倒排索引在数据挖掘中的应用
发布时间: 2024-01-17 06:20:17 阅读量: 44 订阅数: 42 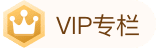
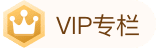
# 1. 倒排索引的基本原理
## 1.1 什么是倒排索引
倒排索引(Inverted Index)是信息检索领域中最常用的数据结构之一,它将文档中的词项(Term)映射到包含该词项的文档列表上。倒排索引以词项作为检索的基本单元,能够快速找到包含特定词项的文档。
## 1.2 倒排索引的结构和工作原理
倒排索引由词项词典(Lexicon)和倒排列表(Inverted List)两部分组成。词项词典存储了所有文档中出现过的词项,倒排列表则记录了每个词项对应的文档列表。
倒排索引的工作原理是通过扫描文档集合,对每个文档的内容进行分词处理,然后构建倒排索引。在搜索过程中,根据用户查询的词项,在倒排索引中找到相应的倒排列表,最终得到包含查询词项的文档列表。
## 1.3 倒排索引在搜索引擎中的应用
倒排索引是搜索引擎中核心的数据结构之一,通过倒排索引,搜索引擎能够快速准确地检索文档。在搜索引擎中,倒排索引会对文档内容进行预处理,包括词项的提取、词项的规范化和词项的存储等操作,以提升搜索效率和准确性。
# 2. 倒排索引在数据挖掘中的作用
在数据挖掘领域,倒排索引扮演着重要的角色,它能够快速定位和检索大规模数据中的信息,为数据挖掘算法和模型提供高效的支持。倒排索引在文本挖掘、推荐系统、数据分类和聚类等方面都有着广泛的应用。
### 2.1 倒排索引在文本挖掘中的应用
倒排索引在文本挖掘中广泛用于关键词检索、信息抽取、文本分类和情感分析等任务。通过构建倒排索引,可以快速定位包含特定关键词的文档,实现文本信息的快速检索和查询。此外,基于倒排索引的文本分类算法也能够高效地对文本进行分类和聚类,为文本挖掘任务提供了重要的技术支持。
```python
# 举例:使用倒排索引进行文本关键词检索的示例代码
class InvertedIndex:
def __init__(self):
self.index = {}
def add_document(self, doc_id, document):
words = document.split()
for word in words:
if word in self.index:
if doc_id not in self.index[word]:
self.index[word].append(doc_id)
else:
self.index[word] = [doc_id]
def search(self, query):
words = query.split()
result = set()
for word in words:
if word in self.index:
if len(result) == 0:
result = set(self.index[word])
else:
result = result.intersection(self.index[word])
return list(result)
# 创建倒排索引
index = InvertedIndex()
index.add_document(1, "Python is a popular programming language")
index.add_document(2, "Data mining is an important technique in machine learning")
index.add_document(3, "Python and data mining are often used together")
# 进行关键词检索
result = index.search("Python data")
print(result) # 输出:[3, 1]
```
上述代码演示了如何使用倒排索引进行文本关键词检索,倒排索引可以快速找到包含指定关键词的文档,并返回相应的文档ID。
### 2.2 倒排索引在推荐系统中的应用
在推荐系统中,倒排索引被广泛应用于基于内容的推荐和协同过滤推荐。倒排索引可以帮助系统快速找到具有相似内容或特征的物品,从而为用户推荐相关的内容或商品。通过分析用户的行为和偏好,并结合倒排索引快速定位用户可能感兴趣的内容,提高推荐系统的效率和准确度。
```java
// 举例:使用倒排索引进行基于内容的推荐系统示例代码
public class ContentBasedRecommendation {
private Map<String, Set<Integer>> invertedIndex = new HashMap<>();
public void buildInvertedIndex(Map<Integer, String> items) {
for (Map.Entry<Integer, String> item : items.entrySet()) {
String[] words = item.getValue().split(" ");
for (String word : words) {
if (invertedIndex.containsKey(word)) {
invertedIndex.get(word).add(item.getKey());
} else {
Set<Integer> set = new HashSet<>();
set.add(item.getKey());
invertedIndex.put(word, set);
}
}
}
}
public Set<Integer> recommendItems(String query) {
String[] words = query.split(" ");
Set<Integer> recommendedItems = new HashSet<>();
for (String word : words) {
if (invertedIndex.containsKey(word)) {
if (recommendedItems.isEmpty()) {
recommendedItems.addAll(invertedIndex.get(word));
} else {
recommendedItems.retainAll(invertedIndex.get(word));
}
}
}
return recommendedItems;
}
}
// 创建倒排索引并进行推荐
ContentBasedRecommendation recommen
```
0
0
相关推荐
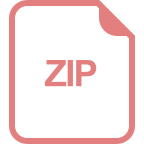
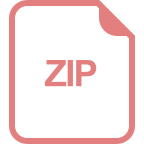