C 语言中的多线程编程与并发控制
发布时间: 2024-01-22 19:43:44 阅读量: 61 订阅数: 29 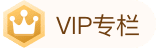
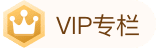
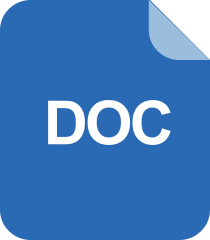
C语言多线程

# 1. 引言
## 1.1 什么是多线程编程
多线程编程是指在同一应用程序中同时运行多个线程的编程技术。每个线程都可以独立执行任务,且在同一时间内,多个线程可以同时运行,从而提高系统的并发处理能力。
## 1.2 并发控制的重要性
并发控制是指在多线程编程中,对共享资源的访问进行管理和调度,以避免出现数据竞争和不一致的情况。合理的并发控制能够确保程序的正确性和性能。
## 1.3 C语言中多线程编程的应用领域
C语言中多线程编程常用于操作系统、网络编程、服务器应用、图像处理和并行计算等领域。通过多线程编程,可以充分利用多核处理器的性能优势,提高程序的运行效率。
以上是文章的第一章节内容,接下来,我们将继续完成文章的其他章节。
# 2. 创建和管理线程
2.1 线程的创建与终止
2.2 线程的属性和状态
2.3 线程同步与互斥机制
#### 2.1 线程的创建与终止
在多线程编程中,我们需要创建线程来执行并发任务。在C语言中,可以使用 `pthread_create` 函数来创建线程。下面是一个简单的例子:
```c
#include <stdio.h>
#include <pthread.h>
void* thread_function(void* arg) {
printf("Hello from the thread!\n");
pthread_exit(NULL);
}
int main() {
pthread_t thread_id;
int result = pthread_create(&thread_id, NULL, thread_function, NULL);
if (result != 0) {
printf("Error creating thread.\n");
return 1;
}
pthread_join(thread_id, NULL);
printf("Thread exited.\n");
return 0;
}
```
在上面的例子中,我们定义了一个线程函数 `thread_function`,该函数将在新创建的线程中执行。通过调用 `pthread_create` 函数,我们创建了一个新的线程,并将 `thread_function` 作为线程的入口函数。最后,我们使用 `pthread_join` 函数来等待线程执行完毕,并输出 "Thread exited."。
#### 2.2 线程的属性和状态
在多线程编程中,线程的属性和状态对于控制和管理线程非常重要。C语言提供了一些函数来获取和设置线程的属性和状态。下面是一个例子:
```c
#include <stdio.h>
#include <pthread.h>
void* thread_function(void* arg) {
printf("Hello from the thread!\n");
pthread_exit(NULL);
}
int main() {
pthread_t thread_id;
pthread_attr_t attr;
int result = pthread_attr_init(&attr);
if (result != 0) {
printf("Error initializing thread attributes.\n");
return 1;
}
result = pthread_create(&thread_id, &attr, thread_function, NULL);
if (result != 0) {
printf("Error creating thread.\n");
return 1;
}
int detachstate;
result = pthread_attr_getdetachstate(&attr, &detachstate);
if (result != 0) {
printf("Error getting thread detach state.\n");
return 1;
}
if (detachstate == PTHREAD_CREATE_JOINABLE) {
printf("Thread is joinable.\n");
} else if (detachstate == PTHREAD_CREATE_DETACHED) {
printf("Thread is detached.\n");
}
pthread_attr_destroy(&attr);
pthread_join(thread_id, NULL);
printf("Thread exited.\n");
return 0;
}
```
在上面的例子中,我们使用 `pthread_attr_init` 函数来初始化线程属性对象,并使用 `pthread_attr_getdetachstate` 函数来获取线程的分离状态。根据返回的状态值,我们可以判断线程是可连接还是已分离。
#### 2.3 线程同步与互斥机制
在多线程编程中,线程之间可能会同时访问共享的数据,这时就需要使用线程同步和互斥机制来保护共享数据的一致性。C语言提供了互斥锁和条件变量等机制来实现线程的同步和互斥。下面是一个简单的例子:
```c
#include <stdio.h>
#include <pthread.h>
int counter = 0;
pthread_mutex_t mutex;
void* thread_function(void* arg) {
pthread_mutex_lock(&mutex);
counter++;
printf("Counter value: %d\n", counter);
pthread_mutex_unlock(&mutex);
pthread_exit(NULL);
}
int main() {
pthread_t thread1, thread2;
pthread_mutex_init(&mutex, NULL);
pthread_create(&thread1, NULL, thread_function, NULL);
pthread_create(&thread2, NULL, thread_function, NULL);
pthread_join(thread1, NULL);
pthread_join(thread2, NULL);
pthread_mutex_destroy(&mutex);
return 0;
}
```
在上面的例子中,我们通过 `pthread_mutex_lock` 和 `pthread_mutex_unlock` 函数来保护 `counter` 变量的访问。在每个线程中修改 `counter` 值之前,我们先通过 `pthread_mutex_lock` 函数获得互斥锁,执行完修改操作后再通过 `pthread_mutex_unlock` 函数释放互斥锁。这样可以确保每个线程先后访问 `counter` 变量,避免出现竞争条件。
以上是线程的创建和管理的简单示例。在实际应用中,需要根据具体场景来选择合适的线程创建方式和使用相应的线程同步机制来确保线程安全。
# 3. 线程间的通信与共享资源管理
在多线程编程中,线程之间需要进行通信并管理共享资源,这是非常重要的一部分。下面将介绍一些相关的内容:
#### 3.1 共享数据的问题
在多线程环境中,多个线程可能需要同时访问和修改共享的数据,这就会引发
0
0
相关推荐
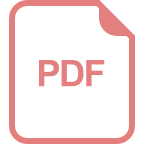
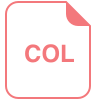
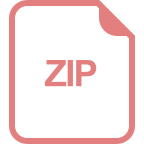
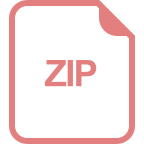
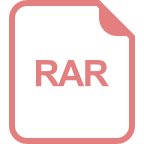
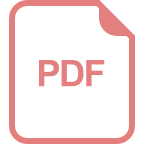
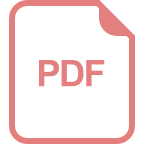