设计模式的本质:深入剖析设计模式的原理和实践
发布时间: 2024-08-26 09:54:37 阅读量: 23 订阅数: 30 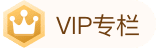
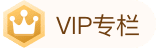
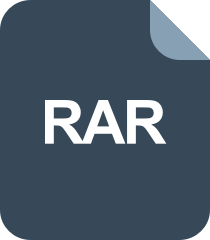
Spring技术内幕:深入解析Spring架构与设计原理(第1部分)
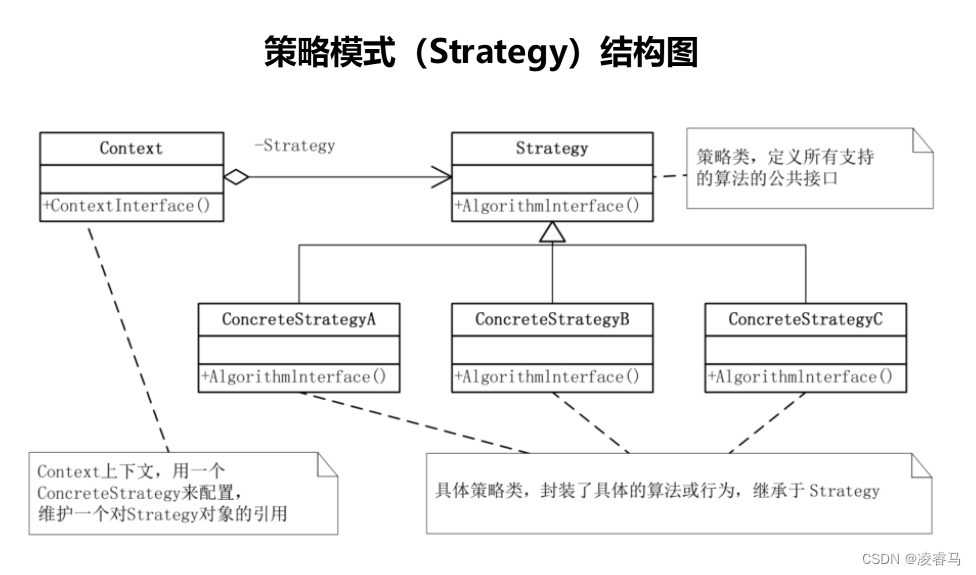
# 1. 设计模式的理论基础**
设计模式是软件开发中经过验证的、可重用的解决方案,用于解决常见的设计问题。它们提供了一种通用语言,使开发人员能够有效地沟通和理解复杂系统。设计模式的本质在于抽象和重用,通过识别和提取软件系统中可重复的结构和行为,它们可以帮助开发人员创建更灵活、更可维护的代码。
设计模式的理论基础建立在面向对象编程 (OOP) 原则之上。OOP 强调封装、继承和多态性,这些概念为设计模式的创建和应用提供了基础。通过利用 OOP 原则,设计模式可以帮助开发人员构建松耦合、高内聚的系统,这些系统易于理解、修改和扩展。
# 2. 设计模式的分类和应用
### 2.1 创建型模式
创建型模式用于创建对象,并控制对象的创建过程。它们提供了一种灵活的方式来创建对象,而无需指定具体的创建类。
#### 2.1.1 单例模式
单例模式确保一个类只有一个实例,并提供全局访问点。它用于创建单例对象,例如缓存系统或日志记录器。
**代码块:**
```python
class Singleton:
_instance = None
def __new__(cls, *args, **kwargs):
if cls._instance is None:
cls._instance = super().__new__(cls, *args, **kwargs)
return cls._instance
```
**逻辑分析:**
* `__new__`方法被重写,以控制对象创建过程。
* 如果`_instance`属性为`None`,则创建一个新实例并将其存储在`_instance`中。
* 否则,返回`_instance`属性中的现有实例。
**参数说明:**
* `*args`和`**kwargs`:传递给构造函数的参数。
#### 2.1.2 工厂模式
工厂模式提供了一个创建对象的接口,而不必指定具体的创建类。它允许应用程序在不修改创建逻辑的情况下创建不同类型的对象。
**代码块:**
```python
class ShapeFactory:
def create_shape(self, shape_type):
if shape_type == "Circle":
return Circle()
elif shape_type == "Square":
return Square()
else:
raise ValueError("Invalid shape type")
class Circle:
def draw(self):
print("Drawing a circle")
class Square:
def draw(self):
print("Drawing a square")
```
**逻辑分析:**
* `ShapeFactory`类定义了一个`create_shape`方法,用于创建不同类型的形状。
* `create_shape`方法根据`shape_type`参数创建一个特定的形状对象。
* `Circle`和`Square`类是具体的形状类,实现`draw`方法。
**参数说明:**
* `shape_type`:要创建的形状类型。
### 2.2 结构型模式
结构型模式用于组织和组合对象,以形成更大的结构。它们提供了一种方式来表示对象之间的关系,并控制对象之间的交互。
#### 2.2.1 适配器模式
适配器模式将一个类的接口转换为另一个类的接口,从而使它们可以一起工作。它允许不兼容的类协同工作,而无需修改它们的源代码。
**代码块:**
```python
class Target:
def request(self):
print("Target request")
class Adaptee:
def specific_request(self):
print("Adaptee specific request")
class Adapter(Target):
def __init__(self, adaptee):
self.adaptee = adaptee
def request(self):
self.adaptee.specific_request()
```
**逻辑分析:**
* `Target`类定义了目标接口。
* `Adaptee`类定义了不兼容的接口。
* `Adapter`类将`Adaptee`类适配到`Target`接口。
* `Adapter`类的`request`方法调用`Adaptee`类的`specific_request`方法。
**参数说明:**
* `adaptee`:要适配的`Adaptee`对象。
#### 2.2.2 代理模式
代理模式为另一个对象提供一个代理或替身,以便控制对该对象的访问。它可以用于保护对象、提供额外的功能或优化性能。
**代码块:**
```python
class RealSubject:
def request(self):
print("Real subject request")
class Proxy:
def __init__(self, real_subject):
self.real_subject = real_subject
def request(self):
print("Proxy request")
self.real_subject.request()
```
**逻辑分析:**
* `RealSubject`类定义了实际对象。
* `Proxy`类是一个代理,为`RealSubject`对象提供了一个接口。
* `Proxy`类的`request`方法调用`RealSubject`类的`request`方法。
**参数说明:**
* `real_subject`:要代理的`RealSubject`对象。
### 2.3 行为型模式
行为型模式用于定义对象之间的通信和交互。它们提供了一种方式来组织和协调对象的行为,以实现特定的设计目标。
#### 2.3.1 策略模式
策略模式定义了一组算法,并允许动态地选择和切换这些算法。它提供了一种方式来更改算法,而无需修改客户端代码。
**代码块:**
```python
class Context:
def __init__(self, strategy):
self.strategy = strategy
def execute_strategy(self):
self.strategy.execute()
class Strategy:
def execute(self):
pass
class ConcreteStrategyA(Strategy):
def execute(self):
print("Concrete strategy A")
class ConcreteStrategyB(Strategy):
def execute(self):
print("Concrete strategy B")
```
**逻辑分析:**
* `Context`类持有`Strategy`对象并调用其`execute`方法。
* `Strategy`类定义了算法的接口。
* `ConcreteStrategyA`和`ConcreteStrategyB`类是具体的算法实现。
**参数说明:**
* `strategy`:要使用的`Strategy`对象。
#### 2.3.2 观察者模式
观察者模式定义了一种一对多的依赖关系,其中一个对象(主题)的状态变化会通知所有依赖于它的对象(观察者)。它提供了一种方式来松散耦合对象,并允许它们响应主题状态的变化。
**代码块:**
```python
class Subject:
def __init__(self):
self.observers = []
def attach(self, observer):
self.observers.append(observer)
def detach(self, observer):
self.observers.remove(observer)
def notify(self):
for observer in self.observers:
observer.update()
class Observer:
def update(self):
pass
class ConcreteObserverA(Observer):
def update(self):
print("Concrete observer A")
class ConcreteObserverB(Observer):
def update(self):
print("Concrete observer B")
```
**逻辑分析:**
* `Subject`类管理`Observer`对象并通知它们状态的变化。
* `Observer`类定义了更新接口。
* `ConcreteObserverA`和`ConcreteObserverB`类是具体的观察者实现。
**参数说明:**
* `observer`:要附加或分离的`Observer`对象。
# 3. 设计模式的实践应用**
**3.1 软件开发中的设计模式应用**
**3.1.1 单例模式在缓存系统的应用**
单例模式是一种创建型设计模式,它确保一个类只有一个实例,并提供一个全局访问点。在缓存系统中,单例模式可以用来管理缓存对象,确保缓存对象在整个系统中只有一份。
```python
class Cache:
__instance = None
def __init__(self):
if Cache.__instance is not None:
raise Exception("Singleton class cannot be instantiated more than once.")
Cache.__instance = self
self.cache = {}
@classmethod
def get_instance(cls):
if Cache.__instance is None:
Cache.__instance = Cache()
return Cache.__instance
def get(self, key):
return self.cache.get(key)
def set(self, key, value):
self.cache[key] = value
```
**逻辑分析:**
* `__instance` 变量用于存储单例类的实例。
* `__init__` 方法检查是否已经存在实例,如果存在则抛出异常。
* `get_instance` 方法返回单例类的实例,如果实例不存在则创建它。
* `get` 和 `set` 方法用于访问和修改缓存。
**3.1.2 工厂模式在对象创建中的应用**
工厂模式是一种创建型设计模式,它将对象的创建过程与对象的表示分离。在对象创建中,工厂模式可以用来创建不同类型的对象,而无需指定具体的类。
```python
class ShapeFactory:
def create_shape(self, shape_type):
if shape_type == "circle":
return Circle()
elif shape_type == "square":
return Square()
elif shape_type == "rectangle":
return Rectangle()
else:
raise Exception("Invalid shape type.")
class Circle:
def draw(self):
print("Drawing a circle.")
class Square:
def draw(self):
print("Drawing a square.")
class Rectangle:
def draw(self):
print("Drawing a rectangle.")
```
**逻辑分析:**
* `ShapeFactory` 类负责创建不同的形状对象。
* `create_shape` 方法根据给定的形状类型返回一个形状对象。
* `Circle`、`Square` 和 `Rectangle` 类是具体形状类的实现。
* `draw` 方法用于绘制形状。
**3.2 系统管理中的设计模式应用**
**3.2.1 适配器模式在跨平台通信中的应用**
适配器模式是一种结构型设计模式,它将一个类的接口转换成另一个类所期望的接口。在跨平台通信中,适配器模式可以用来将不同平台的通信协议适配到一个统一的接口。
```python
class WindowsAdapter:
def __init__(self, windows_object):
self.windows_object = windows_object
def send_message(self, message):
self.windows_object.send_message(message)
class LinuxAdapter:
def __init__(self, linux_object):
self.linux_object = linux_object
def send_message(self, message):
self.linux_object.send_message(message)
class CommunicationManager:
def __init__(self, adapter):
self.adapter = adapter
def send_message(self, message):
self.adapter.send_message(message)
```
**逻辑分析:**
* `WindowsAdapter` 和 `LinuxAdapter` 类是适配器类,它们将不同平台的通信协议适配到统一的 `send_message` 方法。
* `CommunicationManager` 类使用适配器类来发送消息,而无需关心底层通信协议。
**3.2.2 代理模式在安全代理中的应用**
代理模式是一种结构型设计模式,它为另一个对象提供一个代理或替代。在安全代理中,代理模式可以用来控制对敏感对象的访问。
```python
class SecureProxy:
def __init__(self, real_object, user):
self.real_object = real_object
self.user = user
def has_access(self):
return self.user.has_access()
def operate(self):
if self.has_access():
self.real_object.operate()
else:
print("Access denied.")
class RealObject:
def operate(self):
print("Performing operation.")
```
**逻辑分析:**
* `SecureProxy` 类是代理类,它控制对 `RealObject` 类的访问。
* `has_access` 方法检查用户是否具有访问权限。
* `operate` 方法调用 `has_access` 方法来检查权限,然后执行操作或拒绝访问。
# 4. 设计模式的进阶应用
### 4.1 设计模式在分布式系统中的应用
分布式系统是近年来兴起的一种软件架构,它将一个应用程序分解成多个独立的组件,这些组件分布在不同的计算机上。分布式系统具有高可用性、可扩展性和容错性等优点,但同时也带来了新的挑战,如网络延迟、数据一致性和并发控制等。
设计模式可以帮助我们解决分布式系统中遇到的这些挑战。例如:
#### 4.1.1 策略模式在负载均衡中的应用
负载均衡是一种将请求分发到多个服务器上的技术,以提高系统的性能和可用性。策略模式可以用来实现负载均衡,通过定义不同的负载均衡算法(如轮询、加权轮询、最少连接数等)并动态地切换这些算法来适应不同的系统负载情况。
```java
public class LoadBalancer {
private List<Server> servers;
private LoadBalancingStrategy strategy;
public LoadBalancer(List<Server> servers, LoadBalancingStrategy strategy) {
this.servers = servers;
this.strategy = strategy;
}
public Server getServer() {
return strategy.getServer(servers);
}
// ...
}
public interface LoadBalancingStrategy {
Server getServer(List<Server> servers);
// ...
}
public class RoundRobinStrategy implements LoadBalancingStrategy {
private int index = 0;
@Override
public Server getServer(List<Server> servers) {
Server server = servers.get(index);
index = (index + 1) % servers.size();
return server;
}
// ...
}
```
在这个例子中,`LoadBalancer`类负责将请求分发到不同的服务器上,它使用`LoadBalancingStrategy`接口来定义不同的负载均衡算法。`RoundRobinStrategy`类实现了轮询算法,它通过循环遍历服务器列表来选择服务器。
#### 4.1.2 观察者模式在事件驱动的架构中的应用
事件驱动的架构是一种软件设计模式,它使用事件来触发和处理系统中的操作。在分布式系统中,事件驱动的架构可以用来实现异步通信、松耦合和可扩展性。
观察者模式可以用来实现事件驱动的架构。通过定义一个`Event`类来表示事件,并定义一个`EventPublisher`类来发布事件,我们可以让多个`EventSubscriber`类订阅这些事件并做出响应。
```java
public class Event {
private String type;
private Object data;
// ...
}
public class EventPublisher {
private List<EventSubscriber> subscribers;
public EventPublisher() {
this.subscribers = new ArrayList<>();
}
public void publishEvent(Event event) {
for (EventSubscriber subscriber : subscribers) {
subscriber.onEvent(event);
}
}
// ...
}
public interface EventSubscriber {
void onEvent(Event event);
// ...
}
public class ConsoleEventSubscriber implements EventSubscriber {
@Override
public void onEvent(Event event) {
System.out.println("Received event: " + event.getType());
}
// ...
}
```
在这个例子中,`Event`类表示事件,`EventPublisher`类负责发布事件,`EventSubscriber`接口定义了订阅事件并做出响应的方法。`ConsoleEventSubscriber`类实现了`EventSubscriber`接口,它打印出收到的事件类型。
# 5. 设计模式的最佳实践**
**5.1 设计模式的选择和应用原则**
在选择和应用设计模式时,应遵循以下原则:
* **必要性原则:**仅在确实需要时才使用设计模式,避免过度设计。
* **合适性原则:**选择与具体问题域和需求最匹配的设计模式。
* **可组合性原则:**设计模式可以组合使用,以解决更复杂的问题。
* **可扩展性原则:**设计模式应易于扩展和维护,以适应未来的需求变化。
* **性能原则:**考虑设计模式对系统性能的影响,避免引入不必要的开销。
**5.2 设计模式的滥用和陷阱**
设计模式的滥用会导致以下陷阱:
* **过度设计:**过度使用设计模式,导致代码复杂度增加和维护成本提高。
* **错误应用:**不恰当地应用设计模式,导致系统出现问题或性能下降。
* **设计模式迷信:**盲目依赖设计模式,而不考虑具体场景的实际需求。
* **代码重复:**不同类中的相同设计模式实现,导致代码重复和维护困难。
* **可读性下降:**过度使用设计模式会降低代码的可读性和可理解性。
**5.3 设计模式的未来发展趋势**
设计模式的未来发展趋势包括:
* **面向服务的设计(SOA):**设计模式在SOA架构中扮演重要角色,促进服务之间的松耦合和可重用性。
* **云计算:**设计模式在云计算环境中得到广泛应用,以支持弹性、可扩展性和高可用性。
* **大数据:**设计模式在处理和分析大数据时至关重要,帮助解决数据分布、并行处理和可视化等挑战。
* **人工智能(AI):**设计模式在AI系统中发挥着作用,支持机器学习、深度学习和自然语言处理等功能。
* **微服务:**设计模式在微服务架构中得到广泛应用,以促进模块化、可扩展性和独立部署。
0
0
相关推荐
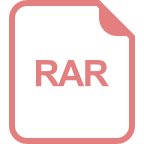
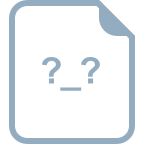





