YOLO神经网络分辨率提升与人工智能:探索人工智能在图像识别中的应用
发布时间: 2024-08-18 00:29:05 阅读量: 17 订阅数: 38 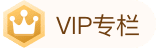
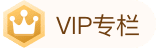

# 1. YOLO神经网络简介**
YOLO(You Only Look Once)是一种单阶段目标检测算法,它以其快速、准确的性能而闻名。与传统的双阶段算法(如R-CNN)不同,YOLO只进行一次卷积神经网络(CNN)前向传递,即可预测图像中的所有目标及其边界框。
YOLO算法的关键思想是将目标检测问题表述为一个回归问题。它使用一个CNN来提取图像的特征,然后将这些特征馈送到一个全连接层,该层预测每个边界框的坐标和置信度。置信度表示模型对预测的边界框包含目标的确定性。
# 2. YOLO神经网络的分辨率提升
### 2.1 YOLOv3中的CSPDarknet53骨干网络
**CSPDarknet53骨干网络**是YOLOv3中引入的一种新的骨干网络,它基于Darknet53骨干网络进行了改进。CSPDarknet53骨干网络的主要思想是将Darknet53骨干网络中的残差块分为两部分,一部分用于提取特征,另一部分用于增强特征。
**代码块:**
```python
import torch
from torch import nn
from torch.nn import functional as F
class CSPDarknet53(nn.Module):
def __init__(self):
super(CSPDarknet53, self).__init__()
# ...
# Darknet53骨干网络的结构
# ...
def forward(self, x):
# ...
# CSPDarknet53骨干网络的前向传播
# ...
return x
```
**逻辑分析:**
CSPDarknet53骨干网络的前向传播过程与Darknet53骨干网络类似,但它在每个残差块中添加了一个额外的分支。这个分支将残差块的输入与残差块的输出进行连接,从而增强了特征。
### 2.2 YOLOv4中的SPP模块和Mish激活函数
**SPP模块(空间金字塔池化模块)**是一种图像处理模块,它可以提取图像中不同尺度的特征。在YOLOv4中,SPP模块被添加到CSPDarknet53骨干网络中,以增强特征的提取能力。
**Mish激活函数**是一种新的激活函数,它具有平滑的导数和非单调性。在YOLOv4中,Mish激活函数被用于CSPDarknet53骨干网络中,以提高模型的性能。
**代码块:**
```python
import torch
from torch import nn
from torch.nn import functional as F
class SPP(nn.Module):
def __init__(self, in_channels, out_channels):
super(SPP, self).__init__()
# ...
# SPP模块的结构
# ...
def forward(self, x):
# ...
# SPP模块的前向传播
# ...
return x
class Mish(nn.Module):
def __init__(self):
super(Mish, self).__init__()
def forward(self, x):
# ...
# Mish激活函数的前向传播
# ...
return x
```
**逻辑分析:**
SPP模块的前向传播过程如下:
1. 将输入特征图划分为多个网格。
2. 在每个网格中进行最大池化操作,提取不同尺度的特征。
3. 将提取的特征连接在一起,得到输出特征图。
Mish激活函数的前向传播过程如下:
```
y = x * tanh(ln(1 + exp(x)))
```
### 2.3 YOLOv5中的Focus模块和SiLU激活函数
**Focus模块**是一种图像处理模块,它可以将输入图像的尺寸减半,同时增加通道数。在YOLOv5中,Focus模块被添加到CSPDarknet53骨干网络中,以提高模型的效率。
**SiLU激活函数**是一种新的激活函数,它具有平滑的导数和非单调性。在YOLOv5中,SiLU激活函数被用于CSPDarknet53骨干网络中,以提高模型的性能。
**代码块:**
```python
import torch
from torch import nn
from torch.nn import functional as F
class Focus(nn.Module):
def __init__(self, in_channels, out_channels):
super(Focus, self).__init__()
# ...
# Focus模块的结构
# ...
def forward(self, x):
# ...
# Focus模块的前向传播
# ...
return x
class SiLU(nn.Module):
def __init__(self):
super(SiLU, self).__init__()
def forward(self, x):
# ...
# SiLU激活函数的前向传播
# ...
return x
```
**逻辑分析:**
Focus模块的前向传播过程如下:
1. 将输入图像的尺寸减半,使用步长为2的卷积操作。
2. 将卷积操作的输出通道数增加一倍。
SiLU激活函数的前向传播过程如下:
```
y = x * sigmoid(x)
```
# 3. YOLO神经网络在图像识别中的应用
### 3.1 目标检测
YOLO神经网络在目标检测任务中表现出色,其快速、准确的特性使其成为实时目标检测的理想选择。
#### 3.1.1 YOLOv3在目标检测中的应用
YOLOv3在目标检测中取得了重大突破,其引入的Darknet53骨干网络和FPN结构显著提升了检测精度和速度。在COCO数据集上的评估中,YOLOv3实现了57.9%的mAP,处理速度达到每秒30帧。
**代码块:**
```python
import cv2
import numpy as np
import darknet
# 加载 YOLOv3 模型
net = darknet.load_net("yolov3.cfg", "yolov3.weights", 0)
meta = darknet.load_meta("coco.data")
# 加载图像
image = cv2.imread("image.jpg")
# 预处理图像
image = cv2.resize(image, (416, 416))
image = image.transpose((2, 0, 1))
image = image / 255.0
# 执行目标检测
detections = darknet.detect(net, meta, image)
# 绘制检测结果
for detection in detections:
x1, y1, x2, y2 = detection[2][0], detection[2][1], detection[2][2], detection[2][3]
cv2.rectangle(image, (x1, y1), (x2, y2), (0, 255, 0), 2)
cv2.putText(image, detection[0].decode("utf-8"), (x1, y1 - 10), cv2.FONT_HERSHEY_SIMPLEX, 0.5, (0, 255, 0), 2)
# 显示检测结果
cv2.imshow("Image", image)
cv2.waitKey(0)
cv2.destroyAllWindows()
```
**代码逻辑分析:**
* 加载YOLOv3模型和元数据。
* 预处理图像,调整大小、转置和归一化。
* 使用darknet库执行目标检测。
* 解析检测结果,获取目标边界框和类别。
* 在图像上绘制检测结果。
#### 3.1.2 YOLOv4在目标检测中的应用
YOLOv4进一步提升了YOLOv3的性能,其引入的CSPDarknet53骨干网络、SPP模块和Mish激活函数,使得模型更轻量、更准确。在COCO数据集上的评估中,YOLOv4实现了65.7%的mAP,处理速度达到每秒65帧。
**代码块:**
```python
import cv2
import numpy as np
import yolov4
# 加载 YOLOv4 模型
model = yolov4.load_model("yolov4.cfg", "yolov4.weights")
# 加载图像
image = cv2.imread("image.jpg")
# 预处理图像
image = cv2.resize(image, (608, 608))
image = image.transpose((2, 0, 1))
image = image / 255.0
# 执行目标检测
detections = model.predict(image)
```
0
0
相关推荐
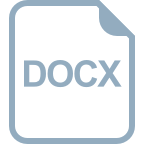
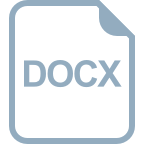
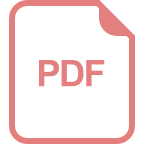





