单片机程序设计流程:从需求分析到代码实现,一步步掌握
发布时间: 2024-07-08 22:47:24 阅读量: 71 订阅数: 42 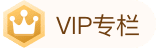
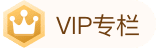
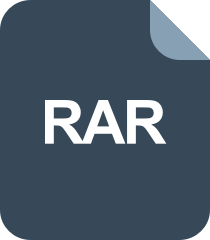
51单片机开发板实验:光敏电阻遮挡实验程序源代码.rar
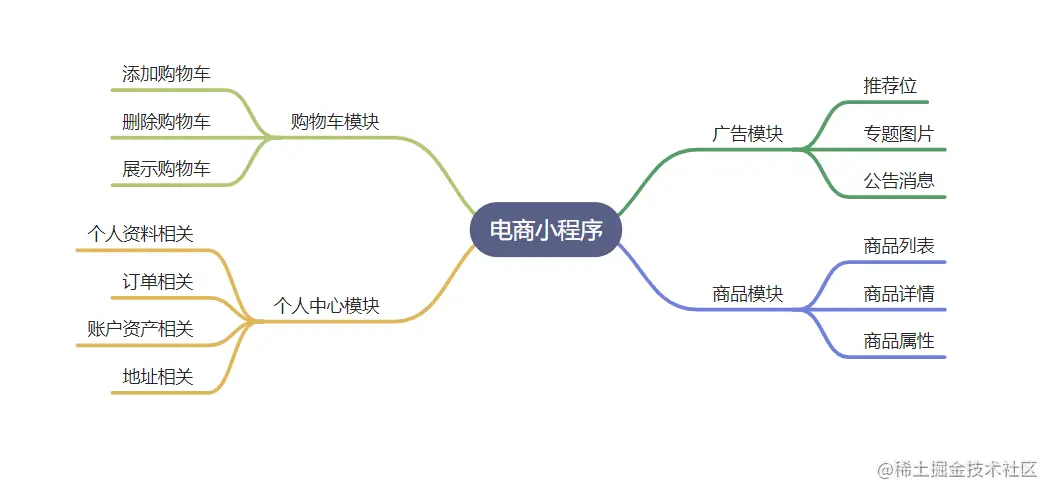
# 1. 单片机程序设计概述**
单片机程序设计是利用单片机芯片进行程序开发的过程,它涉及对单片机硬件架构、指令集、编程语言和开发工具的理解。单片机程序设计的主要目标是编写出能够控制单片机执行特定任务的程序,这些任务通常涉及数据采集、处理和输出。
单片机程序设计是一个多学科交叉的领域,它需要工程师具备计算机科学、电子工程和控制理论方面的知识。单片机程序设计过程通常包括需求分析、系统设计、算法设计、程序编码、调试、优化和验证等步骤。
# 2. 需求分析与系统设计
### 2.1 需求分析与建模
需求分析是单片机系统设计的第一步,其目的是明确系统功能、性能和约束条件。需求分析方法有多种,常用的有:
- **用例分析:**描述系统中不同用户或角色与系统交互的场景。
- **功能分解:**将系统功能分解成更小的子功能,逐层细化需求。
- **需求规格说明:**使用自然语言或建模工具描述系统需求,明确功能、性能、接口等方面。
### 2.2 系统架构设计
系统架构设计是将需求转化为系统结构的过程,其目的是定义系统的整体框架和组件之间的关系。常见的系统架构包括:
- **单片机架构:**所有功能都在单片机芯片上实现。
- **分布式架构:**系统功能分布在多个单片机或其他设备上,通过通信网络协同工作。
- **混合架构:**结合单片机和分布式架构的优点,实现灵活性和可扩展性。
### 2.3 硬件选型与接口设计
硬件选型是根据需求选择合适的单片机芯片和外围器件。需要考虑的因素包括:
- **性能:**处理速度、存储容量、I/O接口等。
- **功耗:**系统运行和待机功耗。
- **成本:**芯片和外围器件的采购成本。
接口设计是定义单片机与外围器件之间的数据交换方式。常用的接口类型包括:
- **并行接口:**数据一次性并行传输。
- **串行接口:**数据逐位串行传输。
- **总线接口:**多个设备共享一条数据总线。
```
// 并行接口示例代码
#define DATA_PORT PORTB
#define DATA_DIR DDRB
void write_parallel(uint8_t data) {
DATA_PORT = data;
}
uint8_t read_parallel() {
return DATA_PORT;
}
// 串行接口示例代码
#define TX_PIN PB0
#define RX_PIN PB1
void write_serial(uint8_t data) {
for (int i = 0; i < 8; i++) {
if (data & (1 << i)) {
PORTB |= (1 << TX_PIN);
} else {
PORTB &= ~(1 << TX_PIN);
}
_delay_ms(1);
}
}
uint8_t read_serial() {
uint8_t data = 0;
for (int i = 0; i < 8; i++) {
if (PINB & (1 << RX_PIN)) {
data |= (1 << i);
}
_delay_ms(1);
}
return data;
}
```
**逻辑分析:**
- `write_parallel`函数将数据写入并行接口,通过设置数据端口的值。
- `read_parallel`函数从并行接口读取数据,通过读取数据端口的值。
- `write_serial`函数将数据写入串行接口,逐位发送数据。
- `read_serial`函数从串行接口读取数据,逐位接收数据。
**参数说明:**
- `data`:要写入或读取的数据。
# 3. 算法设计与数据结构
### 3.1 算法设计原则
算法设计遵循以下基本原则:
- **正确性:**算法必须产生正确的结果,符合预期功能。
- **效率:**算法应尽可能高效,以减少时间和空间复杂度。
- **可读性:**算法应易于理解和维护,采用清晰的代码结构和注释。
- **通用性:**算法应尽可能通用,适用于各种输入和场景。
- **可扩展性:**算法应易于扩展和修改,以适应不断变化的需求。
### 3.2 常用数据结构
数据结构用于组织和存储数据,以提高算法效率和易用性。常见的数据结构包括:
- **数组:**有序的元素集合,通过索引访问。
- **链表:**元素通过指针链接,提供高效的插入和删除操作。
- **栈:**遵循后进先出(LIFO)原则的数据结构,用于函数调用和递归。
- **队列:**遵循先进先出(FIFO)原则的数据结构,用于消息传递和缓冲。
- **树:**分层的数据结构,用于组织和搜索数据。
### 3.3 数据结构与算法的应用
数据结构和算法相互关联,共同影响程序的性能和效率。以下是一些常见的应用:
- **数组:**线性搜索和排序算法。
- **链表:**插入排序和删除操作。
- **栈:**函数调用和递归。
- **队列:**消息传递和缓冲。
- **树:**二叉搜索树和哈希表。
#### 代码示例:二叉搜索树
```python
class Node:
def __init__(self, value):
self.value = value
self.left = None
self.right = None
class BinarySearchTree:
def __init__(self):
self.root = None
def insert(self, value):
if self.root is None:
self.root = Node(value)
else:
self._insert(value, self.root)
def _insert(self, value, node):
if value < node.value:
if node.left is None:
node.left = Node(value)
else:
self._insert(value, node.left)
else:
if node.right is None:
node.right = Node(value)
else:
self._insert(value, node.right)
def search(self, value):
if self.root is None:
return None
else:
return self._search(value, self.root)
def _search(self, value, node):
if value == node.value:
return node
elif value < node.value:
if node.left is None:
return None
else:
return self._search(value, node.left)
else:
if node.right is None:
return None
else:
return self._search(value, node.right)
```
#### 逻辑分析:
- **Node 类:**表示树中的节点,包含值和左右子节点指针。
- **BinarySearchTree 类:**表示二叉搜索树,包含根节点。
- **insert 方法:**插入一个新值,通过递归在树中找到适当的位置。
- **search 方法:**搜索一个值,通过递归在树中查找。
#### 参数说明:
- **value:**要插入或搜索的值。
- **node:**当前正在检查的节点。
# 4. 程序编码与调试
### 4.1 汇编语言简介
汇编语言是一种低级编程语言,它使用助记符来表示机器指令。汇编语言与机器语言一一对应,但比机器语言更易于理解和编写。
汇编语言程序由以下部分组成:
- 指令:表示要执行的操作。
- 操作数:指定要操作的数据。
- 伪指令:用于控制程序的编译和汇编过程。
### 4.2 程序编码规范
为了提高程序的可读性、可维护性和可移植性,应遵循以下编码规范:
- 使用缩进和空格来组织代码。
- 使用有意义的变量和函数名称。
- 使用注释来解释代码的逻辑。
- 避免使用跳转指令。
- 使用模块化设计,将程序分解成可管理的模块。
### 4.3 调试方法与工具
调试是识别和修复程序错误的过程。常用的调试方法包括:
- **单步执行:**逐条执行代码,观察变量的值。
- **断点:**在代码中设置断点,程序执行到断点时暂停。
- **日志记录:**在程序中添加日志语句,记录程序的执行信息。
常用的调试工具包括:
- **调试器:**提供单步执行、断点和日志记录等功能。
- **仿真器:**模拟单片机的行为,允许在计算机上调试程序。
- **逻辑分析仪:**捕获和分析单片机的信号,帮助诊断硬件问题。
#### 代码块:汇编语言程序示例
```汇编语言
; 定义一个函数
func:
; 保存寄存器
push r0
push r1
; 计算 a + b
mov r0, a
add r0, b
; 恢复寄存器
pop r1
pop r0
; 返回结果
ret
```
#### 逻辑分析:
- `push r0` 和 `push r1` 将寄存器 `r0` 和 `r1` 压入堆栈,保存它们的当前值。
- `mov r0, a` 将变量 `a` 的值加载到寄存器 `r0` 中。
- `add r0, b` 将寄存器 `r0` 中的值与变量 `b` 的值相加。
- `pop r1` 和 `pop r0` 将寄存器 `r1` 和 `r0` 从堆栈中弹出,恢复它们的值。
- `ret` 返回到调用函数的代码。
# 5.1 程序优化技巧
### 代码优化
**重用代码:**避免重复编写相同的代码,使用函数或宏来封装通用功能。
**消除冗余计算:**通过缓存中间结果或使用循环展开来避免重复计算。
**优化循环:**使用 for-range 循环代替 for-each 循环,并考虑使用并行循环。
**使用内联函数:**将小函数内联到调用位置,减少函数调用的开销。
**使用汇编代码:**对于关键的性能瓶颈,可以使用汇编代码来直接操作硬件,提高执行效率。
### 数据优化
**选择合适的数据类型:**根据变量的范围和精度选择合适的整数或浮点数类型。
**使用结构体和联合:**将相关数据组织成结构体或联合,提高内存访问效率。
**使用指针:**通过指针直接访问数据,避免不必要的复制。
**使用位操作:**利用位操作来进行高效的位级操作,如设置或清除单个位。
### 编译器优化
**启用优化选项:**在编译器中启用优化选项,如 -O2 或 -O3,以自动执行代码优化。
**使用编译器内联:**启用编译器内联,允许编译器将函数内联到调用位置。
**使用编译器特定的优化:**利用编译器特定的优化选项,如 GCC 的 -march=native,以针对特定硬件平台进行优化。
### 其他优化技巧
**使用性能分析工具:**使用性能分析工具,如 gprof 或 perf,来识别性能瓶颈并指导优化。
**进行基准测试:**对优化后的代码进行基准测试,以验证其性能改进。
**持续优化:**随着项目的发展,定期进行性能分析和优化,以保持代码的最佳性能。
# 6. 系统集成与应用
### 6.1 系统集成流程
单片机系统集成是一个复杂的过程,通常涉及以下步骤:
1. **需求分析:**确定系统的功能和性能要求。
2. **硬件设计:**选择合适的单片机、外围器件和电路。
3. **软件开发:**编写和调试单片机程序。
4. **系统组装:**将硬件和软件集成到一个完整的系统中。
5. **测试和调试:**验证系统是否满足要求并排除故障。
6. **系统部署:**将系统部署到实际应用中。
### 6.2 单片机系统应用实例
单片机系统广泛应用于各种领域,包括:
- **工业控制:**PLC、DCS、仪表等
- **消费电子:**手机、相机、家电等
- **汽车电子:**发动机控制、车载娱乐系统等
- **医疗器械:**监护仪、血糖仪等
- **通信设备:**路由器、交换机等
### 6.3 单片机系统发展趋势
单片机系统不断发展,主要趋势包括:
- **高性能:**单片机的处理能力和存储容量不断提高。
- **低功耗:**单片机功耗越来越低,适合于电池供电的设备。
- **集成度高:**单片机集成越来越多的外围功能,减少了外部器件的使用。
- **无线连接:**单片机越来越多地支持无线连接,如Wi-Fi、蓝牙等。
- **人工智能:**单片机开始支持人工智能功能,如机器学习和神经网络。
0
0
相关推荐
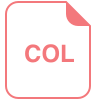
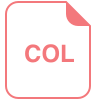
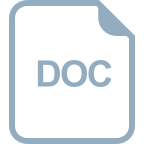
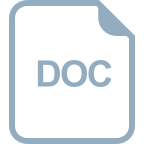
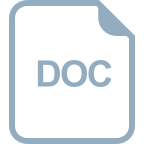
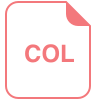
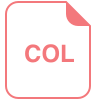
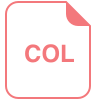