使用useReducer和自定义Hook优化React应用状态管理
发布时间: 2024-01-07 21:07:19 阅读量: 10 订阅数: 13 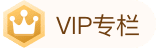
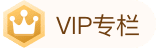
# 1. 引言
## 1.1 问题背景
在开发大型的React应用时,有效的状态管理是至关重要的。随着应用规模的增长,状态管理往往变得复杂且难以维护。传统的状态管理方式,如使用useState钩子或者Redux等库,可能存在一些不足之处,需要更加简洁和可维护的解决方案。
## 1.2 解决方案概述
本文将介绍使用useReducer和自定义Hook来优化React应用的状态管理。使用useReducer可以更好地处理复杂的状态逻辑,而自定义Hook则可以将通用的状态逻辑提取出来,实现状态逻辑的复用。
## 1.3 文章结构
本文将分为六个章节,首先介绍React状态管理的问题和挑战,然后针对useReducer和自定义Hook进行详细的介绍和示例。最后,通过实战示例和总结,帮助读者更好地理解和应用这些优化状态管理的技巧。
# 2. React状态管理简介
2.1 React中的状态管理
2.2 常见的状态管理解决方案
2.3 问题和挑战
### 2.1 React中的状态管理
在React应用中,状态是组件渲染和交互的核心。React组件的状态可以通过state来管理,但随着应用规模增大,状态管理变得复杂起来。在复杂的组件树中,状态的传递和管理变得困难,这就需要一种更加灵活和可控的状态管理方式。
### 2.2 常见的状态管理解决方案
在React中,有多种方式来管理组件之间的状态,包括使用context、Redux、MobX等。这些解决方案各有优劣,可以根据具体场景和需求来选择合适的状态管理工具。
### 2.3 问题和挑战
随着应用规模的扩大,常见的状态管理解决方案可能带来一些性能和复杂性方面的问题。在大型应用中,需要更好地控制状态管理的逻辑,以便提高应用的性能和可维护性。接下来,我们将介绍一种新的状态管理方式:useReducer。
# 3. 介绍useReducer
在本章中,我们将深入介绍React中的useReducer,包括其概念、用途、基本用法以及优势和适用场景。
#### 3.1 useReducer的概念和用途
在React中,useState是最常用的状态管理钩子。然而,对于复杂的状态逻辑,useState可能会导致代码变得难以维护。这时,可以使用useReducer来更好地管理状态。
useReducer是一个基于Reducer模式的状态管理钩子,它可以帮助我们更好地组织复杂的状态逻辑。使用useReducer可以在不增加组件层级的情况下将状态逻辑迁移到组件外部。
#### 3.2 useReducer的基本用法
useReducer接受一个reducer函数和初始状态作为参数,并返回当前状态和一个dispatch函数。
```jsx
import React, { useReducer } from 'react';
const initialState = { count: 0 };
function reducer(state, action) {
switch (action.type) {
case 'increment':
return { count: state.count + 1 };
case 'decrement':
return { count: state.count - 1 };
default:
throw new Error();
}
}
function Counter() {
const [state, dispatch] = useReducer(reducer, initialState);
return (
<div>
Count: {state.count}
<button onClick={() => dispatch({ type: 'increment' })}>+</button>
<button onClick={() => dispatch({ type: 'decrement' })}>-</button>
</div>
);
}
export default Counter;
```
在上面的示例中,我们定义了一个简单的计数器组件,使用了useReducer来管理状态。通过dispatch函数,我们可以触发reducer中定义的操作来更新状态。
#### 3.3 useReducer的优势和适用场景
相比于useState,useReducer具有以下优势:
- 适用于复杂的状态逻辑,使代码更易于维护和扩展
- 可以通过多个reducer拆分状态,使状态管理更加灵活
- 适用于组件之间共享状态的场景
适用场景包括但不限于:表单处理、数据同步、WebSocket连接等需要处理复杂逻辑的场景。
通过使用useReducer,我们可以更好地组织和管理React应用的状态逻辑,从而提高代码的可读性和可维护性。
# 4. 编写自定义Hook
在本章中,我们将深入探讨自定义Hook的概念、作用以及如何创建通用的状态管理器Hook,并演示如何在项目中使用自定义Hook来优化状态管理。让我们一起来了解吧。
### 4.1 自定义Hook的概念和作用
自定义Hook是一种让你可以使用 React 内置特性(例如状态、生命周期方法、context等)的方式,同时可以在组件之间共享逻辑的方法。它可以将组件之间共享的逻辑抽取出来,以函数的形式进行复用。通过自定义Hook,我们可以使代码更加简洁和易于维护。
### 4.2 创建通用的状态管理器Hook
让我们通过一个示例来创建一个通用的状态管理器Hook,来管理一个简单的计数器:
```javascript
import { useReducer } from 'react';
const initialState = { count: 0 };
function reducer(state, action) {
switch (action.type) {
case 'increment':
return { count: state.count + 1 };
case 'decrement':
return { count: state.count - 1 };
default:
throw new Error();
}
}
function useCounter() {
const [state, dispatch] = useReducer(reducer, initialState);
const increment = () => dispatch({ type: 'increment' });
const decrement = () => dispatch({ type: 'decrement' });
return {
count: state.count,
increment,
decrement,
};
}
export default useCounter;
```
在上面的代码中,我们使用了`useReducer`来创建一个状态管理器Hook,它包含了一个计数器的状态及对应的操作。通过这个自定义Hook,我们可以在任何需要计数器的组件中复用这段逻辑,使得组件之间的状态管理更加灵活和可维护。
### 4.3 在项目中使用自定义Hook
在实际项目中,我们可以轻松地在组件中引入并使用自定义Hook `useCounter`:
```javascript
import React from 'react';
import useCounter from './useCounter';
function CounterComponent() {
const { count, increment, decrement } = useCounter();
return (
<div>
<p>Count: {count}</p>
<button onClick={increment}>Increment</button>
<button onClick={decrement}>Decrement</button>
</div>
);
}
export default CounterComponent;
```
通过以上示例,我们看到了如何创建和使用自定义Hook来管理状态,这样可以减少组件间重复的逻辑代码,使得代码更加清晰和可维护。
在下一章节中,我们将学习如何使用`useReducer`优化状态管理,敬请期待!
# 5. 使用useReducer优化状态管理
在前面的章节中,我们介绍了React中的状态管理和常见的解决方案,以及引入了useReducer的概念。本章将重点介绍如何使用useReducer来优化状态管理。
#### 5.1 将状态转移到useReducer中管理
在React中,通常我们会使用useState来管理组件的状态。然而,当组件的状态变得复杂且需要进行复杂的操作时,单纯使用useState可能会导致代码变得难以维护和管理。
而useReducer则可以提供一种更有组织和可预测的方式来管理状态。它通过使用一个reducer函数和初始状态来处理组件的状态逻辑。
```javascript
import React, { useReducer } from 'react';
const initialState = { count: 0 };
function reducer(state, action) {
switch (action.type) {
case 'increment':
return { count: state.count + 1 };
case 'decrement':
return { count: state.count - 1 };
default:
throw new Error();
}
}
function Counter() {
const [state, dispatch] = useReducer(reducer, initialState);
return (
<div>
Count: {state.count}
<button onClick={() => dispatch({ type: 'increment' })}>+</button>
<button onClick={() => dispatch({ type: 'decrement' })}>-</button>
</div>
);
}
```
在上面的示例中,我们使用useReducer来管理一个计数器的状态。reducer函数接收当前的状态和一个action对象作为参数,并根据action的类型返回新的状态。通过dispatch函数,我们可以触发reducer函数并更新组件的状态。
#### 5.2 通过多个reducer拆分状态
一个应用可能包含多个状态需要管理,而且可能存在一些共享状态。使用多个reducer可以帮助我们更好地拆分和管理状态。
```javascript
import React, { useReducer } from 'react';
const initialState = {
user: null,
loggedIn: false,
};
function userReducer(state, action) {
switch (action.type) {
case 'login':
return { ...state, user: action.payload, loggedIn: true };
case 'logout':
return initialState;
default:
return state;
}
}
function appReducer(state, action) {
return {
user: userReducer(state.user, action),
// 其他reducer
};
}
function App() {
const [state, dispatch] = useReducer(appReducer, initialState);
return (
<div>
{state.loggedIn ? (
<p>Welcome, {state.user}!</p>
) : (
<p>Please log in.</p>
)}
<button onClick={() => dispatch({ type: 'login', payload: 'John' })}>
Log In
</button>
<button onClick={() => dispatch({ type: 'logout' })}>Log Out</button>
</div>
);
}
```
在上述示例中,我们使用了多个reducer来拆分和管理不同部分的状态。通过appReducer函数,我们可以将多个reducer组合在一起,并在组件中使用useReducer来管理整体的状态。
#### 5.3 使用useContext在组件树中传递状态
在某些情况下,我们可能需要在组件树中的多个组件之间共享状态。这时,可以使用useContext来传递状态,以避免通过props一层一层传递状态的麻烦。
```javascript
import React, { useReducer, useContext } from 'react';
const CounterContext = React.createContext();
const initialState = { count: 0 };
function reducer(state, action) {
switch (action.type) {
case 'increment':
return { count: state.count + 1 };
case 'decrement':
return { count: state.count - 1 };
default:
throw new Error();
}
}
function Counter() {
const [state, dispatch] = useContext(CounterContext);
return (
<div>
Count: {state.count}
<button onClick={() => dispatch({ type: 'increment' })}>+</button>
<button onClick={() => dispatch({ type: 'decrement' })}>-</button>
</div>
);
}
function App() {
const [state, dispatch] = useReducer(reducer, initialState);
return (
<CounterContext.Provider value={[state, dispatch]}>
<Counter />
</CounterContext.Provider>
);
}
```
在上述示例中,我们使用CounterContext来创建一个共享的上下文,然后在Counter组件中使用useContext来获取共享的状态。
通过以上的方式,我们可以通过useReducer和useContext来更好地管理和共享状态,使我们的组件更加容易维护和扩展。
在下一章中,我们将通过一个实战示例来演示如何使用useReducer和自定义Hook来优化现有的应用。
# 6. 实战示例和总结
在本章中,我们将通过一个具体的实战示例来演示如何使用`useReducer`和自定义Hook来优化React应用的状态管理。我们将展示如何重构一个现有的应用,以及对这种优化方法的总结和展望。
#### 6.1 使用useReducer和自定义Hook重构现有应用
```jsx
// 之前的状态管理方式
import React, { useState } from 'react';
const Counter = () => {
const [count, setCount] = useState(0);
const increment = () => {
setCount(count + 1);
};
const decrement = () => {
setCount(count - 1);
};
return (
<div>
<p>Count: {count}</p>
<button onClick={increment}>Increment</button>
<button onClick={decrement}>Decrement</button>
</div>
);
};
export default Counter;
```
```jsx
// 使用useReducer和自定义Hook重构后
import React from 'react';
const initialState = { count: 0 };
function reducer(state, action) {
switch (action.type) {
case 'increment':
return { count: state.count + 1 };
case 'decrement':
return { count: state.count - 1 };
default:
throw new Error();
}
}
function useCounter() {
const [state, dispatch] = useReducer(reducer, initialState);
const increment = () => {
dispatch({ type: 'increment' });
};
const decrement = () => {
dispatch({ type: 'decrement' });
};
return { count: state.count, increment, decrement };
}
const Counter = () => {
const { count, increment, decrement } = useCounter();
return (
<div>
<p>Count: {count}</p>
<button onClick={increment}>Increment</button>
<button onClick={decrement}>Decrement</button>
</div>
);
};
export default Counter;
```
在这个示例中,我们将原来使用`useState`来管理状态的`Counter`组件,通过`useReducer`和自定义Hook`useCounter`来进行了重构。通过这个实战示例,我们可以看到使用`useReducer`和自定义Hook可以将状态逻辑抽离,使组件更加清晰和灵活。
#### 6.2 总结和展望
通过本文的介绍,我们了解了如何使用`useReducer`和自定义Hook来优化React应用的状态管理。`useReducer`可以帮助我们更好地管理组件的复杂状态逻辑,而自定义Hook可以将状态逻辑进行抽离,使其更易于复用。
在未来的React版本中,我们也可以期待更多关于状态管理的改进和新特性的加入,这将进一步丰富我们在React应用中进行状态管理的工具和技巧。
总的来说,使用`useReducer`和自定义Hook是一种非常有效的方式来优化和管理React应用的状态,它能够提高代码的可维护性和扩展性,同时也提升了开发效率和用户体验。
在未来的项目中,读者可以尝试将这些技术应用到实际项目中,体验其带来的好处。希望本文对读者能有所帮助,谢谢阅读!
0
0
相关推荐
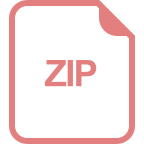
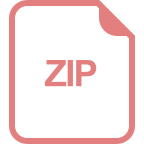
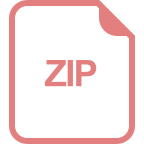





