Python并发编程的资源管理:优化多线程与多进程性能的秘诀
发布时间: 2024-06-22 04:43:29 阅读量: 89 订阅数: 32 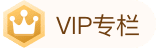
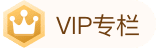
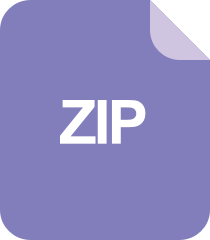
数据挖掘课程:Python实现推荐系统的协同过滤算法
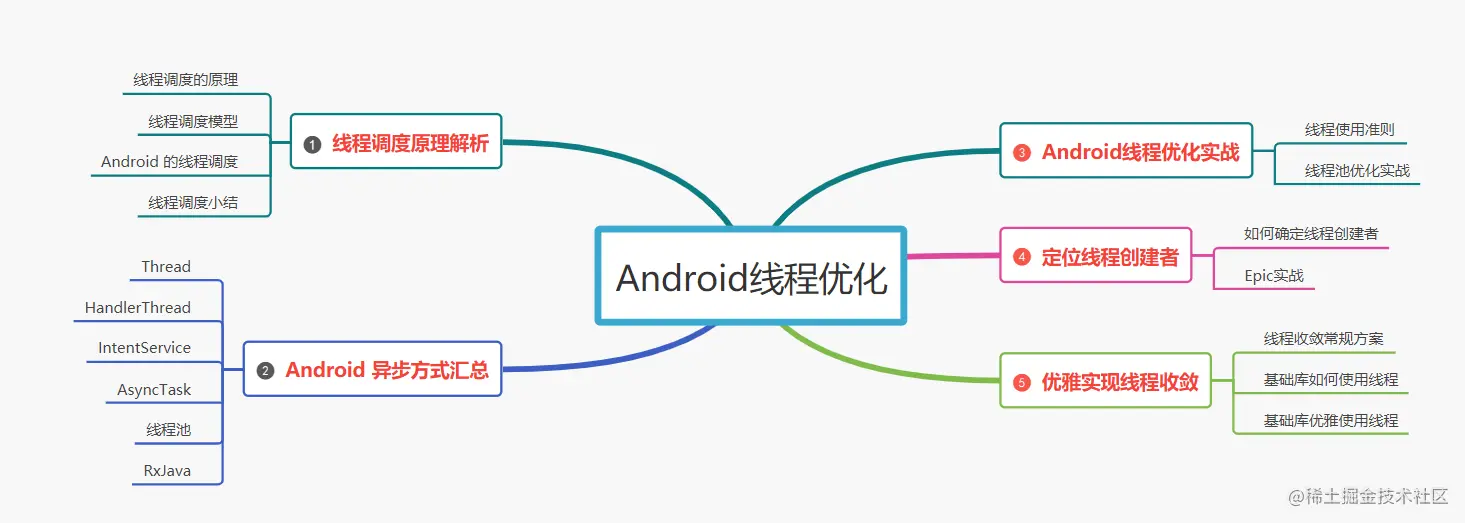
# 1. Python并发编程基础**
并发编程是一种编程范式,它允许在单个计算机系统上同时执行多个任务。Python通过多线程和多进程两种方式支持并发编程。
**多线程并发编程**
多线程并发编程涉及创建多个线程,每个线程都执行一个独立的任务。线程共享同一内存空间,因此可以轻松地访问和修改共享数据。但是,多线程编程需要仔细的同步机制,以避免数据竞争和死锁。
**多进程并发编程**
多进程并发编程涉及创建多个进程,每个进程都有自己的内存空间。进程之间通过消息传递或共享内存进行通信。多进程编程通常比多线程编程开销更大,但它可以提供更好的隔离性和稳定性。
# 2. 多线程并发编程**
**2.1 线程的创建和管理**
**2.1.1 线程的创建**
在Python中,可以使用`threading`模块创建线程。`threading.Thread`类提供了`start()`方法来启动线程,`run()`方法定义了线程执行的代码。
```python
import threading
def task():
print('Hello from thread')
thread = threading.Thread(target=task)
thread.start()
```
**逻辑分析:**
* `threading.Thread(target=task)`创建一个新的线程,`target`参数指定了线程执行的函数。
* `thread.start()`启动线程,调用`run()`方法。
* `run()`方法执行`task`函数中定义的代码。
**2.1.2 线程的同步和通信**
**锁**
锁是一种同步机制,用于防止多个线程同时访问共享资源。`threading`模块提供了`Lock`类来创建锁。
```python
import threading
lock = threading.Lock()
def task():
with lock:
# 临界区代码
pass
thread1 = threading.Thread(target=task)
thread2 = threading.Thread(target=task)
thread1.start()
thread2.start()
```
**逻辑分析:**
* `lock = threading.Lock()`创建一个新的锁。
* `with lock:`语句创建一个上下文管理器,该管理器在进入和离开时分别获取和释放锁。
* 临界区代码在上下文管理器内执行,确保一次只有一个线程可以访问该代码。
**事件**
事件是一种同步机制,用于通知一个或多个线程某个事件已发生。`threading`模块提供了`Event`类来创建事件。
```python
import threading
event = threading.Event()
def task():
event.wait()
# 事件发生后的代码
thread1 = threading.Thread(target=task)
thread2 = threading.Thread(target=task)
thread1.start()
thread2.start()
event.set()
```
**逻辑分析:**
* `event = threading.Event()`创建一个新的事件。
* `event.wait()`阻塞线程,直到事件被设置。
* `event.set()`设置事件,通知等待的线程。
**2.2 线程池的应用**
**2.2.1 线程池的原理和使用**
线程池是一种管理线程的机制,它可以提高线程创建和销毁的效率。`concurrent.futures`模块提供了`ThreadPoolExecutor`类来创建线程池。
```python
import concurrent.futures
executor = concurrent.futures.ThreadPoolExecutor(max_workers=5)
def task(n):
return n * n
with executor as executor:
results = executor.map(task, range(10))
```
**逻辑分析:**
* `executor = concurrent.futures.ThreadPoolExecutor(max_workers=5)`创建一个线程池,最多可以同时运行5个线程。
* `with executor as executor:`创建一个上下文管理器,该管理器在进入和离开时分别启动和关闭线程池。
* `executor.map(task, range(10))`使用线程池并行执行`task`函数,并返回一个包含结果的迭代器。
**2.2.2 线程池的性能优化**
**线程池大小**
线程池大小应根据应用程序的并发需求进行调整。线程池过大会浪费资源,而线程池过小会导致任务排队等待。
**任务分配**
任务分配算法可以影响线程池的性能。`ThreadPoolExecutor`提供了`map()`和`submit()`等方法来分配任务。
**任务调度**
任务调度算法可以影响线程池的性能。`ThreadPoolExecutor`提供了`as_completed()`方法来调度任务,该方法以任务完成的顺序返回结果。
# 3.1 进程的创建和管理
#### 3.1.1 进程的创建
在Python中,可以使用`multiprocessing`模块来创建和管理进程。`multiprocessing`模块提供了`Process`类,用于创建和管理进程。
```python
import multiproc
```
0
0