C++学生成绩管理系统:最佳实践与行业标准,打造专业级应用
发布时间: 2024-07-22 17:44:40 阅读量: 19 订阅数: 24 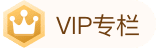
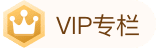
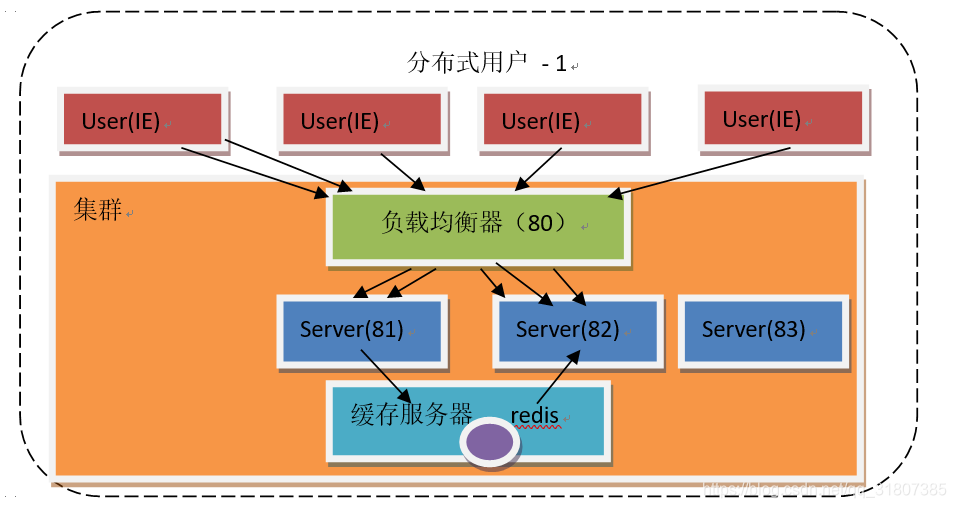
# 1. C++学生成绩管理系统概述
**1.1 系统背景**
学生成绩管理系统是教育机构中不可或缺的工具,用于存储、管理和分析学生成绩数据。它为教师、学生和管理人员提供了一个集中的平台,可以有效地管理学生成绩,跟踪学生进度,并做出明智的决策。
**1.2 系统目标**
本系统旨在创建一个功能齐全、用户友好的学生成绩管理系统,满足以下目标:
* 存储和管理学生个人信息、课程注册和成绩数据
* 提供灵活的查询和报告功能,以分析学生表现和识别趋势
* 确保数据的安全性和完整性,防止未经授权的访问和篡改
# 2. C++编程基础与数据结构
### 2.1 C++语法基础
#### 2.1.1 数据类型和变量
C++支持多种数据类型,包括基本类型(如int、float、char)和用户自定义类型(如类和结构)。变量用于存储数据,其类型决定了变量可以存储的数据类型。
```cpp
int age = 25; // 存储整数
float height = 1.75; // 存储浮点数
char grade = 'A'; // 存储单个字符
```
#### 2.1.2 运算符和表达式
运算符用于对变量和常量执行操作,表达式由运算符和操作数组成。C++支持算术运算符(+、-、*、/)、关系运算符(==、!=、<、>)、逻辑运算符(&&、||、!)等。
```cpp
int sum = a + b; // 算术运算符
bool is_equal = a == b; // 关系运算符
if (a > 0 && b < 10) { // 逻辑运算符
// 执行代码
}
```
#### 2.1.3 流程控制
流程控制语句用于控制程序的执行流程,包括条件语句(if-else)、循环语句(for、while、do-while)和跳转语句(break、continue、return)。
```cpp
if (a > 0) {
// 执行代码
} else {
// 执行代码
}
for (int i = 0; i < 10; i++) {
// 执行代码
}
```
### 2.2 数据结构
数据结构是组织和存储数据的有效方式,C++支持多种数据结构,包括数组、链表、栈、队列、树和图。
#### 2.2.1 数组和链表
数组是一种线性数据结构,其中元素按顺序存储在连续的内存块中。链表也是一种线性数据结构,但其元素存储在动态分配的内存块中,每个元素指向下一个元素。
```cpp
// 数组
int arr[] = {1, 2, 3, 4, 5};
// 链表
struct Node {
int data;
Node* next;
};
Node* head = new Node{1, nullptr};
```
#### 2.2.2 栈和队列
栈是一种后进先出(LIFO)数据结构,元素按后进先出的顺序存储。队列是一种先进先出(FIFO)数据结构,元素按先进先出的顺序存储。
```cpp
// 栈
stack<int> s;
s.push(1); // 入栈
s.pop(); // 出栈
// 队列
queue<int> q;
q.push(1); // 入队
q.pop(); // 出队
```
#### 2.2.3 树和图
树是一种层次结构的数据结构,其中每个节点最多有一个父节点和多个子节点。图是一种非线性数据结构,其中元素(顶点)通过边连接。
```cpp
// 树
struct Node {
int data;
vector<Node*> children;
};
Node* root = new Node{1, {}};
// 图
struct Graph {
map<int, vector<int>> adj_list;
};
Graph graph;
graph.adj_list[1] = {2, 3}; // 顶点1连接顶点2和3
```
# 3. 数据库管理与SQL语言
### 3.1 数据库概念和关系模型
#### 3.1.1 数据库管理系统(DBMS)
数据库管理系统(DBMS)是管理数据库和提供数据库访问的软件。它负责创建、维护和管理数据库,并提供用户和应用程序与数据库交互的接口。DBMS 的主要功能包括:
- 数据定义:创建和修改数据库结构,定义数据类型、表、索引和约束。
- 数据存储和管理:存储、组织和管理数据,确保数据完整性和一致性。
- 数据查询和检索:提供查询和检索数据的机制,支持复杂的查询和数据分析。
- 数据更新和修改:允许用户和应用程序更新和修改数据库中的数据,维护数据的最新性。
- 事务管理:管理数据库事务,确保数据一致性和完整性,防止并发访问导致数据不一致。
- 安全性和访问控制:提供安全机制和访问控制措施,保护数据免受未经授权的访问和修改。
#### 3.1.2 关系数据模型
关系数据模型是数据库中使用最广泛的数据模型。它将数据组织成称为表的关系,每个表包含具有相同结构的记录。关系模型基于以下概念:
- **表:**一个表是一个二维结构,包含行和列。每行代表一个记录,每列代表一个属性。
- **主键:**每个表都有一个主键,它是唯一标识表中每条记录的属性或属性组合。
- **外键:**外键是连接两个表之间的列。它引用另一个表中的主键,建立表之间的关系。
- **关系:**关系是两个或多个表之间的逻辑关联,基于外键连接。
### 3.2 SQL语言基础
结构化查询语言(SQL)是用于与关系数据库交互的标准化语言。它提供了创建、管理和查询数据库的命令。SQL 语句可以分为以下类别:
#### 3.2.1 数据定义语
0
0
相关推荐
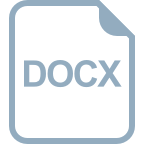
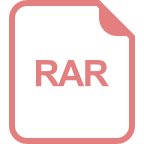
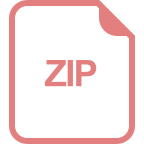





