【JSON Server数据库入门秘籍】:快速搭建RESTful API,解锁数据交互新姿势
发布时间: 2024-07-27 18:11:31 阅读量: 32 订阅数: 31 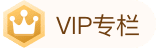
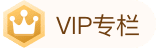

# 1. JSON Server简介
JSON Server是一个轻量级的Node.js框架,用于创建RESTful API。它提供了一个简单的接口,可以轻松地定义数据模型和路由,从而快速构建API。JSON Server通过提供开箱即用的CRUD(创建、读取、更新、删除)操作,简化了API开发过程,无需编写复杂的代码。
JSON Server基于JSON数据格式,这意味着它可以轻松地与其他应用程序和服务集成。它还支持中间件,允许开发人员自定义和扩展API的功能。
# 2. JSON Server RESTful API的构建
### 2.1 创建JSON Server项目
**步骤:**
1. 安装JSON Server包:`npm install -g json-server`
2. 创建项目目录:`mkdir json-server-api`
3. 进入项目目录:`cd json-server-api`
4. 初始化项目:`json-server --init`
**说明:**
`--init`命令将创建以下文件:
* `db.json`:用于存储数据
* `server.js`:包含API路由和逻辑
* `.gitignore`:忽略文件
### 2.2 定义数据模型和路由
**数据模型:**
在`db.json`文件中定义数据模型,例如:
```json
{
"posts": [
{
"id": 1,
"title": "My First Post",
"content": "This is my first post on JSON Server."
}
]
}
```
**路由:**
在`server.js`文件中定义API路由,例如:
```javascript
const jsonServer = require('json-server');
const server = jsonServer.create();
const router = jsonServer.router('db.json');
server.use(jsonServer.bodyParser());
server.use(router);
server.listen(3000, () => {
console.log('JSON Server is running on port 3000');
});
```
**说明:**
* `bodyParser()`解析请求体中的JSON数据。
* `router('db.json')`创建路由,将请求映射到`db.json`文件中的数据。
* `server.listen(3000)`启动服务器,监听3000端口。
### 2.3 CRUD操作的实现
**创建(Create):**
```javascript
// POST /posts
router.post('/posts', (req, res) => {
const newPost = req.body;
newPost.id = posts.length + 1;
posts.push(newPost);
res.json(newPost);
});
```
**读取(Read):**
```javascript
// GET /posts
router.get('/posts', (req, res) => {
res.json(posts);
});
// GET /posts/:id
router.get('/posts/:id', (req, res) => {
const post = posts.find(p => p.id === parseInt(req.params.id));
if (!post) return res.sendStatus(404);
res.json(post);
});
```
**更新(Update):**
```javascript
// PUT /posts/:id
router.put('/posts/:id', (req, res) => {
const updatedPost = req.body;
const post = posts.find(p => p.id === parseInt(req.params.id));
if (!post) return res.sendStatus(404);
Object.assign(post, updatedPost);
res.json(post);
});
```
**删除(Delete):**
```javascript
// DELETE /posts/:id
router.delete('/posts/:id', (req, res) => {
const index = posts.findIndex(p => p.id === parseInt(req.params.id));
if (index === -1) return res.sendStatus(404);
posts.splice(index, 1);
res.sendStatus(204);
});
```
**参数说明:**
* `req`:请求对象
* `res`:响应对象
* `req.body`:请求体中的JSON数据
* `req.params.id`:请求参数中的ID
* `posts`:从`db.json`中加载的数据
# 3.1 数据验证和过滤
#### 数据验证
JSON Server 提供了对请求数据的验证功能,确保数据符合预期的格式和约束。验证规则通过 JSON Schema 定义,它是一个描述 JSON 数据结构和约束的规范。
**创建 JSON Schema**
```json
{
"$schema": "http://json-schema.org/draft-07/schema#",
"title": "Product Schema",
"type": "object",
"properties": {
"id": {
"type": "integer",
"minimum": 1
},
"name": {
"type": "string",
"minLength": 1
},
"price": {
"type": "number",
"minimum": 0
}
},
"required": ["id", "name", "price"]
}
```
**应用 JSON Schema**
在 JSON Server 配置文件中,将 JSON Schema 关联到数据模型:
```json
{
"resources": [
{
"name": "products",
"schema": "./product-schema.json"
}
]
}
```
#### 数据过滤
JSON Server 允许对数据进行过滤,以返回符合特定条件的子集。过滤条件通过查询字符串参数指定。
**基本过滤**
```
GET /products?name=iPhone
```
**高级过滤**
```
GET /products?price_gt=100&price_lt=200
```
**逻辑运算符**
```
GET /products?name_like=iPhone%25&price_gt=100
```
### 3.2 数据关系管理
JSON Server 支持一对多和多对多关系,允许在不同数据模型之间建立关联。
**一对多关系**
```json
{
"resources": [
{
"name": "orders",
"schema": "./order-schema.json"
},
{
"name": "order_items",
"schema": "./order-item-schema.json",
"relations": {
"order_id": {
"resource": "orders",
"type": "belongsTo"
}
}
}
]
}
```
**多对多关系**
```json
{
"resources": [
{
"name": "users",
"schema": "./user-schema.json"
},
{
"name": "roles",
"schema": "./role-schema.json"
},
{
"name": "user_roles",
"schema": "./user-role-schema.json",
"relations": {
"user_id": {
"resource": "users",
"type": "belongsTo"
},
"role_id": {
"resource": "roles",
"type": "belongsTo"
}
}
}
]
}
```
### 3.3 身份验证和授权
JSON Server 提供了身份验证和授权机制,用于保护 API 端点免遭未经授权的访问。
**身份验证**
JSON Server 支持以下身份验证方法:
* 基本身份验证
* JWT 身份验证
**授权**
授权规则通过 JSON Server 的权限文件定义。权限文件指定哪些用户或角色可以访问哪些 API 端点和操作。
```json
{
"rules": [
{
"resource": "products",
"action": "read",
"allow": "all"
},
{
"resource": "products",
"action": "write",
"allow": ["admin"]
}
]
}
```
# 4. JSON Server的实践应用
### 4.1 搭建博客API
**创建博客数据模型**
```json
{
"title": "string",
"content": "string",
"author": "string",
"date": "string"
}
```
**定义路由**
```json
{
"/blogs": {
"GET": "findAll",
"POST": "create"
},
"/blogs/:id": {
"GET": "findById",
"PUT": "update",
"DELETE": "deleteById"
}
}
```
**实现CRUD操作**
```javascript
// GET all blogs
app.get("/blogs", (req, res) => {
res.json(db.blogs);
});
// POST a new blog
app.post("/blogs", (req, res) => {
const newBlog = req.body;
db.blogs.push(newBlog);
res.json(newBlog);
});
// GET a blog by id
app.get("/blogs/:id", (req, res) => {
const blog = db.blogs.find(blog => blog.id === parseInt(req.params.id));
res.json(blog);
});
// PUT a blog by id
app.put("/blogs/:id", (req, res) => {
const updatedBlog = req.body;
const blog = db.blogs.find(blog => blog.id === parseInt(req.params.id));
blog.title = updatedBlog.title;
blog.content = updatedBlog.content;
blog.author = updatedBlog.author;
blog.date = updatedBlog.date;
res.json(blog);
});
// DELETE a blog by id
app.delete("/blogs/:id", (req, res) => {
db.blogs = db.blogs.filter(blog => blog.id !== parseInt(req.params.id));
res.json({ message: "Blog deleted successfully" });
});
```
### 4.2 开发电商系统API
**创建产品数据模型**
```json
{
"id": "string",
"name": "string",
"description": "string",
"price": "number",
"quantity": "number"
}
```
**定义路由**
```json
{
"/products": {
"GET": "findAll",
"POST": "create"
},
"/products/:id": {
"GET": "findById",
"PUT": "update",
"DELETE": "deleteById"
},
"/orders": {
"GET": "findAllOrders",
"POST": "createOrder"
},
"/orders/:id": {
"GET": "findById",
"PUT": "update",
"DELETE": "deleteById"
}
}
```
**实现CRUD操作**
```javascript
// GET all products
app.get("/products", (req, res) => {
res.json(db.products);
});
// POST a new product
app.post("/products", (req, res) => {
const newProduct = req.body;
db.products.push(newProduct);
res.json(newProduct);
});
// GET a product by id
app.get("/products/:id", (req, res) => {
const product = db.products.find(product => product.id === parseInt(req.params.id));
res.json(product);
});
// PUT a product by id
app.put("/products/:id", (req, res) => {
const updatedProduct = req.body;
const product = db.products.find(product => product.id === parseInt(req.params.id));
product.name = updatedProduct.name;
product.description = updatedProduct.description;
product.price = updatedProduct.price;
product.quantity = updatedProduct.quantity;
res.json(product);
});
// DELETE a product by id
app.delete("/products/:id", (req, res) => {
db.products = db.products.filter(product => product.id !== parseInt(req.params.id));
res.json({ message: "Product deleted successfully" });
});
// GET all orders
app.get("/orders", (req, res) => {
res.json(db.orders);
});
// POST a new order
app.post("/orders", (req, res) => {
const newOrder = req.body;
db.orders.push(newOrder);
res.json(newOrder);
});
// GET an order by id
app.get("/orders/:id", (req, res) => {
const order = db.orders.find(order => order.id === parseInt(req.params.id));
res.json(order);
});
// PUT an order by id
app.put("/orders/:id", (req, res) => {
const updatedOrder = req.body;
const order = db.orders.find(order => order.id === parseInt(req.params.id));
order.products = updatedOrder.products;
order.total = updatedOrder.total;
res.json(order);
});
// DELETE an order by id
app.delete("/orders/:id", (req, res) => {
db.orders = db.orders.filter(order => order.id !== parseInt(req.params.id));
res.json({ message: "Order deleted successfully" });
});
```
### 4.3 实现数据可视化仪表盘
**创建图表数据模型**
```json
{
"label": "string",
"value": "number"
}
```
**定义路由**
```json
{
"/charts": {
"GET": "findAll"
}
}
```
**实现获取图表数据**
```javascript
// GET all chart data
app.get("/charts", (req, res) => {
const chartData = [
{ label: "Sales", value: 100 },
{ label: "Marketing", value: 50 },
{ label: "Support", value: 25 }
];
res.json(chartData);
});
```
# 5.1 性能优化策略
JSON Server是一个轻量级的RESTful API框架,但在处理大量数据或并发请求时,可能会遇到性能瓶颈。为了提高JSON Server的性能,可以采取以下优化策略:
### 1. 缓存数据
JSON Server默认情况下不缓存数据,这会导致频繁的数据库查询,从而降低性能。可以通过使用内存缓存或Redis等外部缓存机制来缓存数据,减少数据库访问次数。
```
// 在server.js中添加缓存中间件
const redis = require("redis");
const client = redis.createClient();
app.use(async (req, res, next) => {
const key = req.originalUrl;
const cachedData = await client.get(key);
if (cachedData) {
res.send(cachedData);
} else {
next();
}
});
```
### 2. 优化数据库查询
JSON Server使用MongoDB作为数据库,优化数据库查询可以有效提高性能。可以使用索引、复合索引和查询计划来优化查询。
```
// 创建索引
db.collection("posts").createIndex({ title: "text" });
// 使用复合索引
db.collection("posts").createIndex({ author: 1, title: 1 });
// 使用查询计划
db.collection("posts").explain().find({ author: "John" });
```
### 3. 使用分页和限制
对于返回大量数据的查询,可以使用分页和限制来减少一次性返回的数据量,从而提高性能。
```
// 使用分页
app.get("/posts", (req, res) => {
const page = parseInt(req.query.page) || 1;
const limit = parseInt(req.query.limit) || 10;
db.collection("posts").find().skip((page - 1) * limit).limit(limit).toArray((err, posts) => {
res.send(posts);
});
});
```
### 4. 优化路由
JSON Server使用Express作为路由框架,优化路由可以提高处理请求的效率。可以使用路由分组、中间件和路由缓存来优化路由。
```
// 使用路由分组
app.use("/api/v1", require("./routes/v1"));
// 使用中间件
app.use(express.json());
// 使用路由缓存
const router = express.Router();
router.cacheTime = 600; // 缓存时间为10分钟
router.get("/posts", (req, res) => {
res.send(posts);
});
app.use("/api/v1/posts", router);
```
### 5. 使用CDN
对于需要频繁访问的静态资源,可以使用CDN(内容分发网络)来缓存和加速访问,从而提高性能。
```
// 在server.js中配置CDN
app.use(express.static("public", {
maxAge: 3600000, // 缓存时间为1小时
setHeaders: (res, path, stat) => {
res.set("Cache-Control", "public, max-age=3600");
}
}));
```
0
0
相关推荐
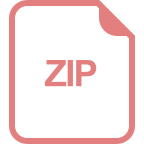
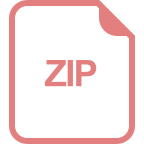
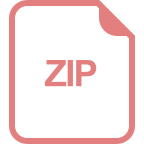
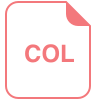
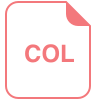
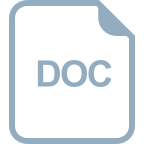
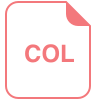
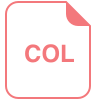
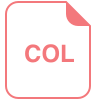