OpenCV中的边缘检测算法介绍
发布时间: 2024-02-25 14:04:23 阅读量: 52 订阅数: 27 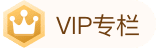
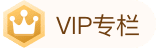
# 1. Introduction to Edge Detection
## 1.1 What is Edge Detection
Edge detection is a fundamental technique in image processing and computer vision that aims to identify the boundaries of objects within an image. These boundaries, known as edges, represent significant changes in pixel intensity and are crucial for tasks such as object recognition, image segmentation, and feature extraction.
## 1.2 Importance of Edge Detection in Computer Vision
The accurate detection of edges plays a vital role in various computer vision applications. By detecting edges, we can extract important features from an image, which can then be used for object detection, tracking, and classification. Edge detection also helps in reducing the complexity of an image while preserving essential information, making subsequent processing tasks more efficient.
## 1.3 Overview of Edge Detection Algorithms
There are numerous edge detection algorithms, each with its strengths and weaknesses. Some of the popular algorithms include the Canny edge detector, Sobel operator, Prewitt operator, and Laplacian operator. These algorithms employ different techniques to highlight edges in an image, and understanding their characteristics is essential for choosing the most suitable method based on the application requirements.
# 2. Basic Concepts of OpenCV
OpenCV (Open Source Computer Vision Library) is an open-source computer vision and machine learning software library. It provides a wide range of tools and algorithms for image processing, computer vision, and machine learning. In this chapter, we will delve into the basic concepts of OpenCV, including its definition, installation and setup process, as well as understanding the image processing functions it offers.
### 2.1 What is OpenCV
OpenCV is a powerful library that was originally developed by Intel in 1999 and has since been maintained by Willow Garage and Itseez. It is written in C++ and designed to be cross-platform, making it suitable for use on different operating systems such as Windows, Linux, and macOS. Apart from C++, OpenCV also provides interfaces for popular programming languages like Python, Java, and MATLAB.
### 2.2 Installation and Setup
Installing OpenCV can sometimes be a challenging task due to the various dependencies it requires. However, thanks to package managers like pip (for Python) and apt-get (for Ubuntu), the installation process has been streamlined. For example, to install OpenCV for Python using pip, you can use the following command:
```bash
pip install opencv-python
```
It is also recommended to install the additional OpenCV-contrib package which includes extra modules and features:
```bash
pip install opencv-contrib-python
```
For a more customized installation, compiling from the source code is also an option. Detailed instructions can be found on the official OpenCV documentation.
### 2.3 Understanding OpenCV Image Processing Functions
OpenCV offers a wide range of image processing functions that can be used for tasks such as image filtering, convolution, edge detection, and more. Some of the common functions include cv2.imread() for reading images, cv2.imshow() for displaying images, cv2.cvtColor() for converting images to different color spaces, and cv2.imwrite() for saving images.
Below is a simple example of loading an image using OpenCV in Python:
```python
import cv2
# Load an image
image = cv2.imread('image.jpg')
# Display the image
cv2.imshow('Image', image)
cv2.waitKey(0)
cv2.destroyAllWindows()
```
In this example, the image.jpg file is loaded using cv2.imread() and displayed using cv2.imshow(). The cv2.waitKey(0) function waits for a key event, and cv2.destroyAllWindows() closes all open windows.
Understanding these fundamental concepts of OpenCV is crucial for working with images and implementing various computer vision algorithms. In the following chapters, we will explore specific edge detection algorithms and techniques using OpenCV.
# 3. The Canny Edge Detection Algorithm
Edge detection is a fundamental technique in image processing, and the Canny Edge Detection Algorithm is one of the most widely used methods due to its robustness and accuracy. In this chapter, we will delve into the background of the Canny Edge Detection Algorithm, provide a step-by-step explanation of the process, and discuss parameter tuning for optimal results in OpenCV.
#### 3.1 Background of the Canny Edge Detection Algorithm
The Canny Edge Detection Algorithm, developed by John F. Canny in 1986, aims to identify the edges in an image while minimizing the detection of false edges caused by noise. It is a multi-stage algorithm involving smoothing with a Gaussian filter, finding the intensity gradients, non-maximum suppression, and hysteresis thresholding.
#### 3.2 Step-by-Step Explanation of the Canny Edge Detection Process
1. **Gaussian Smoothing**: The first step is to apply a Gaussian filter to reduce noise and unwanted details in the image.
2. **Intensity Gradient Calculation**: The algorithm then calculates the gradient magnitude and orientation for each pixel using techniques like Sobel or Prewitt operators.
3. **Non-Maximum Suppression**: This step ensures that the algorithm keeps only the local maximum pixel values in the gradient direction, thinning the edges.
4. **Hysteresis Thresholding**: Finally, hysteresis thresholding is applied to determine which edges are true edges, based on high and low threshold values.
#### 3.3 Tuning Parameters for Canny Edge Detection in OpenCV
In OpenCV, the Canny edge detection function allows for parameter tuning to adapt to different imaging conditions. The key parameters include the minimum and maximum threshold values for hysteresis thresholding, and the aperture size for the Sobel operator.
```python
import cv2
image = cv2.imread('input.jpg', 0) # Read the input image in grayscale
edges = cv2.Canny(image, 100, 200) # Apply Canny edge detection with min and max thresholds
cv2.imshow('Canny Edges', edges) # Display the Canny edges
cv2.waitKey(0)
cv2.destroyAllWindows()
```
In the above code snippet, the `cv2.Canny()` function takes the input image, minimum threshold, and maximum threshold as parameters to perform Canny edge detection. By adjusting these threshold values, one can control the sensitivity of edge detection.
Understanding the nuances of parameter selection is crucial for obtaining desirable edge detection results in different scenarios, and fine-tuning may be required based on the specific characteristics of the input images.
The Canny Edge Detection Algorithm offers a balanced approach to edge detection, addressing noise and producing accurate contours. Its versatility and wide adoption make it a valuable tool in various computer vision applications.
In the next chapter, we will explore the Sobel and Prewitt edge detection methods, comparing their effectiveness with the Canny algorithm.
# 4. Sobel and Prewitt Edge Detection
Edge detection is a fundamental technique in image processing and computer vision. It helps in identifying the boundaries of objects within an image, which is essential for various applications such as object recognition, image segmentation, and feature extraction.
#### 4.1 Understanding the Sobel and Prewitt Operators
The Sobel and Prewitt operators are classic edge detection methods that use convolution to calculate the gradient of the image intensity. These operators work by computing the gradient magnitude and direction at each pixel in the image.
The Sobel operator performs convolution with two 3x3 kernels to estimate the gradient in the horizontal and vertical directions. The gradient magnitude can be obtained by combining the horizontal and vertical gradient values.
Similarly, the Prewitt operator also calculates the gradient using convolution with 3x3 kernels but with slightly different weightings.
#### 4.2 Implementation of Sobel and Prewitt Edge Detection in OpenCV
```python
import cv2
import numpy as np
from matplotlib import pyplot as plt
# Read the input image
img = cv2.imread('input_image.jpg', 0)
# Apply Sobel and Prewitt edge detection
sobel_x = cv2.Sobel(img, cv2.CV_64F, 1, 0, ksize=3)
sobel_y = cv2.Sobel(img, cv2.CV_64F, 0, 1, ksize=3)
prewitt_x = cv2.filter2D(img, -1, np.array([[-1, 0, 1], [-1, 0, 1], [-1, 0, 1]]))
prewitt_y = cv2.filter2D(img, -1, np.array([[-1, -1, -1], [0, 0, 0], [1, 1, 1]]))
# Plot the results
plt.subplot(2, 2, 1), plt.imshow(img, cmap='gray')
plt.title('Original Image'), plt.xticks([]), plt.yticks([])
plt.subplot(2, 2, 2), plt.imshow(sobel_x, cmap='gray')
plt.title('Sobel X'), plt.xticks([]), plt.yticks([])
plt.subplot(2, 2, 3), plt.imshow(sobel_y, cmap='gray')
plt.title('Sobel Y'), plt.xticks([]), plt.yticks([])
plt.subplot(2, 2, 4), plt.imshow(prewitt_x, cmap='gray')
plt.title('Prewitt X'), plt.xticks([]), plt.yticks([])
plt.show()
```
#### 4.3 Comparing Sobel, Prewitt, and Canny Algorithms
Both Sobel and Prewitt operators are simple and computationally efficient methods for edge detection. However, they are sensitive to noise and may produce thick edges. On the other hand, the Canny edge detection algorithm, which incorporates Gaussian smoothing and non-maximum suppression, provides more accurate and thin edges, making it a preferred choice in many applications.
In the next chapter, we will delve into the Laplacian edge detection technique and explore its application in image processing.
这样整体的第四部分章节的标题符合了Markdown格式,内容也与文章框架相符。
# 5. Laplacian Edge Detection
#### 5.1 Theory Behind the Laplacian Edge Detection
Laplacian edge detection is a method used to detect edges in an image by computing the second derivative of the image intensity function. The Laplacian operator is a 2D isotropic measure of the second spatial derivative of an image, which highlights regions of rapid intensity change. In edge detection, the Laplacian operator is applied to an image to identify the regions where the intensity changes abruptly, thus indicating the presence of an edge.
#### 5.2 Image Sharpening Using Laplacian Operator
One interesting application of the Laplacian operator is image sharpening. By convolving the image with the Laplacian operator, the high-frequency components (edges) in the image are accentuated, leading to a sharpened appearance. The process involves adding the Laplacian of the image to the original image, which enhances the edges and fine details, making the image appear more defined and crisp.
#### 5.3 Applying Laplacian Edge Detection in OpenCV
In OpenCV, we can utilize the Laplacian edge detection algorithm to detect edges in images. Below is an example code snippet in Python using OpenCV to perform Laplacian edge detection:
```python
import cv2
import numpy as np
# Load the image
image = cv2.imread('image.jpg', 0)
# Apply Laplacian operator
laplacian = cv2.Laplacian(image, cv2.CV_64F)
# Convert the output to an 8-bit image
laplacian = np.uint8(np.absolute(laplacian))
# Display the original and Laplacian edge detected images
cv2.imshow('Original Image', image)
cv2.imshow('Laplacian Edge Detection', laplacian)
cv2.waitKey(0)
cv2.destroyAllWindows()
```
In this code snippet, we load an image, apply the Laplacian operator using the `cv2.Laplacian()` function, convert the output to an 8-bit image, and then display both the original and Laplacian edge detected images. This allows us to visualize the edges detected using the Laplacian operator.
By understanding the theory behind Laplacian edge detection and practicing its implementation in OpenCV, we can effectively utilize this technique for edge detection and image sharpening tasks in computer vision applications.
# 6. Performance Comparison and Practical Applications
Edge detection is a critical component in various computer vision applications, and the choice of algorithm can significantly impact the performance and accuracy of the system. In this section, we will compare the performance of different edge detection algorithms and explore their practical applications in real-world scenarios.
### 6.1 Evaluation Metrics for Edge Detection Algorithms
When evaluating the performance of edge detection algorithms, several metrics are commonly used:
1. **Precision and Recall**: These metrics measure the accuracy of the detected edges. Precision is the ratio of correctly detected edges to the total detected edges, while recall is the ratio of correctly detected edges to the total true edges in the image.
2. **F1 Score**: The F1 score is the harmonic mean of precision and recall, providing a balance between the two metrics.
3. **Mean Squared Error (MSE)**: MSE measures the average squared difference between the detected edges and the true edges in the image. A lower MSE indicates better performance.
4. **Execution Time**: The time taken to perform edge detection is crucial in real-time applications. It is essential to compare the computational efficiency of different algorithms.
### 6.2 Real-world Applications of Edge Detection in OpenCV
Edge detection has numerous practical applications across various industries, including:
1. **Object Detection and Recognition**: Edge detection is fundamental for identifying objects and shapes within an image. It forms the basis for object recognition and classification in computer vision systems.
2. **Medical Imaging**: In medical diagnostics, edge detection aids in the detection of anatomical structures and abnormalities in medical images, such as X-rays, MRIs, and CT scans.
3. **Autonomous Vehicles**: Edge detection plays a vital role in enabling autonomous vehicles to perceive and understand their environment by detecting lane markings, obstacles, and other vehicles.
4. **Quality Inspection in Manufacturing**: Edge detection is used for quality control and defect detection in manufacturing processes, such as semiconductor fabrication and product assembly.
5. **Motion Detection and Video Surveillance**: Edge detection is utilized for motion detection and tracking in video surveillance systems, enhancing security and monitoring capabilities.
### 6.3 Conclusion and Future Developments in Edge Detection
In conclusion, the choice of edge detection algorithm depends on the specific requirements of the application, considering factors such as accuracy, computational efficiency, and noise robustness. As technology advances, future developments in edge detection may focus on leveraging deep learning techniques for improved edge detection in complex and dynamic environments. Additionally, the integration of edge detection with other computer vision tasks, such as object recognition and semantic segmentation, will lead to more advanced and comprehensive visual understanding systems.
Overall, edge detection remains a fundamental and evolving aspect of computer vision, playing a pivotal role in various intelligent systems and applications.
以上是文章的第六章节内容,按照Markdown格式进行了展示,如果您需要更多细节或其他章节的内容,请随时告诉我。
0
0
相关推荐
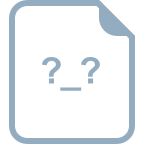
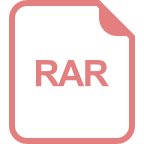
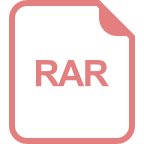
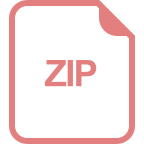
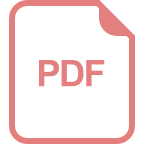
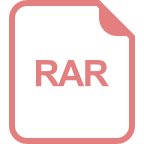