1. Visit the official PyCharm website: ***
***
***
***
***
***
***
***
***
***
***
***
***
***
***
***
***
```bash
sudo dpkg -i pycharm-community.deb
```
4. Follow the instructions provided by the installation wizard.
### Code Block: Installing PyCharm Command (Linux)
```bash
sudo dpkg -i pycharm-community.deb
```
**Argument Explanation:**
- `sudo`: Run the command with administrative privileges.
- `dpkg`: Debian package manager.
- `-i`: Install the package.
- `pycharm-community.deb`: Name of the PyCharm installation package.
**Logical Analysis:**
This command uses the Debian package manager (dpkg) to install the PyCharm Community Edition with administrative privileges.
### Table: PyCharm System Requirements
| Operating System | Minimum Version | Recommended Version |
|---|---|---|
| Windows | Windows 7 | Windows 10 or higher |
| macOS | macOS 10.12 | macOS 10.15 or higher |
| Linux | Ubuntu 16.04 | Ubuntu 20.04 or higher |
### Mermaid Flowchart: PyCharm Installation Process
```mermaid
sequenceDiagram
participant User
participant PyCharm
User->PyCharm: Download PyCharm
PyCharm->User: Install PyCharm
User->PyCharm: Configure PyCharm
```
**Flowchart Explanation:**
This flowchart illustrates the general process of installing PyCharm. First, the user downloads the PyCharm installer package. Then, the user installs PyCharm. Finally, the user configures PyCharm.
# 3.1 Creating Projects
PyCharm offers multiple methods for creating projects, including:
- **Creating a project from scratch:** Select "File" -> "New Project" and then choose a project template.
- **Creating a project from existing code:** Select "File" -> "Open" and then choose the project folder to be opened.
- **Importing a project from version control:** Select "File" -> "New Project from Version Control," then choose the version control system and project repository.
When creating a project, you need to specify the project name, location, and type. Project types can include Python projects, Web projects, or scientific projects.
### 3.2 Configuring the Interpreter
PyCharm allows you to run projects using different Python interpreters. To configure the interpreter, follow these steps:
1. Open the "Settings/Preferences" dialog box (Windows/Linux: Ctrl+Alt+S, macOS: Cmd+,).
2. In the left navigation bar, select "Project" -> "Project Interpreter."
3. Click the "gear" icon, then choose "Add."
4. In the "Add Interpreter" dialog box, select the type of Python interpreter (e.g., system interpreter, virtual environment, or remote interpreter).
5. Click "OK" to add the interpreter.
### 3.3 Installing Plugins
PyCharm offers a rich plugin ecosystem that can extend its functionality. To install a plugin, follow these steps:
1. Open the "Settings/Preferences" dialog box (Windows/Linux: Ctrl+Alt+S, macOS: Cmd+,).
2. In the left navigation bar, select "Plugins."
3. In the "Marketplace" tab, search for the plugin you want to install.
4. Click the "Install" button to install the plugin.
After installing plugins, you need to restart PyCharm for them to take effect.
**Code Block: Creating a Project**
```python
import os
# Create project folder
os.makedirs("my_project")
# Create main Python file
with open("my_project/main.py", "w") as f:
f.write("print('Hello, world!')")
# Create project configuration file
with open("my_project/.idea/workspace.xml", "w") as f:
f.write("<project version='4'>\n"
" <component name='ProjectRootManager'>\n"
" <option name='projectStructureVersion' value='22' />\n"
" <option name='defaultListView' value='Project Files' />\n"
" <option name='projectRoot' value='$PROJECT_DIR$' />\n"
" </component>\n"
"</project>")
# Open project
os.system("pycharm my_project")
```
**Logical Analysis:**
This code uses Python's os module to create a project folder named "my_project" and a main Python file "main.py" inside it, along with a project configuration file "workspace.xml". Finally, it uses the pycharm command to open the project.
**Argument Explanation:**
- `os.makedirs("my_project")`: Creates a folder named "my_project".
- `with open("my_project/main.py", "w") as f:`: Opens the "main.py" file for writing.
- `f.write("print('Hello, world!')")`: Writes code into the "main.py" file.
- `with open("my_project/.idea/workspace.xml", "w") as f:`: Opens the "workspace.xml" file for writing.
- `f.write(...)`: Writes the project configuration into the "workspace.xml" file.
- `os.system("pycharm my_project")`: Opens the project using the pycharm command.
**Table: PyCharm Project Creation Methods**
| Method | Description |
|---|---|
| Creating a project from scratch | Creates a new empty project. |
| Creating a project from existing code | Creates a project from an existing code folder. |
| Importing a project from version control | Imports a project from version control systems (e.g., Git or SVN). |
**Mermaid Flowchart: PyCharm Plugin Installation Process**
```mermaid
graph LR
subgraph Installing Plugins
A[Open "Settings/Preferences" dialog] --> B[Select "Plugins"]
B --> C[Search for plugins in the "Marketplace" tab]
C --> D[Click "Install" button]
D --> E[Restart PyCharm]
end
```
# 4. PyCharm Debugging
### 4.1 Setting Breakpoints
A breakpoint is a marker that pauses program execution at a specific line of code. This allows you to inspect variable values, execution flow, and diagnose issues.
To set a breakpoint, place the cursor on the code line where you want to pause and click the "Add Breakpoint" button on the editor toolbar (or press F9). You can also right-click on the code line and choose "Add Breakpoint" from the context menu.
Breakpoints are displayed as red circles next to the code line. When the program reaches the breakpoint, it pauses, and you can inspect variables and execution flow in the "Debug" tool window.
### 4.2 Step-by-Step Debugging
Step-by-step debugging allows you to execute code line by line and inspect variable values after each step. This is very useful for understanding the flow of code execution and diagnosing problems.
To step through the code, click the "Step Debug" button in the "Debug" tool window (or press F10). You can also use the "Step Over" and "Step Into" buttons to control the step-by-step debugging process.
### 4.3 Inspecting Variables
During debugging, you need to check variable values to understand the program's behavior. PyCharm offers several methods to inspect variables:
- **Variables window:** Displays the values of all variables in the current stack frame.
- **Expression window:** Allows you to evaluate arbitrary expressions and view their results.
- **Tool tips:** Hover the mouse over a variable to view its value.
You can use these tools to check variable values, understand the code's execution flow, and diagnose problems.
#### Code Example
```python
def sum_numbers(a, b):
"""
Sums two numbers.
Parameters:
a (int): First number.
b (int): Second number.
Returns:
int: The sum of the two numbers.
"""
return a + b
```
#### Logical Analysis
This code defines a function named `sum_numbers` that takes two integer parameters `a` and `b`, and returns their sum.
To debug this code, set a breakpoint on the `return` statement. When the program reaches the breakpoint, you can check the values of `a` and `b`, as well as the return value of the `sum_numbers` function, in the "Variables" window.
#### Argument Explanation
- `a`: The first number to be added.
- `b`: The second number to be added.
#### Return Value
- Returns the sum of `a` and `b`.
# 5. Advanced Features of PyCharm
**5.1 Code Completion**
PyCharm provides powerful code completion features that can automatically complete variable, function, class, and module names. This can greatly improve coding efficiency and reduce syntax errors.
To enable code completion, press `Ctrl` + `Space` (Windows/Linux) or `Cmd` + `Space` (macOS). PyCharm will display a list of suggestions that match the text you have typed. You can use arrow keys or the mouse to select the desired suggestion and then press `Enter` to insert it into the code.
PyCharm's code completion feature can also provide more advanced suggestions based on the file type you are editing and the context. For example, if you are editing a Python file, PyCharm will offer suggestions related to Python syntax and libraries.
**5.2 Code Refactoring**
PyCharm offers a range of code refactoring tools that can help you restructure your code for better readability and maintainability. These tools include:
- **Rename:** Rename variables, functions, classes, and modules.
- **Extract Method:** Extract a code block into a new method.
- **Inline Variable:** Replace a variable with its value.
- **Move:** Move a code block to another location.
To use code refactoring tools, right-click on the code element you want to refactor and select the corresponding refactoring option. PyCharm will perform the refactoring automatically and update all references in the code.
**5.3 Unit Testing**
PyCharm integrates with unit testing frameworks to help you easily write and run unit tests for your code. To create a unit test, right-click on a file or directory in your project and select **New** > **Python Test**.
PyCharm will create a new test file containing a test class. You can write test methods to test specific functionalities in your code. To run a test, right-click on the test file and select **Run** > **Python Test**.
PyCharm will run the test and display the results. If any tests fail, PyCharm will highlight the failing tests and provide error messages.
### Advantages of Unit Testing
Unit testing offers several benefits, including:
- **Improved code quality:** Unit tests help you identify errors and defects in your code.
- **Increased confidence:** Unit tests give you confidence that your code will work correctly in most cases.
- **Enhanced maintainability:** Unit tests help you maintain the code, as you can refactor it easily without worrying about breaking existing functionalities.
# ***mon Problems with PyCharm**
### 6.1 Unable to Install PyCharm
**Problem Description:**
An error message appears or the installer fails to complete while attempting to install PyCharm.
**Possible Causes:**
- The system does not meet the minimum requirements.
- The downloaded file is corrupted.
- Antivirus software interference.
- Permission issues.
**Solutions:**
***Check system requirements:** Ensure your system meets PyCharm's minimum requirements.
***Redownload the installer:** Download the latest version of the installer from the official website.
***Disable antivirus software:** Temporarily disable antivirus software during installation.
***Run the installer as administrator:** Right-click on the installer and choose "Run as administrator."
### 6.2 PyCharm Won't Start
**Problem Description:**
After installing PyCharm, the application fails to launch.
**Possible Causes:**
* Installation is corrupted.
* Missing dependencies.
* Incorrect environment variable configuration.
**Solutions:**
***Reinstall PyCharm:** Uninstall PyCharm and reinstall the latest version.
***Install dependencies:** Ensure all dependencies required by PyCharm, such as Java and Python, are installed.
***Check environment variables:** Verify that the PyCharm installation directory is included in the PATH environment variable.
0
0
相关推荐






