汽车单片机程序设计中的性能优化策略:5大秘诀,提升程序运行速度
发布时间: 2024-07-06 10:14:56 阅读量: 61 订阅数: 30 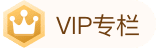
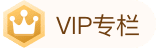
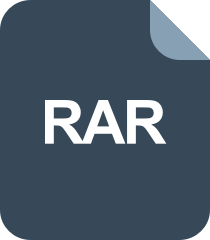
性能优化秘籍:深度解析Hadoop集群监控与调优策略
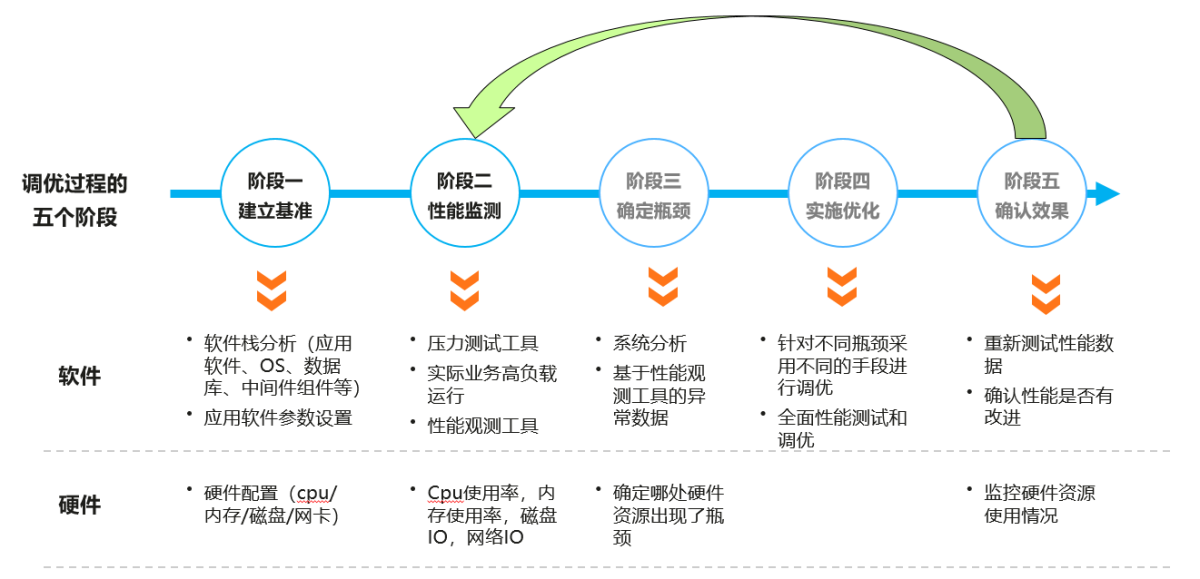
# 1. 汽车单片机程序设计中的性能优化概述
汽车单片机程序设计中,性能优化至关重要,它直接影响系统响应时间、可靠性和功耗。本概述将阐述性能优化的重要性、目标和方法,为后续章节的深入讨论奠定基础。
### 1.1 性能优化的重要性
在汽车电子系统中,单片机负责控制关键功能,如发动机管理、安全气囊部署和信息娱乐系统。性能优化可显著提高系统可靠性、响应速度和功耗效率,从而增强用户体验和安全性。
### 1.2 性能优化目标
汽车单片机程序设计的性能优化目标包括:
- 缩短指令执行时间
- 减少内存占用
- 提高代码可读性和可维护性
# 2. 单片机性能优化原理
### 2.1 单片机架构与性能影响因素
单片机是一种集成在单个芯片上的微型计算机,其性能受其架构和设计的影响。以下是一些关键因素:
- **CPU 架构:**单片机通常采用 RISC(精简指令集计算机)或 CISC(复杂指令集计算机)架构。RISC 指令集简单,执行速度快,而 CISC 指令集复杂,功能强大。
- **时钟频率:**时钟频率决定了单片机执行指令的速度。时钟频率越高,性能越好。
- **内存架构:**单片机通常具有程序存储器(ROM/Flash)和数据存储器(RAM)。程序存储器存储指令,而数据存储器存储数据。内存大小和访问速度影响性能。
- **外设接口:**单片机通常具有各种外设接口,如 GPIO、UART、SPI 等。这些接口允许单片机与外部设备通信,影响其整体性能。
### 2.2 程序优化目标与方法
单片机性能优化旨在提高程序的执行速度、减少内存占用和降低功耗。优化目标包括:
- **执行速度优化:**减少指令执行时间,提高程序吞吐量。
- **内存优化:**减少程序代码和数据占用的内存空间,提高内存利用率。
- **功耗优化:**降低程序运行时的功耗,延长电池寿命。
优化方法包括:
- **指令优化:**选择高效的指令,避免不必要的指令执行。
- **寄存器利用:**充分利用单片机的寄存器,减少内存访问次数。
- **数据结构优化:**选择合适的的数据结构,优化内存布局。
- **算法优化:**选择时间复杂度和空间复杂度较低的算法。
- **并发优化:**利用多任务调度和资源分配,提高程序并发性。
- **硬件优化:**利用外设协处理器和 DMA(直接内存访问)等硬件特性,提升性能。
**代码示例:**
```c
// 未优化代码
for (int i = 0; i < 100; i++) {
// ...
}
// 优化代码
for (int i = 0; i < 100; i += 2) {
// ...
}
```
**代码逻辑分析:**
未优化代码每次循环都会执行一次内存访问,而优化代码通过步长为 2 的循环,减少了一半的内存访问次数,提高了执行速度。
**参数说明:**
- `i`:循环变量
# 3. 5大性能优化秘诀
### 3.1 代码优化:指令选择与寄存器利用
**指令选择**
不同的指令执行效率不同,选择合适的指令可以显著提升代码性能。例如:
- 使用单周期指令代替多周期指令
- 使用位操作指令代替复杂算术指令
- 使用跳转表代替条件分支
**寄存器利用**
寄存器访问速度远高于内存访问,合理利用寄存器可以减少内存访问次数,提升代码性能。例如:
- 将频繁访问的变量存储在寄存器中
- 使用寄存器进行局部变量传递
- 使用寄存器进行循环计数
**代码块示例:**
```c
// 使用单周期指令代替多周期指令
uint32_t sum = 0;
for (int i = 0; i < N; i++) {
sum += i;
}
// 使用位操作指令代替复杂算术指令
uint32_t mask = 0x0000FFFF;
uint32_t value = 0x12345678;
value &= mask; // 清除高 16 位
// 使用跳转表代替条件分支
enum State {
STATE_A,
STATE_B,
STATE_C
};
State state = STATE_A;
void (*state_table[])() = {
state_a,
state_b,
state_c
};
state_table[state](); // 根据状态跳转到相应函数
```
### 3.2 数据结构优化:内存管理与数据布局
**内存管理**
优化内存管理可以减少内存碎片,提升内存访问效率。例如:
- 使用内存池管理动态分配的内存
- 使用对齐分配和对齐访问
- 使用缓存机制提高内存访问速度
**数据布局**
合理的数据布局可以减少内存访问时间,提升代码性能。例如:
- 将相关数据放在连续的内存区域
- 使用结构体和联合体优化数据存储
- 使用位域优化数据存储
**代码块示例:**
```c
// 使用内存池管理动态分配的内存
void* pool_alloc(size_t size) {
return malloc(size) + sizeof(size_t);
}
void pool_free(void* ptr) {
free(ptr - sizeof(size_t));
}
// 使用对齐分配和对齐访问
uint32_t* aligned_ptr = (uint32_t*)__aligned_alloc(4, 1024);
*aligned_ptr = 0x12345678; // 对齐访问
// 使用缓存机制提高内存访问速度
uint32_t cache_line_size = 64;
uint32_t* cached_ptr = (uint32_t*)__cache
```
0
0
相关推荐







