【探索字符串匹配】:next算法变种及其多样应用案例研究
发布时间: 2024-09-10 03:49:59 阅读量: 75 订阅数: 41 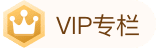
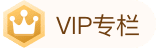
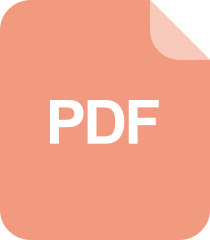
Python实现字符串匹配的KMP算法
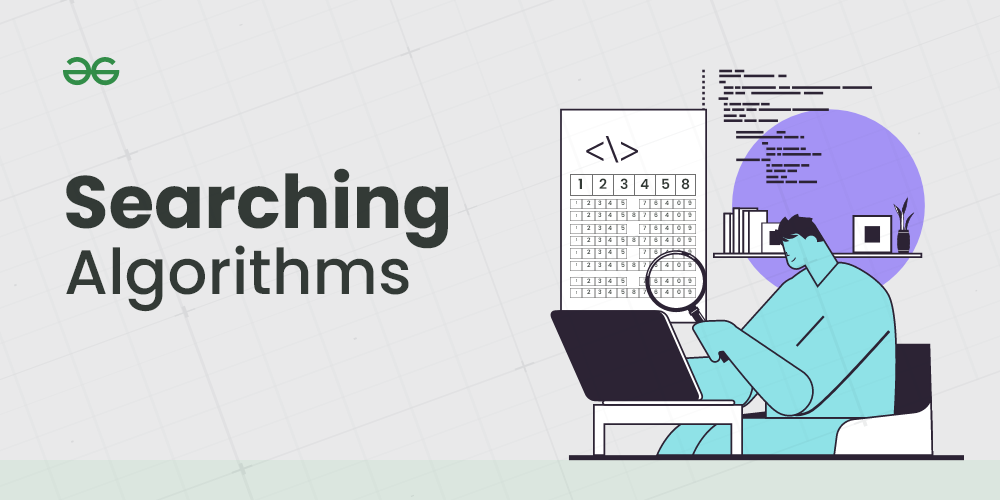
# 1. 字符串匹配与next算法概述
## 1.1 字符串匹配的重要性
在计算机科学中,字符串匹配是一种基础而重要的操作,它广泛应用于文本编辑、数据压缩、网络安全等多个领域。准确快速地进行字符串匹配,能够提升算法效率和用户体验。
## 1.2 字符串匹配的传统方法
传统的字符串匹配方法包括暴力匹配算法、KMP算法、BM算法等。每种算法都试图在不同的情况下优化匹配效率,但同时也伴随着复杂度与适用性的权衡。
## 1.3 next算法的提出
next算法是KMP算法的核心部分,它通过计算模式串的部分匹配表来避免不必要的比较,从而提高匹配效率。它的提出解决了部分匹配问题,并优化了回溯的效率。
在下一章节中,我们将深入探讨next算法的基本原理以及如何实现这一算法,让读者能够理解和掌握next算法在实际问题中的应用。
# 2. next算法的基本原理与实现
### 2.1 字符串匹配问题的提出
#### 2.1.1 字符串匹配的重要性
字符串匹配是计算机科学和信息技术中的一个核心问题,它涉及到数据检索、文本处理、信息抽取和网络安全等多个领域。尤其在大数据时代背景下,高效地进行字符串匹配能够提升搜索引擎、数据库查询、文本编辑软件等多种应用的性能,使得用户可以快速地从海量信息中检索到自己想要的内容。
#### 2.1.2 字符串匹配的传统方法
字符串匹配的经典方法包括暴力匹配算法(Brute Force)、Boyer-Moore算法、KMP算法(Knuth-Morris-Pratt)等。这些方法各有利弊,其中暴力匹配算法简单但效率低下;Boyer-Moore算法对特定情况下的字符串匹配效率较高,但最坏情况下的时间复杂度较高;而KMP算法通过预先计算部分匹配信息,有效避免了不必要的比较,成为了高效字符串匹配的代名词。
### 2.2 next算法的原理分析
#### 2.2.1 next数组的概念
next算法是在KMP算法中提出的一种优化字符串匹配效率的技术。它利用已经匹配的子串信息来确定接下来可能的匹配位置,其核心在于构造一个next数组,该数组用于在发生不匹配时指示模式串应当从哪个位置重新开始比较。next数组的每一个值,实际上表示在模式串中,当前位置之前的子串中,有多大长度的相同前缀后缀。
#### 2.2.2 next数组的计算方法
next数组的计算规则需要对模式串进行逐个字符的考察。对于模式串中的每个字符,都尝试找出它的最长相同前后缀长度,并将这个长度记录在next数组的对应位置。构建next数组时,需要用到一个辅助函数来判断在不匹配发生时,应从模式串的哪个位置重新开始匹配,这个位置就由next数组指出。
### 2.3 next算法的代码实现
#### 2.3.1 next数组的构建过程
构建next数组需要遍历模式串的每一个位置,并根据已有的next数组信息来计算当前字符对应位置的next值。构建next数组的过程分为两个步骤,首先是初始化部分,然后是填充数组,每一步都有详细的逻辑来保证算法的正确性。
```c
void computeNextArray(char* pattern, int patternLen, int* next) {
int len = 0; // 已匹配的前缀长度
next[0] = 0; // 初始化next[0]
for (int i = 1; i < patternLen; i++) {
while (len > 0 && pattern[i] != pattern[len]) {
// 当前字符不匹配时回溯
len = next[len - 1];
}
if (pattern[i] == pattern[len]) {
// 如果当前字符匹配,则增加已匹配的前缀长度
len++;
}
next[i] = len; // 保存当前字符对应位置的next值
}
}
```
#### 2.3.2 next算法匹配过程的代码实现
在拥有了next数组后,可以使用该数组来实现字符串的快速匹配过程。当模式串在主串中进行匹配并遇到不匹配的情况时,根据next数组提供的信息,模式串可以回溯到最有利的位置进行下一轮匹配尝试,从而避免从主串的下一个位置重新开始比较。
```c
int KMPStringMatch(char* text, char* pattern) {
int textLen = strlen(text);
int patternLen = strlen(pattern);
int* next = (int*)malloc(sizeof(int) * patternLen);
computeNextArray(pattern, patternLen, next);
int i = 0; // text的索引
int j = 0; // pattern的索引
while (i < textLen && j < patternLen) {
if (j == -1 || text[i] == pattern[j]) {
// 如果当前字符匹配成功或j为-1(即pattern从头开始匹配)
i++;
j++;
} else {
// 如果不匹配,则根据next数组回溯j的位置
j = next[j - 1];
}
}
free(next);
if (j == patternLen) {
return i - j; // 返回匹配的起始位置
} else {
return -1; // 没有匹配成功
}
}
```
以上代码块通过逐行解读的方式展示了next算法在字符串匹配过程中的应用。通过计算next数组并利用该数组进行优化的匹配过程,next算法大幅度减少了无效的比较次数,提高了字符串匹配的效率。
# 3. next算法的变种与优化
## 3.1 next算法的变种分析
字符串匹配作为计算机科学中的基础问题,其算法随着研究的深入不断演变,以适应日益复杂的实际应用需求。next算法的变种是为了提升算法效率、扩展应用范围而出现的改进算法。接下来,我们将详细探讨next算法的改进版nextval以及不同变种算法之间的比较。
### 3.1.1 next算法的改进版nextval
nextval算法是next算法的一个扩展,它通过引入新的定义来避免重复计算,从而加快匹配过程。在nextval算法中,引入了"nextval数组"的概念,该数组在构建过程中,对next数组中的某些值进行重新定义,以确保在遇到不匹配情况时,模式串能够进行更有效的位移。
```mermaid
graph LR
A[开始] --> B[初始化next数组]
B --> C{是否存在相同前后缀}
C -->|是| D[定义nextval值]
C -->|否| E[继续构建next数组]
D --> F[根据nextval进行模式串位移]
E --> F
F --> G[继续匹配或匹配失败]
```
代码实现nextval算法的关键部分如下:
```c
void computeNextval(char *pattern, int patternLength, int *nextval) {
int len = 0; // len表示当前最长前后缀长度
nextval[0] = -1;
int i = 1;
while (i < patternLength) {
if (pattern[i] == pattern[len]) {
len++;
nextval[i] = len;
i++;
} else {
if (len != 0) {
len = nextval[len]; // 依据nextval进行回溯
} else {
nextval[i] = 0;
i++;
}
}
}
}
```
### 3.1.2 不同变种算法的比较
变种算法的出现旨在解决next算法在某些特定场景下的局限性。例如,next算法在遇到模式串和文本串的部分匹配后,有可能产生不必要的回溯,导致效率降低。而nextval算法通过重新定义next数组,
0
0
相关推荐
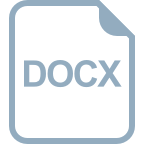
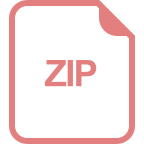
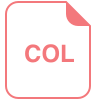
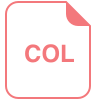
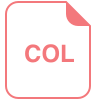
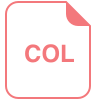
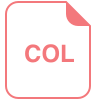
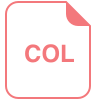