提升人脸识别精度:OpenCV for Unity人脸检测与追踪算法优化
发布时间: 2024-08-10 08:18:01 阅读量: 50 订阅数: 28 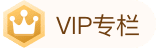
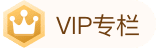
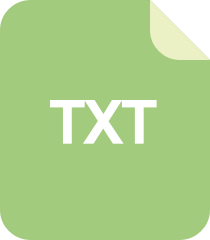
Unity3D 图像识别 人脸识别插件 OpenCV for Unity 2.2.1
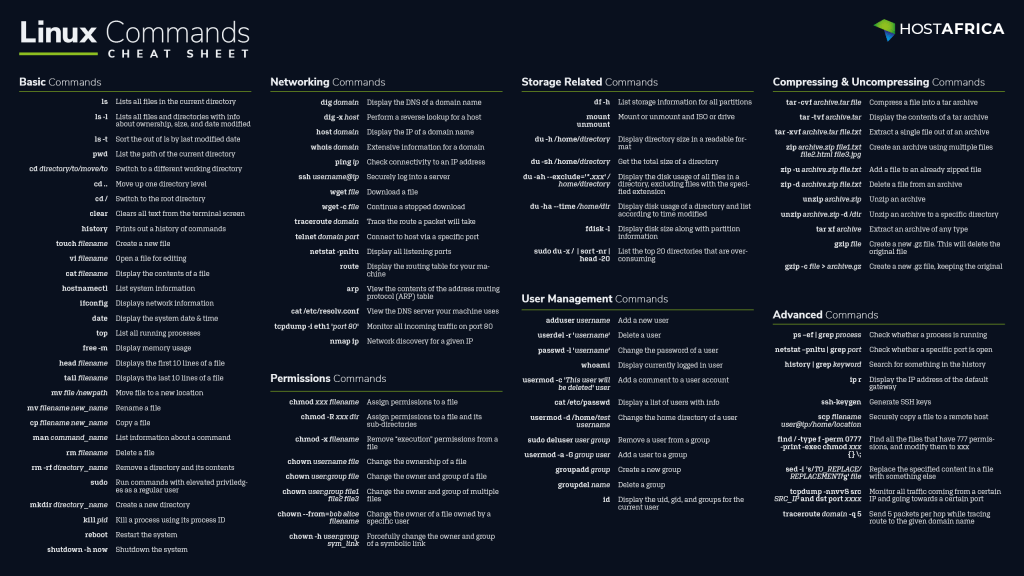
# 1. 人脸识别基础**
人脸识别是一种计算机视觉技术,它使计算机能够识别和验证人脸。它广泛应用于安全、执法和娱乐等领域。人脸识别的基本原理是分析人脸图像中的特征,如眼睛、鼻子和嘴巴,并将其与已知的数据库进行比较。
人脸识别算法通常分为两类:基于特征的算法和基于学习的算法。基于特征的算法,如Haar级联分类器,使用手工设计的特征来检测人脸。基于学习的算法,如深度学习模型,从大量标注的人脸图像中学习特征。
# 2. OpenCV for Unity人脸检测与追踪算法
### 2.1 OpenCV for Unity简介
#### 2.1.1 OpenCV for Unity的安装和配置
**安装步骤:**
1. 下载Unity Package Manager并安装OpenCV for Unity包。
2. 在Unity项目中导入OpenCV for Unity包。
3. 配置OpenCV for Unity的路径和设置。
**配置设置:**
* **OpenCV for Unity Path:**指定OpenCV for Unity库的安装路径。
* **Target Platform:**选择目标平台(例如,Android、iOS)。
* **Additional C++ Compiler Flags:**添加任何必要的编译器标志。
#### 2.1.2 OpenCV for Unity的架构和功能
**架构:**
* **Native C++库:**包含OpenCV核心功能。
* **Unity C# API:**为Unity开发者提供对C++库的访问。
* **Plugin Manager:**管理插件的加载和卸载。
**功能:**
* **图像处理:**图像增强、滤波、分割等。
* **人脸识别:**人脸检测、追踪、识别。
* **物体检测:**物体检测、分类、跟踪。
* **增强现实:**图像叠加、三维物体追踪。
### 2.2 人脸检测算法
#### 2.2.1 Haar级联分类器
**原理:**
Haar级联分类器是一种机器学习算法,用于检测特定对象(例如人脸)。它使用一组预训练的特征来识别对象。
**实现:**
```csharp
using OpenCVForUnity;
using UnityEngine;
public class FaceDetectionHaar : MonoBehaviour
{
private CascadeClassifier faceClassifier;
private Mat frame;
void Start()
{
faceClassifier = new CascadeClassifier(Utils.getFilePath("haarcascade_frontalface_default.xml"));
frame = new Mat();
}
void Update()
{
WebCamTexture webcamTexture = GetComponent<WebCamTexture>();
webcamTexture.GetCopy(frame);
MatOfRect faces = new MatOfRect();
faceClassifier.detectMultiScale(frame, faces);
// Draw rectangles around detected faces
for (int i = 0; i < faces.rows(); i++)
{
Rect faceRect = faces.toArray()[i];
Imgproc.rectangle(frame, faceRect, new Scalar(0, 255, 0), 2);
}
}
}
```
**参数说明:**
* **frame:**输入图像。
* **faces:**输出检测到的面部矩形。
* **scaleFactor:**图像缩放比例。
* **minNeighbors:**每个检测矩形周围的最小邻居数。
* **minSize:**最小检测矩形大小。
* **maxSize:**最大检测矩形大小。
#### 2.2.2 深度学习模型
**原理:**
深度学习模型,如YOLOv5,使用卷积神经网络(CNN)检测和识别对象。它们比Haar级联分类器更准确,但计算成本也更高。
**实现:**
```csharp
using OpenCVForUnity;
using UnityEngine;
public class FaceDetectionYOLOv5 : MonoBehaviour
{
private Net net;
private Mat frame;
void Start()
{
net = new Net(Utils.getFilePath("yolov5s.weights"), Utils.getFilePath("yolov5s.cfg"));
frame = new Mat();
}
void Update()
{
WebCamTexture webcamTexture = GetComponent<WebCamTexture>();
webcamTexture.GetCopy(frame);
Mat blob = Dnn.blobFromImage(frame, 1 / 255.0, new Size(416, 416), new Scalar(0, 0, 0), true, false);
net.setInput(blob);
Mat detections = net.forward();
// Parse detections
for (int i = 0; i < detections.rows(); i++)
{
float confidence = detections.get(i, 2)[0];
if (confidence > 0.5)
{
int classId = (int)detections.get(i, 1)[0];
if (classId == 0) // Person
```
0
0
相关推荐
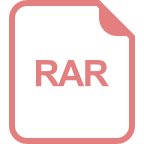
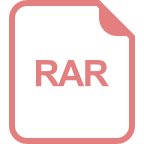
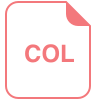
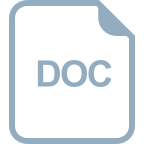
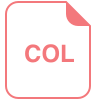
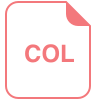
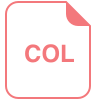
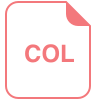