了解神经网络中的Attention机制
发布时间: 2024-04-02 03:41:14 阅读量: 26 订阅数: 18 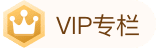
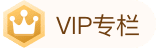
# 1. 什么是Attention机制
神经网络中的Attention机制是一种机制,用于模拟人类在处理任务时的注意力集中方式,能够帮助模型更加关注重要的部分,从而提高模型性能。在这一章节中,我们将介绍Attention机制的概念、起源以及在自然语言处理中的应用。接下来,让我们一起深入了解吧。
# 2. Attention机制的原理
- 2.1 可视化Attention权重
- 2.2 编码器-解码器框架中的Attention
- 2.3 Self-Attention机制与Transformer模型
在接下来的部分中,我们将深入探讨Attention机制的原理,包括如何可视化Attention权重、它在编码器-解码器框架中的应用,以及与Transformer模型相关的Self-Attention机制。
# 3. 不同类型的Attention机制
在神经网络中,Attention机制有多种不同类型,下面我们将介绍一些常见的Attention机制:
#### 3.1 Global Attention
全局Attention机制是将查询和所有键进行关联计算,并通过归一化得到注意力权重,然后将这些权重乘以值的组合来计算最终的输出。这种机制允许模型同时关注输入的所有部分,适用于较短的序列输入。Global Attention有不同的计算方式,包括Dot Product、Scaled Dot-Product和General等。
```python
import torch
import torch.nn.functional as F
# 定义Global Dot Product Attention函数
def global_dot_product_attention(query, key, value):
scores = torch.matmul(query, key.transpose(-2, -1))
attention_weights = F.softmax(scores, dim=-1)
output = torch.matmul(attention_weights, value)
return output
# 测试Global Dot Product Attention
query = torch.randn(2, 5, 3) # (batch_size, seq_len_q, query_dim)
key = torch.randn(2, 5, 3) # (batch_size, seq_len_k, key_dim)
value = torch.randn(2, 5, 2) # (batch_size, seq_len_v, value_dim)
output = global_dot_product_attention(query, key, value)
print(output.shape) # 输出:torch.Size([2, 5, 2])
```
#### 3.2 Local Attention
局部Attention机制与全局Attention不同,它不是直接计算所有位置的注意力权重,而是通过定义一个窗口来限制查询的关注范围,减少计算复杂度。局部Attention可以有效处理长序列输入,并提高模型的效率。
```python
# 实现Local Attention函数(仅演示部分局部窗口的计算,实际实现应考虑更多细节)
def local_attention(query, key, value, window_size=2):
batch_size, seq_len_q, _ = query.size()
_, seq_len_k, _ = key.size()
output = torch.zeros(batch_size, seq_len_q, value.size(-1))
for i in range(seq_len_q):
start = max(0, i - window_size)
end = min(i + window_size, seq_len_k)
local_query = query[:, i, :].unsqueeze(1)
local_keys = key[:, start:end, :]
local_values = value[:, start:end, :]
scores = torch.matmul(local_query, local_keys.transpose(-2, -1))
attention_weights = F.softmax(scores, dim=-1)
context = torch.matmul(attention_weights, local_values)
output[:, i, :] = context.squeeze(1)
return output
# 测试Local Attention
output = local_attention(query, key, value, window_size=1)
print(output.shape) # 输出:torch.Size([2, 5, 2])
```
#### 3.3 Multi-Head Attention
多头Attention机制通过同时学习多组查询、键、值映射来捕捉不同的关注焦点,然后将这些头的输出合并,提高模型对不同表征的关注能力,进而增强模型的泛化和表示能力。
```python
import torch.nn
# 定义Multi-Head Attention模块
class MultiHeadAttention(torch.nn.Module):
def __init__(self, num_heads, model_dim):
super(MultiHeadAttention, self).__init__()
self.num_heads = num_heads
self.model_dim = model_dim
self.head_dim = model_dim // num_heads
self.linear_query = torch.nn.Linear(model_dim, model_dim)
self.linear_key = torch.nn.Linear(model_dim, model_dim)
self.linear_value = torch.nn.Linear(model_dim, model_dim)
self.fc_out = torch.nn.Linear(model_dim, model_dim)
def forward(self, query, key, value):
# 拆分成多个头
batch_size = query.size(0)
query = self.linear_query(query).view(batch_size, -1, self.num_heads, self.head_dim).transpose(1, 2)
key = self.linear_key(key).view(batch_size, -1, self.num_heads, self.head_dim).transpose(1, 2)
value = self.linear_value(value).view(batch_size, -1, self.num_heads, self.head_dim).transpose(1, 2)
# 计算多头注意力
scores = torch.matmul(query, key.transpose(-2, -1)) / math.sqrt(self.head_dim)
attention_weights = F.softmax(scores, dim=-1)
context = torch.matmul(attention_weights, value).transpose(1, 2).contiguous().view(batch_size, -1, self.model_dim)
# 合并多头输出
output = self.fc_out(context)
return output
# 初始化Multi-Head Attention模块
multi_head_attn = MultiHeadAttention(num_heads=4, model_dim=32)
output = multi_head_attn(query, key, value)
print(output.shape) # 输出:torch.Size([2, 5, 32])
```
以上便是一些常见的不同类型的Attention机制,每种机制有着不同的特点和适用场景,可以根据具体任务需求选择合适的Attention类型。
# 4. Attention机制的优势与局限
在神经网络中,Attention机制作为一种重要的机制,具有许多优势,同时也存在一些局限性。
#### 4.1 优势:提高模型性能
Attention机制能够帮助神经网络模型更集中地关注输入数据中与当前任务相关的部分,从而在处理长序列数据或复杂任务时取得更好的性能。通过选取重要的信息进行聚焦,可以减少信息丢失并提高模型的准确性。
另外,Attention机制能够提升模型对不同位置的输入信息的感知,有助于处理变长输入序列,例如在自然语言处理任务中,可以更好地处理不同长度的句子。
#### 4.2 局限:计算复杂度与训练困难
尽管Attention机制在提升模型性能方面具有明显优势,但其计算复杂度较高,需要额外的计算资源和时间。尤其在应用于深层神经网络或大规模数据集时,Attention机制的计算开销会进一步增加,导致训练困难。
此外,Attention机制也容易受到输入序列长度的限制,长序列的处理可能会受到困扰,需要采取一些优化措施来解决这一问题,例如引入Self-Attention机制或多头Attention机制。
综上所述,Attention机制虽然在提高模型性能方面表现突出,但在计算复杂度和训练难度方面也存在一定局限性,需要在实际应用中仔细权衡利弊。
# 5. 注意力机制在计算机视觉中的应用
注意力机制在计算机视觉中也扮演着重要的角色,特别是在图像处理和处理任务中。在这一部分,我们将详细探讨注意力机制在计算机视觉中的具体应用。
### 5.1 图像标注中的Attention机制
在图像标注任务中,我们通常需要让模型生成与图像内容相关的描述。Attention机制可以帮助模型关注图像的不同区域,从而生成更加准确和具体的描述。通过动态地决定每个区域对描述的贡献度,Attention机制提高了图像标注的准确性和连贯性。
下面是一个简单的基于Attention机制的图像标注示例代码:
```python
import torch
import torch.nn as nn
import torchvision.models as models
class ImageCaptioningModel(nn.Module):
def __init__(self, attention):
super(ImageCaptioningModel, self).__init__()
self.cnn_encoder = models.resnet50(pretrained=True)
self.attention = attention
self.lstm_decoder = nn.LSTM(...)
self.fc = nn.Linear(...)
def forward(self, image, caption):
features = self.cnn_encoder(image)
context = self.attention(features, caption)
lstm_output, _ = self.lstm_decoder(context)
outputs = self.fc(lstm_output)
return outputs
```
在上述代码中,我们定义了一个简化的图像标注模型,通过引入Attention机制提高了模型对图像内容的关注度,从而改善了图像标注的效果。
### 5.2 视觉问答任务中的Attention机制
在视觉问答任务中,模型需要同时理解图像内容和问题内容,然后生成准确的答案。Attention机制可以帮助模型动态地选择图像和问题中相关的部分进行关注,从而更好地完成任务。
下面是一个简单的视觉问答任务中的Attention机制示例代码:
```python
import torch
import torch.nn as nn
import torchvision.models as models
class VisualQuestionAnsweringModel(nn.Module):
def __init__(self, attention):
super(VisualQuestionAnsweringModel, self).__init__()
self.cnn_encoder = models.resnet101(pretrained=True)
self.lstm_question_encoder = nn.LSTM(...)
self.attention = attention
self.fc = nn.Linear(...)
def forward(self, image, question):
image_features = self.cnn_encoder(image)
question_features = self.lstm_question_encoder(question)
attention_output = self.attention(image_features, question_features)
outputs = self.fc(attention_output)
return outputs
```
在上述代码中,我们定义了一个简化的视觉问答模型,通过引入Attention机制,模型可以动态地关注图像和问题中相关的信息,从而提高了问题回答的准确性和相关性。
# 6. 未来发展方向与研究趋势
在神经网络中,Attention机制作为一种重要的机制,在未来的发展中有着广阔的应用前景和研究趋势。以下将介绍一些值得关注的未来发展方向:
#### 6.1 强化学习与Attention的结合
强化学习是一种通过试错学习来最大化累积奖励的机制,而Attention机制能够帮助模型关注重要的信息,因此将强化学习与Attention相结合,可以在处理复杂的决策任务中发挥更大的作用。未来可以研究在强化学习中如何更好地利用Attention机制来提高决策性能。
#### 6.2 自适应性Attention机制的探索
当前的Attention机制大多是静态的,即在训练过程中学习得到的权重固定不变。未来的研究可以探索如何使Attention机制更加自适应,根据不同任务和输入调整权重,从而使得模型能够更灵活地应对各种情况,提高性能和泛化能力。
通过不断地探索和创新,Attention机制在神经网络中的应用将会更加广泛,为各种任务带来更大的提升。
0
0
相关推荐
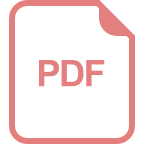
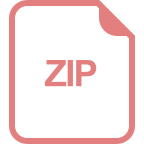
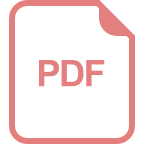





