Spring Boot Security简介与基本配置
发布时间: 2023-12-20 19:52:40 阅读量: 10 订阅数: 19 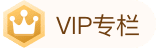
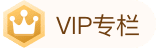
# 简介
## Spring Boot Security的重要性
### 基本概念与原理
Spring Boot Security是Spring框架提供的一个强大的安全性解决方案,用于保护你的应用程序免受各种安全威胁。它基于Spring Security框架,为Spring Boot应用程序提供了许多内置的安全功能。
#### 主要概念
1. **认证(Authentication)**:验证用户的身份以确保其是可信的过程。Spring Boot Security支持多种认证方式,包括基于表单登录、HTTP Basic认证、OAuth等方式。
2. **授权(Authorization)**:确定用户是否有权限执行特定操作或访问特定资源的过程。通过Spring Boot Security,你可以使用注解或配置来定义访问控制规则。
3. **密码加密(Password Encryption)**:Spring Boot Security提供了密码加密的支持,可以确保用户密码在存储和传输过程中的安全性。
4. **会话管理(Session Management)**:管理用户的会话并保护用户不受会话劫持等攻击。
#### 原理解析
Spring Boot Security基于Filter链(Filter Chain)来处理Web请求,它在请求到达应用程序之前拦截请求并对请求进行安全性检查。这些Filter负责执行认证、授权、会话管理等操作,确保应用程序的安全性。通过配置不同的Filter和Provider,开发者可以灵活地定制安全性策略。
此外,Spring Boot Security的安全性依赖于Spring框架的IoC容器和AOP进行实现,它允许开发者通过依赖注入和切面编程等方式来增强应用程序的安全性。
### 基本配置步骤
在使用Spring Boot Security时,需要进行一些基本的配置步骤。以下是一些常见的配置步骤:
1. 添加依赖:首先,在`pom.xml`文件中添加Spring Boot Security的依赖。
```xml
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-security</artifactId>
</dependency>
```
2. 创建Security配置类:创建一个`SecurityConfig`类来配置Security设置。
```java
import org.springframework.context.annotation.Configuration;
import org.springframework.security.config.annotation.web.configuration.EnableWebSecurity;
import org.springframework.security.config.annotation.web.configuration.WebSecurityConfigurerAdapter;
@Configuration
@EnableWebSecurity
public class SecurityConfig extends WebSecurityConfigurerAdapter {
}
```
3. 配置认证信息:在`SecurityConfig`类中,可以重写`configure(AuthenticationManagerBuilder auth)`方法,来配置内存中的用户认证信息。
```java
@Override
protected void configure(AuthenticationManagerBuilder auth) throws Exception {
auth
.inMemoryAuthentication()
.withUser("user")
.password("{noop}password")
.roles("USER");
}
```
4. 配置授权规则:同样在`SecurityConfig`类中,可以重写`configure(HttpSecurity http)`方法,来配置访问路径的授权规则。
```java
@Override
protected void configure(HttpSecurity http) throws Exception {
http
.authorizeRequests()
.antMatchers("/public/**").permitAll()
.anyRequest().authenticated()
.and()
.formLogin()
.loginPage("/login")
.permitAll()
.and()
.logout()
.permitAll();
}
```
### 定制化配置
在实际项目中,通常需要根据具体需求对Spring Boot Security进行定制化配置。以下是一些常见的定制化配置示例:
#### 1. 自定义用户认证
可以通过实现`UserDetailsService`接口自定义用户认证逻辑。我们可以创建一个继承自`UserDetailsService`接口的类,并在其中实现`loadUserByUsername`方法来自定义用户的认证逻辑。下面是一个简单的示例代码:
```java
@Service
public class CustomUserDetailsService implements UserDetailsService {
@Autowired
private UserRepository userRepository;
@Override
public UserDetails loadUserByUsername(String username) throws UsernameNotFoundException {
User user = userRepository.findByUsername(username);
if (user == null) {
throw new UsernameNotFoundException("User not found");
}
return new CustomUserDetails(user);
}
}
```
#### 2. 自定义登录页面
可以通过配置`WebSecurityConfigurerAdapter`类来自定义登录页面。我们可以创建一个继承自`WebSecurityConfigurerAdapter`的类,并覆盖`configure`方法来配置登录页面的定制化逻辑。下面是一个示例代码:
```java
@EnableWebSecurity
public class SecurityConfig extends WebSecurityConfigurerAdapter {
@Override
protected void configure(HttpSecurity http) throws Exception {
http
.authorizeRequests()
.anyRequest().authenticated()
.and()
.formLogin()
.loginPage("/login")
.permitAll();
}
}
```
#### 3. 自定义访问控制
可以通过配置`HttpSecurity`对象来自定义访问控制规则。我们可以在`configure`方法中使用`http.authorizeRequests()`来添加特定的访问控制规则。下面是一个示例代码:
```java
@EnableWebSecurity
public class SecurityConfig extends WebSecurityConfigurerAdapter {
@Override
protected void configure(HttpSecurity http) throws Exception {
http
.authorizeRequests()
.antMatchers("/admin/**").hasRole("ADMIN")
.antMatchers("/user/**").hasAnyRole("ADMIN", "USER")
.anyRequest().authenticated()
.and()
.formLogin()
.permitAll();
}
}
```
### 安全最佳实践
在使用Spring Boot Security时,有一些安全最佳实践可以帮助你确保应用程序的安全性。下面是一些建议:
1. **密码加密存储**:建议使用密码加密存储,可以使用BCryptPasswordEncoder来加密密码,并确保在数据库中存储加密后的密码而不是明文密码。
2. **CSRF保护**:Spring Security提供了跨站请求伪造(CSRF)保护功能,确保你的应用程序能够在处理表单提交时进行CSRF保护。
3. **HTTP安全头**:通过配置HTTP安全头可以防止常见的安全漏洞,比如点击劫持、跨站脚本等。你可以配置HTTP安全头来强制使用安全传输协议、设置X-Content-Type-Options、X-XSS-Protection头等。
4. **权限控制**:在应用程序中实施细粒度的权限控制是非常重要的。确保只有经过授权的用户可以访问特定的资源。
5. **日志和监控**:在代码中加入适当的日志记录以及监控功能可以帮助你发现潜在的安全问题或者恶意攻击。
6. **安全依赖更新**:定期更新你的应用程序所依赖的安全库和框架,确保你的应用程序不受已知安全漏洞的影响。
0
0
相关推荐
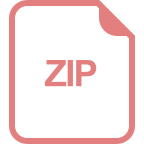
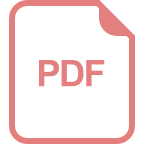





