【Spring Boot框架实战指南】:从入门到精通的进阶之旅(20个实战案例带你掌握核心技术)
发布时间: 2024-07-20 20:02:25 阅读量: 20 订阅数: 33 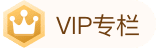
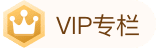
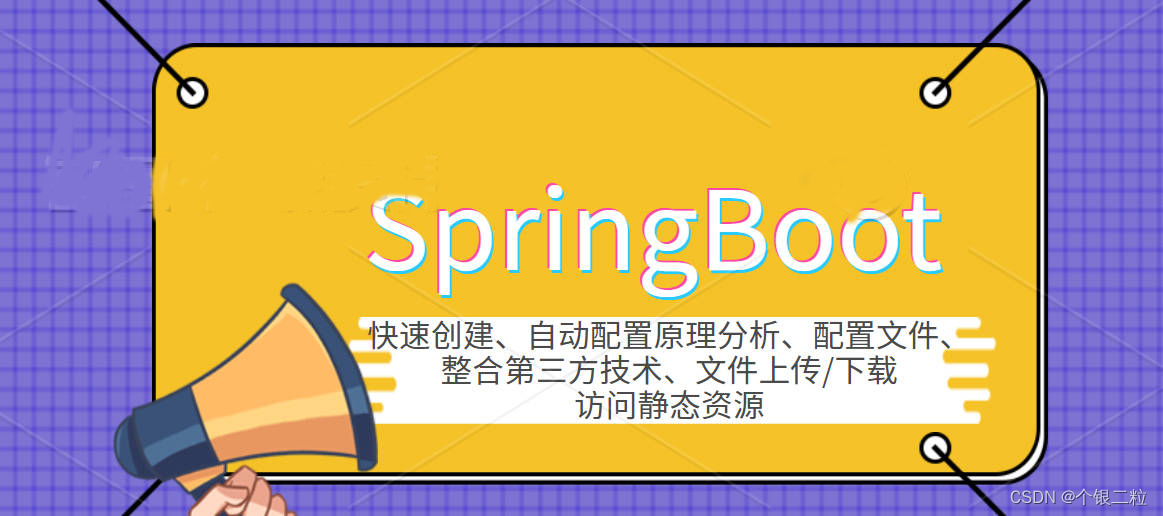
# 1. Spring Boot入门**
Spring Boot是一个开源框架,用于简化基于Java的应用程序开发。它通过自动配置、Starter和Actuator等特性,提供了开箱即用的功能,减少了开发人员的配置和集成工作量。
**1.1 Spring Boot的优势**
* **快速启动:**Spring Boot通过自动配置简化了应用程序的启动过程,无需手动配置繁琐的XML或Java代码。
* **开箱即用:**Spring Boot提供了各种Starter,包含了常用的依赖项和配置,方便开发者快速集成第三方库。
* **监控和管理:**Spring Boot Actuator提供了一组端点,用于监控和管理应用程序的运行状况,包括健康检查、指标收集和配置管理。
# 2. Spring Boot核心技术
### 2.1 Spring Boot自动配置
#### 2.1.1 自动配置原理
Spring Boot自动配置是通过`@SpringBootApplication`注解实现的,该注解整合了多个其他注解,包括`@Configuration`、`@EnableAutoConfiguration`和`@ComponentScan`。
- `@Configuration`:表明该类是一个配置类,用于定义Spring Bean。
- `@EnableAutoConfiguration`:启用Spring Boot自动配置。
- `@ComponentScan`:扫描指定包及其子包下的Spring Bean。
Spring Boot自动配置的原理是:
1. Spring Boot会扫描`@SpringBootApplication`注解所在的包及其子包。
2. 查找并加载所有带有`@Configuration`注解的类,这些类称为配置类。
3. 对于每个配置类,Spring Boot会扫描其内部的`@Bean`注解,这些注解定义了Spring Bean。
4. Spring Boot会根据一定的规则自动选择和加载合适的Spring Bean。
#### 2.1.2 自定义自动配置
Spring Boot允许用户自定义自动配置,以满足特定的需求。可以通过以下步骤实现:
1. 创建一个新的`@Configuration`类。
2. 在该类中定义一个或多个`@Bean`注解的方法。
3. 在`@Bean`注解的方法上添加`@ConditionalOnMissingBean`注解,以确保只有在Spring容器中不存在同类型的Bean时才加载该Bean。
```java
@Configuration
public class MyAutoConfiguration {
@Bean
@ConditionalOnMissingBean
public MyService myService() {
return new MyServiceImpl();
}
}
```
### 2.2 Spring Boot Starter
#### 2.2.1 Starter的组成和使用
Spring Boot Starter是一个预先配置好的依赖项集合,用于简化特定技术的集成。每个Starter包含了一组相关的依赖项,以及一些额外的配置,以简化这些依赖项的配置。
要使用Spring Boot Starter,只需在项目的pom.xml文件中添加以下依赖项:
```xml
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-<module-name></artifactId>
</dependency>
```
其中`<module-name>`是需要集成的模块的名称,例如`web`、`data-jpa`或`security`。
#### 2.2.2 创建自己的Starter
也可以创建自己的Spring Boot Starter,以简化特定库或框架的集成。要创建Starter,需要:
1. 创建一个新的Maven项目。
2. 在pom.xml文件中添加`spring-boot-starter`作为父项目。
3. 在pom.xml文件中添加所需的依赖项。
4. 创建一个`@Configuration`类,以提供额外的配置。
5. 将Starter打包为JAR文件。
### 2.3 Spring Boot Actuator
#### 2.3.1 Actuator的特性和作用
Spring Boot Actuator是一个用于监控和管理Spring Boot应用的工具。它提供了以下特性:
- 端点(Endpoint):用于访问应用程序状态和执行操作的HTTP端点。
- 指标(Metric):应用程序运行时收集的度量数据,例如CPU使用率和内存使用情况。
- 健康检查(Health Check):用于检查应用程序是否正常运行的健康检查。
#### 2.3.2 使用Actuator监控和管理应用
要使用Actuator,需要在pom.xml文件中添加以下依赖项:
```xml
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-actuator</artifactId>
</dependency>
```
然后,可以在`application.properties`文件中配置Actuator端点:
```properties
management.endpoints.web.exposure.include=*
```
这将启用所有Actuator端点。可以通过以下URL访问端点:
```
http://localhost:8080/actuator
```
# 3.1 RESTful API开发
#### 3.1.1 Spring Boot构建RESTful API
Spring Boot提供了强大的RESTful API支持,使开发人员能够轻松创建和管理RESTful API。要构建RESTful API,可以使用以下步骤:
1. **创建Spring Boot项目:**使用Spring Initializr创建一个新的Spring Boot项目,并选择"Web"依赖项。
2. **定义控制器:**创建控制器类,并使用`@RestController`和`@RequestMapping`注解定义RESTful API端点。
3. **处理HTTP请求:**使用`@GetMapping`、`@PostMapping`、`@PutMapping`和`@DeleteMapping`注解处理不同的HTTP请求类型。
4. **返回响应:**使用`@ResponseBody`注解将数据返回给客户端。
5. **测试API:**使用Postman或其他工具测试RESTful API。
#### 3.1.2 RESTful API的测试和调试
测试和调试RESTful API至关重要,以确保其正确性和可靠性。Spring Boot提供了以下测试和调试工具:
1. **JUnit测试:**使用JUnit测试控制器类中的RESTful API方法。
2. **MockMvc:**使用MockMvc模拟HTTP请求和响应,以便进行单元测试。
3. **Postman:**使用Postman发送HTTP请求并查看响应,以便进行手动测试。
4. **Spring Boot Actuator:**使用Actuator的`health`和`info`端点监控和调试RESTful API。
#### 代码示例
以下代码示例展示了如何使用Spring Boot构建一个简单的RESTful API:
```java
@RestController
@RequestMapping("/api/users")
public class UserController {
@GetMapping
public List<User> getAllUsers() {
// Fetch all users from the database
return userRepository.findAll();
}
@PostMapping
public User createUser(@RequestBody User user) {
// Save the user to the database
return userRepository.save(user);
}
@PutMapping("/{id}")
public User updateUser(@PathVariable Long id, @RequestBody User user) {
// Update the user in the database
User existingUser = userRepository.findById(id).orElseThrow(() -> new ResourceNotFoundException("User not found with id: " + id));
existingUser.setName(user.getName());
existingUser.setEmail(user.getEmail());
return userRepository.save(existingUser);
}
@DeleteMapping("/{id}")
public void deleteUser(@PathVariable Long id) {
// Delete the user from the database
userRepository.deleteById(id);
}
}
```
#### 代码逻辑分析
此代码定义了一个`UserController`,其中包含处理HTTP请求的RESTful API方法:
* `getAllUsers`方法使用`@GetMapping`注解处理GET请求,并返回所有用户的列表。
* `createUser`方法使用`@PostMapping`注解处理POST请求,并保存一个新的用户到数据库中。
* `updateUser`方法使用`@PutMapping`注解处理PUT请求,并更新数据库中指定ID的用户。
* `deleteUser`方法使用`@DeleteMapping`注解处理DELETE请求,并从数据库中删除指定ID的用户。
# 4. Spring Boot进阶技术
### 4.1 Spring Boot与Spring Cloud整合
#### 4.1.1 Spring Cloud Eureka服务注册与发现
Spring Cloud Eureka是一个服务注册与发现组件,它允许微服务注册自己并发现其他微服务。Eureka使用一个分布式的、基于键值的存储系统来存储服务信息。
**Eureka的工作原理**
Eureka使用客户端-服务器架构。Eureka客户端是运行在每个微服务中的代理,负责将微服务注册到Eureka服务器。Eureka服务器是一个独立的应用程序,负责存储和管理服务信息。
当一个微服务启动时,其Eureka客户端会向Eureka服务器发送注册请求。注册请求包含微服务的信息,例如其名称、IP地址和端口号。Eureka服务器将这些信息存储在键值存储系统中。
当一个微服务需要发现另一个微服务时,其Eureka客户端会向Eureka服务器发送发现请求。发现请求包含要发现的微服务名称。Eureka服务器将返回该微服务的信息,包括其IP地址和端口号。
**使用Eureka进行服务注册与发现**
要使用Eureka进行服务注册与发现,需要在每个微服务中添加Eureka客户端依赖。然后,需要配置Eureka客户端,指定Eureka服务器的地址。
```java
@SpringBootApplication
public class EurekaClientApplication {
public static void main(String[] args) {
SpringApplication.run(EurekaClientApplication.class, args);
}
@Bean
public EurekaInstanceConfigBean eurekaInstanceConfigBean() {
EurekaInstanceConfigBean bean = new EurekaInstanceConfigBean();
bean.setHostname("localhost");
bean.setPort(8080);
return bean;
}
@Bean
public EurekaClientConfigBean eurekaClientConfigBean() {
EurekaClientConfigBean bean = new EurekaClientConfigBean();
bean.setServiceUrl("http://localhost:8761/eureka/");
return bean;
}
}
```
在Eureka服务器中,需要配置Eureka服务器的端口号和集群名称。
```properties
eureka.client.register-with-eureka=false
eureka.client.fetch-registry=false
eureka.server.port=8761
eureka.cluster.name=my-cluster
```
启动Eureka服务器和微服务后,微服务将自动注册到Eureka服务器,并可以相互发现。
#### 4.1.2 Spring Cloud Config配置管理
Spring Cloud Config是一个配置管理组件,它允许微服务从一个集中式存储库获取其配置。Config使用一个Git存储库来存储配置信息。
**Config的工作原理**
Config使用客户端-服务器架构。Config客户端是运行在每个微服务中的代理,负责从Config服务器获取配置信息。Config服务器是一个独立的应用程序,负责存储和管理配置信息。
当一个微服务启动时,其Config客户端会向Config服务器发送获取配置请求。获取配置请求包含微服务的信息,例如其名称和环境。Config服务器将返回该微服务配置信息。
**使用Config进行配置管理**
要使用Config进行配置管理,需要在每个微服务中添加Config客户端依赖。然后,需要配置Config客户端,指定Config服务器的地址和配置信息存储的Git存储库。
```java
@SpringBootApplication
public class ConfigClientApplication {
public static void main(String[] args) {
SpringApplication.run(ConfigClientApplication.class, args);
}
@Bean
public ConfigClientConfigFactoryBean configClientConfigFactoryBean() {
ConfigClientConfigFactoryBean bean = new ConfigClientConfigFactoryBean();
bean.setUri("http://localhost:8888");
bean.setName("config-client");
bean.setProfile("dev");
return bean;
}
}
```
在Config服务器中,需要配置Config服务器的端口号和Git存储库的地址。
```properties
spring.cloud.config.server.git.uri=https://github.com/my-org/my-repo
spring.cloud.config.server.port=8888
```
启动Config服务器和微服务后,微服务将自动从Config服务器获取其配置信息。
# 5.1 性能优化
### 5.1.1 Spring Boot性能优化技巧
Spring Boot提供了多种优化性能的技巧,包括:
- **使用缓存:**缓存可以将经常访问的数据存储在内存中,从而减少对数据库或其他慢速存储的访问次数。Spring Boot提供了多种缓存实现,如Ehcache和Caffeine。
- **优化数据库查询:**数据库查询是性能瓶颈的常见来源。可以通过使用索引、避免连接查询和使用批量操作来优化查询。
- **使用异步处理:**异步处理允许应用程序在不阻塞主线程的情况下执行任务。这对于处理长时间运行的任务或I/O密集型任务非常有用。
- **使用CDN:**CDN(内容分发网络)可以将静态内容(如图像和CSS文件)存储在离用户更近的位置。这可以减少加载时间并提高应用程序的性能。
- **使用微服务架构:**微服务架构将应用程序分解成更小的、独立的服务。这可以提高可扩展性和性能,因为每个服务可以独立优化。
### 5.1.2 性能监控和分析工具
Spring Boot提供了多种工具来监控和分析应用程序的性能,包括:
- **Spring Boot Actuator:**Actuator提供了一组端点,用于监控应用程序的健康状况、指标和配置。
- **Spring Boot Admin:**Admin是一个基于Actuator的管理工具,用于监控和管理多个Spring Boot应用程序。
- **JMX:**JMX(Java管理扩展)是一个用于监控和管理Java应用程序的标准。Spring Boot提供了一个JMX端点,用于访问应用程序的MBean。
- **Prometheus:**Prometheus是一个开源监控系统,用于收集和存储应用程序指标。Spring Boot提供了Prometheus端点,用于导出应用程序指标。
通过使用这些工具,可以识别性能瓶颈并实施优化措施以提高应用程序的性能。
# 6.1 电商系统
**6.1.1 系统架构设计**
电商系统是一个复杂的系统,涉及到商品管理、订单管理、支付管理、物流管理等多个模块。其系统架构通常采用分层架构,分为以下几个层:
- **展示层:**负责用户界面和交互,展示商品信息、订单信息等。
- **服务层:**负责业务逻辑处理,如订单处理、支付处理等。
- **数据访问层:**负责与数据库交互,获取和存储数据。
**6.1.2 Spring Boot实现**
使用Spring Boot框架构建电商系统,可以大大提高开发效率和系统稳定性。Spring Boot提供了丰富的功能和组件,可以快速构建RESTful API、集成数据库、实现安全认证等。
**商品管理**
```java
@Entity
public class Product {
@Id
@GeneratedValue(strategy = GenerationType.IDENTITY)
private Long id;
private String name;
private double price;
private int stock;
// 省略其他属性和方法
}
```
```java
@RestController
@RequestMapping("/api/products")
public class ProductController {
@Autowired
private ProductService productService;
@GetMapping
public List<Product> getAllProducts() {
return productService.getAllProducts();
}
@GetMapping("/{id}")
public Product getProductById(@PathVariable Long id) {
return productService.getProductById(id);
}
// 省略其他方法
}
```
**订单管理**
```java
@Entity
public class Order {
@Id
@GeneratedValue(strategy = GenerationType.IDENTITY)
private Long id;
private Long userId;
private List<OrderItem> orderItems;
private double totalPrice;
// 省略其他属性和方法
}
```
```java
@RestController
@RequestMapping("/api/orders")
public class OrderController {
@Autowired
private OrderService orderService;
@PostMapping
public Order createOrder(@RequestBody Order order) {
return orderService.createOrder(order);
}
@GetMapping
public List<Order> getAllOrders() {
return orderService.getAllOrders();
}
// 省略其他方法
}
```
**支付管理**
```java
@RestController
@RequestMapping("/api/payments")
public class PaymentController {
@Autowired
private PaymentService paymentService;
@PostMapping
public Payment createPayment(@RequestBody Payment payment) {
return paymentService.createPayment(payment);
}
@GetMapping
public List<Payment> getAllPayments() {
return paymentService.getAllPayments();
}
// 省略其他方法
}
```
**物流管理**
```java
@RestController
@RequestMapping("/api/logistics")
public class LogisticsController {
@Autowired
private LogisticsService logisticsService;
@PostMapping
public Logistics createLogistics(@RequestBody Logistics logistics) {
return logisticsService.createLogistics(logistics);
}
@GetMapping
public List<Logistics> getAllLogistics() {
return logisticsService.getAllLogistics();
}
// 省略其他方法
}
```
0
0
相关推荐
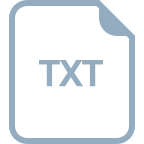
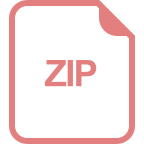
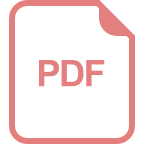





