PHP多数据库数据迁移艺术:跨库数据搬家的艺术,轻松实现数据迁移
发布时间: 2024-08-02 11:26:59 阅读量: 25 订阅数: 27 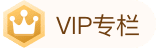
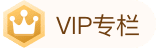
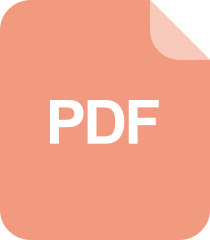
数据迁移的艺术:策略、实践与代码实现
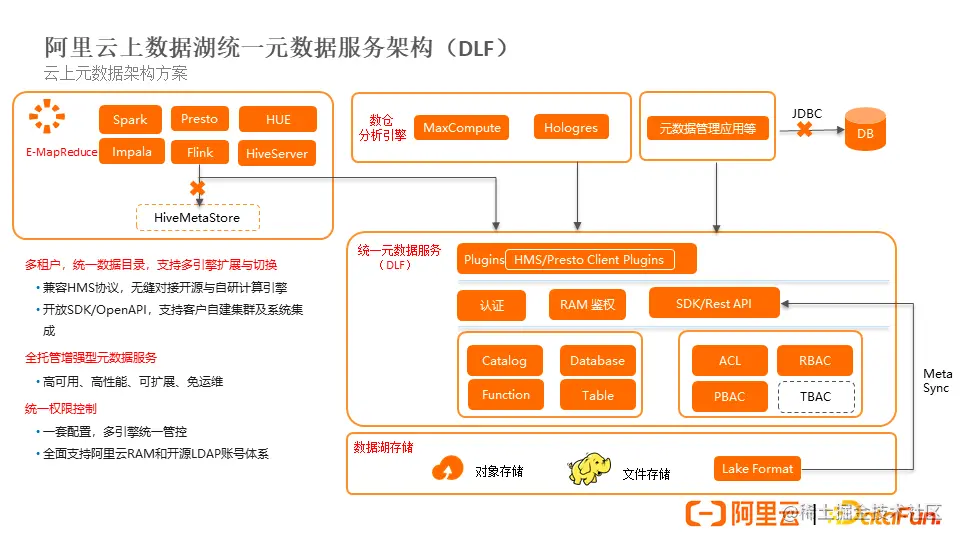
# 1. 数据迁移概述**
数据迁移是一种将数据从一个数据源(源数据库)传输到另一个数据源(目标数据库)的过程。它通常涉及在不同数据库系统之间移动数据,例如从关系数据库到 NoSQL 数据库。
数据迁移的类型包括:
- **同构迁移:**在相同类型的数据库系统之间移动数据,例如从 MySQL 迁移到 PostgreSQL。
- **异构迁移:**在不同类型的数据库系统之间移动数据,例如从 Oracle 迁移到 MongoDB。
# 2. PHP数据迁移基础
### 2.1 数据迁移的概念和类型
**数据迁移**是指将数据从一个数据源(源数据库)转移到另一个数据源(目标数据库)的过程。它通常用于以下场景:
- **数据库升级:**将旧数据库中的数据迁移到新版本或更高版本的数据库中。
- **数据库合并:**将多个数据库中的数据合并到一个单一数据库中。
- **数据备份和恢复:**将数据从生产数据库迁移到备份数据库,以实现数据保护。
- **数据分析和报告:**将数据从操作数据库迁移到数据仓库或数据湖中,以进行分析和报告。
**数据迁移类型**
根据迁移方式,数据迁移可以分为以下类型:
- **同构迁移:**源数据库和目标数据库使用相同的数据库管理系统(DBMS)。
- **异构迁移:**源数据库和目标数据库使用不同的DBMS。
- **全量迁移:**一次性将所有数据从源数据库迁移到目标数据库。
- **增量迁移:**分批次地将源数据库中的数据迁移到目标数据库,通常用于实时数据同步。
- **单向迁移:**数据只能从源数据库迁移到目标数据库,不能反向迁移。
- **双向迁移:**数据可以从源数据库和目标数据库之间双向迁移。
### 2.2 PHP数据迁移工具和技术
PHP提供了多种数据迁移工具和技术,包括:
**PDO(PHP数据对象)**
PDO是一个PHP扩展,提供了一个面向对象的方式来连接和操作不同的数据库。它支持多种数据库类型,包括MySQL、PostgreSQL、Oracle和SQL Server。
```php
// 连接到 MySQL 数据库
$dsn = 'mysql:host=localhost;dbname=my_database';
$username = 'root';
$password = 'secret';
try {
$conn = new PDO($dsn, $username, $password);
} catch (PDOException $e) {
echo 'Connection failed: ' . $e->getMessage();
}
```
**Mysqli**
Mysqli是一个PHP扩展,提供了与MySQL数据库交互的低级API。它比PDO更强大,但使用起来也更复杂。
```php
// 连接到 MySQL 数据库
$mysqli = new mysqli('localhost', 'root', 'secret', 'my_database');
if ($mysqli->connect_errno) {
echo 'Connection failed: ' . $mysqli->connect_error;
}
```
**SQLAlchemy**
SQLAlchemy是一个对象关系映射(ORM)框架,允许您使用Python对象来表示数据库中的数据。它支持多种数据库类型,包括MySQL、PostgreSQL和SQLite。
```python
from sqlalchemy import create_engine
# 连接到 MySQL 数据库
engine = create_engine('mysql+pymysql://root:secret@localhost/my_database')
```
**其他工具**
除了这些内置的PHP工具之外,还有许多第三方PHP数据迁移工具可用,例如:
- **Flyway**:一个用于管理数据库架构和数据迁移的工具。
- **Liquibase**:另一个用于管理数据库架构和数据迁移的工具。
- **DbUnit**:一个用于测试数据库迁移的工具。
# 3. 跨库数据迁移实践**
### 3.1 不同数据库的连接和操作
跨库数据迁移的第一步是建立与源数据库和目标数据库的连接。PHP提供了多种数据库连接器,例如:
- MySQLi:用于连接MySQL数据库
- PDO:用于连接多种数据库,包括MySQL、PostgreSQL、Oracle等
- Doctrine:一个对象关系映射(ORM)框架,可简化数据库操作
**连接示例(使用PDO):**
```php
$dsn = 'mysql:host=localhost;dbname=source_db';
$user = 'root';
$password = '';
try {
$conn = new PDO($dsn, $user, $password);
$conn->setAttribute(PDO::ATTR_ERRMODE, PDO::ERRMODE_EXCEPTION);
} catch (PDOException $e) {
echo 'Connection failed: ' . $e->getMessage();
}
```
连接数据库后,即可执行查询和操作。PHP提供了以下函数进行数据操作:
- `query()`:执行SQL查询并返回结果集
- `prepare()`:准备SQL语句,以便多次执行
- `execute()`:执行准备好的SQL语句
**查询示例:**
```php
$stmt = $conn->prepare('SELECT * FROM users');
$stmt->execute();
$users = $stmt->fetchAll(PDO::FETCH_ASSOC);
```
### 3.2 数据类型转换和数据一致性
在跨库数据迁移中,不同数据库的数据类型可能不一致。因此,需要进行数据类型转换以确保数据完整性。
**数据类型转换示例:**
| 源数据库数据类型 | 目标数据库数据类型 | 转换函数 |
|---|---|---|
| INT | INTEGER | (int) |
| VARCHAR | STRING | (string) |
| DATETIME | TIMESTAMP | date('Y-m-d H:i:s', strtotime($datetime)) |
**数据一致性检查:**
数据迁移后,需要检查数据是否与源数据库保持一致。可以使用以下方法进行一致性检查:
- 比较记录数
- 比较字段值
- 使用校验和或哈希函数
**一致性检
0
0
相关推荐
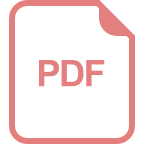
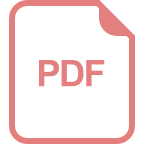
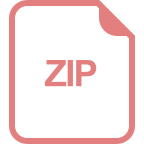
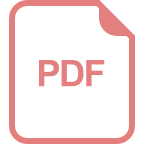
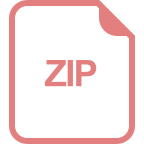
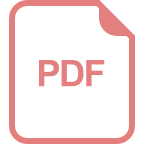
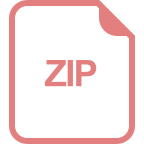
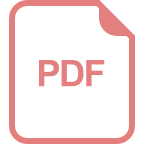