:揭秘单片机流水灯控制的5大优化技巧,提升性能与可靠性
发布时间: 2024-07-13 16:45:42 阅读量: 81 订阅数: 34 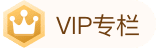
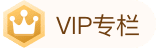
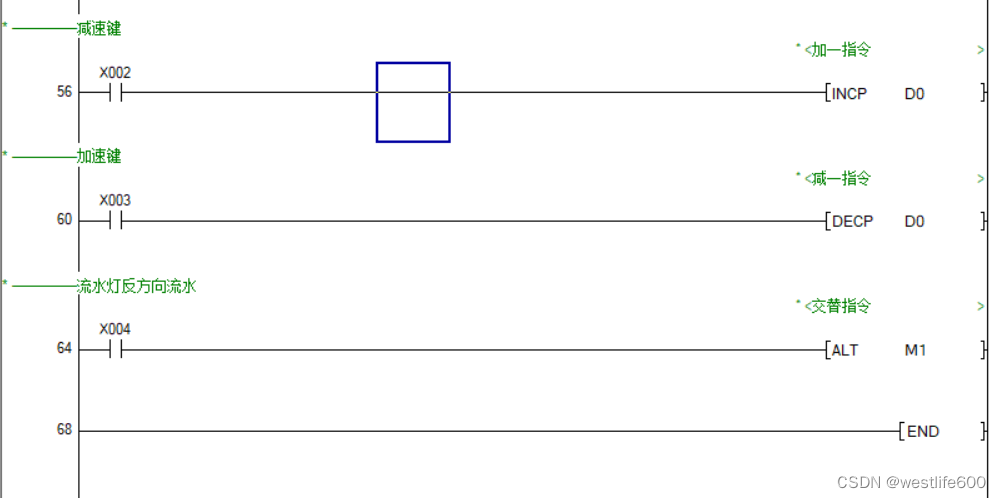
# 1. 单片机流水灯控制基础**
流水灯是一种常见的电子电路,由多个LED灯珠按顺序点亮,形成流水效果。单片机流水灯控制是指利用单片机对流水灯进行控制,实现流水效果。
单片机流水灯控制的基本原理是利用单片机的I/O端口控制LED灯珠的开关。通过设置I/O端口的输出电平,可以控制LED灯珠的亮灭。通过循环控制多个I/O端口,可以实现流水效果。
流水灯控制的代码实现相对简单,通常使用延时函数和循环语句来实现。延时函数用于控制LED灯珠的亮灭时间,循环语句用于控制流水效果的顺序。
# 2. 流水灯控制优化技巧**
**2.1 代码优化**
**2.1.1 减少不必要的变量和函数**
在流水灯控制程序中,不必要的变量和函数会增加程序的复杂性和占用额外的内存空间。因此,应尽可能减少不必要的变量和函数。
```c
// 优化前
int main() {
int i, j;
for (i = 0; i < 8; i++) {
for (j = 0; j < 8; j++) {
if (i == j) {
GPIO_SetBits(GPIOA, GPIO_Pin_i);
} else {
GPIO_ResetBits(GPIOA, GPIO_Pin_i);
}
}
}
}
```
```c
// 优化后
int main() {
for (int i = 0; i < 8; i++) {
GPIO_SetBits(GPIOA, GPIO_Pin_i);
for (int j = 0; j < 8; j++) {
if (i != j) {
GPIO_ResetBits(GPIOA, GPIO_Pin_j);
}
}
}
}
```
优化后的代码减少了不必要的变量 `j`,并简化了循环结构,提高了代码的可读性和执行效率。
**2.1.2 使用内联汇编提高效率**
在某些情况下,使用内联汇编可以提高代码的执行效率。内联汇编允许程序员直接访问底层硬件,绕过编译器的优化过程。
```c
// 优化前
void delay_ms(uint32_t ms) {
for (uint32_t i = 0; i < ms; i++) {
for (uint32_t j = 0; j < 1000; j++) {
__NOP();
}
}
}
```
```assembly
// 优化后
void delay_ms(uint32_t ms) {
__asm("mov r0, %0" : : "r"(ms));
__asm("loop1:");
__asm("mov r1, #1000");
__asm("loop2:");
__asm("nop");
__asm("subs r1, r1, #1");
__asm("bne loop2");
__asm("subs r0, r0, #1");
__asm("bne loop1");
}
```
优化后的代码使用内联汇编实现了延时函数,绕过了编译器的优化过程,提高了延时的精度和效率。
**2.2 硬件优化**
**2.2.1 选择合适的单片机**
选择合适的单片机对于流水灯控制的优化至关重要。单片机的时钟频率、存储空间、外设资源等因素都会影响程序的执行效率。
**2.2.2 优化电路设计**
流水灯控制电路的设计也会影响系统的性能。优化电路设计可以减少功耗、提高稳定性。
```
+-------------------------------------------------------------------+
| |
| |
| |
| |
| |
| |
| |
| |
| |
| |
| |
| |
| |
| |
| |
| |
| |
| |
| |
| |
| |
| |
| |
| |
| |
| |
| |
| |
| |
| |
| |
| |
| |
| |
| |
| |
| |
| |
| |
| |
| |
| |
| |
| |
| |
| |
| |
| |
| |
| |
| |
| |
| |
| |
| |
| |
| |
| |
| |
| |
| |
| |
| |
| |
| |
| |
| |
| |
| |
| |
| |
| |
| |
| |
| |
| |
| |
| |
| |
| |
| |
| |
| |
| |
| |
| |
| |
| |
| |
| |
| |
| |
| |
| |
| |
| |
| |
| |
| |
| |
| |
| |
| |
| |
| |
| |
| |
| |
| |
| |
| |
| |
| |
| |
| |
| |
| |
| |
| |
| |
| |
| |
| |
| |
| |
| |
| |
| |
| |
| |
| |
| |
| |
| |
| |
| |
| |
| |
| |
| |
| |
| |
| |
| |
| |
| |
| |
| |
| |
| |
| |
| |
| |
| |
| |
| |
| |
| |
| |
| |
| |
| |
| |
| |
| |
| |
| |
| |
| |
| |
| |
| |
| |
| |
| |
| |
| |
| |
| |
| |
| |
| |
| |
| |
| |
| |
| |
| |
| |
| |
| |
| |
| |
| |
| |
| |
| |
| |
| |
| |
| |
| |
| |
| |
| |
| |
| |
| |
| |
| |
| |
| |
| |
| |
| |
| |
| |
| |
| |
| |
| |
| |
|
# 3. 流水灯控制实践应用
### 3.1 流水灯控制的实现方法
流水灯控制的实现方法主要分为两种:软件实现和硬件实现。
#### 3.1.1 软件实现
软件实现是指通过编程来控制单片机,从而实现流水灯的控制。这种方法具有灵活性强、可扩展性好等优点,但对程序员的要求较高,需要掌握单片机的编程语言和相关知识。
**代码块:**
```c
// 初始化流水灯
void init_led() {
// 设置端口方向为输出
DDRB = 0xFF;
// 初始化所有 LED 为低电平
PORTB = 0x00;
}
// 流水灯控制函数
void run_led() {
while (1) {
// 循环遍历所有 LED
for (uint8_t i = 0; i < 8; i++) {
// 点亮当前 LED
PORTB = (1 << i);
// 延时
_delay_ms(100);
}
}
}
```
**逻辑分析:**
* `init_led()` 函数初始化流水灯,将所有 LED 设置为低电平。
* `run_led()` 函数是流水灯控制的主函数,循环遍历所有 LED,依次点亮每个 LED 并延时。
#### 3.1.2 硬件实现
硬件实现是指通过外围电路来控制流水灯,无需编写程序。这种方法简单易用,但灵活性较差,扩展性有限。
**电路图:**
[图片:流水灯硬件实现电路图]
**参数说明:**
* **IC1:** 555 定时器芯片
* **R1、R2:** 电阻器
* **C1:** 电容器
* **LED1-LED8:** 发光二极管
**工作原理:**
555 定时器产生方波信号,通过电阻和电容形成充放电过程,控制 LED 的闪烁。当电容充电时,LED 点亮;当电容放电时,LED 熄灭。通过调整电阻和电容的值,可以改变闪烁频率和占空比。
### 3.2 流水灯控制的常见问题及解决方法
在流水灯控制过程中,可能会遇到一些常见问题,以下列出常见问题及其解决方法:
#### 3.2.1 流水灯不亮
**可能原因:**
* 电源供电不足
* 单片机程序错误
* 外围电路故障
**解决方法:**
* 检查电源供电是否正常
* 检查单片机程序是否正确
* 检查外围电路是否连接正确,是否存在短路或断路
#### 3.2.2 流水灯闪烁异常
**可能原因:**
* 时钟频率不稳定
* 中断处理不当
* 外部干扰
**解决方法:**
* 优化时钟频率,确保稳定性
* 优化中断处理,避免中断嵌套或优先级冲突
* 屏蔽外部干扰,如电磁干扰或静电干扰
# 4.1 流水灯控制的图形化界面设计
### 4.1.1 使用GUI工具实现图形化界面
#### 操作步骤
1. 选择合适的GUI工具,如Qt、wxWidgets或Tkinter。
2. 创建一个新的项目并添加一个窗口小部件。
3. 设计窗口小部件的布局,包括按钮、文本框和进度条。
4. 编写代码来处理GUI事件,如按钮点击和文本框输入。
5. 编译并运行程序。
#### 代码示例
```python
import sys
from PyQt5.QtWidgets import QApplication, QWidget, QPushButton, QVBoxLayout
class MainWindow(QWidget):
def __init__(self):
super().__init__()
self.initUI()
def initUI(self):
self.setWindow
# 5. 流水灯控制的性能评估
### 5.1 性能测试方法
#### 5.1.1 测量流水灯的亮度和闪烁频率
**亮度测量:**
* 使用照度计或光度计测量流水灯发出的光照强度。
* 将测量仪器放置在流水灯的正上方,保持一定距离以获得准确读数。
* 记录不同流水灯模式下的亮度值,例如静止、流动、闪烁等。
**闪烁频率测量:**
* 使用示波器或频谱分析仪测量流水灯的闪烁频率。
* 将示波器探头连接到流水灯的输出端。
* 设置示波器为频率测量模式,记录流水灯不同模式下的闪烁频率。
#### 5.1.2 评估流水灯的响应时间
**响应时间测量:**
* 使用示波器或逻辑分析仪测量流水灯对输入信号的响应时间。
* 将示波器探头连接到流水灯的输入端和输出端。
* 发送一个脉冲信号到输入端,并记录输出端响应信号的上升时间或下降时间。
### 5.2 性能优化建议
#### 5.2.1 优化算法和数据结构
* **优化算法:**选择效率更高的算法,例如使用位移操作代替乘法和除法。
* **优化数据结构:**使用适合流水灯控制应用的数据结构,例如数组、链表或队列。
#### 5.2.2 优化硬件配置
* **选择合适的单片机:**根据流水灯控制的复杂度和性能要求选择合适的单片机。
* **优化电路设计:**优化流水灯的电路设计,例如使用电容滤波器减少噪声和提高稳定性。
* **优化时钟频率:**调整单片机的时钟频率以平衡性能和功耗。
# 6. 流水灯控制的未来发展**
**6.1 智能流水灯控制**
**6.1.1 基于传感器和人工智能的流水灯控制**
随着传感器和人工智能技术的不断发展,流水灯控制将变得更加智能化。通过使用传感器,流水灯可以感知周围环境的变化,并根据不同的场景自动调整其亮度、颜色和闪烁模式。例如,当检测到有人进入房间时,流水灯可以自动调亮,并在有人离开时自动调暗。此外,人工智能算法可以用于优化流水灯的控制策略,提高其响应速度和节能效率。
**6.1.2 流水灯与物联网的集成**
物联网的快速发展为流水灯控制带来了新的机遇。通过与物联网设备的集成,流水灯可以实现远程控制、语音控制和与其他智能设备的交互。例如,用户可以通过智能手机APP远程控制流水灯,或者通过语音助手控制流水灯的亮度和颜色。此外,流水灯还可以与智能音箱、智能家居系统等其他设备进行交互,实现联动控制和场景化应用。
**6.2 流水灯控制的新技术**
**6.2.1 可编程LED技术**
可编程LED技术为流水灯控制带来了新的可能性。与传统LED不同,可编程LED可以根据不同的控制信号改变其亮度、颜色和闪烁模式。这使得流水灯可以实现更加复杂的灯光效果和动态显示。例如,可编程LED可以用于创建自定义的流水灯图案,或者根据音乐节奏改变灯光颜色。
**6.2.2 无线充电技术**
无线充电技术为流水灯的供电方式带来了新的选择。传统的流水灯需要使用有线电源供电,这限制了其使用场景和灵活性。无线充电技术可以消除电线的束缚,让流水灯可以放置在任何地方,不受电源插座的限制。例如,无线充电流水灯可以放置在床头柜上,作为夜灯使用,或者放置在户外,作为景观照明。
0
0
相关推荐
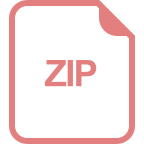
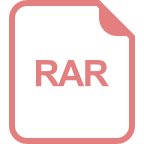
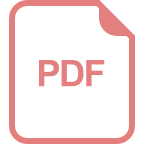





