Exploring Advanced Features of SecureCRT: Scripting and Automated Operations
发布时间: 2024-09-14 20:09:41 阅读量: 41 订阅数: 21 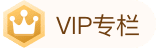
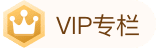
# 1. Introduction to SecureCRT
## 1.1 What is SecureCRT?
SecureCRT is a terminal emulation software developed by VanDyke Software, designed to provide users with a secure and stable solution for remote access. Its powerful features and flexible configurations make it one of the preferred tools for many IT professionals and system administrators.
## 1.2 Advantages of SecureCRT
- Offers robust encryption and authentication features to ensure data security.
- Supports a variety of protocols such as SSH, Telnet, Rlogin, making it widely applicable.
- User-friendly interface with simple and intuitive operations.
- Highly customizable to meet various usage needs.
- Good stability and reliability suitable for critical operations in production environments.
## 1.3 Applications of SecureCRT
SecureCRT is mainly used in the following areas:
***work device management: for managing routers, switches, firewalls, and other network devices.
2. Remote server management: for convenient management and maintenance of remote servers.
3. System administrator tasks: performing system maintenance, daily operations, etc.
4. Software development: debugging and managing code and applications on remote servers.
## Summary
SecureCRT is a powerful terminal emulation software with high security and flexibility, suitable for various network management and system maintenance tasks. Its advantages include strong encryption features, multi-protocol support, a user-friendly interface, and high customizability, making it widely used in network device management, remote server management, system administrator tasks, and software development.
# 2. Basic Operations of SecureCRT
SecureCRT is a commonly used terminal emulation software with extensive applications in daily work. Mastering its basic operations is very important when using SecureCRT for operations. This chapter will introduce the basic operations of SecureCRT, including connecting to remote hosts, password management, and session management.
1. **Connecting to Remote Hosts**:
- Click the "Quick Connect" button on the SecureCRT main interface.
- Enter the IP address, port number, protocol, and other information of the remote host.
- Click the "Connect" button to connect to the remote host.
2. **Password Management**:
- SecureCRT supports saving passwords for remote hosts, facilitating quick login on subsequent occasions.
- Password management options can be set in "Options" -> "Global Options" -> "Passwords".
3. **Session Management**:
- SecureCRT can save session information for different hosts, facilitating user management and switching.
- In "File" -> "Connect" -> "Open", you can view and manage the list of saved sessions.
| Host Name | IP Address | Protocol |
|--------------|----------------------|----------|
| Server1 | ***.***.*.*** | SSH |
| Router1 | **.*.*.* | Telnet |
| Switch1 | ***.**.*.* | SSH |
```python
# Example code: Connecting to a remote host
def connect_remote_host(host, username, password):
session = crt.Session.Connect("/SSH {}/{}".format(host, username))
session.Send(password)
```
:::mermaid
graph LR
A[Enter remote host information] --> B{Correct information?}
B -->|Yes| C[Connect to remote host]
B -->|No| D[Re-enter information]
:::
The above content introduces the basic operations of SecureCRT. By learning these, you can become more proficient in using SecureCRT for remote host operations and management.
# 3. Basics of SecureCRT Script Writing
In this chapter, we will introduce the basics of SecureCRT script writing, including what a script is, script language selection, and a brief introduction to the script editor. By learning the content of this chapter, you will understand how to start writing your own SecureCRT scripts.
1. What is a SecureCRT script:
- A SecureCRT script is a script file used for automating operations and batch processing. Writing scripts can automate operations on remote hosts and improve work efficiency.
2. Script language selection:
- SecureCRT supports various script languages, including VBScript, Python, etc. Users can choose an appropriate scripting language based on their needs and proficiency.
3. Script editor introduction:
- SecureCRT comes with a powerful script editor where users can write, edit, and save script files. The editor provides syntax highlighting, code auto-completion, and other features to facilitate script writing and debugging.
```vbscript
' Example VBScript script
crt.Screen.Send "ls" & vbCr
crt.Screen.WaitForString ">"
crt.Screen.Send "exit" & vbCr
```
| Scripting Language | Support |
|--------------------|---------|
| VBScript | Yes |
| Python | Yes |
| JavaScript | Yes |
**Summary of script writing basics:**
- SecureCRT scripts are used for automating operations and batch processing.
- Supports multiple scripting languages such as VBScript and Python.
- SecureCRT comes with a script editor that offers syntax highlighting and code completion features.
:::mermaid
graph TD;
A(Start)-->B(Script Writing);
B-->C{Successful};
C-->|Yes| D(End);
C-->|No| E(Debug and Modify);
E-->B;
:::
# 4. SecureCRT Script Examples
In this chapter, we will introduce some examples of SecureCRT scripts to help readers better understand how to write and run scripts to achieve automation. We will start with writing simple scripts and gradually delve into how to implement automatic login functions and execute batch operation commands.
1. **Writing a Simple Script Example**
In this example, we will show a simple SecureCRT script that connects to a remote host and outputs a message indicating successful connection.
```python
# Simple Script Example
def connect_to_server(hostname, username, password):
crt.Screen.Synchronous = True
objNewTab = crt.Session.ConnectInTab("/ssh2 /L %s %s" % (username, hostname))
if objNewTab:
crt.Session.CurrentTab.Activate()
crt.Screen.Send(password + '\r')
crt.Screen.WaitForString("login successful", 5)
crt.Dialog.MessageBox('Connected to %s successfully!' % hostname)
else:
crt.Dialog.MessageBox('Connection failed!')
hostname = "***"
username = "user"
password = "password"
connect_to_server(hostname, username, password)
```
2. **Implementing Automatic Login Functionality**
This example demonstrates how to write a script to automatically log into a remote host and execute a series of commands.
```python
# Automatic Login Script Example
def auto_login(hostname, username, password, commands):
crt.Screen.Synchronous = True
objNewTab = crt.Session.ConnectInTab("/ssh2 /L %s %s" % (username, hostname))
if objNewTab:
crt.Session.CurrentTab.Activate()
crt.Screen.Send(password + '\r')
crt.Screen.WaitForString("login successful", 5)
for command in commands:
crt.Screen.Send(command + '\r')
crt.Screen.WaitForString(command, 3)
crt.Dialog.MessageBox('Automatic login and commands execution completed!')
hostname = "***"
username = "user"
password = "password"
commands = ["ls", "cd /path", "pwd"]
auto_login(hostname, username, password, commands)
```
3. **Executing Batch Operation Commands**
This example demonstrates how to write a script to batch execute specified commands and save the results to a file.
```python
# Batch Command Execution Script Example
def batch_command_execution(hostname, username, password, commands, output_file):
crt.Screen.Synchronous = True
objNewTab = crt.Session.ConnectInTab("/ssh2 /L %s %s" % (username, hostname))
if objNewTab:
crt.Session.CurrentTab.Activate()
crt.Screen.Send(password + '\r')
crt.Screen.WaitForString("login successful", 5)
with open(output_file, 'w') as ***
***
*** '\r')
crt.Screen.WaitForString(command, 3)
output = crt.Screen.ReadString(">> ")
file.write(output)
crt.Dialog.MessageBox('Batch command execution completed! Results saved to %s' % output_file)
hostname = "***"
username = "user"
password = "password"
commands = ["ls", "pwd", "ls -l"]
output_file = "command_output.txt"
batch_command_execution(hostname, username, password, commands, output_file)
```
The above is the specific content of Chapter 4, SecureCRT Script Examples. Through these examples, readers can understand how to write different types of scripts to achieve automation. Next, we will continue to explore advanced content of SecureCRT scripts.
# 5. Advanced SecureCRT Scripting
In Chapter 5, we will delve into advanced aspects of SecureCRT scripting, including variables and conditional statements, loop structures, and script debugging techniques.
#### 5.1 Variables and Conditional Statements
In script writing, variables can be used to conveniently store and manipulate data, while conditional statements allow scripts to execute different code blocks based on various conditions. The following is a sample code segment demonstrating how to define variables and use conditional statements:
```python
# Define variables
username = "admin"
password = "password123"
hostname = "***.***.*.*"
# Conditional statement check
if username == "admin" and password == "password123":
crt.Dialog.MessageBox("Login successful")
else:
crt.Dialog.MessageBox("Username or password incorrect")
```
In the above code, we defined variables for the username, password, and hostname, and used a conditional statement to check if the username and password are correct, then display the appropriate prompt box.
#### 5.2 Loop Structures
Loop structures are commonly used control structures in programming that can repeatedly execute a specific block of code. In SecureCRT scripting, we can use loop structures to implement batch operations and other functions. Here is a simple loop example:
```python
# Loop to print numbers
for i in range(5):
crt.Screen.Send("Number: {}\r".format(i))
```
The above code uses a `for` loop to print numbers 0 to 4 and sends them to the terminal using the `crt.Screen.Send` method.
#### 5.3 Script Debugging Techniques
In script writing, debugging is an essential part. SecureCRT provides debugging tools to help us locate and solve problems. By adding output statements, using breakpoints, and stepping through code, we can better debug scripts. The following is a simple example of debugging techniques:
```python
# Output debugging information
crt.Dialog.MessageBox("Starting script execution")
# Breakpoint debugging
crt.Screen.Send("show version\r")
crt.Screen.WaitForString("Switch")
# Stepping through execution
crt.Screen.Send("show interface status\r")
crt.Screen.WaitForString("GigabitEthernet1/0/1")
```
Through the above examples, we can see how to use output information, breakpoints, and step-by-step execution to debug scripts, helping to troubleshoot problems and optimize code logic.
The following is a simple flowchart showing the process of handling variables and conditional statements:
```mermaid
graph LR
A[Username and password check] -->|Correct| B[Login successful]
A -->|Incorrect| C[Username or password incorrect]
```
The above is the content of Chapter 5 on advanced SecureCRT scripting. By learning about variables and conditional statements, loop structures, and script debugging techniques, we can write more flexible and efficient scripts, enhancing our ability for automated operations.
# 6. Security of SecureCRT Scripts
When using SecureCRT for script writing and automated operations, ensuring the security of the scripts is crucial. The following will discuss the security aspects of SecureCRT scripting in detail.
### 6.1 Script Security Risks
When writing scripts, there are some potential security risks to consider, such as:
- **Clear Text Password Exposure**: Hardcoding passwords in scripts could lead to password leaks.
- **Script File Access Permissions**: Incorrectly set file permissions for script files could allow unauthorized users to view or modify the script content.
- **Malicious Scripts**: Unverified scripts may contain malicious code that could harm the system.
### 6.2 How to Protect Scripts
To protect scripts from security threats, you can take the following measures:
- **Use Encryption Algorithms**: Encrypt sensitive information in scripts to ensure it is not displayed in clear text.
- **Limit Access Permissions**: Set appropriate file permissions to prevent unauthorized users from modifying or viewing script files.
- **Secure Transmission**: When transferring script files, use secure protocols (such as SFTP) to ensure security during the transfer process.
### 6.3 Security Practice Suggestions
To improve the security of SecureCRT scripts, the following suggestions can be followed:
| Security Practice | Description |
| ----------------- | ----------- |
| **Regular Script Reviews** | Regularly check script content to ensure it still meets security standards. |
| **Use Secure Connections** | Ensure SecureCRT connections use secure encrypted connections, such as SSH. |
| **Sensitive Information Handling** | Avoid storing sensitive information such as passwords in clear text in scripts; consider using password management tools. |
| **Prevent Injection Attacks** | When executing commands in scripts, filter user input to prevent injection attacks. |
### Security Practice Flowchart:
```mermaid
graph TD
A(Review script content) --> B(Use secure connections)
B --> C(Sensitive information handling)
C --> D(Prevent injection attacks)
```
By following the above security practice measures and flowcharts, you can effectively enhance the security of SecureCRT scripts, ensuring that scripts do not become a source of security vulnerabilities during use.
# 7. SecureCRT Automation Operations
### 7.1 Using Scripts to Implement Automation Tasks
In real-world work, we often need to complete repetitive tasks through automated scripts, and SecureCRT offers powerful scripting features to achieve automation. The general steps to implement automation tasks using scripts are as follows:
1. Write the script:
- Use an appropriate scripting language to write the automation script, such as Python, VBScript, etc.
- Create a new script file in SecureCRT and paste the script code into it.
2. Test the script:
- Load and execute the script in SecureCRT to see if it performs as expected.
3. Debug the script:
- If there are issues with script execution, use the debugging tools provided by SecureCRT to step through the script line by line to locate the problem.
4. Schedule task execution:
- Use the scheduled task functionality provided by the operating system to set up scheduled script execution, achieving automation of scheduled tasks.
Below is a Python script example that demonstrates the functionality of automatically connecting to a host and executing commands in SecureCRT:
```python
# Import the SecureCRT module
import SecureCRT
# Create a SecureCRT object
crt = SecureCRT.Session()
# Connect to the remote host
crt.Connect("hostname", "username", "password")
# Send commands
crt.Send("show version")
# Wait for command execution to complete
crt.WaitForString(">")
```
### 7.2 Script Scheduled Execution
Besides manual script execution, we can also schedule SecureCRT scripts to execute automatically using the operating system's scheduled task feature. The steps to implement script scheduled execution are as follows:
1. Create a scheduled task:
- Set up a scheduled task in the operating system to periodically execute SecureCRT scripts.
2. Configure task parameters:
- Set the execution time and frequency of the scheduled task.
3. Execute the task:
- Allow the system to execute the SecureCRT script at the scheduled time, achieving automated scheduled operations.
### 7.3 Remote Batch Execution of Scripts
For remote management of multiple hosts, we can implement the batch execution of scripts through SecureCRT to improve work efficiency. The general steps for remote batch execution of scripts are as follows:
1. Write the batch execution script:
- Write a script that can loop through multiple hosts and execute commands.
2. Execute the batch script:
- Load and execute the batch execution script in SecureCRT, which will automatically connect to each host to perform the same operations.
Through the above methods, we can achieve unified management and operation of multiple hosts, improving work efficiency.
Below is a mermaid-formatted flowchart that shows the process of remote batch script execution:
```mermaid
graph LR
A[Write batch execution script] --> B[Execute batch script]
B --> C{Operation successful?}
C -- Yes --> D[Record execution results]
C -- No --> E[Debug the script]
```
0
0
相关推荐
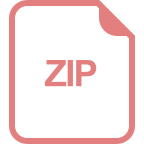
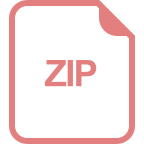





