Thymeleaf在移动Web开发中的角色:响应式设计优化
发布时间: 2024-09-29 18:59:49 阅读量: 153 订阅数: 42 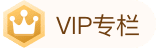
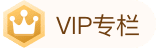
# 1. Thymeleaf简介及其在Web开发中的作用
## 1.1 Thymeleaf概述
Thymeleaf是一款功能强大的现代服务器端Java模板引擎,用于Web和独立环境。它通过自然模板功能,能够在不牺牲设计感的情况下,处理HTML、XML、JavaScript、CSS甚至是纯文本。Thymeleaf的另一大特色是它的可扩展性,通过自定义方言可以提供强大的功能,支持Web应用开发中的各种场景。
## 1.2 Thymeleaf在Web开发中的作用
在Web开发中,Thymeleaf主要被用作视图层技术,生成动态HTML内容。它能够与Spring MVC无缝集成,并且遵循MVC架构。Thymeleaf通过解析模板,将模型数据整合到视图中,生成最终用户可见的页面。使用Thymeleaf可以减少Java代码与HTML之间的耦合度,使得前后端开发分工更加明确,提高开发效率和维护性。同时,Thymeleaf对于提高Web应用的可测试性和可访问性也具有一定的帮助。
## 1.3 Thymeleaf的优势
Thymeleaf的优势在于其对HTML5的全面支持,这使得它非常适合于构建和维护静态原型或静态文档。此外,Thymeleaf能够确保即使在没有Web服务器的情况下,模板也能够正常工作,这一点对于开发和调试阶段尤其重要。它还支持Web环境中的非Web环境特性,如属性默认值、消息本地化和国际化、迭代和条件逻辑,使得它成为多种应用场景下理想的选择。
# 2. Thymeleaf与Spring Boot集成基础
## 2.1 Thymeleaf与Spring Boot的集成方法
### 2.1.1 添加Thymeleaf依赖
在Spring Boot项目中集成Thymeleaf,第一步是在项目的`pom.xml`文件中添加Thymeleaf的依赖。对于Maven项目,可以通过以下代码来实现:
```xml
<dependencies>
<!-- Spring Boot Web Starter -->
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-web</artifactId>
</dependency>
<!-- Thymeleaf Template Engine -->
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-thymeleaf</artifactId>
</dependency>
<!-- Other dependencies omitted for brevity -->
</dependencies>
```
这段依赖的添加确保了Thymeleaf和Spring Boot框架的其他Web相关的依赖(如Spring MVC、Tomcat容器等)能够被正确地引入项目中。
### 2.1.2 配置Thymeleaf模板引擎
集成Thymeleaf的第二步是配置Thymeleaf的模板引擎。Spring Boot会自动配置Thymeleaf,但是有时候我们需要自定义一些配置,比如修改模板文件的存放位置。
以下是一个自定义Thymeleaf配置的示例:
```java
@Configuration
@EnableWebMvc
public class ThymeleafConfig implements WebMvcConfigurer {
@Bean
public SpringTemplateEngine templateEngine(TemplateResolver templateResolver) {
SpringTemplateEngine engine = new SpringTemplateEngine();
engine.setTemplateResolver(templateResolver);
return engine;
}
@Bean
public TemplateResolver templateResolver() {
TemplateResolver resolver = new ClassLoaderTemplateResolver();
resolver.setPrefix("templates/");
resolver.setSuffix(".html");
resolver.setTemplateMode(TemplateMode.HTML);
return resolver;
}
}
```
在这个配置中,我们自定义了`TemplateResolver`来指定模板文件的位置。`spring-boot-starter-thymeleaf`会自动检测并使用这个自定义的配置。
## 2.2 Thymeleaf基本语法和表达式
### 2.2.1 标准表达式语法
Thymeleaf中的标准表达式分为三类:变量表达式、选择/星号表达式和消息表达式。理解这些表达式的语法和用途是使用Thymeleaf的基本要求。
- 变量表达式:`${...}`,用于访问变量值。
- 选择/星号表达式:`*{...}`,常与`th:`属性一起使用,用于访问对象属性。
- 消息表达式:`#{...}`,用于获取国际化消息。
例如,以下代码展示了一个变量表达式的使用:
```html
<p th:text="${name}"></p>
```
在该示例中,`${name}`表达式将会被替换为模型中名为`name`的变量值。
### 2.2.2 表达式基本用法和示例
在Thymeleaf模板中,表达式通常用于输出变量、执行逻辑判断、迭代以及显示国际化消息。下面是一些基本用法的示例:
```html
<!-- 输出变量 -->
<p th:text="${message}"></p>
<!-- 国际化 -->
<p th:text="#{greeting}"></p>
<!-- 条件判断 -->
<div th:if="${user != null}">
<p>Welcome, <span th:text="${user.name}"></span>!</p>
</div>
<!-- 迭代 -->
<ul>
<li th:each="user : ${users}" th:text="${user.name}"></li>
</ul>
```
在上述代码中,`th:if`和`th:each`是Thymeleaf中提供的属性,用于控制条件渲染和迭代渲染,它们使HTML可以进行动态渲染。
## 2.3 Thymeleaf的页面导航和国际化
### 2.3.1 Thymeleaf中的链接构建和页面导航
Thymeleaf提供了`th:href`属性用于构建链接。当使用`th:href`时,它能够帮助我们动态地构建URL,这样可以在不同的环境(例如开发环境和生产环境)中轻松切换。
一个典型的使用示例:
```html
<a th:href="@{/user/{id}(id=${userId})}">Go to User</a>
```
在上面的代码中,`th:href`属性使用了Thymeleaf的URL构建语法,`${userId}`将会被替换为当前上下文中的变量值。
### 2.3.2 国际化支持和资源处理
Thymeleaf天然支持国际化消息,它允许开发者通过简单的配置文件来为不同的语言环境提供文本支持。`th:text`和`th:utext`属性能够与国际化功能结合使用,使得页面内容可以按照用户的语言偏好显示。
以下是如何在Thymeleaf中使用国际化资源文件的一个示例:
```html
<!-- 在HTML中 -->
<h1 th:text="#{welcome}">Welcome</h1>
<!-- 在message_en.properties中 -->
welcome=
```
0
0
相关推荐
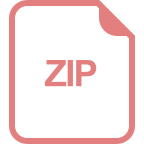
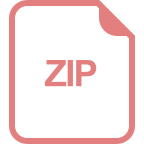
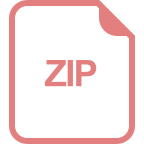
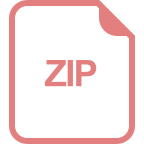
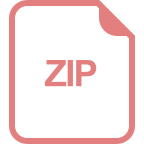
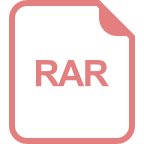
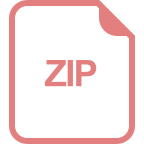
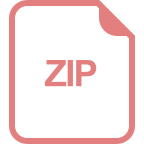
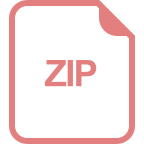