Java设计模式之结构型模式原理解析与实例分析
发布时间: 2024-03-08 01:13:53 阅读量: 72 订阅数: 22 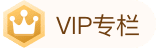
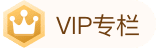
# 1. 引言
## 1.1 什么是设计模式?
设计模式是在软件设计中反复出现的问题的经验性解决方案。它们是针对某类常见问题的可重复使用的模板,可用于指导设计。
## 1.2 设计模式的作用
设计模式可以提供通用的解决方案,帮助开发人员解决设计中的常见问题,并提高代码的可维护性、可扩展性和可重用性。
## 1.3 结构型设计模式概述
结构型设计模式是关于对象组合形成更大的结构以满足实际需求的模式。在这些设计模式中,主要关心的是类和对象的组合。
# 2. 理解结构型设计模式
结构型设计模式主要关注如何组合类和对象以获得更大的结构。下面将介绍几种常见的结构型设计模式。
### 2.1 适配器模式
适配器模式允许接口不兼容的类能够相互合作。通过一个适配器,可以将一个类的接口转换成客户希望的另一个接口。这种模式可以通过类适配器和对象适配器来实现。
```java
// Java类适配器模式示例
interface Target {
void request();
}
class Adaptee {
public void specificRequest() {
System.out.println("Adaptee method is called");
}
}
class ClassAdapter extends Adaptee implements Target {
public void request() {
specificRequest();
}
}
```
### 2.2 装饰器模式
装饰器模式允许在不改变对象接口的情况下,动态地给对象添加新的职责。它是通过创建包装对象来实现的,包装对象中包含了原始对象。
```java
// Java装饰器模式示例
interface Component {
void operation();
}
class ConcreteComponent implements Component {
public void operation() {
System.out.println("ConcreteComponent operation is called");
}
}
class Decorator implements Component {
private Component component;
public Decorator(Component component) {
this.component = component;
}
public void operation() {
component.operation();
}
}
```
### 2.3 代理模式
代理模式通过创建一个代理对象来控制对原始对象的访问,可以在访问对象时做一些控制,如权限控制、延迟加载等。
```java
// Java代理模式示例
interface Subject {
void request();
}
class RealSubject implements Subject {
public void request() {
System.out.println("RealSubject request is called");
}
}
class Proxy implements Subject {
private RealSubject realSubject;
public void request() {
if (realSubject == null) {
realSubject = new RealSubject();
}
realSubject.request();
}
}
```
### 2.4 外观模式
外观模式为复杂的子系统提供一个简单的接口,客户端可以通过外观对象访问子系统的功能,而不需要了解子系统的具体实现。
```java
// Java外观模式示例
class SubSystemA {
public void methodA() {
System.out.println("SubSystemA methodA is called");
}
}
class SubSystemB {
public void methodB() {
System.out.println("SubSystemB methodB is called");
}
}
class Facade {
private SubSystemA subsystemA;
private SubSystemB subsystemB;
public Facade() {
subsystemA = new SubSystemA();
subsystemB = new SubSystemB();
}
public void operation() {
subsystemA.methodA();
subsystemB.methodB();
}
}
```
# 3. 结构型设计模式原理解析
在本章中,我们将深入理解结构型设计模式的原理,并为您详细解析每种设计模式的工作原理和适用场景。通过本章的学习,您将对结构型设计模式有更深入的了解,能够更好地应用到实际的软件开发中。
#### 3.1 设计模式的原理解析方法
在理解设计模式的原理时,我们将采用以下方法:
- 通过实际场景引入,帮助读者更好地理解设计模式的应用价值。
- 结合示例代码,通过具体的编程实例帮助读者理解设计模式的具体实现。
- 分析设计模式的优缺点,帮助读者在实际应用中做出合适的选择。
#### 3.2 结构型设计模式原理分析
结构型设计模式包括以下几种:
- 适配器模式
- 装饰器模式
- 代理模式
- 外观模式
- 桥接模式
- 组合模式
- 享元模式
我们将逐一对这些设计模式进行原理分析,并结合具体的代码示例进行说明。
#### 3.3 结构型设计模式的适用场景
在实际应用中,结构型设计模式有着各自的适用场景,我们将分析每种设计模式在何种情况下能够发挥最大的作用,帮助读者更好地理解设计模式的应用价值。
通过本章的学习,相信您将对结构型设计模式有更深入的认识,能够更好地将其运用到软件开发实践中。
# 4. 结构型设计模式在Java中的实现
在本章中,我们将深入探讨结构型设计模式在Java中的实现,并通过具体的代码示例来演示每种设计模式的应用场景、实现原理和效果。
#### 4.1 适配器模式的实例分析与代码示例
适配器模式是一种结构型设计模式,它允许将接口不兼容的类进行合作。适配器模式通常用于兼容不同接口的类之间的协作。
```java
// 适配器模式示例代码
// 目标接口
interface Target {
void request();
}
// 需要适配的类
class Adaptee {
public void specificRequest() {
System.out.println("Adaptee's specific request");
}
}
// 类适配器
class ClassAdapter extends Adaptee implements Target {
@Override
public void request() {
specificRequest();
}
}
// 对象适配器
class ObjectAdapter implements Target {
private Adaptee adaptee;
public ObjectAdapter(Adaptee adaptee) {
this.adaptee = adaptee;
}
@Override
public void request() {
adaptee.specificRequest();
}
}
// 客户端代码
public class Client {
public static void main(String[] args) {
// 类适配器
Target classAdapter = new ClassAdapter();
classAdapter.request();
// 对象适配器
Adaptee adaptee = new Adaptee();
Target objectAdapter = new ObjectAdapter(adaptee);
objectAdapter.request();
}
}
```
以上示例中演示了适配器模式的两种实现方式:类适配器和对象适配器。通过适配器模式,我们可以让原本不兼容的Adaptee类和Target接口进行协作。
#### 4.2 装饰器模式的实例分析与代码示例
装饰器模式是一种结构型设计模式,它允许向对象动态地添加额外的功能。装饰器模式可以在不影响其他对象的情况下,动态地添加修改类的行为。
```java
// 被装饰的接口
interface Component {
void operation();
}
// 具体组件
class ConcreteComponent implements Component {
@Override
public void operation() {
System.out.println("Concrete Component operation");
}
}
// 抽象装饰器
abstract class Decorator implements Component {
protected Component component;
public Decorator(Component component) {
this.component = component;
}
@Override
public void operation() {
component.operation();
}
}
// 具体装饰器
class ConcreteDecoratorA extends Decorator {
public ConcreteDecoratorA(Component component) {
super(component);
}
@Override
public void operation() {
super.operation();
System.out.println("Added behavior from ConcreteDecoratorA");
}
}
// 客户端代码
public class Client {
public static void main(String[] args) {
Component component = new ConcreteComponent();
component.operation();
Component decoratedComponent = new ConcreteDecoratorA(new ConcreteComponent());
decoratedComponent.operation();
}
}
```
以上示例中演示了装饰器模式的实现方式,通过装饰器模式,我们可以动态地为对象添加额外的功能,而不需要修改原有类的结构。
#### 4.3 代理模式的实例分析与代码示例
代理模式是一种结构型设计模式,它允许通过代理对象控制对目标对象的访问。代理模式可以用于实现对目标对象的访问控制、延迟初始化、记录日志等功能。
```java
// 目标接口
interface Subject {
void request();
}
// 具体目标
class RealSubject implements Subject {
@Override
public void request() {
System.out.println("Real Subject's request");
}
}
// 代理
class Proxy implements Subject {
private RealSubject realSubject;
@Override
public void request() {
if (realSubject == null) {
realSubject = new RealSubject();
}
// 对目标对象的访问控制
realSubject.request();
}
}
// 客户端代码
public class Client {
public static void main(String[] args) {
Subject proxy = new Proxy();
proxy.request();
}
}
```
以上示例中演示了代理模式的实现方式,通过代理模式,我们可以控制对目标对象的访问,并在访问目标对象前后添加额外的逻辑。
#### 4.4 外观模式的实例分析与代码示例
外观模式是一种结构型设计模式,它提供了一个统一的接口,用于访问子系统中的一群接口。外观模式可以简化客户端与子系统之间的交互,提供一个更高层次的接口。
```java
// 子系统接口
class SubSystemA {
void operationA() {
System.out.println("SubSystemA operation");
}
}
class SubSystemB {
void operationB() {
System.out.println("SubSystemB operation");
}
}
class SubSystemC {
void operationC() {
System.out.println("SubSystemC operation");
}
}
// 外观
class Facade {
private SubSystemA subSystemA;
private SubSystemB subSystemB;
private SubSystemC subSystemC;
public Facade() {
subSystemA = new SubSystemA();
subSystemB = new SubSystemB();
subSystemC = new SubSystemC();
}
void operation() {
subSystemA.operationA();
subSystemB.operationB();
subSystemC.operationC();
}
}
// 客户端代码
public class Client {
public static void main(String[] args) {
Facade facade = new Facade();
facade.operation();
}
}
```
以上示例中演示了外观模式的实现方式,通过外观模式,我们可以提供一个统一的接口,简化客户端与子系统的交互过程。
#### 4.5 桥接模式的实例分析与代码示例
桥接模式是一种结构型设计模式,它将抽象部分与实现部分分离,使它们可以独立地变化。桥接模式可以用于处理具有多维度变化的类和系统。
```java
// 实现者接口
interface Implementor {
void operationImpl();
}
// 具体实现者
class ConcreteImplementorA implements Implementor {
@Override
public void operationImpl() {
System.out.println("ConcreteImplementorA operation");
}
}
class ConcreteImplementorB implements Implementor {
@Override
public void operationImpl() {
System.out.println("ConcreteImplementorB operation");
}
}
// 抽象
abstract class Abstraction {
protected Implementor implementor;
public Abstraction(Implementor implementor) {
this.implementor = implementor;
}
abstract void operation();
}
// 扩展抽象
class RefinedAbstraction extends Abstraction {
public RefinedAbstraction(Implementor implementor) {
super(implementor);
}
@Override
void operation() {
implementor.operationImpl();
}
}
// 客户端代码
public class Client {
public static void main(String[] args) {
Implementor implementorA = new ConcreteImplementorA();
Abstraction abstractionA = new RefinedAbstraction(implementorA);
abstractionA.operation();
Implementor implementorB = new ConcreteImplementorB();
Abstraction abstractionB = new RefinedAbstraction(implementorB);
abstractionB.operation();
}
}
```
以上示例中演示了桥接模式的实现方式,通过桥接模式,我们可以将抽象部分与实现部分独立变化,实现具有多维度变化的类和系统。
#### 4.6 组合模式的实例分析与代码示例
组合模式是一种结构型设计模式,它允许将对象组合成树形结构以表示“部分-整体”的层次结构。组合模式可以使客户端统一对待单个对象和组合对象。
```java
// 组件接口
interface Component {
void operation();
}
// 叶子组件
class Leaf implements Component {
private String name;
Leaf(String name) {
this.name = name;
}
@Override
public void operation() {
System.out.println("Leaf " + name + " operation");
}
}
// 容器组件
class Composite implements Component {
private List<Component> children = new ArrayList<>();
void add(Component component) {
children.add(component);
}
void remove(Component component) {
children.remove(component);
}
@Override
public void operation() {
for (Component component : children) {
component.operation();
}
}
}
// 客户端代码
public class Client {
public static void main(String[] args) {
Component leaf1 = new Leaf("leaf1");
Component leaf2 = new Leaf("leaf2");
Composite composite = new Composite();
composite.add(leaf1);
composite.add(leaf2);
composite.operation();
}
}
```
以上示例中演示了组合模式的实现方式,通过组合模式,我们可以将对象组合成树形结构,实现“部分-整体”的层次结构,并使客户端可以统一对待单个对象和组合对象。
#### 4.7 享元模式的实例分析与代码示例
享元模式是一种结构型设计模式,它通过共享技术实现相同或相似对象的重用,以减少内存占用和提高性能。享元模式通常用于管理大量细粒度的对象,以减少内存消耗。
```java
// 享元接口
interface Flyweight {
void operation();
}
// 具体享元
class ConcreteFlyweight implements Flyweight {
private String intrinsicState;
ConcreteFlyweight(String intrinsicState) {
this.intrinsicState = intrinsicState;
}
@Override
public void operation() {
System.out.println("ConcreteFlyweight operation, Intrinsic State: " + intrinsicState);
}
}
// 享元工厂
class FlyweightFactory {
private Map<String, Flyweight> flyweights = new HashMap<>();
Flyweight getFlyweight(String key) {
if (flyweights.containsKey(key)) {
return flyweights.get(key);
} else {
Flyweight flyweight = new ConcreteFlyweight(key);
flyweights.put(key, flyweight);
return flyweight;
}
}
}
// 客户端代码
public class Client {
public static void main(String[] args) {
FlyweightFactory factory = new FlyweightFactory();
Flyweight flyweight1 = factory.getFlyweight("key1");
flyweight1.operation();
Flyweight flyweight2 = factory.getFlyweight("key2");
flyweight2.operation();
Flyweight flyweight3 = factory.getFlyweight("key1");
flyweight3.operation();
}
}
```
以上示例中演示了享元模式的实现方式,通过享元模式,我们可以实现相同或相似对象的重用,减少内存占用并提高性能。
通过以上代码示例,我们深入理解了结构型设计模式在Java中的实现方式,并可以根据具体场景选择合适的设计模式来优化我们的代码结构和性能。
# 5. 结构型设计模式的优缺点分析
结构型设计模式是软件开发中常用的模式之一,它们可以帮助我们优化系统结构,提高代码复用性和灵活性。然而,每种设计模式都有其优点和缺点,开发人员需要在实际应用中进行权衡和选择,以达到最佳的效果。
### 5.1 结构型设计模式的优点
- 提高代码的可读性和可维护性:结构型设计模式可以使代码结构更清晰,降低系统的耦合度,易于理解和维护。
- 提高代码的复用性:通过结构型设计模式,可以将一些常用的设计思路和代码结构封装起来,提高代码的复用性,减少重复编码。
- 灵活性和扩展性:结构型设计模式可以使系统更加灵活,易于扩展和修改,满足系统需求的变化。
### 5.2 结构型设计模式的缺点
- 增加系统的复杂性:使用设计模式会增加系统中类和对象的数量,增加了系统的复杂性。
- 学习和理解成本:设计模式需要开发人员具备一定的抽象思维能力和对设计原则的理解,需要一定的学习和理解成本。
- 滥用设计模式:有时为了追求设计模式而过度设计,反而增加了系统的复杂性,产生“设计模式过度使用”问题。
### 5.3 如何选择合适的设计模式
在使用结构型设计模式时,需要根据实际的系统需求和特点进行选择,不能一概而论。在开发过程中,需要考虑以下几点:
- 系统的稳定性和可靠性要求
- 系统的功能和需求是否会发生变化
- 开发人员的水平和经验
- 是否需要权衡系统的性能和可维护性
总之,选择合适的设计模式需要根据具体情况进行综合考虑,平衡好系统的稳定性、可维护性和灵活性。
以上是结构型设计模式的优缺点分析,希望可以帮助您更好地理解和应用设计模式。
# 6. 结语
在本文中,我们深入探讨了结构型设计模式的概念、原理以及在Java中的实现。设计模式作为软件开发中常用的解决方案之一,能够帮助开发人员解决各种常见问题,并提供灵活、可维护的代码结构。通过对适配器模式、装饰器模式、代理模式、外观模式、桥接模式、组合模式和享元模式的深入讲解,我们希望读者能够更好地理解这些结构型设计模式,并在实际项目中灵活运用。
### 6.1 设计模式的实际应用
结构型设计模式在实际应用中有着广泛的应用场景。比如,当我们需要将一个类的接口转换成客户希望的另一个接口时,可以使用适配器模式;当需要为对象动态添加额外的功能时,可以使用装饰器模式;当需要控制对对象的访问时,可以使用代理模式;当需要为复杂子系统提供一个简单接口时,可以使用外观模式;当需要避免使用继承造成的子类爆炸时,可以使用桥接模式;当需要以一种一致的方式对待个别对象和对象组合时,可以使用组合模式;当需要支持大量细粒度的对象,可以使用享元模式。在实际应用中,结构型设计模式通过合理的组织和管理类与对象之间的关系,帮助开发人员快速解决问题,提高代码的灵活性和可维护性。
### 6.2 后续学习建议
设计模式作为软件开发中重要的知识点之一,需要持续的学习和实践。建议读者在掌握了结构型设计模式的基本原理和实际应用后,可以继续深入学习行为型设计模式和创建型设计模式,从而全面提升自己的设计能力和编程水平。此外,还可以通过阅读优秀的开源项目和参与实际的软件开发项目,不断积累实战经验,从而更加熟练地应用设计模式解决实际问题。
通过对结构型设计模式的深入理解和实际应用,我们相信读者可以在日常的软件开发工作中更加游刃有余,写出高质量、易维护的代码,为自己的职业发展打下坚实的基础。
0
0
相关推荐








