VBA中的数据库连接:利用ADO技术实现与数据库的交互操作
发布时间: 2024-03-30 06:16:35 阅读量: 114 订阅数: 46 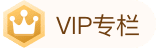
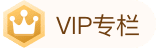
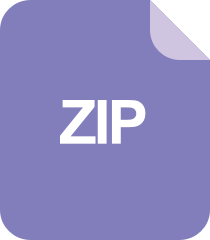
一个基于Qt Creator(qt,C++)实现中国象棋人机对战
# 1. 介绍
## 1.1 VBA在数据库操作中的重要性
在实际的工作中,VBA(Virtual Basic for Applications)作为一种常见的编程语言,广泛应用于各类办公软件中,如Excel、Access等。VBA在数据库操作中扮演着非常重要的角色,能够通过简洁的代码实现与数据库的交互操作,包括数据查询、更新、写入等功能。
## 1.2 ADO技术简介
在VBA中,与数据库进行交互的关键技术之一就是ADO(ActiveX Data Objects)技术。ADO是一种面向对象的数据库访问技术,它允许开发者使用统一的对象模型来访问各种类型的数据源,比如Access数据库、SQL Server、Oracle等,从而实现数据的连接、查询、更新等操作。通过ADO技术,VBA可以方便地操作数据库,提高工作效率和数据处理能力。
# 2. 数据库连接
在VBA中进行数据库操作时,建立数据库连接是至关重要的一步。通过正确的数据库连接方法,可以实现数据的读取、更新、写入等操作。下面将介绍VBA中建立数据库连接的基本步骤以及不同类型数据库的连接方法。
# 3. 数据读取与查询
在VBA中,我们可以利用ADO技术实现从数据库中查询数据的操作。通过执行SQL查询语句,我们可以获取满足条件的数据集合,从而实现数据的读取和查询操作。
#### 3.1 利用VBA实现从数据库中查询数据
在VBA中,我们可以通过以下步骤实现从数据库中查询数据:
```vba
Sub QueryData()
Dim conn As Object
Dim rs As Object
Dim sql As String
Dim result As String
' 创建数据库连接
Set conn = CreateObject("ADODB.Connection")
conn.Open "Provider=SQLOLEDB;Data Source=YourServer;Initial Catalog=YourDatabase;User ID=YourUserID;Password=YourPassword;"
' 执行查询SQL语句
sql = "SELECT * FROM YourTable WHERE Condition;"
Set rs = conn.Execute(sql)
' 遍历查询结果集
Do While Not rs.EOF
result = result & rs("Column1").Value & vbCrLf
rs.MoveNext
Loop
' 输出查询结果
MsgBox result
' 关闭连接
rs.Close
conn.Close
Set rs = Nothing
Set conn = Nothing
End Sub
```
#### 3.2 执行SQL语句的方法
除了查询数据外,我们还可以利用VBA执行SQL语句来实现数据库操作,例如插入、更新、删除数据等操作。通过执行SQL语句,我们可以直接对数据库中的数据进行修改,实现数据的更新和写入功能。
在VBA中执行SQL语句的方法与查询数据类似,只是SQL语句的内容和操作类型不同。我们可以通过以下代码示例来执行SQL语句:
```vba
Sub ExecuteSQL()
Dim conn As Object
Dim sql As String
' 创建数据库连接
Set conn = CreateObject("ADODB.Connection")
conn.Open "Provider=SQLOLEDB;Data Source=YourServer;Initial Catalog=YourDatabase;User ID=YourUserID;Password=YourPassword;"
' 执行SQL语句
sql = "INSERT INTO YourTable (Column1, Column2) VALUES ('Value1', 'Value2');"
conn.Execute sql
' 关闭连接
conn.Close
Set conn = Nothing
End Sub
```
通过以上方法,我们可以在VBA中实现数据的读取与查询,以及数据的更新与写入操作,从而实现与数据库的交互操作。
# 4. 数据更新与写入
在VBA中,我们可以利用ADO技术实现数据库中数据的更新和写入操作。这些操作对于维护数据库中的信息非常关键,下面我们将详细介绍如何使用VBA来实现数据更新与写入的功能。
#### 4.1 使用VBA更新数据库中的数据
数据更新操作可以通过执行SQL UPDATE语句来实现。在VBA中,通过创建一个Command对象,然后将UPDATE语句作为CommandText,再执行该Command,就可以实现数据库中数据的更新。
```vba
Sub UpdateData()
Dim conn As Object
Set conn = CreateObject("ADODB.Connection")
conn.Open "Provider=Microsoft.ACE.OLEDB.12.0;Data Source=C:\path\to\your\database.accdb;"
Dim cmd As Object
Set cmd = CreateObject("ADODB.Command")
cmd.ActiveConnection = conn
cmd.CommandType = adCmdText
cmd.CommandText = "UPDATE TableName SET ColumnName = NewValue WHERE Condition;"
cmd.Execute
conn.Close
Set conn = Nothing
End Sub
```
**代码说明:**
- `conn.Open`:打开数据库连接。
- `cmd.CommandType = adCmdText`:设置Command对象的类型为文本。
- `cmd.CommandText`:设置要执行的SQL UPDATE语句。
- `cmd.Execute`:执行更新操作。
#### 4.2 将VBA中的数据写入数据库
将VBA中的数据写入数据库通常需要先将数据保存到变量或数组中,然后通过INSERT语句将数据插入到数据库中。
```vba
Sub WriteDataToDB()
Dim conn As Object
Set conn = CreateObject("ADODB.Connection")
conn.Open "Provider=Microsoft.ACE.OLEDB.12.0;Data Source=C:\path\to\your\database.accdb;"
Dim cmd As Object
Set cmd = CreateObject("ADODB.Command")
cmd.ActiveConnection = conn
cmd.CommandType = adCmdText
cmd.CommandText = "INSERT INTO TableName (Column1, Column2) VALUES (Value1, Value2);"
cmd.Execute
conn.Close
Set conn = Nothing
End Sub
```
**代码说明:**
- `cmd.CommandText`:设置要执行的SQL INSERT语句,将数据插入到指定的表中。
通过以上代码示例,我们可以实现在VBA中进行数据更新和写入操作,从而实现与数据库的交互。
# 5. 事务处理
在VBA中,事务处理是一种非常重要的机制,可以确保数据库操作的安全性和稳定性。通过事务处理,我们可以将一系列数据库操作作为一个整体,要么全部执行成功,要么全部不执行,从而避免因为某些操作失败而导致数据不一致的情况。
#### 5.1 事务处理在VBA中的应用
在VBA中,我们可以利用ADO技术来实现事务处理。首先,我们需要通过`Connection`对象的`BeginTrans`方法开始一个事务;然后在事务中执行一系列数据库操作;最后根据执行结果,使用`CommitTrans`方法提交事务或者使用`RollbackTrans`方法回滚事务。
下面是一个简单的示例代码,演示了如何在VBA中使用事务处理:
```vba
Sub TransactionExample()
Dim con As ADODB.Connection
Dim cmd As ADODB.Command
Set con = New ADODB.Connection
con.ConnectionString = "Provider=Microsoft.ACE.OLEDB.12.0;Data Source=C:\path\to\your\database.accdb;"
con.Open
con.BeginTrans ' 开始事务
' 在事务中执行多条SQL语句
Set cmd = New ADODB.Command
Set cmd.ActiveConnection = con
cmd.CommandType = adCmdText
cmd.CommandText = "UPDATE Table1 SET Field1 = 'Value1' WHERE ID = 1"
cmd.Execute
cmd.CommandText = "INSERT INTO Table2 (Field1) VALUES ('Value2')"
cmd.Execute
' 根据需要提交或者回滚事务
If <some condition> Then
con.CommitTrans ' 提交事务
Else
con.RollbackTrans ' 回滚事务
End If
con.Close
Set con = Nothing
End Sub
```
#### 5.2 提高数据库操作的安全性和稳定性
通过使用事务处理机制,我们可以确保数据库操作的原子性,即要么全部成功,要么全部失败。这种方式有效地提高了数据库操作的安全性和稳定性,减少了数据异常的风险。在编写VBA程序时,合理利用事务处理是非常重要的,尤其在需要执行多个相关数据库操作时。
# 6. 错误处理与调试
在数据库操作过程中,错误处理和调试是非常重要的环节,可以帮助我们及时发现问题并解决。下面将介绍在VBA中如何处理数据库操作中的错误以及如何调试代码中的数据库连接问题。
1. **处理数据库操作中的错误**
在VBA中,我们可以使用`On Error Resume Next`和`Err`对象来处理数据库操作中的错误。示例代码如下:
```vba
Sub ErrorHandlingDemo()
On Error Resume Next
Dim conn As Object
Dim rs As Object
Set conn = CreateObject("ADODB.Connection")
conn.Open "Provider=Microsoft.ACE.OLEDB.12.0;Data Source=C:\path\to\your\database.accdb;"
If Err.Number <> 0 Then
MsgBox "Connection Error: " & Err.Description
Exit Sub
End If
Set rs = CreateObject("ADODB.Recordset")
rs.Open "SELECT * FROM Table1", conn
If Err.Number <> 0 Then
MsgBox "Query Error: " & Err.Description
Exit Sub
End If
' Continue with the rest of the code...
conn.Close
Set conn = Nothing
Set rs = Nothing
End Sub
```
上述代码演示了如何使用`On Error Resume Next`和`Err`对象来在数据库操作中捕获错误,并给出相应的提示信息。
2. **调试VBA代码中的数据库连接问题**
如果在数据库连接过程中出现问题,可以通过逐步调试的方式来排查。在VBA中,可以使用`Debug.Print`输出调试信息,以便查看变量的取值情况。示例代码如下:
```vba
Sub DebuggingDemo()
Dim conn As Object
Set conn = CreateObject("ADODB.Connection")
Debug.Print "Connection String: " & conn.ConnectionString
conn.Open "Provider=Microsoft.ACE.OLEDB.12.0;Data Source=C:\path\to\your\database.accdb;"
If conn.State = 1 Then
Debug.Print "Connection successful!"
Else
Debug.Print "Connection failed!"
End If
Set conn = Nothing
End Sub
```
通过逐行执行代码并查看输出的调试信息,可以帮助我们发现数据库连接问题的根本原因,并进行相应的修复。
以上便是在VBA中处理数据库操作中的错误和调试代码中的数据库连接问题的方法。在实际应用中,及时处理错误和调试代码将大大提高数据库操作的效率和准确性。
0
0
相关推荐

