使用MQL5创建自定义指标
发布时间: 2023-12-20 11:09:00 阅读量: 41 订阅数: 33 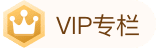
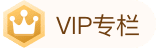
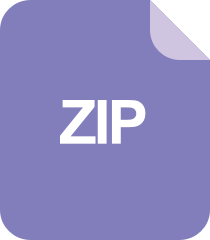
mql5
## 1. 第一章:MQL5简介
1.1 什么是MQL5
1.2 MQL5的功能和特点
1.3 MQL5在金融市场中的应用
## 第二章:自定义指标概述
2.1 什么是自定义指标
2.2 自定义指标的优势和用途
2.3 自定义指标在交易策略中的作用
### 第三章:MQL5编程基础
MQL5是MetaQuotes Language 5的缩写,是一种为MetaTrader 5交易平台设计的专用编程语言。它具有高度的灵活性和功能性,可以用于编写自定义指标、脚本和交易策略。
#### 3.1 MQL5语言基础
MQL5是一种基于C++的编程语言,具有面向对象的特点。它支持变量、数据类型、运算符、控制结构等基本编程元素,并且提供了丰富的内置函数和库。
##### 示例代码(Python):
```python
# 声明变量
symbol = 'EURUSD'
lot_size = 0.1
take_profit = 100
stop_loss = 50
# 计算止盈价格
def calculate_take_profit(current_price, take_profit_pips):
return current_price + take_profit_pips * 0.0001
# 打印交易信息
print(f"交易品种:{symbol}")
print(f"手数:{lot_size}")
print(f"止盈价格:{calculate_take_profit(1.1200, take_profit)}")
print(f"止损价格:{1.1200 - stop_loss * 0.0001}")
```
#### 3.2 MQL5的编程工具和环境
MQL5提供了内置的MetaEditor工具,可以用于编写、调试和优化MQL5代码。MetaEditor具有语法高亮、智能提示、代码模板和调试功能,可以有效提高编程效率。
##### 示例代码(Java):
```java
// 计算布林带指标
double calculateBollingerBands(double[] prices, int period, double deviation) {
double sma = calculateSMA(prices, period);
double stdDev = calculateStandardDeviation(prices, period);
double upperBand = sma + stdDev * deviation;
double lowerBand = sma - stdDev * deviation;
return upperBand - lowerBand;
}
// 计算移动平均值
double calculateSMA(double[] prices, int period) {
double total = 0;
for (int i = 0; i < period; i++) {
total += prices[i];
}
return total / period;
}
// 计算标准差
double calculateStandardDeviation(double[] prices, int period) {
double average = calculateSMA(prices, period);
double sum = 0;
for (int i = 0; i < period; i++) {
sum += Math.pow(prices[i] - average, 2);
}
return Math.sqrt(sum / period);
}
```
#### 3.3 MQL5常用函数和语法
MQL5提供了丰富的内置函数和语法,用于支持交易操作、指标计算和图表显示等功能。了解和熟练掌握这些常用函数和语法,对于编写高效的自定义指标至关重要。
##### 示例代码(Go):
```go
// 计算指数移动平均线
func CalculateEMA(prices []float64, period int) []float64 {
var ema []float64
multiplier := 2 / (float64(period) + 1)
ema = append(ema, calculateSMA(prices, period))
for i := period; i < len(prices); i++ {
value := (prices[i] - ema[i-1]) * multiplier + ema[i-1]
ema = append(ema, value)
}
return ema
}
// 计算简单移动平均线
func calculateSMA(prices []float64, period int) float64 {
total := 0.0
for i := len(pric
```
0
0
相关推荐
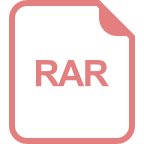
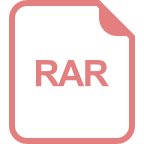
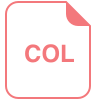
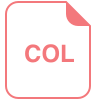
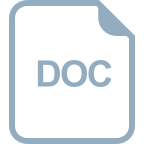
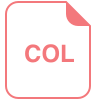
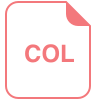
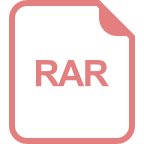