11. 使用Redux在SPFx中进行状态管理
发布时间: 2024-01-09 00:20:42 阅读量: 10 订阅数: 11 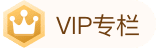
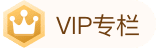
# 1. 介绍SPFx和Redux
## 1.1 什么是SPFx?
SharePoint Framework(简称SPFx)是一种用于开发SharePoint Online和SharePoint 2016的现代Web部件和扩展的模型。它允许开发人员使用现代Web技术(如React和TypeScript)构建高度可定制的SharePoint用户界面。
## 1.2 什么是Redux?
Redux是一个用于JavaScript应用程序的可预测状态容器。它是一个轻量级的库,可以帮助我们管理应用程序的状态。
## 1.3 为什么在SPFx中使用Redux进行状态管理?
在大型应用程序中,状态管理变得复杂且容易出错。而使用Redux可以帮助我们更好地组织和管理应用程序的状态,提高代码的可维护性和扩展性。
在SPFx中使用Redux可以带来以下几个好处:
- 集中化的状态管理:Redux将应用程序的状态集中存储在一个单一的store中,方便进行统一管理和调试。
- 可预测的状态变化:通过使用Redux的单向数据流和纯函数reducer来处理状态变化,我们可以更好地预测和控制应用程序的状态。
- 异步操作的处理:Redux配合中间件(如redux-thunk)可以很好地处理异步操作,如数据获取和API调用等。
- 与React的兼容性:Redux与React非常配合,可以帮助我们更好地管理React组件的状态。
接下来,我们将学习如何在SPFx中集成和使用Redux来进行状态管理。
# 2. 在SPFx中集成Redux
### 2.1 安装和配置Redux
首先,在SPFx项目目录中打开命令行窗口,使用以下命令安装Redux和相关依赖:
```bash
npm install redux react-redux @types/react-redux
```
安装完成后,我们需要在SPFx应用的主要入口文件(通常是index.tsx)中引入Redux的必要组件和函数:
```typescript
import { createStore, applyMiddleware } from 'redux';
import { Provider } from 'react-redux';
import thunk from 'redux-thunk';
```
### 2.2 创建Redux store和reducer
在SPFx项目的src文件夹中创建一个新文件夹,命名为store。在store文件夹中创建一个新文件,命名为index.ts。在index.ts文件中,我们将配置Redux的store和reducer:
```typescript
import { createStore, applyMiddleware, combineReducers } from 'redux';
import thunk from 'redux-thunk';
// 定义初始状态
const initialState = {
counter: 0
};
// 创建reducer函数
function counterReducer(state = initialState, action) {
switch (action.type) {
case 'INCREMENT':
return { ...state, counter: state.counter + 1 };
case 'DECREMENT':
return { ...state, counter: state.counter - 1 };
default:
return state;
}
}
// 合并reducer
const rootReducer = combineReducers({
counter: counterReducer
});
// 创建store
const store = createStore(rootReducer, applyMiddleware(thunk));
export default store;
```
### 2.3 在SPFx组件中引入Redux
在需要使用Redux进行状态管理的SPFx组件中,我们首先需要引入React和Redux相关的组件和函数:
```typescript
import * as React from 'react';
import { connect } from 'react-redux';
import { RootState } from '../../store';
```
然后,通过connect函数将组件与Redux store连接起来,并从store中获取需要的state和dispatch方法:
```typescript
interface CounterProps {
counter: number;
increment: () => void;
decrement: () => void;
}
class CounterComponent extends React.Component<CounterProps, {}> {
render() {
const { counter, increment, decrement } = this.props;
return (
<div>
<p>Counter: {counter}</p>
<button onClick={increment}>Increment</button>
<button onClick={decrement}>Decrement</button>
</div>
);
}
}
const mapStateToProps = (state: RootState) => ({
counter: state.counter
});
const mapDispatchToProps = {
increment: () => ({ type: 'INCREMENT' }),
decrement: () => ({ type: 'DECREMENT' })
};
export const Counter = connect(
mapStateToProps,
mapDispatchToProps
)(CounterComponent);
```
在上述代码中,mapStateToProps函数将Redux store中的state映射为组件的props,mapDispatchToProps函数将dispatch方法映射为组件的props。
最后,使用connect函数将CounterComponent与Redux store连接起来,并导出最终的Counter组件,供其他组件使用。
```typescript
import * as React from 'react';
import * as ReactDom from 'react-dom';
import { Provider } from 'react-redux';
import store from './store';
import { Counter } from './components/Counter';
ReactDom.render(
<Provider store={store}>
<Counter />
</Provider>,
document.getElementById('root')
);
```
在SPFx应用的入口文件中通过Provider组件将Redux store注入整个应用,并将Counter组件包裹在Provider中。
到此为止,我们已经完成了在SPFx中集成Redux的工作。接下来,我们将学习如何使用Redux来管理SPFx组件的状态。
以上是第二章节,在SPFx中集成Redux,包含了安装和配置Redux、创建Redux store和reducer以及在SPFx组件中引入Redux的内容。接下来的章节将进一步介绍如何使用Redux管理SPFx组件的状态,以及如何优化SPFx应用的性能。
0
0
相关推荐
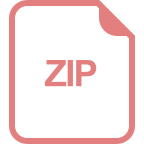
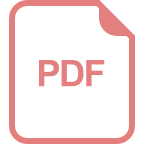
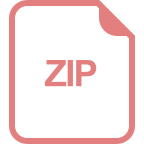
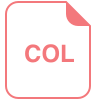
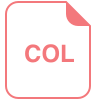
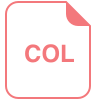
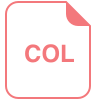

