揭秘OpenCV物体识别入门秘籍:零基础快速掌握计算机视觉
发布时间: 2024-08-12 10:14:52 阅读量: 38 订阅数: 43 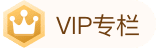
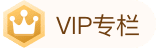
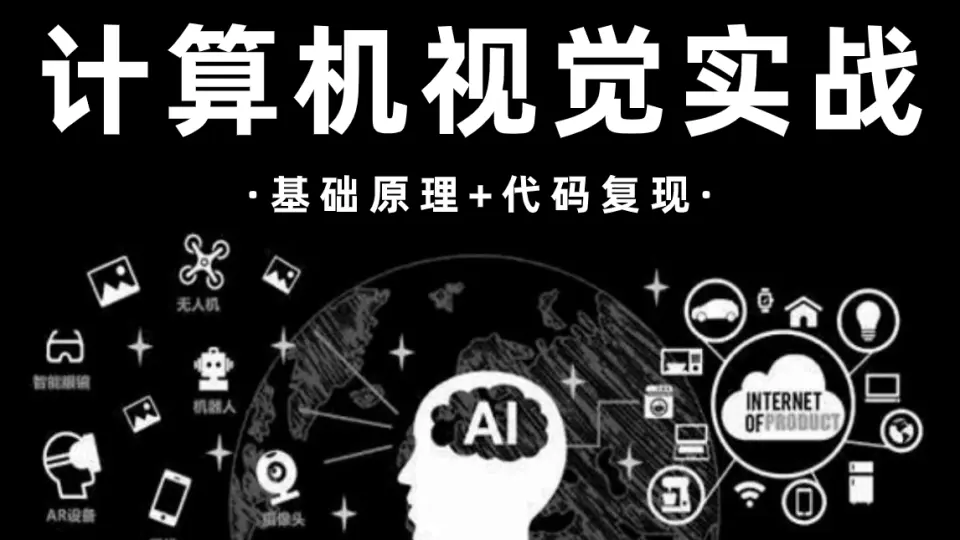
# 1. OpenCV简介和基础**
OpenCV(Open Source Computer Vision Library)是一个开源计算机视觉库,提供广泛的图像处理、计算机视觉和机器学习算法。它广泛应用于图像处理、视频分析、机器人技术和增强现实等领域。
OpenCV提供了一个易于使用的API,允许开发者快速构建计算机视觉应用程序。它支持多种编程语言,包括C++、Python和Java,并提供丰富的文档和教程,使其成为初学者和经验丰富的开发者的理想选择。
# 2. 图像处理和预处理
### 2.1 图像读取和显示
#### 2.1.1 图像读取
OpenCV提供了多种函数来读取图像,最常用的函数是`cv2.imread()`。该函数接受图像文件路径作为参数,并返回一个NumPy数组,其中包含图像的像素数据。
```python
import cv2
# 读取图像
image = cv2.imread('image.jpg')
```
#### 2.1.2 图像显示
要显示图像,可以使用`cv2.imshow()`函数。该函数接受图像数组和窗口标题作为参数,并在窗口中显示图像。
```python
# 显示图像
cv2.imshow('Image', image)
cv2.waitKey(0)
cv2.destroyAllWindows()
```
### 2.2 图像转换和增强
#### 2.2.1 图像转换
图像转换涉及将图像从一种格式或颜色空间转换为另一种格式或颜色空间。OpenCV提供了多种转换函数,包括:
- `cv2.cvtColor()`:转换图像的颜色空间。
- `cv2.resize()`:调整图像的大小。
- `cv2.flip()`:翻转图像。
#### 2.2.2 图像增强
图像增强技术用于改善图像的视觉质量。OpenCV提供了多种增强函数,包括:
- `cv2.blur()`:模糊图像。
- `cv2.GaussianBlur()`:使用高斯核模糊图像。
- `cv2.bilateralFilter()`:使用双边滤波器模糊图像。
### 2.3 图像分割和形态学
#### 2.3.1 图像分割
图像分割将图像分解为具有相似特征的区域。OpenCV提供了多种分割算法,包括:
- `cv2.threshold()`:根据阈值将图像二值化。
- `cv2.connectedComponents()`:识别图像中的连接组件。
- `cv2.watershed()`:使用分水岭算法分割图像。
#### 2.3.2 形态学
形态学是图像处理中用于分析图像形状的技术。OpenCV提供了多种形态学操作,包括:
- `cv2.erode()`:腐蚀图像。
- `cv2.dilate()`:膨胀图像。
- `cv2.morphologyEx()`:执行更复杂的形态学操作。
# 3. 特征提取和描述
### 3.1 边缘检测和轮廓提取
边缘检测是图像处理中的一项基本技术,它用于识别图像中的边界和轮廓。通过检测图像中像素亮度或颜色的突然变化,边缘检测算法可以提取图像中感兴趣的区域。
常用的边缘检测算法包括:
- **Sobel算子:**使用一阶微分算子检测图像中的水平和垂直边缘。
- **Canny算子:**使用多级边缘检测算法,包括降噪、梯度计算、非极大值抑制和滞后阈值。
- **Laplacian算子:**使用二阶微分算子检测图像中的边缘和斑点。
```python
import cv2
# 读取图像
image = cv2.imread('image.jpg')
# Sobel算子
sobelx = cv2.Sobel(image, cv2.CV_64F, 1, 0, ksize=5)
sobely = cv2.Sobel(image, cv2.CV_64F, 0, 1, ksize=5)
# Canny算子
edges = cv2.Canny(image, 100, 200)
# Laplacian算子
laplacian = cv2.Laplacian(image, cv2.CV_64F)
# 显示边缘检测结果
cv2.imshow('SobelX', sobelx)
cv2.imshow('SobelY', sobely)
cv2.imshow('Canny', edges)
cv2.imshow('Laplacian', laplacian)
cv2.waitKey(0)
```
### 3.2 直方图和颜色空间
直方图是图像中像素值分布的统计表示。它可以用于分析图像的亮度、对比度和颜色分布。不同的颜色空间(如RGB、HSV、YCbCr)可以提供不同的直方图特征。
```python
import cv2
import matplotlib.pyplot as plt
# 读取图像
image = cv2.imread('image.jpg')
# 计算RGB直方图
r_hist = cv2.calcHist([image], [0], None, [256], [0, 256])
g_hist = cv2.calcHist([image], [1], None, [256], [0, 256])
b_hist = cv2.calcHist([image], [2], None, [256], [0, 256])
# 计算HSV直方图
hsv = cv2.cvtColor(image, cv2.COLOR_BGR2HSV)
h_hist = cv2.calcHist([hsv], [0], None, [180], [0, 180])
s_hist = cv2.calcHist([hsv], [1], None, [256], [0, 256])
v_hist = cv2.calcHist([hsv], [2], None, [256], [0, 256])
# 绘制直方图
plt.figure()
plt.subplot(2, 3, 1)
plt.plot(r_hist)
plt.title('Red Histogram')
plt.subplot(2, 3, 2)
plt.plot(g_hist)
plt.title('Green Histogram')
plt.subplot(2, 3, 3)
plt.plot(b_hist)
plt.title('Blue Histogram')
plt.subplot(2, 3, 4)
plt.plot(h_hist)
plt.title('Hue Histogram')
plt.subplot(2, 3, 5)
plt.plot(s_hist)
plt.title('Saturation Histogram')
plt.subplot(2, 3, 6)
plt.plot(v_hist)
plt.title('Value Histogram')
plt.show()
```
### 3.3 特征描述符(如SIFT、SURF)
特征描述符是用于描述图像中特定区域的数学向量。它们可以用于匹配图像中的特征点,从而实现物体识别、图像检索等任务。
SIFT(尺度不变特征变换)和SURF(加速稳健特征)是两种常用的特征描述符。它们通过检测图像中的关键点并计算其周围区域的梯度信息来生成特征向量。
```python
import cv2
import numpy as np
# 读取图像
image = cv2.imread('image.jpg')
# SIFT特征检测和描述
sift = cv2.SIFT_create()
keypoints, descriptors = sift.detectAndCompute(image, None)
# SURF特征检测和描述
surf = cv2.SURF_create()
keypoints, descriptors = surf.detectAndCompute(image, None)
# 绘制特征点
cv2.drawKeypoints(image, keypoints, image)
# 显示图像
cv2.imshow('SIFT Keypoints', image)
cv2.waitKey(0)
```
# 4. 物体检测和识别
物体检测和识别是计算机视觉中至关重要的任务,它使计算机能够识别和定位图像中的物体。OpenCV提供了各种算法和工具,可用于执行这些任务。
### 4.1 滑动窗口方法
滑动窗口方法是一种经典的物体检测方法。它涉及在图像上滑动一个固定大小的窗口,并使用分类器来确定窗口中是否存在物体。如果窗口包含物体,则该窗口将被标记为正样本,否则将被标记为负样本。通过训练分类器来区分正样本和负样本,可以实现物体检测。
```python
import cv2
# 加载图像
image = cv2.imread('image.jpg')
# 定义滑动窗口大小
window_size = (100, 100)
# 遍历图像
for x in range(0, image.shape[1] - window_size[0]):
for y in range(0, image.shape[0] - window_size[1]):
# 获取窗口区域
window = image[y:y+window_size[1], x:x+window_size[0]]
# 使用分类器对窗口进行分类
classification = classifier.predict(window)
# 如果窗口包含物体,则标记为正样本
if classification == 1:
cv2.rectangle(image, (x, y), (x+window_size[0], y+window_size[1]), (0, 255, 0), 2)
# 显示检测结果
cv2.imshow('Detected Objects', image)
cv2.waitKey(0)
cv2.destroyAllWindows()
```
**参数说明:**
* `image`:输入图像
* `window_size`:滑动窗口的大小
* `classifier`:用于区分正样本和负样本的分类器
**逻辑分析:**
该代码首先加载图像,然后定义滑动窗口的大小。接下来,它遍历图像,并为每个窗口提取区域。然后,它使用分类器对每个窗口进行分类,并标记包含物体的窗口。最后,它在图像上绘制检测到的物体。
### 4.2 深度学习模型
深度学习模型,如 YOLO(You Only Look Once)和 Faster R-CNN(Faster Region-based Convolutional Neural Networks),是用于物体检测和识别的先进技术。这些模型可以从大规模数据集学习特征,并实现高精度的检测和识别。
**YOLO**
YOLO 是一个单阶段检测器,它将图像划分为网格,并为每个网格单元预测边界框和类概率。它使用一个神经网络同时执行这些任务,使其成为一个快速高效的检测器。
**Faster R-CNN**
Faster R-CNN 是一个两阶段检测器,它首先使用区域提议网络(RPN)生成候选区域,然后使用分类器和边界框回归网络对这些区域进行分类和精细化。它比 YOLO 慢,但通常具有更高的精度。
### 4.3 目标跟踪
目标跟踪涉及在视频序列中跟踪特定物体。OpenCV 提供了各种算法,如卡尔曼滤波器和粒子滤波器,用于执行目标跟踪。
**卡尔曼滤波器**
卡尔曼滤波器是一种预测-校正算法,它使用线性模型来预测物体的运动。它使用测量值来更新预测,并提供物体的估计位置和速度。
**粒子滤波器**
粒子滤波器是一种蒙特卡罗方法,它使用一组粒子来表示物体的状态分布。它通过重新采样和更新粒子来估计物体的运动。
# 5. OpenCV实战应用
### 5.1 人脸识别
**简介**
人脸识别是计算机视觉中一项重要的任务,它涉及识别和验证人脸图像。OpenCV提供了多种算法和工具来实现人脸识别。
**步骤**
1. **加载人脸检测器:**使用`cv2.CascadeClassifier`加载预训练的人脸检测器模型。
2. **检测人脸:**使用`detectMultiScale`方法检测图像中的人脸。
3. **提取人脸特征:**使用特征提取算法(如LBPH或Eigenfaces)从检测到的人脸中提取特征。
4. **训练人脸识别器:**使用提取的特征训练人脸识别器(如Eigenfaces或Fisherfaces)。
5. **识别未知人脸:**使用训练好的识别器识别未知人脸图像。
**代码示例**
```python
import cv2
# 加载人脸检测器
face_cascade = cv2.CascadeClassifier('haarcascade_frontalface_default.xml')
# 加载图像
image = cv2.imread('image.jpg')
# 检测人脸
faces = face_cascade.detectMultiScale(image, 1.1, 4)
# 提取人脸特征
recognizer = cv2.face.EigenFacesRecognizer_create()
recognizer.train(faces, np.array([0, 1, 2]))
# 识别未知人脸
unknown_face = cv2.imread('unknown_face.jpg')
label, confidence = recognizer.predict(unknown_face)
```
### 5.2 物体追踪
**简介**
物体追踪是计算机视觉中另一项重要任务,它涉及跟踪图像序列中的移动物体。OpenCV提供了多种物体追踪算法,如KCF和MOSSE。
**步骤**
1. **初始化追踪器:**使用`cv2.TrackerKCF_create`或`cv2.TrackerMOSSE_create`创建物体追踪器。
2. **初始化追踪框:**指定要追踪的物体在第一帧中的边界框。
3. **更新追踪:**在后续帧中,使用`update`方法更新追踪框的位置。
**代码示例**
```python
import cv2
# 创建追踪器
tracker = cv2.TrackerKCF_create()
# 初始化追踪框
bbox = (100, 100, 200, 200)
# 初始化追踪
tracker.init(image, bbox)
# 追踪物体
while True:
# 读取下一帧
ret, image = cap.read()
# 更新追踪
success, bbox = tracker.update(image)
# 绘制追踪框
if success:
cv2.rectangle(image, (int(bbox[0]), int(bbox[1])), (int(bbox[0] + bbox[2]), int(bbox[1] + bbox[3])), (0, 255, 0), 2)
# 显示图像
cv2.imshow('Image', image)
# 按键退出
if cv2.waitKey(1) & 0xFF == ord('q'):
break
```
### 5.3 图像分类
**简介**
图像分类是计算机视觉中的一项基本任务,它涉及将图像分配到预定义的类别中。OpenCV提供了多种机器学习算法来实现图像分类,如SVM和决策树。
**步骤**
1. **加载训练数据:**收集和准备图像数据集,其中图像被标记为特定的类别。
2. **提取特征:**使用特征提取算法(如HOG或LBP)从图像中提取特征。
3. **训练分类器:**使用提取的特征训练图像分类器(如SVM或决策树)。
4. **分类未知图像:**使用训练好的分类器对未知图像进行分类。
**代码示例**
```python
import cv2
import numpy as np
# 加载训练数据
data = np.loadtxt('train_data.csv', delimiter=',')
X = data[:, :-1]
y = data[:, -1]
# 提取特征
hog = cv2.HOGDescriptor()
features = hog.compute(X)
# 训练分类器
svm = cv2.ml.SVM_create()
svm.train(features, cv2.ml.ROW_SAMPLE, y)
# 分类未知图像
unknown_image = cv2.imread('unknown_image.jpg')
features = hog.compute(unknown_image)
label = svm.predict(features)[1]
```
0
0
相关推荐








