Android布局文件的设计方法
发布时间: 2024-01-30 03:39:40 阅读量: 25 订阅数: 17 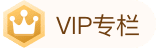
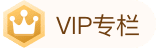
# 1. 简介
## 1.1 什么是Android布局文件
Android布局文件是用于定义Android应用程序界面的XML文件。它描述了UI组件在屏幕上的位置、大小和样式,以及它们之间的关系和交互。
## 1.2 布局文件的重要性
布局文件在Android应用程序开发中起着至关重要的作用。它不仅决定了应用程序的外观和用户体验,还能帮助开发者有效地组织和管理UI组件。
## 1.3 布局文件的设计原则
在设计布局文件时,应遵循以下原则:
- **灵活性**:布局应具备良好的灵活性,能够适应不同分辨率和屏幕尺寸的设备。
- **可复用性**:布局应该设计成可复用的组件,便于在不同的界面中重复使用。
- **易维护性**:布局应具备良好的可维护性,便于后续修改和扩展。
- **可读性**:布局文件的结构和命名应清晰明确,便于他人理解和维护。
通过遵循这些设计原则,可以使布局文件更加灵活、可扩展和易维护,提高开发效率和用户体验。
# 2. 布局文件的基本结构
在Android开发中,布局文件是构建界面的重要组成部分。不同的布局文件可以实现不同的界面效果,而Android提供了多种不同类型的布局来满足各种需求。接下来,我们将介绍布局文件的基本结构以及常用的布局类型。
### 2.1 LinearLayout布局
LinearLayout是Android中最常用的布局之一,它可以让子控件在单一方向上依次排列,包括水平排列(`android:orientation="horizontal"`)和垂直排列(`android:orientation="vertical"`)。下面是一个简单的LinearLayout布局示例:
```xml
<LinearLayout
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:orientation="vertical">
<TextView
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:text="Hello, LinearLayout!" />
<Button
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="Click Me" />
</LinearLayout>
```
在上面的示例中,LinearLayout垂直排列了一个TextView和一个Button。
### 2.2 RelativeLayout布局
RelativeLayout允许子控件相对于父布局或其他子控件进行定位,这使得RelativeLayout在复杂布局中非常有用。以下是一个简单的RelativeLayout布局示例:
```xml
<RelativeLayout
android:layout_width="match_parent"
android:layout_height="wrap_content">
<ImageView
android:id="@+id/imageView"
android:layout_width="100dp"
android:layout_height="100dp"
android:src="@drawable/ic_launcher"
android:layout_alignParentTop="true"
android:layout_centerHorizontal="true" />
<TextView
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="Centered Text"
android:layout_below="@id/imageView"
android:layout_centerHorizontal="true" />
</RelativeLayout>
```
在上面的示例中,ImageView被定位在父布局的顶部中间,而TextView则被定位在ImageView的下方并水平居中。
### 2.3 ConstraintLayout布局
ConstraintLayout是Android Studio推荐使用的布局,它允许通过约束条件来定义子控件之间的关系,从而实现灵活的布局。以下是一个简单的ConstraintLayout布局示例:
```xml
<androidx.constraintlayout.widget.ConstraintLayout
android:layout_width="match_parent"
android:layout_height="wrap_content">
<Button
android:id="@+id/button"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="Click Me"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toTopOf="parent" />
<Button
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="Button 2"
app:layout_constraintEnd_toEndOf="parent"
app:layout_constraintTop_toTopOf="parent" />
</androidx.constraintlayout.widget.ConstraintLayout>
```
在上面的示例中,两个Button分别被约束在父布局的左上角和右上角。
### 2.4 FrameLayout布局
FrameLayout是一种最简单的布局,它会将子控件叠放在左上角,常用于显示单个子控件的情况。以下是一个简单的FrameLayout布局示例:
```xml
<FrameLayout
android:layout_width="match_parent"
android:layout_height="match_parent">
<ImageView
android:layout_width="match_parent"
android:layout_height="match_parent"
android:src="@drawable/background_image" />
<TextView
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="Overlaid Text"
android:layout_gravity="center" />
</FrameLayout>
```
在上面的示例中,ImageView占据整个父布局,并且TextView叠放在其中间位置。
### 2.5 嵌套布局
在实际布局设计中,可以使用多种布局进行嵌套组合,以实现复杂的界面效果。如在LinearLayout内嵌套RelativeLayout、ConstraintLayout内嵌套LinearLayout等。嵌套布局的合理使用可以更加灵活地完成界面的设计。
通过以上示例,我们介绍了LinearLayout、RelativeLayout、ConstraintLayout和FrameLayout这几种常用的布局类型,以及嵌套布局的使用。在实际开发中,选择合适的布局类型并合理嵌套是实现界面效果的关键之一。接下来,我们将继续介绍布局文件中的常见控件。
# 3. 布局文件中的常见控件
在Android布局文件中,常常会包含一些常见的控件,这些控件用于显示文本、图片、按钮等内容。下面将介绍Android布局文件中常见的控件及其基本用法。
#### 3.1 TextView控件
TextView控件用于显示文本内容,可以用来展示静态文本、动态文本或者格式化文本。以下是一个TextView控件的示例:
```xml
<TextView
android:id="@+id/textView"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="Hello, World!"
android:textSize="24sp"
android:textColor="@android:color/black" />
```
**代码解析:**
- `android:id`:指定控件的唯一标识符。
- `android:layout_width`和`android:layout_height`:指定控件的宽度和高度。
- `android:text`:设置要显示的文本内容。
- `android
0
0
相关推荐
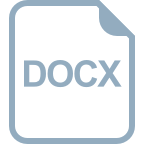
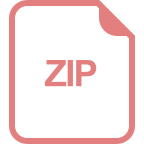
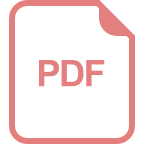





