shell脚本中的字符串处理技巧与细节注意事项
发布时间: 2024-02-27 13:07:15 阅读量: 37 订阅数: 29 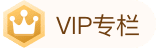
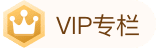
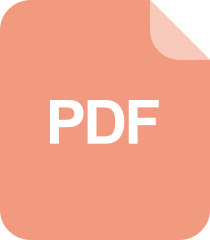
Linux shell脚本中字符串连接的方法
# 1. 字符串处理基础
在shell脚本编程中,字符串处理是非常常见的操作之一。本章节将介绍在shell脚本中进行字符串处理的基础知识,包括字符串的定义、长度、提取等操作。
## 字符串的定义与赋值
在shell脚本中,字符串可以使用单引号或双引号进行定义,并且可以通过变量赋值来进行操作。
```bash
# 使用单引号定义字符串
str1='Hello, world!'
echo $str1
# 使用双引号定义字符串
str2="Hello, shell!"
echo $str2
# 使用变量赋值
name="John"
str3="Hello, $name!"
echo $str3
```
通过以上示例,我们可以看到不同类型的字符串定义和赋值方式。
## 获取字符串长度
在shell脚本中,获取字符串的长度是一个常见的操作,可以通过内置的字符串长度计算命令 `expr length` 来实现。
```bash
str="Hello, world!"
length=$(expr length "$str")
echo "The length of the string is: $length"
```
通过这段代码,我们可以获取到字符串的长度并输出。
### 总结
本节我们介绍了shell脚本中字符串的定义、赋值和获取长度的基础知识。在下一节中,我们将进一步探讨字符串查找与替换技巧。
# 2. 字符串处理基础
在shell脚本中,对字符串进行处理是非常常见的操作。字符串处理基础包括字符串长度、提取子字符串、字符串连接等操作。以下是一些常见的字符串处理基础操作:
### 1. 获取字符串长度
使用`expr length`命令可以获取字符串的长度,示例代码如下:
```bash
#!/bin/bash
str="hello world"
len=$(expr length "$str")
echo "The length of the string is $len"
```
运行结果:
```bash
The length of the string is 11
```
### 2. 提取子字符串
使用`${str: start: length}`可以从字符串中提取子字符串,其中`start`表示起始位置,`length`表示子字符串长度,示例代码如下:
```bash
#!/bin/bash
str="hello world"
substr=${str: 6: 5}
echo "The substring is $substr"
```
运行结果:
```bash
The substring is world
```
### 3. 字符串连接
使用`+`或者`+=`可以进行字符串连接,示例代码如下:
```bash
#!/bin/bash
str1="hello"
str2="world"
result=$str1" "$str2
echo "The concatenated string is $result"
```
运行结果:
```bash
The concatenated string is hello world
```
通过以上基础操作,可以完成对字符串的常见处理需求。接下来,我们将介绍更多字符串处理技巧及注意事项。
# 3. 字符串查找与替换技巧
在
0
0
相关推荐
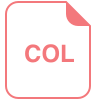
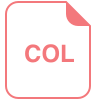
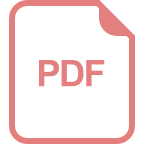
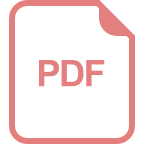
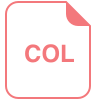
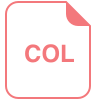