Java算法:深入研究快速排序算法的实现及性能优化
发布时间: 2024-04-04 00:06:40 阅读量: 53 订阅数: 24 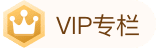
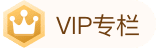
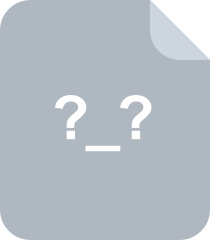
快速排序算法的java实现

# 1. 快速排序算法概述
快速排序(Quicksort)是一种高效的排序算法,广泛应用于各种编程语言和系统中。它采用分治法策略,在平均情况下具有较好的时间复杂度,是一种不稳定的排序方法。
### 1.1 快速排序算法原理简介
快速排序的原理是通过一趟排序将待排序的数据分割成独立的两部分,其中一部分的所有数据都比另一部分小,然后递归地对这两部分数据进行排序。具体步骤如下:
1. 选择一个基准元素(pivot)。
2. 将小于基准的元素放在基准元素的左边,大于基准的元素放在右边。
3. 递归地对左右两部分进行排序。
### 1.2 快速排序算法的优缺点分析
#### 优点:
- 高效:平均时间复杂度为O(nlogn)。
- 原地排序:不需要额外的存储空间。
- 算法简单:易于实现。
#### 缺点:
- 不稳定性:相等元素经过排序可能改变相对位置。
- 最坏情况下性能较差:当数据已经有序时,时间复杂度为O(n^2)。
### 1.3 快速排序算法的时间复杂度分析
- 最佳情况:O(nlogn)
- 最坏情况:O(n^2)
- 平均情况:O(nlogn)
快速排序的时间复杂度取决于基准值的选择和数据分布情况。在大多数情况下,快速排序是一种高效的排序算法。
# 2. 基本快速排序算法实现
快速排序算法是一种经典的排序算法,其实现相对简单而且效率高。在这一章节中,我们将介绍基本的快速排序算法实现方法,并对其稳定性进行分析。
### 2.1 递归实现快速排序算法
递归是实现快速排序的常用方法之一。其基本思路是选择一个基准元素,将数组分割成两部分,小于基准的元素放在基准的左边,大于基准的元素放在右边,然后递归地对左右两部分进行排序。
```java
public class QuickSort {
public static void quickSort(int[] arr, int low, int high) {
if (low < high) {
int pivot = partition(arr, low, high);
quickSort(arr, low, pivot - 1);
quickSort(arr, pivot + 1, high);
}
}
public static int partition(int[] arr, int low, int high) {
int pivot = arr[high];
int i = low - 1;
for (int j = low; j < high; j++) {
if (arr[j] < pivot) {
i++;
int temp = arr[i];
arr[i] = arr[j];
arr[j] = temp;
}
}
int temp = arr[i + 1];
arr[i + 1] = arr[high];
arr[high] = temp;
return i + 1;
}
public static void main(String[] args) {
int[] arr = {4, 2, 7, 5, 9, 1, 3};
quickSort(arr, 0, arr.length - 1);
for (int num : arr) {
System.out.print(num + " ");
}
}
}
```
**代码场景解释**:以上代码展示了递归实现快速排序的Java代码。首先选择数组最后一个元素作为基准,然后按照比基准小的元素放在左边,比基准大的元素放在右边的方式进行排序。
**代码注释**:代码中partition方法实现了元素的重新排列,将比基准小的元素移到左边,比基准大的元素移到右边。quickSort方法用于递归调用,直到排序完成。
**代码总结**:递归实现快速排序是一种简单而有效的排序算法,但需要注意在极端情况下可能出现性能问题。
### 2.2 迭代实现快速排序算法
除了递归实现外,我们也可以使用迭代的方式实现快速排序算法。迭代实现相对更加复杂,但可以避免递归带来的栈溢出问题。
```java
import java.util.Stack;
public class QuickSort {
public static void quickSort(int[] arr, int low, int high) {
Stack<Integer> stack = new Stack<>();
stack.push(low);
stack.push(high);
while (!stack.isEmpty()) {
high = stack.pop();
low = stack.pop();
int pivot = partition(arr, low, high);
if (pivot - 1 > low) {
stack.push(low);
stack.push(pivot - 1);
}
if (pivot + 1 < high) {
stack.push(pivot + 1);
stack.push(high);
}
}
}
public static int partition(int[] arr, int low, int high) {
int pivot = arr[high];
int i = low - 1;
for (int j = low; j < high; j++) {
if (arr[j] < pivot) {
i++;
int temp = arr[i];
arr[i] = arr[j];
arr[j] = temp;
}
}
int temp = arr[i + 1];
arr[i + 1] = arr[high];
arr[high] = temp;
return i + 1;
}
public static void mai
```
0
0
相关推荐





