面向对象编程基础:类和对象
发布时间: 2023-12-29 10:44:33 阅读量: 42 订阅数: 44 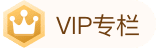
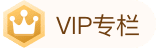
# 第一章:面向对象编程概述
## 1.1 什么是面向对象编程
面向对象编程(Object-Oriented Programming,简称OOP)是一种程序设计范式,它使用对象和类的概念来组织代码。在面向对象编程中,一切皆为对象,对象间通过消息传递进行交互。
## 1.2 面向对象编程的优点
面向对象编程具有模块化、可复用、可拓展、灵活性强等优点。通过封装、继承和多态等特性,可以更好地管理代码复杂度和提高代码的可维护性。
## 1.3 对象和类的概念
对象是面向对象编程的基本单元,它包含数据和方法。而类则是对象的抽象,是用来创建对象的蓝图或模板。
## 1.4 面向对象编程的基本原则
面向对象编程遵循四个基本原则:封装(Encapsulation)、继承(Inheritance)、多态(Polymorphism)和抽象(Abstraction)。这些原则帮助程序员设计出结构良好、易于理解和维护的代码。
接下来,我们将深入探讨面向对象编程的各个方面,包括类的定义、对象的创建和初始化、继承与多态、类的设计与实践以及面向对象编程的应用与发展。
## 2. 第二章:类的定义和属性
在面向对象编程中,类是一种抽象数据类型,用来描述具有相同属性和方法的对象集合。在本章中,我们将深入探讨类的定义和属性,包括类的基本概念、属性和方法的定义、构造方法和析构方法的使用,以及类的封装和访问控制。
### 2.1 类的基本概念
在面向对象编程中,类是对一类对象的抽象描述,它包含了对象的属性和方法。通过类,我们可以创建具体的对象实例,并对其进行操作和管理。一个类通常由类名、属性和方法组成。
```python
# Python示例
class Person:
def __init__(self, name, age):
self.name = name
self.age = age
def greet(self):
print(f"Hello, my name is {self.name} and I am {self.age} years old.")
# 创建对象实例
person1 = Person("Alice", 25)
person1.greet()
```
```java
// Java示例
public class Person {
private String name;
private int age;
// 构造方法
public Person(String name, int age) {
this.name = name;
this.age = age;
}
// 方法
public void greet() {
System.out.println("Hello, my name is " + name + " and I am " + age + " years old.");
}
// 创建对象实例
public static void main(String[] args) {
Person person1 = new Person("Alice", 25);
person1.greet();
}
}
```
### 2.2 类的属性和方法
类的属性是描述对象特征的变量,而类的方法则是描述对象行为的函数。通过属性和方法,我们可以对对象进行状态管理和行为操作。
```python
# Python示例
class Car:
def __init__(self, make, model, year):
self.make = make
self.model = model
self.year = year
def display_info(self):
print(f"The car is a {self.year} {self.make} {self.model}.")
car1 = Car("Tesla", "Model S", 2020)
car1.display_info()
```
```java
// Java示例
public class Car {
private String make;
private String model;
private int year;
// 构造方法
public Car(String make, String model, int year) {
this.make = make;
this.model = model;
this.year = year;
}
// 方法
public void displayInfo() {
System.out.println("The car is a " + year + " " + make + " " + model + ".");
}
// 创建对象实例
public static void main(String[] args) {
Car car1 = new Car("Tesla", "Model S", 2020);
car1.displayInfo();
}
}
```
### 2.3 类的构造方法和析构方法
构造方法用于初始化对象的属性,而析构方法则用于释放对象所占用的资源。在不同的编程语言中,构造方法和析构方法的实现方式可能会有所不同。
```python
# Python示例
class Book:
def __init__(self, title, author):
self.title = title
self.author = author
print("A book is created.")
def __del__(self):
print("A book is destroyed.")
book1 = Boo
```
0
0
相关推荐
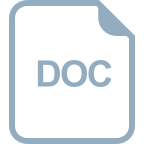
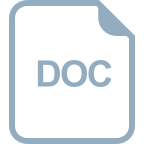
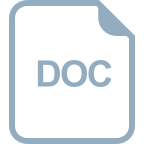
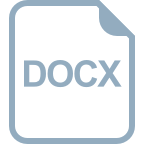
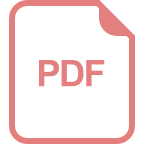
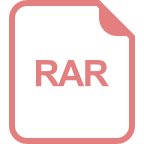
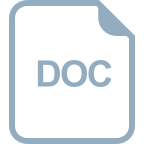
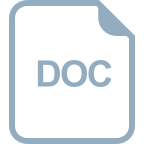