Python实现OpenCV腐蚀与膨胀:图像处理实战指南,助你成为图像处理大师
发布时间: 2024-08-10 18:21:03 阅读量: 156 订阅数: 22 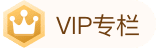
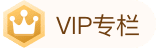

# 1. 图像处理基础**
图像处理是一门利用计算机对图像进行处理、分析和修改的学科。它广泛应用于计算机视觉、医学成像、遥感和工业检测等领域。图像处理的基础知识包括:
* **图像表示:**图像由像素组成,每个像素表示图像中一个特定位置的颜色或灰度值。图像通常使用二维数组或矩阵来表示。
* **图像格式:**常见的图像格式包括 JPEG、PNG、BMP 和 TIFF。每种格式都有自己的特点,例如压缩率、颜色深度和支持的透明度。
* **图像处理操作:**图像处理操作包括图像变换(如旋转、缩放和裁剪)、图像增强(如对比度调整和锐化)和图像分析(如边缘检测和对象识别)。
# 2. OpenCV腐蚀与膨胀理论
### 2.1 腐蚀和膨胀的概念
**腐蚀**是一种图像处理操作,它通过使用称为内核的结构元素来缩小图像中的物体。内核通常是一个矩形或圆形的掩码,其中心像素为1,其余像素为0。腐蚀操作将内核中心像素与图像中的相应像素进行比较。如果内核中心像素为1,并且所有其他内核像素与图像中的相应像素匹配,则图像中的相应像素被保留。否则,图像中的相应像素被设置为0。
**膨胀**是腐蚀的反向操作。它使用相同的内核结构,但将内核中心像素与图像中的相应像素进行比较。如果内核中心像素为1,或者任何其他内核像素与图像中的相应像素匹配,则图像中的相应像素被保留。否则,图像中的相应像素被设置为0。
### 2.2 腐蚀和膨胀的数学形态学基础
腐蚀和膨胀是数学形态学中的基本操作。数学形态学是一门处理图像形状的学科。腐蚀和膨胀操作可以用来提取图像中的特定形状或特征。
**腐蚀**操作可以用来缩小图像中的物体,同时保持其形状。**膨胀**操作可以用来扩大图像中的物体,同时保持其形状。
腐蚀和膨胀操作可以组合起来执行更复杂的图像处理任务。例如,腐蚀操作可以用来去除图像中的噪声,而膨胀操作可以用来连接图像中的断开部分。
**代码块:**
```python
import cv2
# 腐蚀操作
eroded_image = cv2.erode(image, kernel)
# 膨胀操作
dilated_image = cv2.dilate(image, kernel)
```
**逻辑分析:**
* `erode()`函数接受图像和内核作为参数,并返回腐蚀后的图像。
* `dilate()`函数接受图像和内核作为参数,并返回膨胀后的图像。
* 内核是一个矩形或圆形的掩码,其中心像素为1,其余像素为0。
**参数说明:**
* `image`:要处理的图像。
* `kernel`:用于腐蚀或膨胀操作的内核。
* `eroded_image`:腐蚀后的图像。
* `dilated_image`:膨胀后的图像。
**mermaid格式流程图:**
```mermaid
graph LR
subgraph 腐蚀
image --> erode() --> eroded_image
end
subgraph 膨胀
image --> dilate() --> dilated_image
end
```
# 3. OpenCV腐蚀与膨胀实践**
### 3.1 OpenCV腐蚀操作
#### 3.1.1 erode()函数的使用
腐蚀操作由`erode()`函数实现,其语法如下:
```python
cv2.erode(src, kernel, dst=None, anchor=None, iterations=1, borderType=None, borderValue=None)
```
**参数说明:**
* `src`: 输入图像
* `kernel`: 腐蚀核,指定腐蚀操作的形状和大小
* `dst`: 输出图像,可省略
* `anchor`: 锚点,指定腐蚀核的中心位置,默认为`(-1, -1)`,表示核的中心位于图像的左上角
* `iterations`: 腐蚀操作的迭代次数,默认为1
* `borderType`: 边界处理类型,默认为`cv2.BORDER_CONSTANT`
* `borderValue`: 边界填充值,默认为0
**代码示例:**
```python
import cv2
import numpy as np
# 读取图像
image = cv2.imread('image.jpg')
# 创建腐蚀核
kernel = np.ones((3, 3), np.uint8)
# 执行腐蚀操作
eroded_image = cv2.erode(image, kernel)
# 显示结果
cv2.imshow('Original Image', image)
cv2.imshow('Eroded Image', eroded_image)
cv2.waitKey(0)
cv2.destroyAllWindows()
```
**逻辑分析:**
1. 读取原始图像并创建腐蚀核。
2. 使用`erode()`函数执行腐蚀操作,指定腐蚀核和迭代次数。
3. 显示原始图像和腐蚀后的图像。
#### 3.1.2 腐蚀的应用场景
腐蚀操作广泛应用于图像处理中,主要用于:
* **图像降噪:**去除图像中的小噪点和孤立像素。
* **图像分割:**分离图像中的不同区域。
* **图像形态学:**进行图像形态学变换,如开运算和闭运算。
### 3.2 OpenCV膨胀操作
#### 3.2.1 dilate()函数的使用
膨胀操作由`dilate()`函数实现,其语法如下:
```python
cv2.dilate(src, kernel, dst=None, anchor=None, iterations=1, borderType=None, borderValue=None)
```
**参数说明:**
与`erode()`函数的参数相同。
**代码示例:**
```python
import cv2
import numpy as np
# 读取图像
image = cv2.imread('image.jpg')
# 创建膨胀核
kernel = np.ones((3, 3), np.uint8)
# 执行膨胀操作
dilated_image = cv2.dilate(image, kernel)
# 显示结果
cv2.imshow('Original Image', image)
cv2.imshow('Dilated Image', dilated_image)
cv2.waitKey(0)
cv2.destroyAllWindows()
```
**逻辑分析:**
与腐蚀操作类似,使用`dilate()`函数执行膨胀操作,指定膨胀核和迭代次数。
#### 3.2.2 膨胀的应用场景
膨胀操作也广泛应用于图像处理中,主要用于:
* **图像连接:**连接图像中分离的区域。
* **图像填充:**填充图像中的孔洞和空隙。
* **图像形态学:**进行图像形态学变换,如开运算和闭运算。
# 4. 腐蚀与膨胀的图像处理应用**
**4.1 图像降噪**
图像降噪是图像处理中一项重要的任务,腐蚀和膨胀操作可以有效地去除图像中的噪声。
**4.1.1 腐蚀去噪原理**
腐蚀操作可以去除图像中的小物体,包括噪声点和噪声区域。腐蚀的原理是使用一个结构元素(通常是一个矩形或圆形)在图像上滑动。当结构元素完全包含在图像的一个区域内时,该区域将被保留。否则,该区域将被移除。
**4.1.2 膨胀去噪原理**
膨胀操作可以填充图像中的小孔洞,包括噪声点和噪声区域。膨胀的原理是使用一个结构元素在图像上滑动。当结构元素与图像中的一个区域重叠时,该区域将被保留。否则,该区域将被移除。
**4.2 图像增强**
腐蚀和膨胀操作还可以用于图像增强。
**4.2.1 腐蚀图像增强**
腐蚀操作可以锐化图像中的边缘。腐蚀的原理是使用一个小的结构元素在图像上滑动。当结构元素与图像中的边缘重叠时,边缘将被保留。否则,边缘将被移除。
**4.2.2 膨胀图像增强**
膨胀操作可以平滑图像中的边缘。膨胀的原理是使用一个大的结构元素在图像上滑动。当结构元素与图像中的边缘重叠时,边缘将被保留。否则,边缘将被移除。
**代码示例:**
```python
import cv2
import numpy as np
# 腐蚀去噪
image = cv2.imread('noisy_image.jpg')
kernel = np.ones((3, 3), np.uint8)
eroded_image = cv2.erode(image, kernel)
# 膨胀去噪
dilated_image = cv2.dilate(image, kernel)
# 腐蚀图像增强
sharpened_image = cv2.erode(image, np.ones((2, 2), np.uint8))
# 膨胀图像增强
smoothed_image = cv2.dilate(image, np.ones((5, 5), np.uint8))
# 显示结果
cv2.imshow('Original Image', image)
cv2.imshow('Eroded Image', eroded_image)
cv2.imshow('Dilated Image', dilated_image)
cv2.imshow('Sharpened Image', sharpened_image)
cv2.imshow('Smoothed Image', smoothed_image)
cv2.waitKey(0)
cv2.destroyAllWindows()
```
**逻辑分析:**
* `cv2.erode()`函数使用指定的结构元素对图像进行腐蚀操作。
* `cv2.dilate()`函数使用指定的结构元素对图像进行膨胀操作。
* `np.ones()`函数创建一个填充有1的矩形结构元素。
* `cv2.imshow()`函数显示图像。
* `cv2.waitKey()`函数等待用户按下任意键。
* `cv2.destroyAllWindows()`函数关闭所有打开的窗口。
# 5.1 自定义腐蚀和膨胀核
### 5.1.1 getStructuringElement()函数的使用
OpenCV提供了`getStructuringElement()`函数来创建自定义的腐蚀和膨胀核。该函数接受两个参数:
- `shape`:指定核的形状,可以是`MORPH_RECT`(矩形)、`MORPH_CROSS`(十字形)、`MORPH_ELLIPSE`(椭圆)等。
- `ksize`:指定核的大小,是一个元组,表示核的宽度和高度。
```python
import cv2
# 创建一个 3x3 的矩形核
kernel = cv2.getStructuringElement(cv2.MORPH_RECT, (3, 3))
# 创建一个 5x5 的十字形核
kernel = cv2.getStructuringElement(cv2.MORPH_CROSS, (5, 5))
# 创建一个 7x7 的椭圆核
kernel = cv2.getStructuringElement(cv2.MORPH_ELLIPSE, (7, 7))
```
### 5.1.2 自定义核的应用
自定义核可以用于腐蚀和膨胀操作,以实现特定的图像处理效果。例如,矩形核可以用于水平或垂直方向上的腐蚀或膨胀,而十字形核可以用于对角线方向上的腐蚀或膨胀。椭圆核可以用于平滑图像或填充孔洞。
```python
import cv2
# 读取图像
image = cv2.imread('image.jpg')
# 创建一个 3x3 的矩形核
kernel = cv2.getStructuringElement(cv2.MORPH_RECT, (3, 3))
# 进行腐蚀操作
eroded_image = cv2.erode(image, kernel)
# 进行膨胀操作
dilated_image = cv2.dilate(image, kernel)
# 显示结果图像
cv2.imshow('Eroded Image', eroded_image)
cv2.imshow('Dilated Image', dilated_image)
cv2.waitKey(0)
cv2.destroyAllWindows()
```
0
0
相关推荐








