【代码重构核心:FBP模型角色】:Python中的先决条件与实践
发布时间: 2024-11-13 03:18:56 阅读量: 40 订阅数: 33 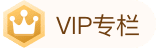
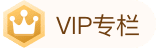
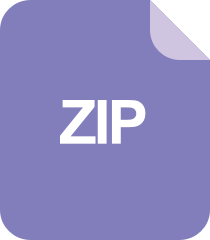
fbp:FBP流定义语言解析器
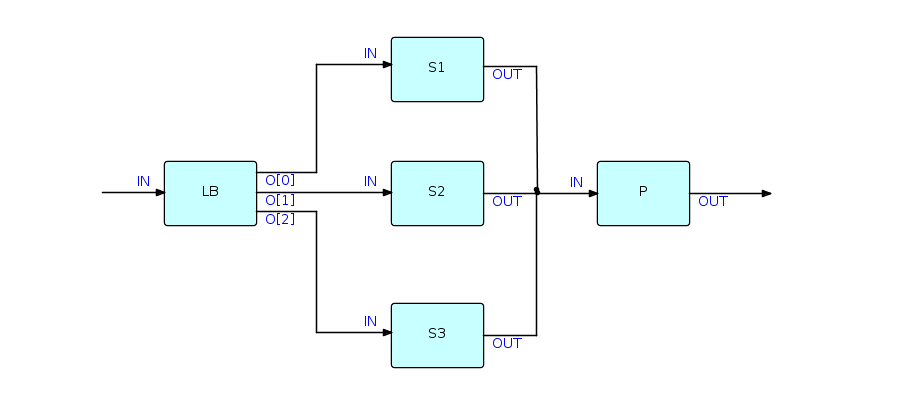
# 1. 代码重构的核心概念与重要性
代码重构是软件开发过程中的一个关键环节,它关注于提高现有代码的质量而不改变其外部行为。代码重构不仅对改善代码的可读性、可维护性和可扩展性至关重要,而且对于长期项目来说是保持代码库健康和项目可管理性的必要手段。通过重构,开发者可以移除重复的代码,优化设计模式,降低复杂性,并通过持续改进来适应新的业务需求。重构还帮助减少技术债务,即未来对软件进行修改时可能增加的额外工作量。本章将介绍代码重构的核心概念,并探讨其在现代软件开发中的重要性。
# 2. FBP模型简介及其在Python中的应用
### 2.1 FBP模型理论基础
#### 2.1.1 FBP模型的定义和原理
**FBP模型**,全称为**流式编程模型**(Flow-Based Programming),是一种编程范式,它强调数据流而不是控制流。在FBP中,程序由一系列的组件组成,这些组件通过数据流相连接。每个组件完成特定的任务,并通过端口接收和发送数据。这种方法的一个关键特性是组件之间的异步处理和并发执行,这使得程序设计更加模块化,更容易理解和维护。
FBP模型的核心原理是基于数据流的概念,即程序的执行取决于数据的到达,而不是执行的顺序。组件是独立运行的实体,它们可以在任何时候处理输入数据,并生成输出数据流。这种模型提供了一种高级的抽象,可以隐藏底层并发和同步的复杂性,使开发者可以专注于解决问题的逻辑。
#### 2.1.2 FBP模型与传统编程模型的对比
与传统的顺序编程(imperative programming)或面向对象编程(object-oriented programming)相比,FBP模型在多个方面提供了不同的优势和挑战:
1. **并发性**:在传统编程模型中,并发通常需要明确的代码控制,如线程或进程的创建和管理。而在FBP模型中,由于其本质上是基于数据流的并发,开发者不需要直接管理并发细节,这降低了编程的复杂性。
2. **模块化**:FBP鼓励更高的模块化设计,因为每个组件都是独立的、自包含的,并且有着明确的输入输出接口。相比之下,传统的模型可能需要更深入地了解程序的全局状态。
3. **调试和维护**:在FBP模型中,组件的独立性简化了调试过程。每个组件可以单独测试,而且数据流的可视化使得问题的定位变得容易。传统模型中,由于所有组件共享同一执行流程和数据环境,调试可能更为复杂。
4. **重构**:FBP模型使得代码重构变得更加容易和安全。由于组件间的耦合度较低,更改一个组件的影响可以被很好地限制在局部,而不像传统模型中更改可能会涉及到整个程序。
### 2.2 FBP模型在Python中的实现
#### 2.2.1 Python环境下的FBP模型框架
Python,作为一种动态类型语言,具有高度的灵活性,这使得它成为实现FBP模型的理想选择。目前,有多个Python库和框架支持FBP模型的实现,例如**Marconi**,**Luigi**,和**Plumber**。这些工具提供了构建FBP应用所需的组件和数据流管理机制。
#### 2.2.2 FBP模型在Python中的组件和连接
在Python中,FBP模型的组件可以通过定义函数、类或使用特定框架的组件类来创建。每个组件通常具有输入端口和输出端口,用于接收和发送数据。这些组件通过数据流连接起来,通常这些连接可以是内存中的队列、文件或其他存储机制。
下面是一个简单的Python代码示例,展示了如何使用Python构建FBP组件和数据流:
```python
import queue
import threading
import time
def component_a(input_port, output_port):
while True:
data = input_port.get() # Get data from input port
if data is None:
break
result = data * 2 # Perform some operation
output_port.put(result) # Send result to output port
def component_b(input_port, output_port):
while True:
data = input_port.get()
if data is None:
break
print(f"Received data: {data}") # Print data
output_port.put(data + 1) # Send modified data
def main():
port_a_to_b = queue.Queue()
port_b_to_c = queue.Queue()
# Define component instances with input and output ports
component_a_instance = threading.Thread(target=component_a, args=(port_a_to_b, port_b_to_c))
component_b_instance = threading.Thread(target=component_b, args=(port_b_to_c, port_a_to_b))
# Start components
component_a_instance.start()
component_b_instance.start()
# Send data through the system
for i in range(5):
port_a_to_b.put(i)
# Stop components by sending None into the input ports
port_a_to_b.put(None)
port_b_to_c.put(None)
# Wait for components to finish
component_a_instance.join()
component_b_instance.join()
if __name__ == "__main__":
main()
```
在此示例中,`component_a`和`component_b`是两个简单的FBP组件,它们通过队列进行通信。主线程启动这两个组件,并通过队列发送数据。每个组件独立运行,异步处理数据,然后将结果传给下一个组件。
#### 2.2.3 实例分析:构建一个简单的FBP应用
为了更深入地理解FBP模型在Python中的应用,我们构建一个简单的例子:一个FBP应用,用于处理文本文件,执行词频统计,并输出结果。我们的应用将包括三个组件:文件读取器(FileReader),词频计算器(WordCounter),和结果输出器(ResultPrinter)。
首先,我们定义`FileReader`组件:
```python
def file_reader(input_port, output_port):
with open(input_port.get()) as ***
***
***
```
然后,`WordCounter`组件:
```python
def word_counter(input_port, output_port):
word_count = {}
for line in iter(input_port.get, None):
for word in line.split():
word_count[word] = word_count.get(word, 0) + 1
output_port.put(word_count) # Send word count dictionary to output port
```
最后,`ResultPrinter`组件:
```python
def result_printer(input_port, output_port):
while True:
word_count = input_port.get()
if word_count is None:
break
for word, count in word_count.items():
print(f"{word}: {count}")
output_port.put(None) # Signal completion to the next component
```
现在,我们把这三个组件连接起来,构建一个完整的FBP应用:
```python
def main():
file_name = "example.txt"
file_queue = queue.Queue()
word_queue = queue.Queue()
result_queue = queue.Queue()
component_file_reader = threading.Thread(target=file_reader, args=(file_name, file_queue))
component_word_counter = threading.Thread(target=word_counter, args=(file_queue, word_queue))
component_result_printer = threading.Thread(target=result_printer, args=(word_queue, result_queue))
```
0
0
相关推荐






