字符设备文件操作及数据传输
发布时间: 2023-12-15 12:26:09 阅读量: 95 订阅数: 46 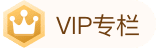
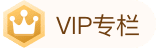
# 1. 简介
## 1.1 什么是字符设备文件
字符设备文件是指在Linux系统中用来访问设备驱动程序的一种文件类型,它通过系统调用来与设备进行通信,将设备看作是一个字节流。典型的字符设备文件包括键盘、鼠标、串口等可以逐个字符进行读写的设备。
## 1.2 字符设备文件的重要性
字符设备文件对于与用户空间进行通信以及访问硬件设备非常重要。它提供了一种标准的接口来访问硬件设备,使得用户空间的程序可以方便地进行输入输出操作,而不需要关心底层硬件设备的具体细节。
## 1.3 字符设备文件与块设备文件的区别
字符设备文件与块设备文件在数据传输方式以及设备访问方式上有所不同。块设备文件是按块传输数据,而字符设备文件则是按字符传输数据。块设备文件通常用于磁盘、U盘等存储设备,而字符设备文件通常用于串口、音频设备等逐字符读写的设备。
## 2. 字符设备文件操作
字符设备文件是操作系统中的一种特殊文件类型,用于与设备驱动程序进行通信。在Linux系统中,字符设备文件以字符流的形式读写数据,例如键盘、鼠标、串口等设备。在本章节中,我们将详细介绍字符设备文件的操作方法。
### 2.1 创建字符设备文件
要操作字符设备文件,首先需要创建相应的字符设备文件。在Linux系统中,可以使用`mknod`命令或者`mkdev`命令来创建字符设备文件。下面是使用`mknod`命令创建字符设备文件的示例:
```bash
$ sudo mknod /dev/mydevice c 240 0
```
上述命令将创建一个名为`mydevice`的字符设备文件,并将其主设备号设置为240,次设备号设置为0。创建字符设备文件成功后,就可以对其进行读写操作。
### 2.2 打开和关闭字符设备文件
在C语言中,可以使用`open`函数来打开字符设备文件。`open`函数接受一个文件路径作为参数,并返回一个文件描述符,用于后续的文件操作。
```c
#include <fcntl.h>
int open(const char *pathname, int flags);
```
下面是打开字符设备文件的示例代码:
```c
#include <stdio.h>
#include <fcntl.h>
int main() {
int fd = open("/dev/mydevice", O_RDWR);
if (fd < 0) {
perror("Failed to open device");
return -1;
}
// Do something with the device
close(fd);
return 0;
}
```
在打开字符设备文件后,可以进行读取、写入等操作。操作完成后,需要使用`close`函数关闭文件描述符,释放资源。
### 2.3 读取字符设备文件
在C语言中,可以使用`read`函数从字符设备文件中读取数据。`read`函数接受三个参数,文件描述符、数据缓冲区和读取的最大字节数。
```c
#include <unistd.h>
ssize_t read(int fd, void *buf, size_t count);
```
下面是从字符设备文件中读取数据的示例代码:
```c
#include <stdio.h>
#include <fcntl.h>
#include <unistd.h>
int main() {
int fd = open("/dev/mydevice", O_RDONLY);
if (fd < 0) {
perror("Failed to open device");
return -1;
}
char buf[1024];
ssize_t bytesRead = read(fd, buf, sizeof(buf));
if (bytesRead < 0) {
perror("Failed to read device");
close(fd);
return -1;
}
// Process the read data
close(fd);
return 0;
}
```
### 2.4 写入字符设备文件
在C语言中,可以使用`write`函数向字符设备文件中写入数据。`write`函数接受三个参数,文件描述符、数据缓冲区和写入的字节数。
```c
#include <unistd.h>
ssize_t write(int fd, const void *buf, size_t count);
```
下面是向字符设备文件中写入数据的示例代码:
```c
#include <stdio.h>
#include <fcntl.h>
#include <unistd.h>
int main() {
int fd = open("/dev/mydevice", O_WRONLY);
if (fd < 0) {
perror("Failed to open device");
return -1;
}
char data[] = "Hello, device!";
ssize_t bytesWritten = write(fd, data, sizeof(data) - 1);
if (bytesWritten < 0) {
perror("Failed to write device");
close(fd);
return -1;
}
// Process the written data
close(fd);
return 0;
}
```
### 2.5 定位和修改字符设备文件
在C语言中,可以使用`lseek`函数在字符设备文件中进行定位。`lseek`函数接受三个参数,文件描述符、偏移量和定位基准。
```c
#include <unistd.h>
off_t lseek(int fd, off_t offset, int whence);
```
下面是在字符设备文件中进行定位的示例代码:
```c
#include <stdio.h>
#include <fcntl.h>
#include <unistd.h>
int main() {
int fd = open("/dev/mydevice", O_RDWR);
if (fd < 0) {
perror("Failed to open device");
return -1;
}
off_t offset = lseek(fd, 0, SEEK_SET);
if (offset < 0) {
perror("Failed to seek device");
close(fd);
return -1;
}
// Modify the device at the specified offset
cl
```
0
0
相关推荐
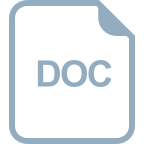
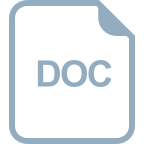
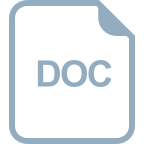
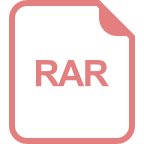
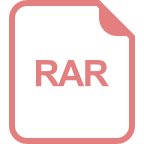
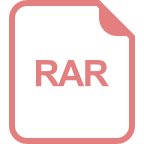
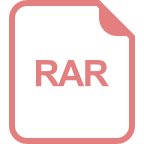
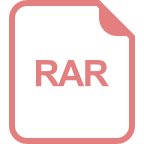
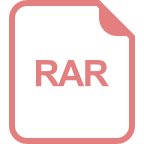