单片机按键控制流水灯:深入分析按键去抖与流水灯算法
发布时间: 2024-07-12 16:19:14 阅读量: 36 订阅数: 34 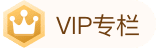
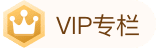
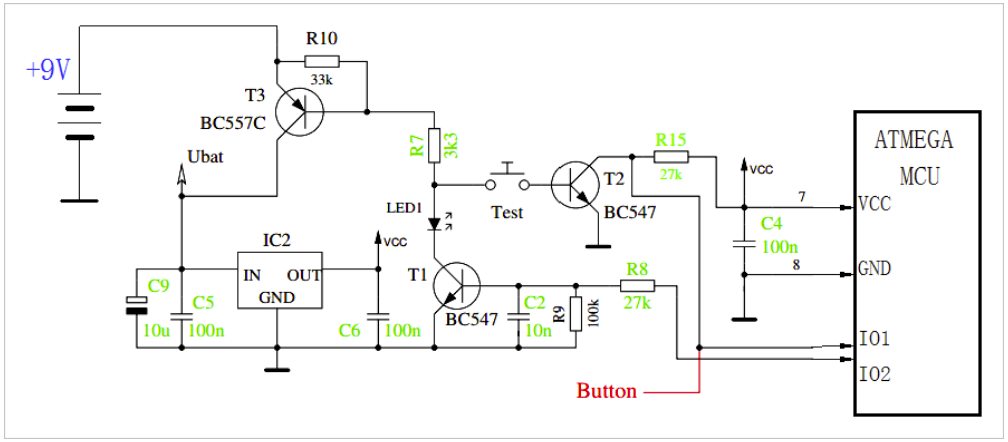
# 1. 单片机按键控制流水灯概述
单片机按键控制流水灯系统是一个经典的嵌入式系统,它利用单片机作为控制核心,通过按键控制流水灯的亮灭状态。本系统主要涉及按键去抖、流水灯算法和单片机系统设计等方面。
本系统的主要功能是通过按键控制流水灯的亮灭。当按下按键时,单片机检测到按键信号,并通过软件或硬件去抖消除按键抖动。然后,单片机根据流水灯算法控制流水灯的亮灭,实现流水灯效果。
本系统具有以下特点:
* **可控性:**通过按键控制流水灯的亮灭状态,方便用户操作。
* **可扩展性:**系统可以根据需要扩展其他功能,如增加按键数量、改变流水灯模式等。
* **低成本:**单片机系统成本低廉,适合于小型嵌入式系统应用。
# 2. 按键去抖理论与实践
### 2.1 按键去抖的原理和方法
在实际应用中,按键开关由于机械接触不良或环境干扰等因素,往往会出现短暂的抖动现象。这种抖动会导致单片机接收到的按键信号不稳定,影响系统的正常工作。因此,需要对按键进行去抖处理,消除抖动带来的影响。
按键去抖的方法主要分为软件去抖和硬件去抖。
#### 2.1.1 软件去抖
软件去抖是通过程序代码实现的。其基本原理是:当检测到按键按下时,不立即执行相应的动作,而是等待一段时间(通常为10-20ms),如果在这段时间内按键一直处于按下状态,则认为按键有效,执行相应的动作。
软件去抖的优点是实现简单,不需要额外的硬件电路。但其缺点是延时较长,可能会影响系统的实时性。
#### 2.1.2 硬件去抖
硬件去抖是通过外部电路实现的。其基本原理是:在按键开关的两端并联一个电容,当按键按下时,电容充电,按键松开时,电容放电。通过控制电容的充放电时间,可以消除按键抖动。
硬件去抖的优点是去抖效果好,延时短。但其缺点是需要额外的硬件电路,增加系统的复杂性。
### 2.2 按键去抖的代码实现
#### 2.2.1 软件去抖的代码实现
```c
#define KEY_DEBOUNCE_TIME 10 // 按键去抖时间,单位:ms
uint8_t key_debounce_flag = 0; // 按键去抖标志位
void key_debounce(void)
{
if (key_debounce_flag == 0)
{
if (KEY_PORT & KEY_PIN) // 按键按下
{
key_debounce_flag = 1;
SysTick_DelayMs(KEY_DEBOUNCE_TIME); // 延时去抖
}
}
else
{
if (!(KEY_PORT & KEY_PIN)) // 按键松开
{
key_debounce_flag = 0;
}
}
}
```
**代码逻辑分析:**
* 定义按键去抖时间为10ms。
* 定义按键去抖标志位`key_debounce_flag`,用于指示按键状态。
* 在`key_debounce()`函数中:
* 如果按键去抖标志位为0,表示按键处于未按下状态。
* 如果按键被按下,则将按键去抖标志位置1,并延时10ms。
* 如果按键去抖标志位为1,表示按键处于按下状态。
* 如果按键被松开,则将按键去抖标志位置0。
#### 2.2.2 硬件去抖的代码实现
```c
#define KEY_DEBOUNCE_CAP_VALUE 10000 // 按键去抖电容值,单位:nF
void key_debounce_hardware(void)
{
if (KEY_PORT & KEY_PIN) // 按键按下
{
if (HAL_GPIO_ReadPin(KEY_PORT, KEY_PIN) == GPIO_PIN_SET) // 硬件去抖
{
// 按键有效,执行相应动作
}
}
}
```
**代码逻辑分析:**
* 定义按键去抖电容值为10000nF。
* 在`key_debounce_hardware()`函数中:
* 如果按键被按下,则通过`HAL_GPIO_ReadPin()`函数读取按键引脚的状态。
* 如果按键引脚状态为高电平,表示按键有效,执行相应动作。
# 3.1 流水灯算法的原理和特点
#### 3.1.1 基本流水灯算法
流水灯算法是一种循环点亮一组 LED 的算法,形成一种流水灯效果。基本流水灯算法的原理如下:
1. 定义一个变量 `led_index`,表示当前点亮的 LED 序号。
2. 在一个循环中,依次点亮每个 LED。
3. 点亮当前 LED 后,将 `led_index` 加 1,并判断是否超过 LED 总数。
4. 如果 `led_index` 超过 LED 总数,则将其重置为 0。
#### 3.1.2 优化流水灯算法
基本流水灯算法存在一个问题,当 LED 数量较多时,流水效果会比较慢。为了优化算法,可以采用以下方法:
1. **并行点亮 LED:**使用多个 GPIO 端口同时点亮多个 LED,从而提高流水速度。
2. **使用 DMA:**利用 DMA(直接内存访问)技术,将 LED 点亮数据直接写入到 LED 寄存器,减少 CPU 开销。
3. **优化循环结构:**使用高效的循环结构,如 `for` 循环或 `while` 循环,减少循环开销。
### 3.2 流水灯算法的代码实现
#### 3.2.1 基本流水灯算法的代码实现
```c
#define LED_NUM 8
void basic_led_loop() {
uint8_t led_index = 0;
while (1) {
// 点亮当前 LED
GPIO_SetBits(LED_PORT, 1 << led_index);
// 延时
Delay_ms(100);
// 关闭当前 LED
GPIO_ResetBits(LED_PORT, 1 << led_index);
// 更新 LED 序号
led_index++;
// 判断是否超过 LED 总数
if (led_index >= LED_NUM) {
led_index = 0;
}
}
}
```
**逻辑分析:**
* `basic_led_loop()` 函数是一个无限循环,不断点亮和关闭 LED。
* `led_index` 变量记录当前点亮的 LED 序号。
* 循环中,依次点亮每个 LED,并延时 100ms。
* 点亮 LED 后,关闭当前 LED 并更新 `led_index`。
* 如果 `led_index` 超过 LED 总数,则将其重置为 0。
#### 3.2.2 优化流水灯算法的代码实现
```c
#define LED_NUM 8
void optimized_led_loop() {
uint8_t led_index = 0;
uint8_t led_data = 0x01;
while (1) {
// 点亮当前 LED
GPIO_SetBits(LED_PORT, led_data);
// 延时
Delay_ms(100);
// 关闭当前 LED
GPIO_ResetBits(LED_PORT, led_data);
// 更新 LED 数据
led_data <<= 1;
// 判断是否超过 LED 总数
if (led_data == 0x00) {
led_data = 0x01;
}
}
}
```
**逻辑分析:**
* `optimized_led_loop()` 函数也采用无限循环,但优化了点亮 LED 的方式。
* `led_data` 变量记录当前点亮的 LED 数据,使用二进制位移操作实现流水效果。
* 循环中,点亮当前 LED,并延时 100ms。
* 点亮 LED 后,关闭当前 LED 并更新 `led_data`。
* 如果 `led_data` 为 0x00,表示已经点亮了所有 LED,则将其重置为 0x01。
# 4. 单片机按键控制流水灯系统设计
### 4.1 系统硬件设计
#### 4.1.1 按键和流水灯的连接
**按键连接:**
* 按键一端连接到单片机的输入/输出端口。
* 另一端连接到地线(GND)。
**流水灯连接:**
* 流水灯的正极连接到单片机的输出端口。
* 流水灯的负极连接到地线(GND)。
#### 4.1.2 单片机芯片的选择
单片机芯片的选择需要考虑以下因素:
* **输入/输出端口数量:**需要足够数量的输入/输出端口来连接按键和流水灯。
* **处理能力:**单片机需要具有足够的处理能力来处理按键去抖和流水灯算法。
* **功耗:**单片机应具有低功耗,以延长电池寿命。
### 4.2 系统软件设计
#### 4.2.1 按键去抖模块设计
按键去抖模块负责消除按键抖动,防止单片机误识别按键按下或松开。
**软件去抖:**
```c
#define DEBOUNCE_TIME 10 // 去抖时间(ms)
uint8_t key_state = 0; // 按键状态(0:松开,1:按下)
void key_debounce(void)
{
static uint8_t key_count = 0; // 按键计数器
// 读取按键状态
uint8_t key_input = GPIO_ReadInputDataBit(GPIOA, GPIO_Pin_0);
// 去抖处理
if (key_input != key_state) {
key_count = 0;
} else {
key_count++;
}
// 当按键稳定按下或松开一定时间后,更新按键状态
if (key_count >= DEBOUNCE_TIME) {
key_state = key_input;
}
}
```
**逻辑分析:**
* `DEBOUNCE_TIME`定义了去抖时间,当按键状态稳定保持该时间时,才更新按键状态。
* `key_state`记录了按键的当前状态。
* `key_debounce()`函数不断读取按键输入,并根据去抖算法更新按键状态。
#### 4.2.2 流水灯算法模块设计
流水灯算法模块负责控制流水灯的亮灭顺序。
**基本流水灯算法:**
```c
uint8_t led_state = 0; // 流水灯状态(每一位对应一个流水灯)
void led_loop(void)
{
// 更新流水灯状态
led_state = (led_state << 1) | (led_state >> 7);
// 根据流水灯状态点亮相应的流水灯
for (uint8_t i = 0; i < 8; i++) {
if (led_state & (1 << i)) {
GPIO_SetBits(GPIOA, GPIO_Pin_i);
} else {
GPIO_ResetBits(GPIOA, GPIO_Pin_i);
}
}
}
```
**逻辑分析:**
* `led_state`记录了流水灯的当前状态,每一位对应一个流水灯。
* `led_loop()`函数不断更新流水灯状态并点亮相应的流水灯。
* 流水灯状态通过移位操作实现,每一步将状态左移一位,并用状态最右一位填充状态最左一位,从而形成流水效果。
**优化流水灯算法:**
```c
uint8_t led_state = 0; // 流水灯状态(每一位对应一个流水灯)
void led_loop(void)
{
// 更新流水灯状态
led_state = (led_state << 1) | (led_state >> 7);
// 根据流水灯状态点亮相应的流水灯
for (uint8_t i = 0; i < 8; i++) {
if (led_state & (1 << i)) {
GPIO_SetBits(GPIOA, GPIO_Pin_i);
} else {
GPIO_ResetBits(GPIOA, GPIO_Pin_i);
}
}
// 优化:当流水灯状态达到最大值或最小值时,直接更新状态
if (led_state == 0xFF || led_state == 0x00) {
led_state = 0x01;
}
}
```
**逻辑分析:**
* 优化后的算法在流水灯状态达到最大值(0xFF)或最小值(0x00)时,直接将状态更新为0x01,避免了多余的移位操作,提高了算法效率。
# 5.1 系统调试
### 5.1.1 按键去抖调试
1. **硬件调试:**检查按键和单片机的连接是否正确,确保按键按下时能够产生稳定的电平变化。
2. **软件调试:**使用示波器或逻辑分析仪观察按键引脚上的电平变化,验证去抖算法是否有效。
3. **参数调整:**根据按键的实际情况调整去抖算法中的参数,如滤波时间常数或采样频率,以获得最佳的去抖效果。
### 5.1.2 流水灯算法调试
1. **硬件调试:**检查流水灯和单片机的连接是否正确,确保流水灯能够正常点亮。
2. **软件调试:**使用示波器或逻辑分析仪观察流水灯引脚上的电平变化,验证流水灯算法是否正确。
3. **参数调整:**根据流水灯的实际情况调整算法中的参数,如流水速度或流水方向,以获得最佳的流水效果。
0
0
相关推荐
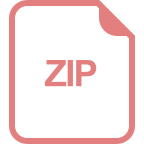
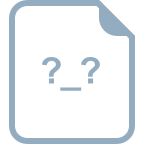
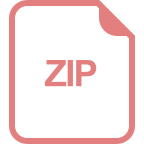





