掌握Selenium中的异常处理与错误排查
发布时间: 2023-12-19 20:11:42 阅读量: 64 订阅数: 46 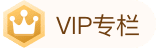
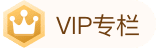
# 1. Selenium异常处理概述
### 1.1 什么是Selenium异常?
Selenium异常是指在使用Selenium自动化测试过程中,由于各种原因导致的程序无法正常执行,从而抛出的异常情况。这些异常可能来自于网络延迟、页面元素未找到、执行超时等各种情况。
### 1.2 为什么需要处理Selenium异常?
Selenium异常处理的主要目的是确保自动化测试的稳定性和健壮性。在实际操作中,页面的元素可能随时发生变化,网络环境也可能不稳定,如果不处理这些异常,就会导致测试用例失败,甚至整个测试流程中断。
### 1.3 常见的Selenium异常类型
常见的Selenium异常包括但不限于:
- NoSuchElementException:无法找到页面元素的异常
- ElementNotVisibleException:页面元素不可见异常
- TimeoutException:执行操作超时异常
- StaleElementReferenceException:页面元素已经过时异常
以上就是对Selenium异常处理的概述,接下来我们将深入探讨各种异常处理技术以及常见错误的排查方法。
# 2. Selenium异常处理技术
在使用Selenium进行自动化测试时,经常会遇到各种异常情况。为了保证测试的稳定性和可靠性,我们需要学会如何处理这些异常情况。本章将介绍几种常见的Selenium异常处理技术,帮助我们更好地排查和处理异常。
### 2.1 try-catch语句的运用
try-catch语句是一种常见的异常处理机制,可以用来捕获和处理异常。在Selenium中,我们可以将可能抛出异常的操作放在try块中,在catch块中处理异常情况。
```python
from selenium import webdriver
from selenium.common.exceptions import NoSuchElementException
# 创建WebDriver对象
driver = webdriver.Chrome()
try:
# 访问网页
driver.get("http://www.example.com")
# 进行可能抛出异常的操作
element = driver.find_element_by_id("some-element")
# 其他操作...
except NoSuchElementException:
# 处理NoSuchElementException异常
print("元素未找到")
finally:
# 关闭浏览器
driver.quit()
```
在上述示例中,我们使用了try-catch语句来处理NoSuchElementException异常。如果find_element_by_id方法找不到指定的元素,将抛出NoSuchElementException异常,并被catch块捕获。我们可以在catch块中编写处理该异常的逻辑,例如输出错误信息。
### 2.2 使用finally块的收尾工作
finally块是try-catch语句的可选部分,用于定义收尾工作,无论是否发生异常都会执行其中的代码。在Selenium中,finally块通常用来执行一些收尾操作,比如关闭浏览器。
```java
import org.openqa.selenium.By;
import org.openqa.selenium.NoSuchElementException;
import org.openqa.selenium.WebDriver;
import org.openqa.selenium.WebElement;
import org.openqa.selenium.chrome.ChromeDriver;
public class ExceptionHandlingExample {
public static void main(String[] args) {
// 设置ChromeDriver路径
System.setProperty("webdriver.chrome.driver", "path/to/chromedriver");
// 创建WebDriver对象
WebDriver driver = new ChromeDriver();
try {
// 访问网页
driver.get("http://www.example.com");
// 进行可能抛出异常的操作
WebElement element = driver.findElement(By.id("some-element"));
// 其他操作...
} catch (NoSuchElementException e) {
// 处理NoSuchElementException异常
System.out.println("元素未找到");
} finally {
// 关闭浏览器
driver.quit();
}
}
}
```
在上述Java代码示例中,我们使用了finally块来关闭WebDriver实例,无论是否发生异常都会执行driver.quit()方法,确保浏览器被正确关闭。
### 2.3 自定义异常处理函数
除了使用try-catch语句来处理异常外,我们也可以自定义异常处理函数来进行异常处理。
```javascript
const { Builder, By, Key, until } = require("selenium-webdriver");
// 自定义异常处理函数
function handleException(error) {
if (error.name === "NoSuchElementException") {
console.log("元素未找到");
} else if (error.name === "TimeoutError") {
console.log("操作超时");
} else {
console.log("发生未知异常");
}
}
async function example() {
// 创建WebDriver对象
let driver = await new Builder().forBrowser("chrome").build();
try {
// 访问网页
await driver.get("http://www.example.com");
// 进行可能抛出异常的操作
let element = await driver.findElement(By.id("some-element"));
```
0
0
相关推荐
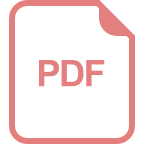
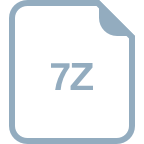
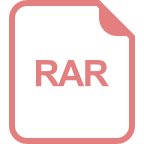





