【单片机LED程序设计入门指南】:从零开始点亮LED
发布时间: 2024-07-09 14:24:11 阅读量: 45 订阅数: 36 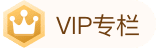
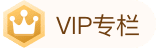
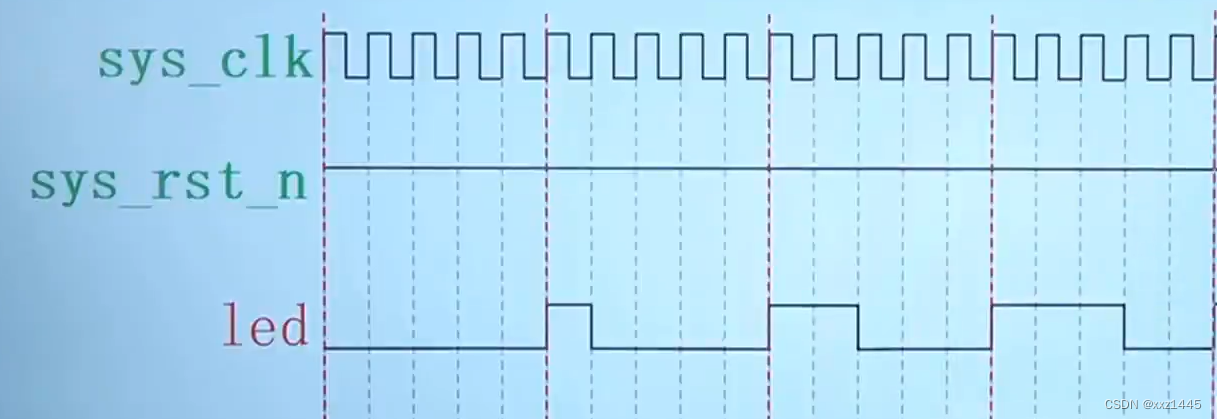
# 1. 单片机LED程序设计基础**
单片机LED程序设计是嵌入式系统开发中的一项基本技能,涉及单片机、LED驱动电路和程序设计等方面的知识。本章将介绍单片机LED程序设计的相关基础知识,为后续的实践和应用打下基础。
**1.1 单片机简介**
单片机是一种集成在单一芯片上的微型计算机,具有CPU、存储器、输入/输出接口等功能。单片机广泛应用于各种电子设备中,负责控制和处理数据。
**1.2 LED简介**
LED(发光二极管)是一种半导体器件,当电流通过时会发光。LED具有体积小、功耗低、响应快等优点,广泛应用于指示灯、显示屏和照明等领域。
# 2. LED驱动电路与原理
### 2.1 LED的特性与驱动方式
#### 2.1.1 LED的正向压降和电流
LED是一种发光二极管,其工作原理是当电流通过PN结时,电子与空穴复合,释放出能量以光的形式发出。LED的正向压降(Vf)是指在LED导通时,两端的电压降。Vf的大小与LED的材料和颜色有关,一般在1.8V~3.6V之间。
LED的正向电流(If)是指流过LED的电流。If的大小影响LED的亮度,一般在10mA~20mA之间。超过额定If会缩短LED的寿命,甚至烧毁LED。
#### 2.1.2 LED的驱动电路类型
根据LED的驱动方式,可以分为以下几种驱动电路类型:
- **限流电阻驱动电路**:最简单的一种驱动电路,通过一个电阻限制流过LED的电流。电阻值根据LED的Vf和If计算得出。
- **恒流驱动电路**:通过一个恒流源为LED提供稳定的电流,不受电源电压波动影响。
- **PWM驱动电路**:通过脉宽调制(PWM)技术,控制流过LED的平均电流,从而调节LED的亮度。
### 2.2 单片机GPIO与LED的连接
#### 2.2.1 GPIO的配置与输出
单片机GPIO(通用输入/输出)引脚可以配置为输入或输出模式。当配置为输出模式时,GPIO引脚可以输出高电平(Vcc)或低电平(GND)。
```c
// 设置GPIO引脚为输出模式
GPIO_InitTypeDef GPIO_InitStructure;
GPIO_InitStructure.Pin = GPIO_PIN_13;
GPIO_InitStructure.Mode = GPIO_MODE_OUT_PP;
GPIO_Init(GPIOC, &GPIO_InitStructure);
```
#### 2.2.2 LED的接线方式
LED的接线方式有两种:
- **共阳极连接**:所有LED的阳极连接到单片机GPIO引脚,阴极连接到GND。
- **共阴极连接**:所有LED的阴极连接到单片机GPIO引脚,阳极连接到Vcc。
```c
// 共阳极连接
GPIO_SetBits(GPIOC, GPIO_PIN_13); // 点亮LED
// 共阴极连接
GPIO_ResetBits(GPIOC, GPIO_PIN_13); // 点亮LED
```
# 3.1 LED闪烁程序设计
**3.1.1 延时函数的实现**
延时函数是单片机程序设计中常用的函数,用于在程序中实现时间延迟。在LED闪烁程序中,延时函数的作用是控制LED的亮灭时间。
常用的延时函数有两种:软件延时和硬件延时。软件延时通过软件循环的方式实现,硬件延时则利用单片机的定时器外设实现。
**软件延时**
```c
void delay_ms(unsigned int ms)
{
unsigned int i, j;
for (i = 0; i < ms; i++)
{
for (j = 0; j < 125; j++)
{
// 延时1ms
}
}
}
```
**硬件延时**
```c
void delay_ms(unsigned int ms)
{
TIM_SetCounter(TIMx, 0);
TIM_SetAutoreload(TIMx, ms * 1000);
TIM_Cmd(TIMx, ENABLE);
while (TIM_GetCounter(TIMx) < ms * 1000)
{
// 延时1ms
}
TIM_Cmd(TIMx, DISABLE);
}
```
**3.1.2 LED闪烁程序的编写**
```c
void main()
{
// 初始化GPIO
GPIO_Init(GPIOA, GPIO_Pin_0, GPIO_Mode_Out_PP);
while (1)
{
// LED点亮
GPIO_SetBits(GPIOA, GPIO_Pin_0);
delay_ms(500);
// LED熄灭
GPIO_ResetBits(GPIOA, GPIO_Pin_0);
delay_ms(500);
}
}
```
**程序逻辑分析:**
该程序首先初始化GPIO,将PA0配置为输出模式。然后进入一个死循环,在循环中,程序先将PA0置位,点亮LED,然后调用延时函数,延时500ms。接着,程序将PA0复位,熄灭LED,并再次调用延时函数,延时500ms。如此循环,实现LED闪烁的效果。
# 4.1 LED显示数字与字符
### 4.1.1 数码管的驱动原理
数码管是一种常见的显示器件,广泛应用于电子设备中。它由七个发光二极管组成,分别对应数字0到9。通过控制这七个LED的亮灭,可以显示不同的数字。
数码管的驱动原理比较简单。每个LED都有一个正极和一个负极,正极连接到电源正极,负极连接到单片机的GPIO引脚。当GPIO引脚输出高电平时,对应的LED就会亮起;当GPIO引脚输出低电平时,对应的LED就会熄灭。
### 4.1.2 LED显示数字与字符的程序设计
利用数码管显示数字和字符需要一定的编程技巧。首先,需要编写一个函数来控制数码管的显示。这个函数可以根据要显示的数字或字符,计算出需要点亮的LED组合,然后设置相应的GPIO引脚电平。
```c
void display_digit(int digit) {
switch (digit) {
case 0:
// 点亮LED a、b、c、d、e、f
GPIOA->ODR |= (1 << 0) | (1 << 1) | (1 << 2) | (1 << 3) | (1 << 4) | (1 << 5);
break;
case 1:
// 点亮LED b、c
GPIOA->ODR |= (1 << 1) | (1 << 2);
break;
// ...其他数字的点亮方式
}
}
```
然后,需要编写一个程序来调用这个函数,根据要显示的内容,逐个点亮数码管上的LED。
```c
int main() {
// 初始化GPIO
RCC->AHB1ENR |= RCC_AHB1ENR_GPIOAEN;
GPIOA->MODER &= ~(0x3 << 0);
GPIOA->MODER |= (0x1 << 0);
// 循环显示数字0-9
while (1) {
for (int i = 0; i < 10; i++) {
display_digit(i);
delay_ms(500);
}
}
}
```
通过这种方式,就可以利用单片机控制数码管显示数字和字符了。
# 5.1 交通灯控制系统
### 5.1.1 交通灯的原理与状态
交通灯是一种交通信号灯,用于控制车辆和行人的通行。它通常由红、黄、绿三种颜色组成,分别代表禁止通行、注意通行和允许通行。
交通灯的状态由一个控制器控制,控制器根据预先设定的时间周期或感应器检测到的交通流量来切换灯的状态。常见的交通灯状态包括:
- **红灯:**禁止车辆和行人通行。
- **黄灯:**警告车辆和行人即将发生状态变化,应做好准备。
- **绿灯:**允许车辆和行人通行。
### 5.1.2 交通灯控制程序的编写
使用单片机控制交通灯需要编写一个控制程序,该程序根据预先设定的时间周期或感应器检测到的交通流量来切换灯的状态。
下面是一个使用单片机控制交通灯的程序示例:
```c
#include <reg51.h>
// 定义LED端口
#define RED_LED P1_0
#define YELLOW_LED P1_1
#define GREEN_LED P1_2
// 定义状态
#define RED 0
#define YELLOW 1
#define GREEN 2
// 定义时间周期
#define RED_TIME 5
#define YELLOW_TIME 1
#define GREEN_TIME 5
// 当前状态
int state = RED;
// 定时器中断服务程序
void timer0_isr() interrupt 1 {
// 清除中断标志位
TH0 = 0;
TL0 = 0;
// 根据当前状态切换灯的状态
switch (state) {
case RED:
RED_LED = 1;
YELLOW_LED = 0;
GREEN_LED = 0;
state = YELLOW;
break;
case YELLOW:
RED_LED = 0;
YELLOW_LED = 1;
GREEN_LED = 0;
state = GREEN;
break;
case GREEN:
RED_LED = 0;
YELLOW_LED = 0;
GREEN_LED = 1;
state = RED;
break;
}
}
void main() {
// 初始化定时器0
TMOD = 0x01;
TH0 = 0xFF;
TL0 = 0xFF;
EA = 1;
ET0 = 1;
// 初始化LED端口
P1 = 0x00;
// 进入死循环
while (1) {
// 根据时间周期切换灯的状态
if (state == RED) {
TH0 = RED_TIME;
TL0 = 0;
} else if (state == YELLOW) {
TH0 = YELLOW_TIME;
TL0 = 0;
} else if (state == GREEN) {
TH0 = GREEN_TIME;
TL0 = 0;
}
}
}
```
0
0
相关推荐
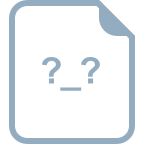
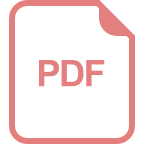
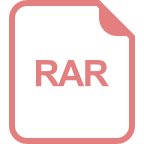





