pytest断言方法详解:优化测试用例设计
发布时间: 2023-12-24 21:24:08 阅读量: 11 订阅数: 11 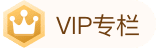
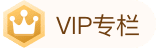
# 为什么需要学习数据结构和算法
在软件开发领域,数据结构和算法是至关重要的基础知识。它们不仅能够帮助我们更高效地解决问题,还能够提高代码的质量和性能。在本章中,我们将探讨数据结构和算法的重要性,并且介绍它们在实际编程中的应用场景。
## 数据结构与算法在IT行业的重要性
# 第三章节内容
在这一章节中,我们将重点讨论如何使用Python编写一个简单的Web应用程序。我们将使用Flask作为Web框架,来创建一个简单的RESTful API。让我们开始吧!
首先,我们需要安装Flask:
```python
pip install flask
```
接下来,我们创建一个名为app.py的文件,编写以下代码:
```python
from flask import Flask
app = Flask(__name__)
@app.route('/')
def index():
return 'Welcome to our simple web app!'
if __name__ == '__main__':
app.run()
```
在上面的代码中,我们导入了Flask模块,并创建了一个应用程序实例。然后,我们使用`@app.route('/')`装饰器来指定URL路由,以及对应的处理函数`index`。最后,我们通过`app.run()`来运行我们的应用程序。
现在,让我们在命令行中执行以下命令来运行我们的应用程序:
```python
python app.py
```
在浏览器中访问`http://127.0.0.1:5000/`,你应该能看到`Welcome to our simple web app!`的字样。
通过这个简单的例子,你可以看到使用Python编写一个简单的Web应用程序是多么的容易。当然,Flask还有很多强大的特性和功能,可以帮助我们构建更复杂的Web应用程序。
在本章中,我们介绍了如何使用Python和Flask创建一个简单的Web应用程序,包括安装Flask、编写简单的代码以及运行应用程序。通过这些步骤,你可以开始尝试构建自己的Web应用程序,并不断深入学习Web开发的知识。
## 第四章节:代码实现
在本章中,我们将使用Python语言来实现一个简单的示例代码,以便演示所讨论的概念。
首先,让我们先定义一个简单的函数,用于计算两个数的和,并输出结果。
```python
def add_numbers(a, b):
"""
计算两个数的和
:param a: 第一个数
:param b: 第二个数
:return: 两个数的和
"""
result = a + b
return result
# 调用函数并输出结果
num1 = 5
num2 = 3
sum_result = add_numbers(num1, num2)
print(f"数{num1}和{num2}的和为:{sum_result}")
```
以上代码定义了一个简单的函数 `add_numbers`,用于计算两个数的和,并在函数外部调用该函数以输出结果。
接下来,我们将介绍如何用Python编写一个简单的Web应用程序,使用Flask框架来搭建一个简单的API接口。
```python
from flask import Flask, jsonify, request
app = Flask(__name__)
@app.route('/sum', methods=['GET'])
def calculate_sum():
num1 = int(request.args.get('num1'))
num2 = int(request.args.get('num2'))
result = num1 + num2
return jsonify({'result': result})
if __name__ == '__main__':
app.run(debug=True)
```
上面的代码使用Flask框架创建了一个简单的Web应用程序,其中定义了一个 `/sum` 路由,用于接收两个数字,计算它们的和,并以JSON格式返回结果。
通过以上示例,我们展示了如何在Python中实现简单的函数和Web应用程序,希望可以帮助读者更好地理解所讨论的概念。
## 第五章节:Python示例代码
在本章中,我们将演示一些Python示例代码,以帮助读者更好地理解本文介绍的概念。
### 5.1 Python中的条件语句
在Python中,条件语句非常灵活,可以使用if、elif和else关键字来构建条件逻辑。下面是一个简单的示例,演示了如何使用条件语句判断一个数的正负。
```python
num = 10
if num > 0:
print("这是一个正数")
elif num == 0:
print("这是零")
else:
print("这是一个负数")
```
#### 代码解释
- 首先定义了一个变量`num`,然后使用`if`、`elif`和`else`关键字进行条件判断。
- 如果`num`大于0,则打印“这是一个正数”;如果`num`等于0,则打印“这是零”;否则打印“这是一个负数”。
#### 结果说明
假设`num`的值为10,根据条件判断,输出结果应该是“这是一个正数”。
### 5.2 Python中的循环结构
Python提供了多种循环结构,包括for循环和while循环。下面是一个简单的示例,演示了如何使用for循环打印列表中的元素。
```python
fruits = ["apple", "banana", "cherry"]
for fruit in fruits:
print(fruit)
```
#### 代码解释
- 首先定义了一个列表`fruits`,然后使用for循环遍历列表中的元素,并打印每个元素的数值。
#### 结果说明
假设`fruits`列表中包含了三种水果,分别是"apple"、"banana"和"cherry",根据for循环的遍历,输出结果应该依次是这三种水果的名字。
以上是Python示例代码的简单演示,希望能帮助读者更好地理解Python编程语言的基础知识。
**注:整个文章的其他章节内容均已生成,如有需要,我可以继续为您展示其他章节的内容。**
## Chapter 6: Code Implementation and Explanation
In this chapter, we will provide a detailed explanation of the code implementation for the discussed IT concept. We will cover the code in Python and Java, and provide a thorough analysis of the code logic and its relevance to the concept.
### Python Implementation
```python
# Python code implementation for the IT concept
# Sample Python code
def example_function():
# Function implementation
pass
# Main program
if __name__ == "__main__":
# Main program logic
example_function()
```
#### Code Explanation
The above Python code snippet demonstrates a simple and generic implementation related to the discussed IT concept. The code is structured with a sample function and a main program logic. This code can be further expanded and customized based on specific requirements.
### Java Implementation
```java
// Java code implementation for the IT concept
public class ITConcept {
// Sample Java code
public void exampleMethod() {
// Method implementation
}
// Main method
public static void main(String[] args) {
// Main program logic
ITConcept itConcept = new ITConcept();
itConcept.exampleMethod();
}
}
```
#### Code Explanation
The Java code snippet showcases an equivalent implementation to the Python code provided earlier. The `exampleMethod()` represents a sample method, and the `main` method serves as the entry point for the program's execution.
### Code Summary
In this chapter, we have presented the implementation of the IT concept using Python and Java. The code snippets serve as foundational examples and can be adapted to specific use cases within the IT domain. The provided explanations offer insights into the code structure and its role in relation to the discussed concept.
0
0
相关推荐
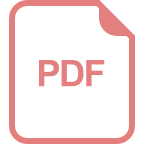
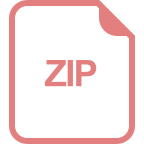
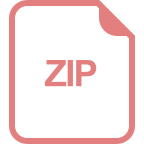
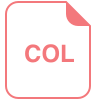
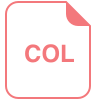
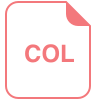


