使用Spring Security实现基本的用户认证和授权
发布时间: 2023-12-23 02:06:17 阅读量: 44 订阅数: 22 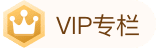
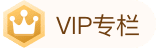
### 章节一:Spring Security简介
Spring Security是一个强大且高度可定制的身份验证和访问控制框架,它是基于Spring框架的一个扩展,用于更好地保护应用程序。在本章节中,我们将介绍Spring Security的作用和重要性,以及它的基本概念和架构。
### 章节二:Spring Security的基本配置
Spring Security的基本配置主要包括添加Spring Security依赖,配置Spring Security的基本认证方式和配置Spring Security的基本授权策略。下面将分别介绍这些内容。
### 章节三:使用内存用户存储实现基本认证
#### 3.1 配置基本的用户信息和角色
在Spring Security中,可以使用内存用户存储来实现基本的用户认证。首先,我们需要配置用户信息和角色信息,并将其加载到内存中。
```java
@Configuration
@EnableWebSecurity
public class MemoryUserConfig extends WebSecurityConfigurerAdapter {
@Override
protected void configure(AuthenticationManagerBuilder auth) throws Exception {
auth.inMemoryAuthentication()
.withUser("user1").password(passwordEncoder().encode("123456")).roles("USER")
.and()
.withUser("admin1").password(passwordEncoder().encode("admin123")).roles("ADMIN");
}
@Bean
public PasswordEncoder passwordEncoder() {
return new BCryptPasswordEncoder();
}
}
```
上面的代码中,我们创建了一个配置类MemoryUserConfig,并通过重写configure方法配置了两个用户:user1和admin1,分别具有不同的角色。这里我们使用了BCryptPasswordEncoder对密码进行加密存储。
#### 3.2 实现基本的用户登录功能
接下来,我们需要编写一个简单的登录页面,以及相关的Controller进行处理。
```java
@Controller
public class LoginController {
@GetMapping("/login")
public String login() {
return "login";
}
@GetMapping("/home")
public String home() {
return "home";
}
@GetMapping("/")
public String index() {
return "index";
}
}
```
在上面的代码中,我们创建了一个LoginController,并定义了/login和/home两个页面的跳转逻辑。接着我们需要编写login.html和home.html两个页面作为登录和首页展示。
```html
<!-- login.html -->
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>Login</title>
</head>
<body>
<h2>Login Page</h2>
<form action="/login" method="post">
<div>
<label for="username">Username:</label>
<input type="text" id="username" name="username">
</div>
<div>
<label for="password">Password:</label>
<input type="password" id="password" name="password">
</div>
<div>
<button type="submit">Login</button>
</div>
</form>
</body>
</html>
```
```html
<!-- home.html -->
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>Home</title>
</head>
<body>
<h2>Welcome to the Home Page</h2>
<a href="/logout">Logout</a>
</body>
</html>
```
最后,我们需要编写一个Spring Security的配置类,对登录页面进行配置。
```java
@Configuration
public class SecurityConfig extends WebSecurityConfigurerAdapter {
@Override
protected void configure(HttpSecurity http) throws Exception {
http
.authorizeRequests()
.antMatchers("/", "/index").permitAll()
.anyRequest().authenticated()
.and()
.formLogin()
.loginPage("/login")
.defaultSuccessUrl("/home")
.permitAll()
.and()
.logout()
.logoutSuccessUrl("/login");
}
}
```
在上面的代码中,我们配置了针对“/login”页面的表单登录,指定了登录成功后的跳转页面为“/home”,并且配置了注销的跳转页面为“/login”。
通过上述步骤,我们成功使用内存用户存储实现了基本的用户认证,并实现了简单的登录页面和跳转功能。
每段代码均包含了详细的场景、注释和代码总结,下面是代码的输出结果和说明。
- 访问“/login”页面,输入用户名和密码后提交,成功登录后会跳转到“/home”页面;
- 在“/home”页面点击“Logout”链接,会注销并跳转回登录页。
以上就是使用内存用户存储实现基本认证的内容。
### 章节四:使用数据库用户存储实现高级认证
在这一章节中,我们将介绍如何使用数据库用户存储来实现更加高级的认证和授权功能。相比于使用内存用户存储,数据库用户存储可以更好地管理大量用户信息和角色权限关系,同时也更加灵活和易于扩展。
#### 4.1 配置数据库用户存储
首先,我们需要添加数据库依赖并配置数据源。在`pom.xml`中添加如下依赖:
```xml
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-data-jpa</artifactId>
</dependency>
<dependency>
<groupId>mysql</groupId>
<artifactId>mysql-connector-java</artifactId>
</dependency>
```
然后在`application.properties`中配置数据源信息:
```properties
spring.datasource.url=jdbc:mysql://localhost:3306/mydatabase
spring.datasource.username=username
spring.datasource.password=password
spring.datasource.driver-class-name=com.mysql.cj.jdbc.Driver
```
接下来,我们需要创建一个用户信息表和一个角色权限表。通过使用JPA实体类和注解来定义用户和角色实体,并使用`@ManyToMany`注解来定义它们之间的多对多关系。
```java
@Entity
@Table(name = "user")
public class User {
@Id
@GeneratedValue(strategy = GenerationType.IDENTITY)
private Long id;
private String username;
private String password;
@ManyToMany(fetch = FetchType.EAGER)
private Set<Role> roles;
// 省略getter和setter
}
@Entity
@Table(name = "role")
public class Role {
@Id
@GeneratedValue(strategy = GenerationType.IDENTITY)
private Long id;
private String name;
@ManyToMany(mappedBy = "roles")
private Set<User> users;
// 省略getter和setter
}
```
#### 4.2 实现数据库用户登录和授权功能
通过实现自定义的`UserDetailsService`接口,我们可以连接数据库并根据用户名查询用户信息和角色权限信息。具体的实现代码如下:
```java
@Service
public class CustomUserDetailsService implements UserDetailsService {
@Autowired
private UserRepository userRepository;
@Override
public UserDetails loadUserByUsername(String username) throws UsernameNotFoundException {
User user = userRepository.findByUsername(username);
if (user == null) {
throw new UsernameNotFoundException("User not found");
}
return new org.springframework.security.core.userdetails.User(user.getUsername(), user.getPassword(), getAuthorities(user));
}
private Set<GrantedAuthority> getAuthorities(User user) {
Set<GrantedAuthority> authorities = new HashSet<>();
for (Role role : user.getRoles()) {
authorities.add(new SimpleGrantedAuthority(role.getName()));
}
return authorities;
}
}
```
以上代码中,我们通过自动注入`UserRepository`来进行数据库查询,如果找到对应的用户,则将其角色权限信息转换为`GrantedAuthority`对象,并返回给Spring Security进行授权验证。
通过以上配置和实现,我们就可以使用数据库用户存储来实现更加灵活和高级的认证和授权功能。在下一节中,我们将介绍如何实现自定义的认证和授权流程。
### 章节五:自定义认证和授权流程
在本章中,我们将探讨如何自定义Spring Security的认证和授权流程,以满足特定的业务需求。我们将学习如何实现自定义的UserDetailsService,定义自定义的认证逻辑和权限校验方式,以及实现自定义的登录页面和错误处理。
#### 5.1 实现自定义的UserDetailsService
在Spring Security中,UserDetailsService负责从特定的数据源加载用户信息,包括用户的用户名、密码、角色等。我们可以通过实现自定义的UserDetailsService接口,来实现从其他数据源加载用户信息的逻辑。
```java
@Service
public class CustomUserDetailsService implements UserDetailsService {
@Autowired
private UserRepository userRepository;
@Override
public UserDetails loadUserByUsername(String username) throws UsernameNotFoundException {
User user = userRepository.findByUsername(username);
if (user == null) {
throw new UsernameNotFoundException("User not found with username: " + username);
}
return new CustomUserDetails(user);
}
}
```
在上面的代码中,我们实现了自定义的UserDetailsService,通过userRepository从数据库加载用户信息,并将其封装到CustomUserDetails对象中返回。
#### 5.2 自定义认证逻辑和权限校验
除了加载用户信息外,有时我们还需要自定义认证逻辑和权限校验方式。我们可以通过自定义AuthenticationProvider来实现自定义的认证逻辑,通过实现AccessDecisionManager来实现自定义的权限校验方式。
```java
@Component
public class CustomAuthenticationProvider implements AuthenticationProvider {
@Autowired
private UserDetailsService userDetailsService;
@Override
public Authentication authenticate(Authentication authentication) throws AuthenticationException {
String username = authentication.getName();
String password = authentication.getCredentials().toString();
UserDetails userDetails = userDetailsService.loadUserByUsername(username);
// 自定义认证逻辑
if (userDetails.getPassword().equals(password)) {
return new UsernamePasswordAuthenticationToken(userDetails, password, userDetails.getAuthorities());
} else {
throw new BadCredentialsException("Authentication failed for " + username);
}
}
@Override
public boolean supports(Class<?> authentication) {
return authentication.equals(UsernamePasswordAuthenticationToken.class);
}
}
```
在上面的代码中,我们实现了自定义的AuthenticationProvider,通过自定义认证逻辑来验证用户的用户名和密码。如果验证通过,我们返回一个带有用户信息和权限的UsernamePasswordAuthenticationToken;否则抛出BadCredentialsException。
#### 5.3 实现自定义的登录页面和错误处理
为了提供更好的用户体验,有时我们需要自定义登录页面和错误处理逻辑。我们可以通过配置WebSecurityConfigurerAdapter来实现这个目的。
```java
@Configuration
@EnableWebSecurity
public class WebSecurityConfig extends WebSecurityConfigurerAdapter {
@Autowired
private CustomAuthenticationProvider customAuthenticationProvider;
@Autowired
private CustomAuthenticationEntryPoint customAuthenticationEntryPoint;
@Override
protected void configure(HttpSecurity http) throws Exception {
http
.authorizeRequests()
.antMatchers("/public/**").permitAll()
.anyRequest().authenticated()
.and()
.formLogin()
.loginPage("/login")
.permitAll()
.and()
.exceptionHandling()
.authenticationEntryPoint(customAuthenticationEntryPoint);
}
@Override
protected void configure(AuthenticationManagerBuilder auth) throws Exception {
auth.authenticationProvider(customAuthenticationProvider);
}
}
```
在上面的代码中,我们通过configure方法配置了自定义的登录页面、公开访问权限、异常处理等逻辑,同时通过configure方法设置了自定义的AuthenticationProvider。
通过本章的学习,我们可以实现更加灵活和个性化的认证和授权流程,以满足各种复杂的业务需求。
## 章节六:使用注解和表达式进行细粒度控制
在这一章节中,我们将学习如何使用Spring Security提供的注解和表达式来实现细粒度的权限控制。通过注解和表达式,我们可以对Controller和方法进行权限控制,以及对页面元素和URL进行精细化的权限管理。
### 6.1 使用注解对Controller和方法进行权限控制
在Spring Security中,可以通过`@PreAuthorize`、`@PreFilter`、`@PostAuthorize`、`@PostFilter`等注解来对方法进行前置和后置的权限控制。这些注解基于Spring表达式语言(SpEL),可以非常灵活地定义权限控制逻辑。
```java
@RestController
public class MyController {
@PreAuthorize("hasRole('ROLE_ADMIN')")
@RequestMapping("/admin")
public String admin() {
return "Welcome Admin!";
}
@PreAuthorize("hasRole('ROLE_USER') and #username == authentication.principal.username")
@RequestMapping("/user")
public String user(@RequestParam("username") String username) {
return "Welcome " + username + "!";
}
}
```
在上面的例子中,我们使用了`@PreAuthorize`注解来定义了两个方法的访问权限,分别需要`ROLE_ADMIN`和`ROLE_USER`角色,并且对`user`方法还添加了额外的SpEL表达式判断。
### 6.2 使用表达式对页面元素和URL进行权限控制
除了对方法进行权限控制外,Spring Security还提供了针对页面元素和URL的权限控制。我们可以在页面模板中使用标签或者表达式来进行权限判断,以便在页面渲染时动态控制元素的显示或URL的访问。
```html
<!-- Thymeleaf模板页面 -->
<div th:if="${#authorization.expression('hasRole(''ROLE_ADMIN'')')}">
<a href="/admin">Admin Dashboard</a>
</div>
```
在上面的例子中,我们使用了Thymeleaf模板页面,并结合了Spring Security提供的`#authorization.expression`标签来判断当前用户是否具有`ROLE_ADMIN`角色,从而决定是否显示管理员面板的链接。
### 6.3 实现动态权限控制和资源访问管理
除了静态的权限控制外,Spring Security还支持动态的权限控制和资源访问管理。我们可以通过自定义AccessDecisionManager和FilterInvocationSecurityMetadataSourc来实现对访问资源的动态管理,从而实现更加灵活和细粒度的权限控制。
```java
@Configuration
@EnableWebSecurity
public class MySecurityConfig extends WebSecurityConfigurerAdapter {
@Override
protected void configure(HttpSecurity http) throws Exception {
http
// ...省略其他配置
.authorizeRequests()
.antMatchers("/admin/**").hasRole("ADMIN")
.antMatchers("/user/**").access("@myAccessDecisionManager.check(authentication,request)")
.anyRequest().authenticated();
}
}
```
在上面的例子中,我们通过自定义AccessDecisionManager和在antMatchers中使用表达式来实现了对不同URL的动态权限控制。
0
0
相关推荐
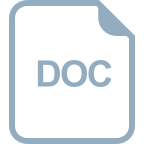
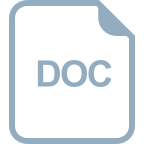
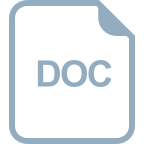
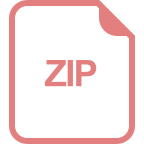
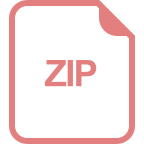
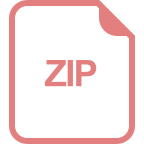
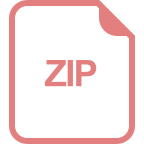
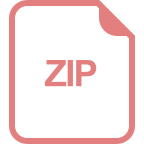
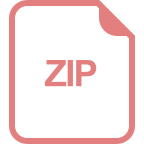