Mat类图像边缘检测:图像特征提取的利器,识别图像中的物体轮廓
发布时间: 2024-08-13 10:58:17 阅读量: 23 订阅数: 33 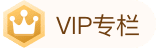
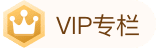

# 1. 图像边缘检测概述
图像边缘检测是计算机视觉领域的一项基本技术,它用于识别和提取图像中的边缘,即图像中亮度或颜色的突然变化。边缘检测算法通过计算图像每个像素的梯度或二阶导数来检测这些变化。边缘检测在图像分析、目标识别、图像分割和图像增强等广泛的应用中发挥着至关重要的作用。
# 2. Mat类图像边缘检测算法
### 2.1 Canny边缘检测算法
#### 2.1.1 算法原理
Canny边缘检测算法是一种多阶段的边缘检测算法,它通过以下步骤来检测图像中的边缘:
1. **高斯滤波:**使用高斯滤波器对图像进行平滑,以去除噪声和保留边缘信息。
2. **计算梯度:**使用Sobel算子或Prewitt算子计算图像中每个像素的梯度幅度和方向。
3. **非极大值抑制:**沿梯度方向遍历每个像素,并保留梯度幅度最大的像素,同时抑制其他像素。
4. **双阈值化:**使用两个阈值(高阈值和低阈值)对梯度幅度进行阈值化。高阈值用于确定强边缘,而低阈值用于确定弱边缘。
5. **滞后滞后连接:**使用滞后滞后连接技术将弱边缘连接到强边缘,形成完整的边缘。
#### 2.1.2 算法实现
```python
import cv2
import numpy as np
def canny_edge_detection(image, sigma=1.4, low_threshold=0.05, high_threshold=0.1):
"""
Canny边缘检测算法
参数:
image: 输入图像
sigma: 高斯滤波器的标准差
low_threshold: 低阈值
high_threshold: 高阈值
返回:
边缘检测后的图像
"""
# 高斯滤波
blurred_image = cv2.GaussianBlur(image, (5, 5), sigma)
# 计算梯度
sobelx = cv2.Sobel(blurred_image, cv2.CV_64F, 1, 0, ksize=3)
sobely = cv2.Sobel(blurred_image, cv2.CV_64F, 0, 1, ksize=3)
gradient_magnitude = np.sqrt(sobelx**2 + sobely**2)
gradient_direction = np.arctan2(sobely, sobelx)
# 非极大值抑制
nonmax_suppressed_image = np.zeros_like(gradient_magnitude)
for i in range(1, gradient_magnitude.shape[0] - 1):
for j in range(1, gradient_magnitude.shape[1] - 1):
if gradient_magnitude[i, j] == np.max(gradient_magnitude[i-1:i+2, j-1:j+2]):
nonmax_suppressed_image[i, j] = gradient_magnitude[i, j]
# 双阈值化
thresholded_image = np.zeros_like(nonmax_suppressed_image)
thresholded_image[nonmax_suppressed_image > high_threshold] = 255
thresholded_image[np.logical_and(nonmax_suppressed_image > low_threshold, nonmax_suppressed_image <= high_threshold)] = 127
# 滞后滞后连接
connected_image = np.zeros_like(thresholded_image)
for i in range(1, connected_image.shape[0] - 1):
for j in range(1, connected_image.shape[1] - 1):
if thresholded_image[i, j] == 255:
connected_image[i, j] = 255
connected_image = connect_edges(connected_image, i, j, thresholded_image)
return connected_image
```
### 2.2 Sobel边缘检测算法
#### 2.2.1 算法原理
Sobel边缘检测算法是一种一阶微分边缘检测算法,它使用以下步骤来检测图像中的边缘:
1. **使用Sobel算子计算图像中每个像素的梯度:**
- 水平梯度:`Gx = [[-1, 0, 1], [-2, 0, 2], [-1, 0, 1]]`
- 垂直梯度:`Gy = [[-1, -2, -1], [0, 0, 0], [1, 2, 1]]`
2. **计算梯度幅度和方向
0
0
相关推荐
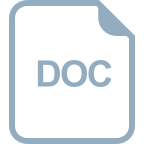
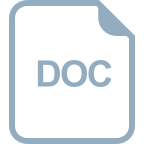
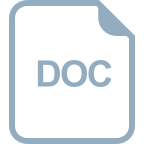
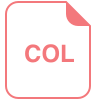
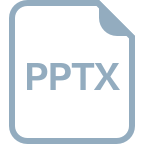
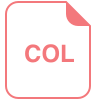
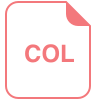
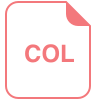
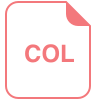