mobx中的Actions和Action Creators详解
发布时间: 2023-12-20 11:16:39 阅读量: 13 订阅数: 19 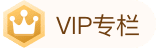
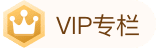
# 1. 章节一:理解MobX中的Actions和Action Creators
## 1.1 介绍MobX中的Actions和Action Creators
在MobX中,Actions和Action Creators扮演着至关重要的角色。它们用于改变应用状态并触发响应式更新,是在MobX中实现数据变化的关键方法。在本章节中,我们将深入介绍Actions和Action Creators的概念,以及它们在MobX数据流中的作用。
## 1.2 Actions和Action Creators的作用
Actions是对应用状态进行修改的纯函数,它们定义了对数据进行改变的逻辑。而Action Creators则是用于创建包裹Actions的函数,它们帮助我们组织和管理复杂的行为。
## 1.3 MobX中的数据流概念
在MobX中,数据流是指状态管理的一种模式,通过Actions来改变状态,并在状态发生变化时自动更新相关的组件。了解数据流的概念有助于我们更好地使用Actions和Action Creators来管理应用状态。
### 2. 章节二:创建和使用Actions
在MobX中,Actions是用来改变状态的函数,它可以是同步的,也可以是异步的。在这一章节中,我们将学习如何创建和使用Actions来管理应用的状态。
#### 2.1 创建简单的Action
在MobX中,我们可以使用`@action`装饰器来将普通函数转换成Action,从而可以修改状态。下面是一个简单的例子:
```python
from mobx import action, observable, configure
configure(allow_duplicate_actions=True)
class CounterStore:
def __init__(self):
self.count = 0
@action
def increment(self):
self.count += 1
counter = CounterStore()
print(counter.count) # 输出: 0
counter.increment()
print(counter.count) # 输出: 1
```
在这个例子中,`@action`装饰器将`increment`方法转换成了一个Action,当调用`increment`时,`count`会自动更新。
#### 2.2 异步操作和Action
有时候我们需要执行一些异步操作,比如从服务器获取数据。在MobX中,可以使用`@action`和`async/await`来处理异步操作:
```python
import asyncio
from mobx import action, observable, configure
configure(allow_duplicate_actions=True)
class UserStore:
def __init__(self):
self.users = []
@action
async def fetchUsers(self):
# 模拟从服务器获取数据的操作
await asyncio.sleep(1)
self.users = ['Alice', 'Bob', 'Chris']
user = UserStore()
print(user.users) # 输出: []
await user.fetchUsers()
print(user.users) # 输出: ['Alice', 'Bob', 'Chris']
```
在这个例子中,`fetchUsers`是一个异步的Action,使用`async/await`语法来模拟异步操作,并更新`users`状态。
#### 2.3 在React组件中使用Actions
在React组件中使用Actions非常简单,只需要将Actions作为方法传递给组件即可:
```jsx
import { observer } from 'mobx-react';
import React from 'react';
const CounterComponent = ({ counterStore }) => {
const handleIncrement = () => {
counterStore.increment();
};
return (
<div>
```
0
0
相关推荐
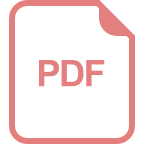
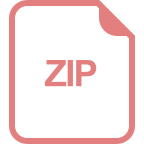
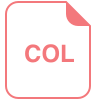




