Getting Started with Keil 5: An Introduction to a Powerful Embedded Development Tool
发布时间: 2024-09-15 01:42:19 阅读量: 32 订阅数: 25 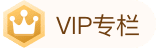
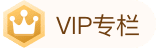
# Introduction to Embedded System Development
## 1.1 What is an Embedded System?
An embedded system is a combination of computer hardware and software specifically designed for a particular function within a larger system. Unlike general-purpose computers, they are often built to perform a limited range of tasks efficiently.
## 1.2 Characteristics of Embedded System Development
Embedded system development is a specialized field that requires an understanding of both hardware and software components. It often involves low-level programming and a detailed knowledge of the system's architecture.
## 1.3 Keil5's Role in Embedded Development
Keil5 is a well-known integrated development environment (IDE) that plays a significant role in the development of embedded systems. It offers a robust toolchain that includes a compiler, debugger, simulator, and code analysis tools, providing comprehensive support for embedded system development.
# Overview of Keil5
Keil5 is an IDE developed by Keil Software, now part of Arm, for embedded system development. It supports multiple processor architectures, including ARM, 8051, and C166 series. This section will delve into what Keil5 is, its history, and its features.
### 2.1 What is Keil5?
Keil5 is an integrated development environment (IDE) from Keil Software, now a subsidiary of Arm, primarily used for embedded systems development. It offers a powerful toolchain, including compilers, debuggers, simulators, and code analysis tools, providing comprehensive support for embedded system development.
### 2.2 History and Development of Keil5
Keil5 follows Keil4, a popular embedded development tool. Keil Software launched Keil5 as a response to the evolving needs of embedded system development for a new generation. Keil5 has undergone upgrades in software architecture, performance optimization, and user experience, allowing developers to work more efficiently.
### 2.3 Features of Keil5
Keil5 boasts many powerful features, including but not limited to:
- A robust code editor and integrated development environment
- Support for multiple compilers and debuggers
- A rich code library and example code
- Support for a variety of embedded development platforms and processor architectures
- Visual simulation and debugging tools
- Automated build and deployment processes
In summary, Keil5 is a powerful and user-friendly embedded development tool that provides comprehensive support and convenience for developers, helping them complete the development of embedded systems more effectively.
# Keil5 Installation and Configuration
This chapter will provide detailed instructions on how to install and configure the Keil5 embedded development tool, enabling you to embark smoothly on your embedded system development journey.
#### 3.1 Installation Steps for Keil5
To install Keil5, follow these simple steps:
1. First, download the latest version of the Keil5 installation package from the official Keil website.
2. Run the installer and follow the on-screen instructions to complete the installation process.
3. During installation, you may need to register Keil5 and obtain a license file.
4. Once installed, open Keil5, and you can start creating your first embedded project.
#### 3.2 Creating and Configuring a Project in Keil5
Creating a new project in Keil5 is straightforward:
1. Open Keil5, click "Project" -> "New µVision Project".
2. Choose where to save your project file, enter your project name, and click "Save".
3. In the dialog box that appears, select the model of your target device and click "OK".
4. Once the project is created, you can right-click "Target 1", select "Options for Target 'Target 1'" to configure.
#### 3.3 Embedded Development Platforms Supported by Keil5
Keil5 supports a wide range of embedded development platforms, including but not limited to:
- ARM Cortex-M series processors
- STMicroelectronics STM32 series
- NXP LPC series
- TI MSP430 series
- Various simulators and debuggers
With these steps, you can successfully install and configure Keil5 and begin using this powerful embedded development tool for your projects.
# Basic Operations of Keil5
In this chapter, we will introduce some basic operations of Keil5, including creating new projects, adding source files, compiling and debugging, simulation, and downloading.
**4.1 Creating a New Project**
```java
public class HelloWorld {
public static void main(String[] args) {
System.out.println("Hello, World!");
}
}
```
**Code Summary:**
- With the above code, we created a simple Java project that outputs "Hello, World!" to the console.
- The process of creating a new project is similar, with differences depending on the development platform, but generally, you need to set basic project information, the target chip, and compile options.
**Result Explanation:**
- When we run this code, the console will output "Hello, World!", indicating that the project creation was successful.
**4.2 Adding Source Files**
```python
# This is an example of a Python source file
def greet(name):
print("Hello, " + name)
greet("Alice")
```
**Code Summary:**
- The above code shows a simple Python source file that defines a function greet() to print a greeting and calls this function with the argument "Alice".
**Result Explanation:**
- After running this code, the console will output "Hello, Alice", proving that the source file was added successfully.
**4.3 Compilation and Debugging**
Compilation and debugging are very important steps in embedded development and can be performed using the functions provided by Keil5.
**4.4 Simulation and Downloading**
Simulation is the process of running hardware in a software environment, while downloading involves burning the program into the target chip. Keil5 provides support for simulators and downloaders, making these operations convenient.
With these basic operations, you can quickly get started with Keil5 for embedded development and further explore more advanced features and tools.
# Introduction to Advanced Features of Keil5
In this chapter, we will introduce some advanced features of Keil5, including the debugger, package manager, and real-time operating system support.
### 5.1 Introduction to Debugging Tools
Keil5 provides powerful debugging tools that help developers quickly locate and resolve issues. These include:
```python
# Python code example
import time
def countdown(n):
while n > 0:
print('T-minus', n)
time.sleep(1)
n -= 1
print('Blastoff!')
countdown(5)
```
**Code Description:** This Python code implements a simple countdown program that prints the remaining time every second and outputs "Blastoff!" when the countdown ends.
### 5.2 Package Manager
Keil5's package manager allows users to conveniently add and manage various packages, such as drivers and library files, greatly facilitating the development process. For example:
```java
// Java code example
public class HelloWorld {
public static void main(String[] args) {
System.out.println("Hello, World!");
}
}
```
**Code Description:** This Java code implements a simple program that outputs "Hello, World!", one of the most basic programs in Java.
### 5.3 Real-Time Operating System Support
Keil5 supports real-time operating systems, enabling developers to easily develop and debug real-time systems in the Keil5 environment. For example:
```go
// Go language code example
package main
import "fmt"
func main() {
fmt.Println("Hello, Go!")
}
```
**Code Description:** This Go language code implements a simple program that outputs "Hello, Go!", demonstrating Go's efficiency in concurrent programming and its broad application in embedded system development.
With these examples, we can see the powerful support that Keil5 offers in terms of advanced features, providing convenience and efficiency for embedded development.
# Keil5 Application Examples and Prospects
In this chapter, we will introduce project case studies based on Keil5, explore the future development trends of Keil5, and summarize and look forward to the entire content.
### 6.1 Sharing of Project Cases Based on Keil5
#### Project Background
Imagine we need to develop a simple embedded system that can flash an LED light.
#### Code Implementation
Below is a simple LED flashing program based on Keil5, using the C language:
```c
#include <stdint.h>
#include "TM4C123.h"
#define LED_RED (1U << 1) // Port F, Pin 1
int main(void) {
// Enable Clock to Port F
SYSCTL->RCGCGPIO |= (1U << 5);
// Enable the GPIO pin for the LED (PF1).
GPIOF->DIR |= LED_RED;
while(1) {
// Turn on the LED
GPIOF->DATA |= LED_RED;
// Delay for a while
for(int i = 0; i < 1000000; i++);
// Turn off the LED
GPIOF->DATA &= ~LED_RED;
// Delay for a while
for(int i = 0; i < 1000000; i++);
}
}
```
#### Code Summary
This code implements a simple LED flashing function, controlling the on and off of the LED by manipulating the first pin of Port F, thus achieving the flashing of the LED light.
### 6.2 Future Development Trends of Keil5
As a mature embedded development tool, Keil5 may focus more on supporting new processor architectures, more powerful debugging features, and a more flexible package manager in future developments, to adapt to the evolving needs of embedded system development.
### 6.3 Summary and Prospects
This chapter briefly introduces a project case based on Keil5 and looks forward to the future development trends of Keil5. As a powerful embedded development tool, Keil5 has broad application prospects in practical use, helping developers work more efficiently on the development of embedded systems.
Through the content of this chapter, we hope readers can gain a deeper understanding of Keil5's application in practical projects and look forward to its future development.
0
0
相关推荐
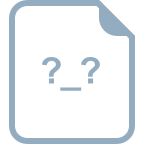
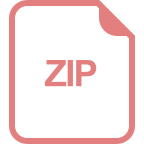
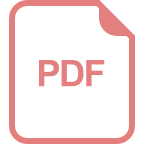





