JSON数据解析与处理:深入剖析JSON数据结构
发布时间: 2024-07-27 22:00:01 阅读量: 26 订阅数: 30 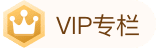
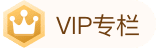
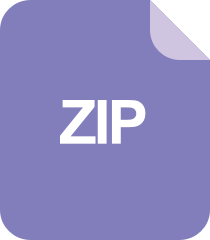
javabiginteger源码-universal-binary-json-java:通用二进制JSONJava库
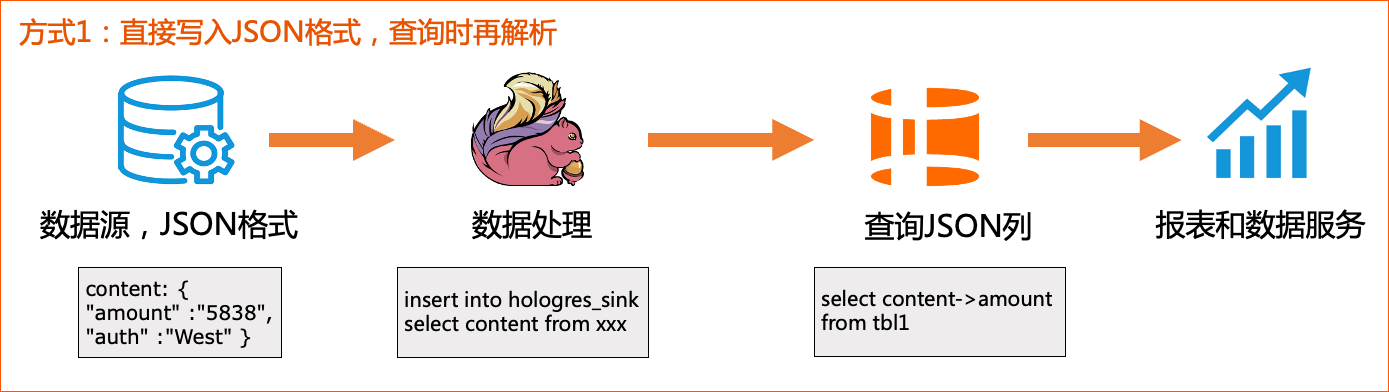
# 1. JSON数据结构概述**
JSON(JavaScript Object Notation)是一种轻量级的数据交换格式,广泛用于Web开发和数据科学领域。它采用键值对的形式组织数据,具有以下特点:
- **层次化结构:**JSON数据可以包含嵌套的对象和数组,形成层次化的数据结构。
- **键值对:**每个键值对由一个字符串键和一个值组成,值可以是字符串、数字、布尔值、对象或数组。
- **无模式:**JSON数据没有预定义的模式,可以灵活地存储各种类型的数据。
# 2. JSON数据解析技术
### 2.1 解析JSON数据的常用工具和库
#### 2.1.1 Python中的JSON模块
Python中提供了内置的`json`模块用于解析JSON数据。该模块包含了以下主要函数:
- `json.load()`: 从文件或类似文件对象中加载JSON数据,并将其解析为Python对象。
- `json.loads()`: 从字符串中加载JSON数据,并将其解析为Python对象。
- `json.dump()`: 将Python对象序列化为JSON数据,并将其写入文件或类似文件对象。
- `json.dumps()`: 将Python对象序列化为JSON数据,并返回字符串。
**代码块:**
```python
import json
# 从文件加载JSON数据
with open('data.json', 'r') as f:
data = json.load(f)
# 从字符串加载JSON数据
json_str = '{"name": "John Doe", "age": 30}'
data = json.loads(json_str)
# 将Python对象序列化为JSON数据
data = {"name": "Jane Doe", "age": 25}
json_str = json.dumps(data)
```
**逻辑分析:**
* `json.load()`和`json.loads()`函数将JSON数据解析为Python字典或列表。
* `json.dump()`和`json.dumps()`函数将Python对象序列化为JSON字符串。
* `with`语句用于管理文件打开和关闭,确保资源正确释放。
#### 2.1.2 JavaScript中的JSON.parse()方法
JavaScript中提供了`JSON.parse()`方法用于解析JSON数据。该方法将JSON字符串解析为JavaScript对象。
**代码块:**
```javascript
// 从字符串解析JSON数据
const json_str = '{"name": "John Doe", "age": 30}';
const data = JSON.parse(json_str);
// 将JavaScript对象序列化为JSON字符串
const data = {name: "Jane Doe", age: 25};
const json_str = JSON.stringify(data);
```
**逻辑分析:**
* `JSON.parse()`方法将JSON字符串解析为JavaScript对象。
* `JSON.stringify()`方法将JavaScript对象序列化为JSON字符串。
### 2.2 JSON数据的解析流程和注意事项
#### 2.2.1 数据类型转换
在解析JSON数据时,需要考虑数据类型转换。JSON数据中的数据类型与Python或JavaScript中的数据类型可能不完全一致。例如:
* JSON中的数字类型在Python中解析为`int`或`float`类型。
* JSON中的布尔类型在Python中解析为`bool`类型。
* JSON中的null值在Python中解析为`None`。
**代码块:**
```python
# JSON数据
json_data = '{"name": "John Doe", "age": 30, "is_active": true}'
# 解析JSON数据
data = json.loads(json_data)
# 检查数据类型
print(type(data['name'])) # <class 'str'>
print(type(data['age'])) # <class 'int'>
print(type(data['is_active'])) # <class 'bool'>
```
**逻辑分析:**
* JSON中的字符串类型解析为Python中的`str`类型。
* JSON中的数字类型解析为Python中的`int`类型。
* JSON中的布尔类型解析为Python中的`bool`类型。
#### 2.2.2 数据结构处理
JSON数据可以包含嵌套的对象和数组。在解析JSON数据时,需要考虑数据结构的处理。例如:
* JSON中的对象在Python中解析为字典。
* JSON中的数组在Python中解析为列表。
**代码块:**
```python
# JSON数据
json_data = '{"name": "John Doe", "address": {"street": "Main Street", "city": "New York"}}'
# 解析JSON数据
data = json.loads(json_data)
# 检查数据结构
print(type(data)) # <class 'dict'>
print(type(data['address'])) # <class 'dict'>
```
**逻辑分析:**
* JSON中的对象解析为Python中的字典。
* JSON中的嵌套对象也解析为Python中的字典。
# 3. JSON数据处理实践
### 3.1 JSON数据的修改和更新
JSON数据是一种可变的数据结构,可以进行修改和更新。
#### 3.1.1 修改JSON对象中的值
要修改JSON对象中的值,可以使用赋值操作符
0
0
相关推荐
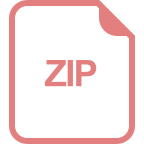
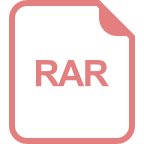
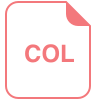
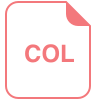
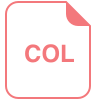
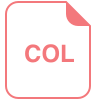
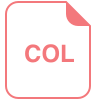
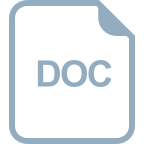